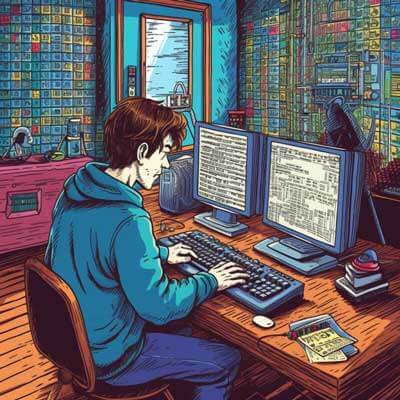
Table of Contents
JavaScript Execution Context: What You Need to Know
JavaScript is a popular programming language that is widely used for creating dynamic web applications. To understand how JavaScript code is executed, it is important to have a good understanding of the concept of execution context.
An execution context can be defined as the environment in which JavaScript code is executed. It consists of two main components: the variable environment and the lexical environment. The variable environment contains all the variables and function declarations that have been defined within the current scope. The lexical environment, on the other hand, maintains a reference to the outer scope, allowing the code to access variables and functions defined in higher-level scopes.
When JavaScript code is executed, an execution context is created for each function call. This execution context is then pushed onto the call stack, which keeps track of the order in which the functions are called. The execution context at the top of the stack is the one that is currently being executed.
One important concept related to execution context is hoisting. Hoisting is a behavior in JavaScript where variable and function declarations are moved to the top of their containing scope during the compilation phase. This means that you can use variables and call functions before they are actually declared in your code.
Let's take a look at an example to understand hoisting better:
console.log(x); // Output: undefined var x = 5;
In this example, even though the variable x
is declared and assigned a value later in the code, the console.log statement does not throw an error. This is because the variable declaration is hoisted to the top of its containing scope. However, the value of x
is not hoisted, so it is still undefined
at the point of the console.log statement.
It is important to note that only the declarations are hoisted, not the initializations. This means that when a variable is hoisted, it is assigned the value undefined
by default until it is actually assigned a value in the code.
Function declarations are also hoisted in JavaScript. This means that you can call a function before it is declared. Let's see an example:
foo(); // Output: "Hello, World!" function foo() { console.log("Hello, World!"); }
In this example, the function foo
is called before it is declared. However, since function declarations are hoisted, the code executes without any errors and the expected output is displayed.
Understanding execution context and hoisting is crucial for writing efficient and bug-free JavaScript code. It allows you to have a clear understanding of how variables and functions are scoped and accessed within your code. By being aware of hoisting, you can avoid potential issues and write more maintainable code.
To dive deeper into the topic of execution context and hoisting, you can refer to the Mozilla Developer Network for detailed documentation and examples.
Understanding Variable and Function Hoisting
In JavaScript, hoisting is a behavior that allows variables and functions to be used before they are declared. This means that regardless of where variables and functions are declared in your code, they are moved to the top of their respective scopes during the compilation phase. This behavior is known as hoisting.
Variable Hoisting:
In JavaScript, variables declared with the var
keyword are hoisted to the top of their scope. However, only the declaration is hoisted, not the initialization. Let's take a look at an example:
console.log(variable); // Output: undefined var variable = 10; console.log(variable); // Output: 10
In the above example, the variable variable
is hoisted to the top of its scope, which in this case is the global scope. When we try to log its value before it is declared, it outputs undefined
. This is because only the declaration is hoisted, not the initialization. After the declaration, when we log its value again, it outputs 10
.
Function Hoisting:
Similar to variable hoisting, function declarations are also hoisted to the top of their scope. This means that you can call a function before it is actually defined in your code. Here's an example:
hoistedFunction(); // Output: "Hello, hoisting!" function hoistedFunction() { console.log("Hello, hoisting!"); }
In the above example, the function hoistedFunction
is hoisted to the top of its scope, which in this case is the global scope. We can call the function before it is defined, and it will output "Hello, hoisting!"
without any errors.
