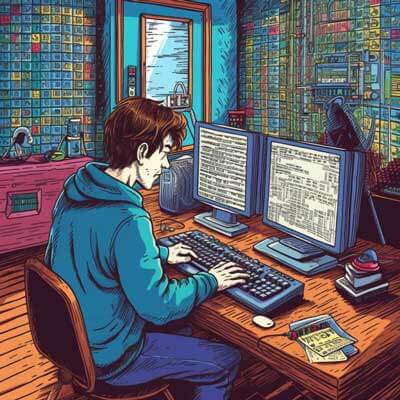
- Debugging Techniques for Efficient JavaScript Development
- Console.log
- Debugger Statement
- Essential Debugging Tools for JavaScript
- Chrome DevTools
- Breakpoints
- Step-by-Step Execution
- Best Practices for Effective JavaScript Debugging
- 1. Understand the Expected Behavior
- 2. Reproduce the Issue
- 3. Start with the Simplest Case
- 4. Use Version Control
- 5. Document Your Findings
- Helpful Tips for Debugging JavaScript Code
- 1. Use the Console API
- 2. Inspect Objects and Arrays
- 3. Use Conditional Breakpoints
- Leveraging Debugger Statements in JavaScript
- The debugger Statement
- The console.log Statement
- The console.error Statement
- Understanding JavaScript Breakpoints
- Setting Breakpoints in Chrome DevTools
- Common Use Cases for Breakpoints
- Step-by-Step Guide to Stepping Through Code in JavaScript
- 1. Open Chrome DevTools
- 2. Navigate to the “Sources” Tab
- 3. Set a Breakpoint
- 4. Start Debugging
- 5. Step Through the Code
- Monitoring Variables with JavaScript During Debugging
- The Scope Panel
- Modifying Variables in the Console
- Troubleshooting Issues in getInitialProps with Next.js
- 1. Data Not Loading
- 2. Infinite Loop
- 3. Performance Issues
- 4. Error Handling
- Additional Resources
Next.js is a popular framework for building server-side rendered React applications. One of its key features is the ability to fetch data and pre-render pages on the server using the getInitialProps
function. This function allows you to fetch data from APIs, databases, or any other data source and pass it as props to your React components.
However, debugging the getInitialProps
function can be challenging, as it runs on the server and not in the browser. In this article, we will explore how to effectively use the debugger inside the getInitialProps
function in Next.js to troubleshoot issues and improve the performance of your application.
Debugging Techniques for Efficient JavaScript Development
Debugging is an essential skill for every software developer. It allows you to identify and fix issues in your code, improve performance, and optimize your application. In this section, we will discuss some debugging techniques that can help you become a more efficient JavaScript developer.
Related Article: Using the JavaScript Fetch API for Data Retrieval
Console.log
The simplest and most common way to debug JavaScript code is by using the console.log
function. This function allows you to print values and messages to the browser console, which can help you understand the flow of your code and identify any issues.
Here’s an example of how you can use console.log
to debug the getInitialProps
function in Next.js:
async function getInitialProps() { console.log('Fetching data...'); const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log('Data fetched:', data); return { data }; }
In this example, we use console.log
to print a message before fetching the data and another message after the data is fetched. This can help us understand the timing and order of the function’s execution.
Debugger Statement
Another useful debugging technique is using the debugger
statement. This statement allows you to pause the execution of your code and inspect the state of your variables and objects.
To use the debugger
statement in the getInitialProps
function, simply add the statement where you want the code execution to pause:
async function getInitialProps() { debugger; const response = await fetch('https://api.example.com/data'); const data = await response.json(); return { data }; }
When the code reaches the debugger
statement, it will pause execution and open the browser’s developer tools. From there, you can inspect the variables, step through the code, and analyze the state of your application.
Essential Debugging Tools for JavaScript
In addition to the basic debugging techniques mentioned earlier, there are several useful tools that can greatly enhance your debugging experience in JavaScript. Let’s explore some of these tools:
Related Article: Understanding JavaScript Execution Context and Hoisting
Chrome DevTools
Chrome DevTools is a set of web developer tools built directly into the Google Chrome browser. It provides a comprehensive suite of debugging and profiling tools that can help you diagnose and fix issues in your JavaScript code.
