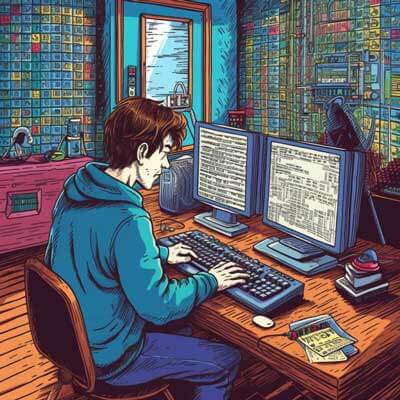
JavaScript plays a critical role in AJAX (Asynchronous JavaScript and XML) technology. It is the primary programming language used to implement the client-side functionality of AJAX applications. In the context of AJAX, JavaScript is responsible for making asynchronous requests to the server, handling responses, and updating the user interface dynamically without requiring a full page reload.
When a user interacts with an AJAX-enabled web application, JavaScript is used to capture the event and initiate an asynchronous request to the server. This request is sent to the server in the background, allowing the user to continue interacting with the application without any interruption. Once the server processes the request and sends back a response, JavaScript is used to handle the response and update the relevant parts of the user interface.
Let’s take a look at a simple example to illustrate the role of JavaScript in AJAX:
// JavaScript code to make an AJAX request // Create a new XMLHttpRequest object var xhr = new XMLHttpRequest(); // Define the callback function to handle the response xhr.onload = function() { if (xhr.status >= 200 && xhr.status < 300) { // Update the UI with the response data document.getElementById("output").innerHTML = xhr.responseText; } else { // Handle errors console.error(xhr.statusText); } }; // Open a GET request to the server xhr.open("GET", "/api/data", true); // Send the request xhr.send();
In this example, JavaScript is used to create a new XMLHttpRequest object, which is the core component of AJAX. The onload
event handler is defined to handle the response from the server. If the response status is in the success range (200-299), the innerHTML
property of an HTML element with the ID "output" is updated with the response text. Otherwise, an error message is logged to the console.
This simple example demonstrates how JavaScript is responsible for making asynchronous requests, handling responses, and updating the UI in an AJAX application.
Understanding AJAX and How It Works
AJAX is a set of web development techniques that allows for asynchronous communication between a client and a server. It enables web applications to retrieve data from the server, send data to the server, and update the user interface without requiring a full page reload.
At the core of AJAX is the XMLHttpRequest object, which provides the ability to make HTTP requests from JavaScript. This object allows developers to send requests to the server, specify the desired HTTP method (e.g., GET, POST, PUT, DELETE), include request headers, and handle the server’s response.
The main idea behind AJAX is to leverage the XMLHttpRequest object to make asynchronous requests, which means that the requests are sent in the background without blocking the user’s interaction with the web application. This asynchronous nature allows for a more responsive and interactive user experience.
When an AJAX request is sent, the server processes the request and sends back a response. The response can be in various formats, such as HTML, XML, JSON, or plain text. Upon receiving the response, JavaScript is used to handle the response data and update the user interface accordingly.
The use of AJAX in web development has revolutionized the way web applications are built. It has enabled developers to create dynamic and interactive web pages that can retrieve and display data from the server without requiring a full page reload. This has greatly improved the user experience and made web applications more responsive.
Related Article: How to Compare Arrays in Javascript
Examining the XMLHttpRequest Object
The XMLHttpRequest object is the cornerstone of AJAX technology. It provides the functionality to send HTTP requests and handle responses asynchronously. Let’s take a closer look at the various properties and methods of the XMLHttpRequest object.
Properties:
– onreadystatechange
: A callback function that is called whenever the readyState
property changes. This allows developers to handle the different stages of the request-response lifecycle.
– readyState
: An integer indicating the current state of the request. It can have the following values:
– 0: UNSENT – The request has not been opened yet.
– 1: OPENED – The request has been opened.
– 2: HEADERS_RECEIVED – The request headers have been received.
– 3: LOADING – The response body is being received.
– 4: DONE – The request has been completed.
– responseText
: The response body as a string.
– status
: The HTTP status code of the response.
– statusText
: The status message corresponding to the HTTP status code.
Methods:
– open(method, url, async)
: Initializes a request. The method
parameter specifies the HTTP method to be used, the url
parameter specifies the URL to which the request is sent, and the async
parameter determines whether the request should be asynchronous (true) or synchronous (false).
– setRequestHeader(header, value)
: Sets a request header with the specified name and value.
– send(data)
: Sends the request. The data
parameter is optional and can be used to send data along with the request, such as form data or JSON payloads.
– abort()
: Cancels the request.
– getAllResponseHeaders()
: Returns all the response headers as a string.
– getResponseHeader(header)
: Returns the value of the specified response header.
Here’s an example that demonstrates the usage of the XMLHttpRequest object:
var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { console.log(xhr.responseText); } }; xhr.open("GET", "/api/data", true); xhr.setRequestHeader("Content-Type", "application/json"); xhr.send();
In this example, a new XMLHttpRequest object is created, and an event listener is attached to the onreadystatechange
event. When the readyState
changes to 4 (DONE) and the status
is 200 (OK), the response is logged to the console. The open
method is used to initialize a GET request to “/api/data”. The setRequestHeader
method is used to set the “Content-Type” header to “application/json”. Finally, the request is sent using the send
method.