However, it's important to note that function expressions are not hoisted. Only function declarations are hoisted. Here's an example:
expressionFunction(); // Output: Uncaught TypeError: expressionFunction is not a function var expressionFunction = function() { console.log("Hello, expression!"); };
In the above example, we declare a variable expressionFunction
and assign it an anonymous function. When we try to call the function before it is declared, it throws a TypeError
. This is because function expressions are not hoisted, and the variable expressionFunction
is hoisted with an initial value of undefined
.
Hoisting in Practice:
Understanding hoisting is important because it helps you understand how JavaScript code behaves. By being aware of hoisting, you can avoid potential bugs and write cleaner code.
It's important to note that although hoisting moves declarations to the top of their scopes, it does not change the order of execution. JavaScript still executes code in the same order as it appears in your script.
Related Article: Next.js Bundlers Quick Intro
Global Execution Context: The Basics
The global execution context is the default execution context in JavaScript. It represents the outermost scope and is created when your code is first loaded into the browser. All the code that is not inside any function or block is part of the global execution context.
In the global execution context, two important things are created: the global object and the 'this' keyword. The global object is different depending on the environment where your JavaScript code is running. In a browser, the global object is the 'window' object. In Node.js, it is the 'global' object.
The 'this' keyword in the global execution context refers to the global object. Therefore, when you access a global variable or a function directly in the global scope, you are actually accessing properties and methods of the global object.
Here's an example:
var globalVariable = 'Hello, world!'; function globalFunction() { console.log(globalVariable); // 'Hello, world!' console.log(this.globalVariable); // 'Hello, world!' } console.log(globalVariable); // 'Hello, world!' console.log(this.globalVariable); // 'Hello, world!' globalFunction();
In this example, we define a global variable called 'globalVariable' and a global function called 'globalFunction'. When we log the value of 'globalVariable' both outside and inside the function, we get the same result. This is because the variable is defined in the global execution context and can be accessed from anywhere within the code.
Similarly, when we log the value of 'this.globalVariable', we also get the same result. The 'this' keyword, in the global execution context, refers to the global object, which in this case is the 'window' object.
It's important to note that any variables or functions declared inside other execution contexts, such as function or block scopes, are not accessible in the global execution context unless they are explicitly attached to the global object.
Understanding the global execution context is crucial because it helps you understand how variables and functions are scoped and accessed in your JavaScript code. It also provides a foundation for understanding how hoisting.
Local Execution Context: Dive into the Details
In JavaScript, every time a function is called, a new execution context is created. This execution context consists of two main components: the Variable Environment and the Lexical Environment. These environments are used to store variables and functions, as well as to keep track of the scope chain.
The Variable Environment is where all the variables and function declarations are stored. When a function is called, JavaScript sets up this environment and initializes all the variables within the function. The function's arguments are also defined within the Variable Environment.
The Lexical Environment, on the other hand, is responsible for keeping track of the scope chain. It contains a reference to the outer environment, which is the environment in which the function was lexically defined. This allows JavaScript to look up variables and functions in the correct scope.
Let's take a look at an example to better understand local execution contexts:
function calculateSum(a, b) { var result = a + b; return result; } var x = 5; var y = 10; var sum = calculateSum(x, y); console.log(sum); // Output: 15
In this example, when the calculateSum
function is called with the arguments x
and y
, a new execution context is created. The Variable Environment of this execution context will contain the variables a
, b
, and result
, which are initialized with the values passed as arguments and the sum of x
and y
respectively.
The Lexical Environment of the calculateSum
execution context will have a reference to the outer environment, which in this case is the global environment. This allows the function to access variables defined outside its own scope, such as x
and y
.
Once the function finishes executing, its execution context is destroyed, and the variables within it are no longer accessible. However, the result of the function is stored in the global environment, and we can access it using the sum
variable.
It's important to note that JavaScript uses hoisting to move variable and function declarations to the top of their respective scopes. This means that even though a variable or function declaration may appear later in the code, it can still be accessed from anywhere within its scope.
