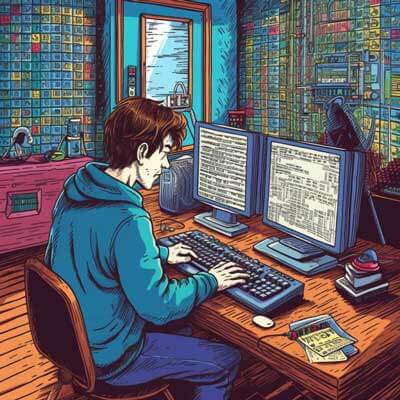
- Component-Based Development: Overview
- Example 1: Creating a Simple React Component
- Example 2: Creating a Vue Component
- JavaScript Frameworks That Support Component-Based Development
- React
- Angular
- Vue.js
- Web Components in JavaScript
- Example 1: Creating a Custom Element
- Example 2: Using Shadow DOM
- React: A Deep Dive into Component Handling
- Component Creation
- Component Composition
- Component Props
- Exploring Popular JavaScript Frameworks for Building Components
- Ember.js
- Backbone.js
- Stencil.js
- The Component Lifecycle in JavaScript
- Mounting
- Updating
- Unmounting
- Passing Props to Components in JavaScript
- Example 1: Passing Props in React
- Example 2: Default Props in React
- Demystifying Component State in JavaScript
- Class Components and State
- Functional Components and Hooks
- How Component Rendering Works in JavaScript
- React Rendering
- Vue Rendering
- Additional Resources
Component-Based Development: Overview
Component-based development is a software development paradigm that focuses on building software systems by breaking them down into smaller, reusable, and independent components. These components can be combined to create complex applications, providing modularity, reusability, and maintainability.
In JavaScript, component-based development has gained significant popularity, thanks to the rise of modern frameworks like React, Angular, and Vue.js. These frameworks provide developers with useful tools and patterns to create and manage components effectively.
The key benefits of component-based development are:
1. Reusability: Components can be reused across different parts of an application or even in multiple applications, reducing duplication of code and effort.
2. Modularity: Components encapsulate their logic, styles, and dependencies, making it easier to understand, test, and maintain them.
3. Scalability: By breaking down an application into smaller components, it becomes easier to scale and extend functionalities without affecting the entire system.
4. Collaboration: Teams can work on different components independently, promoting parallel development and collaboration.
5. Maintainability: Components with well-defined responsibilities and clear interfaces are easier to maintain and debug.
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
Example 1: Creating a Simple React Component
import React from 'react'; const Button = ({ onClick, label }) => { return ( <button> {label} </button> ); }; export default Button;
In this example, we create a simple React component called Button
. It accepts two props, onClick
and label
, and renders a button element with the provided label. This component can be reused throughout the application.
Example 2: Creating a Vue Component
<button> {{ label }} </button> export default { props: ['onClick', 'label'] } button { /* Styles specific to this component */ }
Here, we create a Vue component with similar functionality as the React component. The template defines the structure of the component, the script section handles the props, and the style section contains component-specific styles.
JavaScript Frameworks That Support Component-Based Development
Several JavaScript frameworks provide robust support for component-based development. Let’s explore some of the popular ones:
Related Article: Accessing Parent State from Child Components in Next.js
React
React is a widely used JavaScript library for building user interfaces. It introduced the concept of virtual DOM and popularized the idea of component-based development. React follows a unidirectional data flow and provides a rich set of tools and patterns for building reusable components.
Angular
Angular is a full-fledged JavaScript framework developed by Google. It uses TypeScript and provides a comprehensive set of features for building complex web applications. Angular embraces component-based development and offers a useful component architecture, dependency injection, and a rich ecosystem of libraries and tools.
Vue.js
Vue.js is a progressive JavaScript framework that focuses on simplicity and ease of use. It offers a gentle learning curve and provides a flexible and intuitive approach to building web interfaces. Vue.js promotes component-based development and provides a rich set of features like single-file components, reactivity, and a vibrant ecosystem.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
Web Components in JavaScript
Web Components are a set of web platform APIs that allow developers to create reusable custom elements with their own encapsulated styles and behaviors. They are built using a combination of HTML, CSS, and JavaScript, and can be used in any web application regardless of the underlying framework or library.
Web Components consist of three main specifications:
1. Custom Elements: Custom Elements allow developers to define their own HTML elements with custom behavior and rendering. These elements can be used and reused across different web applications.
2. Shadow DOM: Shadow DOM provides encapsulation for the styles and structure of a web component. It ensures that the styles defined within a component do not affect the rest of the page, and vice versa.
3. HTML Templates: HTML Templates allow developers to define reusable chunks of markup that can be cloned and inserted into the DOM. They provide a way to define a structure without rendering it immediately.
