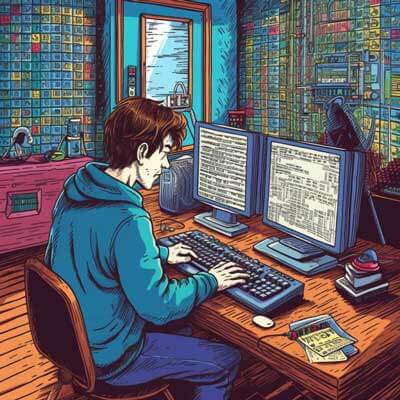
- What is the best way to pass data from parent to child component in Next.js?
- How can I update parent state from a child component in Next.js?
- What is the role of props in parent-child communication in Next.js?
- How does component hierarchy affect state access in Next.js?
- What are the alternatives to passing data through props in Next.js?
- How can I share state between sibling components in Next.js?
- What is the purpose of context in managing state in Next.js?
- How can I handle events in child components in Next.js?
- What is the lifecycle of a component in Next.js?
- Additional Resources
In Next.js, accessing the parent state from a child component can be achieved by passing down the state as a prop to the child component. The parent component can then update the state and pass it down to the child component whenever necessary.
Here’s an example of how to access parent state from a child component in Next.js:
ParentComponent.js:
import React, { useState } from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); } return ( <div> <h2>Parent Component</h2> <p>Count: {count}</p> <button>Increment</button> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ count }) => { return ( <div> <h3>Child Component</h3> <p>Count from parent: {count}</p> </div> ); } export default ChildComponent;
In this example, the ParentComponent has a state variable called “count” which is initially set to 0 using the useState hook. The incrementCount function is used to update the count state. The count state is passed down to the ChildComponent as a prop. The ChildComponent then displays the count value received from the parent component.
What is the best way to pass data from parent to child component in Next.js?
In Next.js, the best way to pass data from a parent component to a child component is by using props. Props allow you to pass data from a parent component to its child components.
Here’s an example of how to pass data from a parent component to a child component in Next.js:
ParentComponent.js:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const data = { name: 'John Doe', age: 30, }; return ( <div> <h2>Parent Component</h2> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ data }) => { return ( <div> <h3>Child Component</h3> <p>Name: {data.name}</p> <p>Age: {data.age}</p> </div> ); } export default ChildComponent;
In this example, the ParentComponent has a data object with name and age properties. The data object is passed down to the ChildComponent as a prop. The ChildComponent then accesses the name and age properties of the data prop and displays them.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
How can I update parent state from a child component in Next.js?
In Next.js, updating the parent state from a child component can be achieved by passing down a callback function from the parent component to the child component as a prop. The child component can then call this callback function to update the parent state.
Here’s an example of how to update parent state from a child component in Next.js:
ParentComponent.js:
import React, { useState } from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); } return ( <div> <h2>Parent Component</h2> <p>Count: {count}</p> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ increment }) => { return ( <div> <h3>Child Component</h3> <button>Increment Count</button> </div> ); } export default ChildComponent;
In this example, the ParentComponent has a state variable called “count” which is initially set to 0 using the useState hook. The incrementCount function is used to update the count state. The incrementCount function is passed down to the ChildComponent as a prop called “increment”. When the button in the ChildComponent is clicked, it calls the increment function from the props, which in turn updates the count state in the parent component.
What is the role of props in parent-child communication in Next.js?
In Next.js, props play a crucial role in enabling communication between parent and child components. Props allow data to be passed from a parent component to its child components, enabling the child components to access and use that data.
Here’s an example of how props are used in parent-child communication in Next.js:
ParentComponent.js:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const name = 'John Doe'; return ( <div> <h2>Parent Component</h2> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ name }) => { return ( <div> <h3>Child Component</h3> <p>Name: {name}</p> </div> ); } export default ChildComponent;
In this example, the ParentComponent has a name variable. The name variable is passed down to the ChildComponent as a prop. The ChildComponent then accesses the name prop and displays it.
Props allow parent components to pass data and functionality to their child components, enabling the child components to render dynamic content and respond to user interactions. This makes parent-child communication flexible and allows for reusable and modular code.
How does component hierarchy affect state access in Next.js?
In Next.js, component hierarchy affects state access. Child components can only access the state of their immediate parent or any ancestor in the component hierarchy. They cannot directly access the state of sibling components or components that are higher up in the hierarchy.