Understanding local execution contexts and the role of the Variable Environment and Lexical Environment is crucial for writing clean and efficient JavaScript code. It allows you to have a clear understanding of how variables and functions are stored and accessed, and helps you avoid unexpected behavior or bugs in your code.
To dive deeper into this topic, you can refer to the official Mozilla Developer Network documentation on JavaScript's execution context and hoisting.
Scope Chain: Navigating Through the Contexts
When JavaScript code is executed, it creates an execution context for that code. Each execution context has its own scope, which determines the accessibility of variables, functions, and objects within the code. Understanding the scope chain is crucial for navigating through these contexts and accessing the desired variables and functions.
The scope chain in JavaScript is a hierarchical structure that represents the variables and functions available in the current execution context. It allows the code to access variables and functions defined in its own scope, as well as those defined in its parent scopes.
Let's take a look at an example to understand how the scope chain works. Consider the following code snippet:
function outerFunction() { var outerVariable = 'I am outer'; function innerFunction() { var innerVariable = 'I am inner'; console.log(outerVariable + ' and ' + innerVariable); } innerFunction(); } outerFunction();
In this example, we have an outer function that declares a variable outerVariable
and defines an inner function innerFunction
. The inner function also declares a variable innerVariable
and logs the concatenation of both variables to the console.
When the code is executed, an execution context is created for the outerFunction
. This execution context has its own scope, which includes the variable outerVariable
. When the innerFunction
is called, it creates its own execution context with its own scope. The scope chain for the inner function includes both its own scope and the scope of the outer function.
Thus, when innerFunction
tries to access outerVariable
, it first looks for it in its own scope but doesn't find it. It then looks up the scope chain and finds the outerVariable
in the scope of the outer function. As a result, the log statement in the inner function outputs "I am outer and I am inner" to the console.
It's important to note that the scope chain is determined by the lexical structure of the code, also known as the order in which functions are defined. This means that the functions defined inside another function have access to the variables and functions defined in their parent function.
In addition to the parent scope, the scope chain also includes the global scope, which is the outermost scope in JavaScript. Variables and functions defined in the global scope are accessible from any other scope in the code.
Understanding the scope chain is crucial for avoiding variable conflicts and accessing the correct variables and functions in JavaScript. It allows you to navigate through the different execution contexts and leverage the power of lexical scoping.
We will explore the concept of hoisting, which is closely related to the execution context and the scope chain.
To learn more about scope chain and execution contexts, you can refer to the MDN documentation on scope and Function objects.
Related Article: How to Set Select Option Selected By Value in JQuery
Lexical Scope: How JavaScript Determines Scope
In JavaScript, scope refers to the accessibility or visibility of variables, functions, and objects in some particular part of your code during runtime. Lexical scope, also known as static scope, is one of the most fundamental concepts in JavaScript that determines how scope is defined and resolved.
Lexical scope is based on the physical placement of variables and blocks of code in your source code. It is determined at the time of writing your code and remains the same during runtime. In other words, the scope of a variable is determined by its location within the source code structure.
Let's consider an example to understand how lexical scope works:
function outer() { var x = 10; function inner() { console.log(x); // Accessible because of lexical scope } inner(); } outer(); // Output: 10
In the example above, the variable x
is defined inside the outer
function. The inner
function is nested within the outer
function. Since JavaScript uses lexical scoping, the inner
function has access to the variables defined in its outer scope, which in this case is the outer
function. Therefore, the inner
function can access and log the value of the variable x
.
One important thing to note is that lexical scope is not affected by the order in which functions are called or when they are executed. The scope is determined solely by the structure of the code. This means that even if the inner
function is called before the variable x
is assigned a value, it will still have access to the value of x
because of lexical scoping.
function outer() { console.log(x); // Output: undefined var x = 10; } outer();
In the example above, the variable x
is defined after the console.log
statement inside the outer
function. Although the variable x
is defined after the console.log
statement, it is still accessible within the function due to lexical scoping. However, since the variable x
is not assigned a value before the console.log
statement, its value is undefined
.