To access Chrome DevTools, simply right-click on a web page and select “Inspect” from the context menu. This will open the DevTools panel, where you can navigate to the “Sources” tab to debug your code.
Breakpoints
One of the most useful features of Chrome DevTools is the ability to set breakpoints in your code. A breakpoint is a specific line of code where you want the execution to pause so that you can inspect the state of your application.
To set a breakpoint in Chrome DevTools, simply click on the line number in the “Sources” tab. When the code execution reaches that line, it will pause and you can examine the variables and step through the code.
Step-by-Step Execution
Chrome DevTools also allows you to step through your code line by line, which can be extremely helpful for understanding the flow of your code and identifying any issues.
You can use the following shortcuts to step through your code:
– F10: Step over (execute the current line and move to the next line)
– F11: Step into (if the current line contains a function call, jump into the function)
– Shift + F11: Step out (if the current line is inside a function, jump out of the function)
Related Article: Tutorial: Role of JavaScript in AJAX Technology
Best Practices for Effective JavaScript Debugging
Effective debugging requires a systematic approach and adherence to best practices. By following these best practices, you can save time and effort in identifying and fixing issues in your JavaScript code.
1. Understand the Expected Behavior
Before diving into debugging, it’s important to have a clear understanding of the expected behavior of your code. This includes understanding the requirements, specifications, and use cases of your application.
2. Reproduce the Issue
To effectively debug an issue, you need to be able to reproduce it consistently. This means having a set of steps or inputs that reliably trigger the issue.
Once you can reproduce the issue, you can isolate it and focus your debugging efforts on the relevant code. This can save you time and prevent you from getting lost in unrelated parts of your codebase.
Related Article: Top Algorithms Implemented in JavaScript
3. Start with the Simplest Case
When debugging, it’s often helpful to start with the simplest case that exhibits the issue. This allows you to eliminate any unnecessary complexity and focus on the core problem.
4. Use Version Control
Version control systems, such as Git, can be invaluable when debugging complex issues. By using version control, you can easily revert to a previous working state of your code and compare it with the current state to identify any changes that may have introduced the issue.
Version control also allows you to create branches and experiment with different solutions without risking the stability of your codebase.
5. Document Your Findings
As you debug an issue, it’s important to document your findings along the way. This includes recording the steps you’ve taken, the code you’ve modified, and any insights or hypotheses you’ve formed.
Documenting your findings not only helps you keep track of your progress but also serves as a valuable resource for future debugging sessions or for sharing your knowledge with others.
Related Article: The Most Common JavaScript Errors and How to Fix Them
Helpful Tips for Debugging JavaScript Code
In addition to the best practices mentioned earlier, here are some helpful tips that can further improve your debugging process:
1. Use the Console API
The Console API provides a wide range of methods that can help you debug your JavaScript code. In addition to console.log
, you can use methods like console.error
, console.warn
, and console.info
to log specific types of messages.
You can also use the %c
format specifier to apply CSS styles to your console messages, making them more visually distinct and easier to differentiate.
Here’s an example of using the Console API to log an error message:
console.error('An error occurred:', error);
2. Inspect Objects and Arrays
When debugging JavaScript code, it’s common to encounter complex objects and arrays. The Console API provides methods like console.dir
and console.table
that can help you inspect these data structures in a structured and readable format.
For example, you can use console.table
to display an array of objects as a table:
const users = [ { id: 1, name: 'John Doe', age: 30 }, { id: 2, name: 'Jane Smith', age: 25 }, { id: 3, name: 'Bob Johnson', age: 35 } ]; console.table(users);
Related Article: Sharing State Between Two Components in React JavaScript
3. Use Conditional Breakpoints
Conditional breakpoints allow you to pause the execution of your code only when a specific condition is met. This can be extremely useful when debugging loops or complex conditions.
To set a conditional breakpoint in Chrome DevTools, right-click on a line of code, select “Add conditional breakpoint”, and enter the condition in the input field.
For example, you can set a conditional breakpoint to pause the execution only when a variable reaches a certain value:
for (let i = 0; i < 10; i++) { // Pause only when i is equal to 5 if (i === 5) { debugger; } console.log(i); }
Leveraging Debugger Statements in JavaScript
In addition to using the debugger
statement, JavaScript provides several other debugging statements that can be useful in different scenarios.