Here’s an example to illustrate how component hierarchy affects state access in Next.js:
GrandparentComponent.js:
import React, { useState } from 'react'; import ParentComponent from './ParentComponent'; const GrandparentComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); } return ( <div> <h2>Grandparent Component</h2> <p>Count: {count}</p> </div> ); } export default GrandparentComponent;
ParentComponent.js:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = ({ increment }) => { return ( <div> <h3>Parent Component</h3> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ increment }) => { return ( <div> <h4>Child Component</h4> <button>Increment Count</button> </div> ); } export default ChildComponent;
In this example, the GrandparentComponent has a state variable called “count” which is initially set to 0 using the useState hook. The incrementCount function is used to update the count state. The incrementCount function is passed down to the ParentComponent as a prop called “increment”. The ParentComponent then passes down the increment prop to the ChildComponent. When the button in the ChildComponent is clicked, it calls the increment function from the props, which in turn updates the count state in the GrandparentComponent.
Child components can access the state of their immediate parent (ParentComponent in this case) because it is passed down as a prop. However, the ChildComponent cannot access the state of the GrandparentComponent directly. The state access is limited to the immediate parent and the ancestors in the component hierarchy.
Related Article: How to Use the forEach Loop with JavaScript
What are the alternatives to passing data through props in Next.js?
In addition to passing data through props, Next.js provides other alternatives for managing and sharing data between components:
1. Context API: The Context API allows you to create a global state that can be accessed by any component in the component tree, regardless of their position in the hierarchy. It eliminates the need to pass data through multiple levels of props.
Here’s an example of how to use the Context API in Next.js:
// DataContext.js import React, { createContext, useState } from 'react'; export const DataContext = createContext(); export const DataProvider = ({ children }) => { const [data, setData] = useState([]); const addData = (newData) => { setData([...data, newData]); } return ( {children} ); }
// ParentComponent.js import React, { useContext } from 'react'; import { DataContext } from './DataContext'; const ParentComponent = () => { const { data } = useContext(DataContext); return ( <div> <h2>Parent Component</h2> <ul> {data.map((item, index) => ( <li>{item}</li> ))} </ul> </div> ); } export default ParentComponent;
// ChildComponent.js import React, { useContext } from 'react'; import { DataContext } from './DataContext'; const ChildComponent = () => { const { data, addData } = useContext(DataContext); const handleAddData = () => { addData('New Data'); } return ( <div> <h3>Child Component</h3> <button>Add Data</button> <ul> {data.map((item, index) => ( <li>{item}</li> ))} </ul> </div> ); } export default ChildComponent;
In this example, the DataContext provides a global state called “data” and a function called “addData” to add new data to the state. The ParentComponent and ChildComponent both access the “data” and “addData” from the DataContext using the useContext hook. This allows them to share and modify the data without passing it through props.
2. Redux: Redux is a state management library that can be used with Next.js to manage and share data across components. It provides a centralized store where the application state is stored and can be accessed by any component.
Here’s a simplified example of how to use Redux with Next.js:
// store.js import { createStore } from 'redux'; const initialState = { count: 0, }; const reducer = (state = initialState, action) => { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1, }; default: return state; } }; const store = createStore(reducer); export default store;
// ParentComponent.js import React from 'react'; import { useDispatch, useSelector } from 'react-redux'; const ParentComponent = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); const incrementCount = () => { dispatch({ type: 'INCREMENT' }); } return ( <div> <h2>Parent Component</h2> <p>Count: {count}</p> <button>Increment</button> </div> ); } export default ParentComponent;
// ChildComponent.js import React from 'react'; import { useSelector, useDispatch } from 'react-redux'; const ChildComponent = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); const incrementCount = () => { dispatch({ type: 'INCREMENT' }); } return ( <div> <h3>Child Component</h3> <p>Count: {count}</p> <button>Increment</button> </div> ); } export default ChildComponent;
In this example, the store.js file sets up the Redux store with an initial state and a reducer function. The ParentComponent and ChildComponent both access the “count” state from the store using the useSelector hook and dispatch actions to update the state using the useDispatch hook.
These alternatives to passing data through props provide more flexibility and scalability when managing and sharing data between components in Next.js.
How can I share state between sibling components in Next.js?
In Next.js, sharing state between sibling components can be achieved by lifting the state up to their common parent component. The parent component can then pass the state down to both sibling components as props.