Understanding lexical scope is crucial when it comes to understanding how JavaScript determines the scope of variables and functions. It provides a foundation for understanding how variables are accessed and resolved during the execution of your code.
To learn more about lexical scope and how it impacts JavaScript execution context, you can refer to the following resources:
- MDN Web Docs: Scope
- FreeCodeCamp: What is Scope in JavaScript?
- DigitalOcean: Understanding Lexical Scope in JavaScript
This Keyword: Unraveling Its Mystery
The this
keyword in JavaScript is one of the most fascinating and often misunderstood aspects of the language. It is a special identifier that is automatically defined in every execution context and refers to the object on which a function is invoked or the context in which a function is executed.
Understanding how this
works is crucial for writing effective and maintainable JavaScript code. We will demystify the this
keyword and explore its behavior in different contexts.
Default Binding
When a function is invoked independently (i.e., not as a property of an object or using the new
keyword), the this
value is set to the global object (window
in browsers, global
in Node.js). This is known as default binding. Let's take a look at an example:
function sayHello() { console.log("Hello, " + this.name); } var name = "John"; sayHello(); // Output: Hello, John
In the above code snippet, this.name
refers to the global variable name
because sayHello()
is invoked independently. If we hadn't defined a global variable name
, it would have resulted in undefined
.
Implicit Binding
When a function is invoked as a method of an object, the this
value is set to the object on which the method is called. This is known as implicit binding. Take a look at the following example:
var person = { name: "Alice", sayHello: function() { console.log("Hello, " + this.name); } }; person.sayHello(); // Output: Hello, Alice
In this case, this.name
refers to the name
property of the person
object because sayHello()
is invoked as a method of the person
object.
Related Article: How to Parse a String to a Date in JavaScript
Explicit Binding
JavaScript provides methods like call()
, apply()
, and bind()
that allow us to explicitly set the value of this
when invoking a function. This is known as explicit binding. Let's see an example using the call()
method:
function sayHello() { console.log("Hello, " + this.name); } var person1 = { name: "Alice" }; var person2 = { name: "Bob" }; sayHello.call(person1); // Output: Hello, Alice sayHello.call(person2); // Output: Hello, Bob
In the above code snippet, call()
is used to invoke the sayHello()
function with different this
values (person1
and person2
objects).
New Binding
When a function is invoked using the new
keyword, a new object is created and the this
value inside the function is set to that new object. This is known as new binding. Consider the following example:
function Person(name) { this.name = name; } var person = new Person("Alice"); console.log(person.name); // Output: Alice
In this case, the Person
function is used as a constructor to create a new object person
with the name
property set to "Alice".
Arrow Functions and Lexical Binding
Arrow functions, introduced in ECMAScript 6, do not bind their own this
value. Instead, they inherit the this
value from the surrounding lexical context. This is known as lexical binding. Take a look at the following example:
var person = { name: "Alice", sayHello: () => { console.log("Hello, " + this.name); } }; person.sayHello(); // Output: Hello, undefined
In this case, the arrow function sayHello()
does not have its own this
value, so it inherits the this
value from the surrounding context, which is the global object. As a result, this.name
is undefined
because the global object does not have a name
property.
Understanding the behavior of this
in different contexts is essential for writing robust JavaScript code. It allows you to control how functions interact with objects and enables you to write more reusable and modular code.
Closures: Harnessing the Power of Lexical Scoping
Closures are a powerful feature in JavaScript that allows functions to retain access to variables from their parent scope, even after the parent function has finished executing. This concept is made possible by the lexical scoping nature of JavaScript.
To understand closures, it's important to first understand lexical scoping. Lexical scoping means that the scope of a variable is determined by its location within the source code, at the time of declaration. In other words, variables are accessible within the block they are defined in, as well as any nested blocks.
