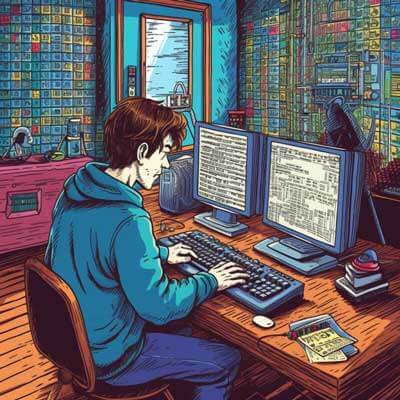
Table of Contents
To convert an array to JSON in JavaScript, you can use the built-in JSON object. The JSON object provides two methods: JSON.stringify()
and JSON.parse()
.
Using JSON.stringify()
The JSON.stringify()
method converts a JavaScript object or value to a JSON string. To convert an array to JSON, you can pass the array as an argument to the JSON.stringify()
method. Here's an example:
const array = ['apple', 'banana', 'orange']; const jsonString = JSON.stringify(array); console.log(jsonString); // Output: ["apple","banana","orange"]
In the example above, the JSON.stringify()
method is called with the array
as the argument. The method returns a JSON string representation of the array. The JSON string can then be used for various purposes, such as sending data to a server or storing it in a file.
Related Article: Quick Intro on JavaScript Objects
Using JSON.parse()
The JSON.parse()
method parses a JSON string and returns a JavaScript object or value. To convert a JSON string back to an array, you can pass the JSON string as an argument to the JSON.parse()
method. Here's an example:
const jsonString = '["apple","banana","orange"]'; const array = JSON.parse(jsonString); console.log(array); // Output: ['apple', 'banana', 'orange']
In the example above, the JSON.parse()
method is called with the jsonString
as the argument. The method returns a JavaScript array representing the JSON string.
Best Practices
When converting an array to JSON in JavaScript, it is important to keep the following best practices in mind:
1. Ensure that the array contains only JSON-serializable values: JSON supports a limited set of data types, including strings, numbers, booleans, null, objects, and arrays. If the array contains values of unsupported data types, such as functions or undefined, an error will occur.
2. Handle circular references: If the array contains circular references (references to the same object multiple times), the JSON.stringify()
method will throw an error. To handle circular references, you can use a third-party library or implement custom logic to handle the serialization.
3. Use the replacer
parameter: The JSON.stringify()
method accepts a second parameter called replacer
, which allows you to customize the serialization process by specifying a function or an array of properties to include or exclude. This can be useful when you want to exclude certain properties or convert them to a different format during serialization.
4. Use the space
parameter for pretty-printing: The JSON.stringify()
method accepts a third parameter called space
, which allows you to specify the indentation level for pretty-printing the JSON string. This can be useful for human-readable output or debugging purposes.
Alternative Ideas
Apart from using the JSON.stringify()
and JSON.parse()
methods, there are alternative ways to convert an array to JSON in JavaScript. Here are a few alternatives:
1. Using the toString()
method: You can convert an array to a comma-separated string using the toString()
method and then manually wrap it with square brackets to create a valid JSON string. However, this approach has limitations as it does not handle nested arrays or objects.
const array = ['apple', 'banana', 'orange']; const jsonString = '[' + array.toString() + ']'; console.log(jsonString); // Output: ["apple","banana","orange"]
2. Using the join()
method: Similar to the toString()
method, you can use the join()
method to concatenate the elements of the array with commas and manually wrap it with square brackets. However, this approach also has limitations and does not handle nested arrays or objects.
const array = ['apple', 'banana', 'orange']; const jsonString = '[' + array.join(',') + ']'; console.log(jsonString); // Output: ["apple","banana","orange"]
3. Using third-party libraries: There are several third-party libraries available that provide additional functionality and flexibility for working with JSON in JavaScript. These libraries often offer advanced features such as handling circular references, preserving the order of object properties, and supporting custom serializers and deserializers.
Some popular third-party libraries for JSON manipulation in JavaScript include:
- JSON-js
- lodash
- ramda
These libraries can be useful when you require more advanced JSON manipulation capabilities or need to work with complex data structures.