The debugger Statement
The debugger
statement is a useful tool that allows you to pause the execution of your code and inspect the state of your variables and objects. It can be used in both browser-based JavaScript and server-side JavaScript environments.
To use the debugger
statement, simply add it in your code where you want to pause the execution:
function myFunction() { debugger; // Code to be debugged }
When the code reaches the debugger
statement, it will pause execution and open the debugger of your chosen environment, allowing you to inspect variables, step through code, and analyze the state of your application.
Related Article: Server-side rendering (SSR) Basics in Next.js
The console.log Statement
The console.log
statement is a simple yet effective way to debug JavaScript code. It allows you to print values and messages to the browser console or server logs, providing insights into the flow of your code and helping you identify issues.
To use the console.log
statement, simply pass the value or message you want to log:
function myFunction() { console.log('Value:', value); // Code to be debugged }
In this example, the value of the value
variable will be logged to the console, giving you visibility into its current state.
The console.error Statement
The console.error
statement is similar to console.log
, but it is specifically designed for logging error messages. When an error is logged using console.error
, it is visually distinct in the console and often accompanied by a stack trace.
To use the console.error
statement, simply pass the error message you want to log:
function myFunction() { console.error('An error occurred:', error); // Code to be debugged }
In this example, the error message and the associated error object will be logged to the console, making it easier to identify and track down the cause of the error.
Understanding JavaScript Breakpoints
Breakpoints are an essential tool in the debugging process. They allow you to pause the execution of your code at a specific line or condition, giving you the opportunity to inspect the state of your application and identify any issues.
When a breakpoint is encountered, the code execution will pause and control will be transferred to the debugger. From there, you can step through the code, examine variables, and analyze the state of your application.
Related Article: Resolving Declaration File Issue for Vue-Table-Component
Setting Breakpoints in Chrome DevTools
In Chrome DevTools, you can set breakpoints by clicking on the line number in the “Sources” tab. When the code execution reaches that line, it will pause and the debugger will be activated.
You can also set breakpoints conditionally by right-clicking on a line of code, selecting “Add conditional breakpoint”, and entering the condition.
Once a breakpoint is set, you can step through the code using the debugger controls or inspect variables and objects in the “Scope” panel.
Common Use Cases for Breakpoints
Breakpoints can be used in a variety of scenarios to aid in debugging. Here are some common use cases:
– Debugging loops: Set a breakpoint inside a loop to pause execution at each iteration and inspect the state of the loop variables.
– Investigating conditions: Set a conditional breakpoint to pause execution only when a specific condition is met, allowing you to inspect the state of the application at that point.
– Analyzing function calls: Set a breakpoint inside a function to pause execution when the function is called, allowing you to trace the flow of your code and inspect the arguments and return values of the function.
Step-by-Step Guide to Stepping Through Code in JavaScript
Stepping through code is a valuable technique for understanding the flow of your code and identifying any issues. In this section, we will provide a step-by-step guide on how to step through your JavaScript code using Chrome DevTools.
Related Article: Quick Intro on JavaScript Objects
1. Open Chrome DevTools
To start stepping through your code, open Chrome DevTools by right-clicking on a web page and selecting “Inspect” from the context menu. This will open the DevTools panel.
2. Navigate to the “Sources” Tab
In the DevTools panel, navigate to the “Sources” tab. This tab contains the source code of your application, and it is where you will be able to set breakpoints and step through your code.
3. Set a Breakpoint
To set a breakpoint, click on the line number in the “Sources” tab where you want the code execution to pause. A blue marker will appear on the line, indicating that a breakpoint has been set.
Related Article: Overriding Document in Next.js
4. Start Debugging
Once you have set a breakpoint, refresh the web page or trigger the code that you want to debug. When the code execution reaches the line with the breakpoint, it will pause, and the debugger will be activated.
5. Step Through the Code
With the debugger activated, you can now step through the code using the debugger controls. The available controls are:
– Step over (F10): Executes the current line and moves to the next line.
– Step into (F11): If the current line contains a function call, it will jump into the function and pause at the first line of the function.
– Step out (Shift + F11): If the debugger is currently inside a function, it will jump out of the function and pause at the line after the function call.
Monitoring Variables with JavaScript During Debugging
When debugging JavaScript code, it’s often crucial to monitor the state of variables and objects to understand how they change over time and identify any issues. In this section, we will explore how you can monitor variables using Chrome DevTools.
