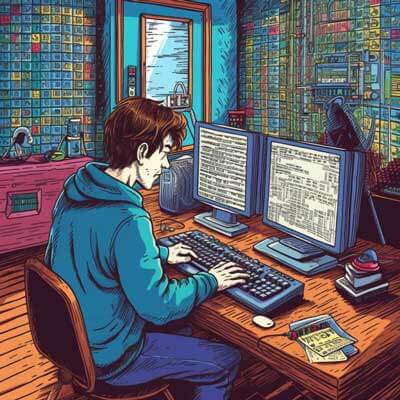
To generate a GUID/UUID (Globally Unique Identifier/Universally Unique Identifier) in JavaScript, you can use the following approaches:
Approach 1: Using the uuid Package
One of the easiest ways to generate a GUID/UUID in JavaScript is by using the uuid
package. This package provides a simple and efficient API for generating universally unique identifiers.
To use the uuid
package, you need to install it first. Open your terminal or command prompt and run the following command:
npm install uuid
Once the package is installed, you can generate a GUID/UUID by importing the v4
function from the uuid
package and calling it. Here’s an example:
const { v4: uuidv4 } = require('uuid'); const guid = uuidv4(); console.log(guid);
This code will generate a new GUID/UUID and log it to the console.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Approach 2: Using the crypto API
If you prefer not to use a third-party package, you can also generate a GUID/UUID using the crypto
API available in modern browsers and Node.js.
Here’s an example of how you can generate a GUID/UUID using the crypto
API in Node.js:
const crypto = require('crypto'); function generateGuid() { return crypto.randomBytes(16).toString('hex'); } const guid = generateGuid(); console.log(guid);
In this code, we use the crypto.randomBytes()
function to generate a buffer of random bytes. We then convert the buffer to a hexadecimal string using the toString('hex')
method.
Best Practices
When generating GUIDs/UUIDs in JavaScript, it’s important to follow some best practices to ensure uniqueness and randomness:
1. Use a reliable method: Instead of implementing your own GUID/UUID generation algorithm, it’s recommended to use established libraries or built-in APIs that have been thoroughly tested and proven to be reliable.
2. Use a version 4 UUID: Version 4 UUIDs are randomly generated and have a very low probability of collisions. They are suitable for most use cases and don’t require any additional information or context.
3. Avoid using sequential or time-based UUIDs: Sequential or time-based UUIDs may leak information about the time or order of creation, which might not be desirable in certain scenarios.
4. Consider the security requirements: If your application requires secure GUID/UUID generation, make sure to use a cryptographically secure random number generator, such as the one provided by the crypto
API.
5. Keep the GUID/UUID generation code separate: It’s a good practice to encapsulate the GUID/UUID generation logic in a separate function or module, making it easier to maintain and test.
Alternative Ideas
Apart from the approaches mentioned above, there are other libraries and techniques available for generating GUIDs/UUIDs in JavaScript. Some popular alternatives include:
– nanoid
: A tiny, secure, and URL-friendly UUID generation library. It generates unique IDs with a customizable length and can be used both in the browser and Node.js. You can find more information and examples in the [nanoid documentation](https://github.com/ai/nanoid).
– Custom implementation: If you have specific requirements or constraints, you can also implement your own GUID/UUID generation algorithm. However, this approach requires a deep understanding of the underlying concepts and careful consideration of the uniqueness and randomness factors.
Related Article: How to Use the forEach Loop with JavaScript