Let's take a look at an example to illustrate this concept:
function outerFunction() { const outerVariable = 'I am from the outer function'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const closure = outerFunction(); closure(); // Output: I am from the outer function
In the above code snippet, we have an outer function outerFunction
that defines a variable outerVariable
. Inside outerFunction
, there is an inner function innerFunction
that logs the value of outerVariable
to the console.
When outerFunction
is invoked and assigned to closure
, it returns the innerFunction
. We then call closure
, which still has access to the outerVariable
even though outerFunction
has already finished executing. This is possible because innerFunction
forms a closure over the variables in its lexical scope.
Closures are commonly used to create private variables and encapsulation in JavaScript. By defining variables within a closure, they are not accessible from the outside world, effectively creating private variables. This is often referred to as the module pattern.
function counter() { let count = 0; return function() { count++; console.log(count); }; } const incrementCounter = counter(); incrementCounter(); // Output: 1 incrementCounter(); // Output: 2 incrementCounter(); // Output: 3
In this example, the counter
function returns an anonymous inner function that increments and logs the count
variable. The count
variable is only accessible within the returned inner function, making it effectively private.
By assigning the result of counter
to incrementCounter
, we create a closure that retains access to the count
variable. Each time incrementCounter
is called, it increments and logs the count
variable, producing the expected output.
Closures are a powerful tool for managing state and creating reusable code in JavaScript. They allow functions to remember and access variables from their parent scope, even after the parent function has completed execution. Understanding closures and lexical scoping is crucial for mastering advanced JavaScript concepts.
Related Article: How to Get the Value of Text Input Field in Javascript
Asynchronous Execution: Promises and Callbacks
JavaScript is a single-threaded language, meaning that it can only execute one task at a time. However, there are situations where we need to perform time-consuming operations or make requests to external resources without blocking the execution of other tasks. This is where asynchronous execution comes into play.
Asynchronous execution allows JavaScript to handle time-consuming operations efficiently by not blocking the execution of other tasks. This is achieved through the use of promises and callbacks.
Promises
Promises are objects that represent the eventual completion or failure of an asynchronous operation and its resulting value. They provide a way to handle asynchronous operations in a more readable and manageable manner.
Here's an example of using a promise to fetch data from an API:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Do something with the data }) .catch(error => { // Handle any errors that occurred during the fetch });
In this example, the fetch
function returns a promise that resolves to a Response
object. We can then call the json
method on the response object, which also returns a promise. Finally, we can chain another then
method to handle the data returned by the previous promise.
If any errors occur during the fetch or any of the subsequent operations, the catch
method will be called to handle the error.
Callbacks
Callbacks are functions that are passed as arguments to other functions and are executed at a later point in time. They are a common way to handle asynchronous operations in JavaScript, especially in older codebases.
Here's an example of using a callback to handle the result of an asynchronous operation:
function fetchData(callback) { setTimeout(() => { const data = 'Hello, world!'; callback(data); }, 1000); } fetchData((data) => { console.log(data); });
In this example, the fetchData
function simulates an asynchronous operation by using the setTimeout
function. After a delay of 1000 milliseconds, it calls the provided callback function with the data.
The provided callback function is then executed, and in this case, it simply logs the data to the console.
Callbacks can become difficult to manage when dealing with multiple asynchronous operations or when there are dependencies between them. This can lead to what is commonly known as "callback hell." Promises provide a more elegant solution to handle these situations.
Event Loop: How JavaScript Handles Asynchrony
JavaScript is a single-threaded programming language, which means it can only execute one task at a time. However, it often needs to perform tasks that are time-consuming, such as making network requests or reading from a file. To handle these tasks efficiently without blocking the main execution, JavaScript uses an event loop.
The event loop is a mechanism that allows JavaScript to handle asynchronous operations. It keeps track of all the tasks that need to be executed and schedules them accordingly. When an asynchronous task is initiated, it is added to a task queue. The event loop continuously checks this queue and executes tasks one by one, in the order they were added.