Related Article: Optimal Practices for Every JavaScript Component
The Scope Panel
Chrome DevTools provides a “Scope” panel that allows you to monitor the state of variables and objects during debugging. This panel displays the variables and objects in the current scope, providing insights into their current values and allowing you to track changes.
To access the “Scope” panel, open Chrome DevTools and navigate to the “Sources” tab. In the panel on the right-hand side, you will find the “Scope” panel.
In the “Scope” panel, you will see a list of variables and objects available in the current scope. You can expand each variable or object to view its properties and their values.
Modifying Variables in the Console
In addition to monitoring variables in the “Scope” panel, you can also modify their values directly in the Chrome DevTools console. This can be helpful for experimenting with different values or fixing issues on the fly.
To modify a variable, simply type its name in the console and assign a new value to it:
let count = 0; console.log(count); // Outputs 0 count = 10; console.log(count); // Outputs 10
In this example, we modify the value of the count
variable from 0 to 10 and log the updated value to the console.
Troubleshooting Issues in getInitialProps with Next.js
The getInitialProps
function in Next.js is a useful tool for fetching data and pre-rendering pages on the server. However, it can sometimes be challenging to troubleshoot issues that arise when using this function.
In this section, we will discuss some common issues that you may encounter when using getInitialProps
in Next.js and provide troubleshooting techniques to help you resolve them.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
1. Data Not Loading
If the data is not loading as expected in the getInitialProps
function, there are several potential causes to consider:
– Check the API endpoint: Ensure that the API endpoint you are fetching data from is correct and accessible. You can use tools like Postman or cURL to test the API endpoint independently of Next.js.
– Verify the data format: Make sure that the data returned by the API is in the expected format. You can use console.log
or console.dir
to inspect the data and verify its structure.
– Check for errors: Use console.error
or console.log
to check for any errors that may be occurring during the data fetching process. Ensure that error handling is in place and that any error responses from the API are properly handled.
2. Infinite Loop
An infinite loop can occur in the getInitialProps
function if the logic within the function triggers a re-render of the component, which in turn triggers another call to getInitialProps
. This can result in an endless loop.
To troubleshoot an infinite loop issue, consider the following:
– Check for component re-render: Verify if the component is being re-rendered unnecessarily, which may trigger multiple calls to getInitialProps
. Ensure that the component’s dependencies are properly set and that any state changes are handled correctly.
– Debug the component lifecycle: Use console.log
or debugger
statements to inspect the component lifecycle and identify any unexpected re-renders or calls to getInitialProps
.
– Review the logic within getInitialProps
: Analyze the code inside the getInitialProps
function to identify any potential issues that may lead to an infinite loop. Make sure that any conditions or branches within the function are properly handled and do not result in an endless loop.
3. Performance Issues
If you are experiencing performance issues with the getInitialProps
function, it’s important to analyze and optimize the code to improve efficiency. Here are some tips to troubleshoot performance issues:
– Minimize API calls: Avoid making unnecessary API calls within getInitialProps
by caching data or optimizing the data fetching process. Consider using techniques like memoization or server-side caching to reduce the number of API requests.
– Optimize data processing: If the data returned by the API requires processing or transformation, ensure that this is done efficiently. Avoid unnecessary iterations or expensive operations that may impact performance.
– Limit the amount of data fetched: If the amount of data being fetched is large, consider implementing pagination or lazy loading techniques to fetch only the necessary data. This can significantly improve performance, especially for large datasets.
Related Article: Next.js Bundlers Quick Intro
4. Error Handling
Proper error handling is crucial when using getInitialProps
in Next.js. If error handling is not implemented correctly, it can lead to issues such as unhandled promise rejections or unexpected behavior.
To troubleshoot error handling issues, consider the following:
– Check for unhandled promise rejections: Unhandled promise rejections can occur if an error is thrown within the getInitialProps
function but not properly caught or handled. Use console.error
or console.log
statements to identify any unhandled promise rejections and ensure that they are properly handled.
– Validate error responses: Ensure that error responses from the API are properly validated and handled within the getInitialProps
function. Use conditional statements or try-catch blocks to catch and handle errors appropriately.
– Test error scenarios: Test the getInitialProps
function with different error scenarios to ensure that errors are handled correctly. Mock the API responses or introduce deliberate errors to verify that the error handling logic is working as expected.