Example 1: Creating a Custom Element
class MyComponent extends HTMLElement { connectedCallback() { this.innerHTML = '<p>Hello, World!</p>'; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called my-component
using the customElements
API. When the element is connected to the DOM, the connectedCallback
method is called, and we set the inner HTML of the element to display “Hello, World!”.
Example 2: Using Shadow DOM
class MyComponent extends HTMLElement { constructor() { super(); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.innerHTML = ` p { color: blue; } <p>Hello, World!</p> `; } } customElements.define('my-component', MyComponent);
In this example, we use the attachShadow
method to attach a shadow DOM to our custom element. We define the styles and markup within the shadow DOM, ensuring that they are encapsulated and do not affect the rest of the page.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
React: A Deep Dive into Component Handling
React provides a useful and flexible component model that allows developers to create reusable and interactive user interfaces. Let’s dive deeper into how React handles components.
Component Creation
In React, components can be created using either functional components or class components.
Functional components are defined as plain JavaScript functions that accept props as an argument and return JSX (a syntax extension for JavaScript that allows HTML-like code) to describe the component’s structure.
const MyComponent = (props) => { return <p>Hello, {props.name}!</p>; };
Class components are defined as ES6 classes that extend the React.Component
class. They must implement a render
method that returns the component’s structure.
class MyComponent extends React.Component { render() { return <p>Hello, {this.props.name}!</p>; } }
Component Composition
React supports component composition, allowing developers to combine multiple components to create more complex user interfaces. This is achieved by including one component within another component’s JSX.
const App = () => { return ( <div> <Header /> <Footer /> </div> ); };
In this example, the App
component includes the Header
, Content
, and Footer
components to create a complete page layout.
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
Component Props
Props are used to pass data from a parent component to its child components. They are read-only and should not be modified within the child component. Props are passed as attributes to the child component in JSX.
const ParentComponent = () => { return ; }; const ChildComponent = (props) => { return ( <div> <p>Name: {props.name}</p> <p>Age: {props.age}</p> </div> ); };
In this example, the ParentComponent
passes the name
and age
props to the ChildComponent
, which then displays them in its JSX.
Exploring Popular JavaScript Frameworks for Building Components
Apart from React, Angular, and Vue.js, there are several other JavaScript frameworks and libraries that provide support for building components. Let’s explore a few of them:
Ember.js
Ember.js is a JavaScript framework that focuses on productivity and convention over configuration. It provides a useful and opinionated approach to building web applications, including a component-based architecture. Ember.js leverages the concept of computed properties and observers to handle complex component dependencies.
Related Article: AngularJS: Check if a Field Contains a MatFormFieldControl
Backbone.js
Backbone.js is a lightweight JavaScript library that provides structure to web applications. While it does not have native support for components, it allows developers to create reusable views and provides tools for managing events, data, and synchronization.
Stencil.js
Stencil.js is a compiler for building fast and efficient web components. It is framework-agnostic and can be used with any JavaScript framework or library. Stencil.js focuses on performance and provides features like lazy-loading, server-side rendering, and automatic code splitting.
The Component Lifecycle in JavaScript
The component lifecycle refers to the different stages a component goes through during its existence. Understanding the component lifecycle is crucial for managing component state, handling side effects, and optimizing performance. Let’s explore the component lifecycle in JavaScript.
Related Article: Big Data Processing with Node.js and Apache Kafka
Mounting
The mounting phase occurs when a component is being initialized and inserted into the DOM. The following methods are called during the mounting phase:
– constructor
: The constructor is called when the component is first created. It is used to initialize component state and bind event handlers.
– render
: The render method is responsible for returning the JSX that represents the component’s structure. It is called whenever the component needs to be rendered.
– componentDidMount
: This method is called immediately after the component has been inserted into the DOM. It is often used to fetch data from APIs, set up subscriptions, or initialize third-party libraries.
Updating
The updating phase occurs when a component’s props or state change. The following methods are called during the updating phase:
– shouldComponentUpdate
: This method determines whether the component should update or not. It is called before the component is re-rendered. By default, it returns true
, but it can be overridden to optimize performance by preventing unnecessary re-renders.
– render
: The render method is called again to update the component’s structure based on the new props or state.
– componentDidUpdate
: This method is called after the component has been re-rendered. It is often used for performing side effects, such as updating the DOM or making additional API calls.
Unmounting
The unmounting phase occurs when a component is being removed from the DOM. The following method is called during the unmounting phase:
– componentWillUnmount
: This method is called just before the component is removed from the DOM. It is often used to clean up any resources or subscriptions created during the component’s lifecycle.