Let's take a look at a simple example to understand how the event loop works:
console.log("First"); setTimeout(function() { console.log("Third"); }, 2000); console.log("Second");
In this example, we have three console.log statements. The first and second statements are synchronous and will be executed immediately. The third statement, however, is asynchronous and will be added to the task queue after a delay of 2000 milliseconds.
When this code runs, the output will be:
First Second Third
The event loop ensures that the asynchronous task inside setTimeout is only executed after the synchronous tasks are completed. It does this by continuously checking the task queue while waiting for the main execution to finish.
JavaScript's event loop also handles other asynchronous operations, such as handling user input, making AJAX requests, or listening for events. Whenever an asynchronous task is completed, a callback function is added to the task queue, which is then executed by the event loop.
Understanding how the event loop works is crucial for writing efficient JavaScript code. It helps in avoiding blocking operations that can cause the application to become unresponsive. By utilizing asynchronous operations and callbacks, JavaScript can efficiently handle tasks without freezing the main execution.
To learn more about the event loop and JavaScript's asynchronous nature, you can refer to the official documentation on the Mozilla Developer Network.
We will explore the concept of closures in JavaScript and how they affect the execution context.
Related Article: How to Generate a GUID/UUID in JavaScript
Common Mistakes: Pitfalls to Avoid
One of the most common mistakes when working with JavaScript execution context and hoisting is misunderstanding the concept of hoisting itself. Hoisting refers to the behavior of moving variable and function declarations to the top of their containing scope. However, it is important to note that only the declarations are hoisted, not the initializations or assignments.
For example, consider the following code snippet:
console.log(myVar); // Output: undefined var myVar = 10;
In this example, the variable myVar
is hoisted to the top of its containing scope, which in this case is the global scope. However, only the declaration of myVar
is hoisted, not the assignment of its value. Therefore, when we try to access myVar
before it is assigned a value, it will return undefined
.
Another common mistake is not understanding the difference between function declarations and function expressions when it comes to hoisting. Function declarations are hoisted, which means they can be called before they are declared. On the other hand, function expressions are not hoisted and cannot be called before they are declared.
Consider the following example:
myFunction(); // Output: "Hello, world!" function myFunction() { console.log("Hello, world!"); }
In this example, the function declaration myFunction
is hoisted to the top, allowing us to call it before the actual declaration. The output will be "Hello, world!".
However, if we try to do the same with a function expression, we will get an error:
myFunction(); // Error: myFunction is not a function var myFunction = function() { console.log("Hello, world!"); };
In this case, the variable myFunction
is hoisted, but its value is undefined
until the actual assignment is reached. Therefore, when we try to call myFunction
before it is assigned a value, we get an error.
Lastly, a common mistake is not paying attention to the scope of variables declared with var
. Variables declared with var
have function scope, not block scope like variables declared with let
or const
. This can lead to unexpected behavior, especially when using loops.
Consider the following example:
for (var i = 0; i < 5; i++) { setTimeout(function() { console.log(i); }, 1000); }
In this example, we expect the numbers 0 to 4 to be printed to the console, each with a delay of 1 second. However, due to the function scope of var
, by the time the setTimeout
function is executed, the loop has already finished and the value of i
is 5. As a result, the number 5 is printed 5 times instead.
To avoid this issue, we can use an immediately invoked function expression (IIFE) to create a new scope for each iteration of the loop:
for (var i = 0; i < 5; i++) { (function(index) { setTimeout(function() { console.log(index); }, 1000); })(i); }
In this modified example, we pass the value of i
to the IIFE as an argument, which creates a new scope with its own copy of index
. As a result, each iteration of the loop will have its own unique index
value, and the expected output of 0 to 4 will be printed to the console.
By being aware of these common mistakes and pitfalls, you can avoid unnecessary bugs and improve your understanding of JavaScript execution context and hoisting.
Real World Examples: Applying Execution Context and Hoisting
We learned about JavaScript execution context and hoisting and how they work. Now, let's explore some real-world examples to see how these concepts apply in practice.