The XMLHttpRequest object provides a useful and flexible API for making AJAX requests and handling responses. It is widely supported by modern web browsers and is the foundation of AJAX technology.
The Benefits of Using AJAX in Web Development
AJAX technology has revolutionized web development by enabling developers to create fast, interactive, and dynamic web applications. Here are some of the key benefits of using AJAX:
Improved User Experience:
AJAX allows web applications to update the user interface dynamically without requiring a full page reload. This results in a more responsive and interactive user experience. For example, when submitting a form, instead of reloading the entire page, AJAX can be used to send the form data to the server in the background and update only the relevant parts of the page with the server’s response.
Faster Performance:
Reduced Bandwidth Usage:
AJAX allows for partial updates of web pages, which means that only the necessary data is fetched from the server. This results in reduced bandwidth usage, as there is no need to transfer the entire page content for every request. This is particularly beneficial for users with limited bandwidth or on mobile devices with slower connections.
Seamless User Interaction:
With AJAX, web applications can provide real-time feedback to users. For example, as a user types in a search box, AJAX can be used to send the input to the server and fetch search results in real-time, without the need for manual form submissions or page reloads. This seamless interaction enhances the user experience and makes web applications feel more intuitive and responsive.
Modularity and Reusability:
AJAX allows developers to decouple the server-side logic from the client-side presentation. This separation of concerns enables modularity and reusability, as the server can expose APIs that can be consumed by different client applications, such as web, mobile, or desktop. This allows for code reuse and easier maintenance of the overall system.
Enhanced Interoperability:
AJAX is based on open web standards, such as JavaScript and XML (although JSON is more commonly used today), making it compatible with most modern web browsers. This ensures interoperability across different platforms and devices, enabling web applications to reach a wider audience.
Front-end Development and its Significance
Front-end development refers to the practice of building and maintaining the user interface of a website or web application. It involves writing HTML, CSS, and JavaScript code to create the visual and interactive elements that users see and interact with.
Front-end development is significant because it directly impacts the user experience of a website or web application. It is responsible for creating layouts, designing user interfaces, handling user interactions, and ensuring the overall usability of the application. A well-designed and responsive front-end can greatly enhance the user experience and make the application more engaging and intuitive.
In addition to creating visually appealing interfaces, front-end development also involves ensuring cross-browser compatibility, optimizing performance, and adhering to web standards and best practices. This ensures that the application works seamlessly across different browsers and devices, providing a consistent experience to all users.
Front-end development has evolved significantly over the years, with the introduction of new technologies, frameworks, and tools. It has become more complex and requires a deep understanding of HTML, CSS, JavaScript, and other related technologies. Front-end developers need to stay up-to-date with the latest trends and techniques to build modern, responsive, and user-friendly applications.
Overall, front-end development plays a crucial role in web development, as it is responsible for creating the user interface and ensuring a seamless and enjoyable user experience. It requires a combination of technical skills, creativity, and attention to detail to create visually appealing and functional web applications.
Related Article: How to Create a Countdown Timer with Javascript
The Main Languages Used in Web Development
Web development involves several languages and technologies that work together to create and deliver web applications. The main languages used in web development include HTML, CSS, and JavaScript.
HTML:
HTML (Hypertext Markup Language) is the standard markup language for creating the structure and content of web pages. It defines the elements and tags used to format and organize the content, such as headings, paragraphs, lists, images, and links. HTML provides the basic building blocks for web pages and is the foundation of web development.
CSS:
CSS (Cascading Style Sheets) is a stylesheet language used to describe the presentation and layout of web pages. It allows developers to define styles, such as colors, fonts, spacing, and positioning, to control the visual appearance of HTML elements. CSS enables the separation of content and presentation, making it easier to maintain and update the styling of web pages.
JavaScript:
JavaScript is a useful and versatile programming language that is used to add interactivity and dynamic behavior to web pages. It allows developers to manipulate the DOM (Document Object Model), handle user events, make AJAX requests, perform calculations, and create interactive elements, such as sliders, menus, and forms. JavaScript is essential for creating dynamic and responsive web applications.
In addition to these core languages, web development often involves the use of other technologies and frameworks, such as:
Backend Languages:
Backend languages, such as PHP, Python, Ruby, Java, and .NET, are used to handle server-side logic and interact with databases. They are responsible for processing requests, handling business logic, and generating dynamic content to be displayed on web pages.
Frameworks and Libraries:
Frameworks and libraries, such as React, Angular, Vue.js, and jQuery, provide pre-built components and abstractions that simplify the development of web applications. They offer tools and utilities for managing state, handling routing, creating reusable UI components, and optimizing performance.
Database Technologies:
Database technologies, such as MySQL, PostgreSQL, MongoDB, and Redis, are used to store and retrieve data for web applications. They provide mechanisms for data persistence, querying, and indexing, allowing web applications to store and manipulate large amounts of data efficiently.
Version Control Systems:
Version control systems, such as Git, enable developers to track changes to their codebase, collaborate with others, and manage different versions of their projects. They provide features for branching, merging, and reverting changes, ensuring the stability and integrity of the codebase.
These are some of the main languages and technologies used in web development. The choice of languages and technologies depends on the specific requirements of the project and the preferences of the development team.
Additional Resources
– What is JavaScript used for?
– What is the XMLHttpRequest object?