Related Article: Conditional Flow in JavaScript: Understand the 'if else' and 'else if' Syntax and More
Passing Props to Components in JavaScript
Passing props to components is an essential aspect of component-based development. It allows data to be passed from parent components to child components, enabling component composition and reusability. Here are some best practices for passing props in JavaScript.
1. Use descriptive prop names: Choose prop names that clearly describe the data being passed. This makes the code more readable and self-explanatory.
2. Avoid excessive prop drilling: Prop drilling occurs when props have to be passed through multiple levels of components. To avoid this, consider using state management libraries like Redux or context APIs like React’s Context API.
3. Use default props: Specify default values for props using default assignments or the defaultProps
static property in React. This ensures that components can still function correctly even if certain props are not provided.
4. Destructure props: Destructuring props allows for cleaner and more concise code. Instead of accessing props through this.props
or props
, destructure them in the component’s function signature or within the component’s body.
5. Avoid mutating props: Props should be treated as read-only and should not be mutated within the component. Instead, use props to drive the component’s behavior and manage state separately if needed.
Example 1: Passing Props in React
const ParentComponent = () => { const name = "John"; const age = 25; return ; }; const ChildComponent = ({ name, age }) => { return ( <div> <p>Name: {name}</p> <p>Age: {age}</p> </div> ); };
In this example, the ParentComponent
passes the name
and age
props to the ChildComponent
. The props are then accessed and displayed within the ChildComponent
using destructuring.
Example 2: Default Props in React
const Button = ({ label }) => { return <button>{label}</button>; }; Button.defaultProps = { label: "Click me" };
In this example, the Button
component defines a default prop label
with the value “Click me”. If the prop is not provided when using the Button
component, it will default to “Click me”.
Related Article: Creating Dynamic and Static Pages with Next.js
Demystifying Component State in JavaScript
Component state is a crucial concept in JavaScript component-based development. It allows components to manage and track internal data that can change over time. Let’s demystify component state and explore how it works in JavaScript.
Class Components and State
In class components, state is managed using the this.state
object. The state
object holds the component’s internal data, and when the state changes, React triggers a re-render to reflect the updated state.
State should be initialized in the component’s constructor
using this.state = initialState
. To update the state, use the this.setState
method, which merges the new state with the existing state.
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button> this.increment()}>Increment</button> </div> ); } }
In this example, the Counter
component maintains a count
state that is initially set to 0. When the button is clicked, the increment
method is called, updating the state and triggering a re-render.
Functional Components and Hooks
Functional components, introduced in React 16.8, can also manage state using hooks. Hooks are functions that allow functional components to use React features like state and lifecycle methods.
The useState
hook is used to define and manage state within a functional component. It returns an array with two elements: the current state value and a function to update the state.
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); };
In this example, the Counter
component uses the useState
hook to define the count
state with an initial value of 0. The increment
function updates the state using the setCount
function returned by useState
.
Related Article: Creating Web Workers with NextJS in JavaScript
How Component Rendering Works in JavaScript
Component rendering is the process of generating the actual HTML output for a component. It involves evaluating the component’s JSX or template and converting it into a format that the browser can understand. Let’s explore how component rendering works in JavaScript.
React Rendering
In React, rendering is triggered by changes in the component’s state or props. When a component is rendered, React generates a virtual DOM, a lightweight representation of the actual DOM. React then compares the previous virtual DOM with the new one to determine the minimal set of changes needed to update the actual DOM.
React uses a process called reconciliation to efficiently update the DOM. It compares the previous and new virtual DOM trees and performs the necessary updates, such as adding, removing, or updating DOM elements.
class MyComponent extends React.Component { render() { return <p>Hello, World!</p>; } } ReactDOM.render(, document.getElementById('root'));
In this example, the render
method of the MyComponent
class returns a JSX element <p>Hello, World!</p>
. React takes this JSX and converts it into a virtual DOM representation. Finally, React renders the component by updating the actual DOM with the changes determined by the reconciliation process.
Vue Rendering
Vue.js follows a similar rendering process as React. When a component is rendered in Vue, it generates a virtual DOM, known as the vnode (virtual node), which represents the component’s structure and state. Vue then compares the previous vnode with the new one to determine the minimal set of changes needed to update the actual DOM.
Vue uses a reactive system to track dependencies between components and automatically updates the DOM when the data changes. This allows for efficient rendering and automatic reactivity.
<p>Hello, World!</p> export default { // Component options } /* Component styles */
In this example, the Vue component template contains the HTML structure <p>Hello, World!</p>
. When the component is rendered, Vue generates a vnode based on the template and performs the necessary updates to the actual DOM.
Related Article: Enhancing React Applications with Third-Party Integrations
Additional Resources
– Understanding Props in React
– Angular – Input Properties (Props)
– Angular Lifecycle Hooks – What, When and How