Example 1: Function Declaration Hoisting
Consider the following code snippet:
sayHello(); function sayHello() { console.log("Hello!"); }
In this example, even though the function sayHello()
is called before it is declared, it still works. This is because function declarations are hoisted to the top of their containing scope. So, when the code is executed, the function is already available and can be invoked.
Example 2: Variable Hoisting
Now, let's look at an example involving variable hoisting:
console.log(name); var name = "John Doe";
In this case, the variable name
is hoisted to the top of its containing scope, but its value is not hoisted. So, when we try to log the value of name
before assigning it, we get undefined
. To get the expected output, we need to assign a value to name
before logging it:
var name = "John Doe"; console.log(name);
Related Article: How to Check for String Equality in Javascript
Example 3: Lexical Scope and Execution Context
Lexical scope determines the visibility and accessibility of variables in JavaScript. Let's see an example that showcases how execution context and lexical scope work together:
function outer() { var outerVar = "Hello from outer!"; function inner() { var innerVar = "Hello from inner!"; console.log(innerVar); // Output: Hello from inner! console.log(outerVar); // Output: Hello from outer! } inner(); } outer();
In this example, the function inner()
has access to variables defined in the outer function outer()
. This is possible because of lexical scoping, where inner functions have access to variables in their outer scope. When outer()
is called, a new execution context is created, and the variables outerVar
and inner()
are hoisted within their respective scopes.
Example 4: hoisting and let/const
Hoisting works differently with let
and const
compared to var
. Let's see an example:
console.log(name); let name = "John Doe";
If we run this code, we'll encounter a ReferenceError because variables declared with let
and const
are not hoisted to the top of their containing scope. This behavior ensures that variables are not accessed before they are declared, promoting better code readability and avoiding potential bugs.
We explored several real-world examples that demonstrate how execution context and hoisting work in JavaScript. Understanding these concepts is crucial for writing efficient and bug-free code.
Advanced Techniques: Mastering Complex Scenarios
1. Lexical Environment
In JavaScript, every function has its own lexical environment, which consists of the variables and functions that are in scope at the time the function is defined. The lexical environment is created when a function is defined and remains unchanged throughout the function's execution. This concept is particularly important when dealing with closures.
function outer() { var x = 10; function inner() { console.log(x); } return inner; } var closure = outer(); closure(); // Output: 10
In this example, the inner function has access to the variables in the lexical environment of its parent function, even after the parent function has finished executing. This is because the inner function forms a closure over the variables in its lexical environment.
2. Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that is immediately executed after it is defined. It is often used to create a new execution context and prevent variable hoisting from polluting the global scope.
(function() { var x = 10; console.log(x); })(); // Output: 10
In this example, the function is defined and executed immediately, encapsulating the variable x
within its own execution context. This helps prevent naming conflicts and keeps the global scope clean.
3. Block Scope with let and const
Prior to ES6, JavaScript only had function scope, meaning variables were accessible within the entire function. With the introduction of let
and const
, block scope was introduced.
function example() { if (true) { let x = 10; const y = 20; console.log(x, y); } console.log(x, y); // ReferenceError: x is not defined } example();
In this example, the variables x
and y
are only accessible within the if
block. Attempting to access them outside of the block will result in a ReferenceError
.
4. Hoisting with Function Declarations and Variables
Hoisting is a JavaScript behavior where function declarations and variable declarations are moved to the top of their containing scope during the compilation phase. However, it's important to note that only the declarations are hoisted, not the initializations.
console.log(x); // Output: undefined var x = 10; hoisted(); function hoisted() { console.log('hoisted'); }
In this example, the variable x
is hoisted to the top, but its value is undefined
until the line var x = 10
is encountered during runtime. Similarly, the function declaration hoisted
is also hoisted to the top and can be called before its actual declaration.
These advanced techniques will help you navigate more complex scenarios involving JavaScript execution context and hoisting. Understanding lexical environments, using IIFEs, leveraging block scope, and being aware of hoisting behavior will greatly enhance your JavaScript programming skills.