Here’s an example of how to share state between sibling components in Next.js:
ParentComponent.js:
import React, { useState } from 'react'; import SiblingComponentOne from './SiblingComponentOne'; import SiblingComponentTwo from './SiblingComponentTwo'; const ParentComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); } return ( <div> <h2>Parent Component</h2> <p>Count: {count}</p> <button>Increment</button> </div> ); } export default ParentComponent;
SiblingComponentOne.js:
import React from 'react'; const SiblingComponentOne = ({ count }) => { return ( <div> <h3>Sibling Component One</h3> <p>Count: {count}</p> </div> ); } export default SiblingComponentOne;
SiblingComponentTwo.js:
import React from 'react'; const SiblingComponentTwo = ({ count }) => { return ( <div> <h3>Sibling Component Two</h3> <p>Count: {count}</p> </div> ); } export default SiblingComponentTwo;
In this example, the ParentComponent has a state variable called “count” which is initially set to 0 using the useState hook. The incrementCount function is used to update the count state. The count state is passed down to both SiblingComponentOne and SiblingComponentTwo as a prop. Both sibling components then access the count value received from the parent component and display it.
What is the purpose of context in managing state in Next.js?
In Next.js, context is used for managing and sharing state across components without the need to pass props through every level of the component tree. Context provides a way to create a global state that can be accessed by any component in the component tree, regardless of their position in the hierarchy.
The purpose of context in managing state in Next.js is to simplify the process of sharing state between components and avoid prop drilling. Prop drilling can lead to code complexity and make it difficult to maintain and update the codebase.
Here’s an example of how to use context to manage state in Next.js:
// ThemeContext.js import React, { createContext, useState } from 'react'; export const ThemeContext = createContext(); export const ThemeProvider = ({ children }) => { const [theme, setTheme] = useState('light'); const toggleTheme = () => { setTheme(theme === 'light' ? 'dark' : 'light'); } return ( {children} ); }
// ParentComponent.js import React, { useContext } from 'react'; import { ThemeContext } from './ThemeContext'; const ParentComponent = () => { const { theme, toggleTheme } = useContext(ThemeContext); return ( <div> <h2>Parent Component</h2> <p>Theme: {theme}</p> <button>Toggle Theme</button> </div> ); } export default ParentComponent;
// ChildComponent.js import React, { useContext } from 'react'; import { ThemeContext } from './ThemeContext'; const ChildComponent = () => { const { theme } = useContext(ThemeContext); return ( <div> <h3>Child Component</h3> <p>Theme: {theme}</p> </div> ); } export default ChildComponent;
In this example, the ThemeContext provides a global state called “theme” and a function called “toggleTheme” to toggle the theme. The ParentComponent and ChildComponent both access the “theme” state from the ThemeContext using the useContext hook. The ParentComponent also accesses the “toggleTheme” function to toggle the theme. This allows both components to share and update the theme state without passing it through props.
Context simplifies the process of managing state by providing a centralized location for storing and accessing shared data. It reduces the complexity and number of props passed between components, resulting in cleaner and more maintainable code.
Related Article: How to Use Javascript Substring, Splice, and Slice
How can I handle events in child components in Next.js?
In Next.js, you can handle events in child components by defining event handlers in the child component and passing them down as props from the parent component. The child component can then trigger the event handler when the event occurs.
Here’s an example of how to handle events in child components in Next.js:
ParentComponent.js:
import React from 'react'; import ChildComponent from './ChildComponent'; const ParentComponent = () => { const handleClick = () => { console.log('Button clicked in ParentComponent'); } return ( <div> <h2>Parent Component</h2> </div> ); } export default ParentComponent;
ChildComponent.js:
import React from 'react'; const ChildComponent = ({ onClick }) => { const handleClick = () => { onClick(); } return ( <div> <h3>Child Component</h3> <button>Click me</button> </div> ); } export default ChildComponent;
In this example, the ParentComponent defines an event handler called “handleClick”. The handleClick function logs a message to the console when the button is clicked in the ParentComponent. The handleClick function is passed down to the ChildComponent as a prop called “onClick”. The ChildComponent then triggers the onClick event handler when the button is clicked in the ChildComponent.
What is the lifecycle of a component in Next.js?
In Next.js, components have a lifecycle that consists of several phases from creation to destruction. These lifecycle phases allow you to perform actions at specific points during the component’s lifespan, such as initializing state, fetching data, and cleaning up resources.
The lifecycle of a component in Next.js can be divided into three main phases:
1. Mounting: This phase occurs when a component is being created and inserted into the DOM. The following methods are invoked in the mounting phase:
– constructor: This is the first method called when a component is created. It is used to initialize the component’s state and bind event handlers.
– render: This method is responsible for rendering the component’s UI based on its props and state. It returns JSX or null.
– componentDidMount: This method is called once the component has been mounted to the DOM. It is commonly used to fetch data from an API, set up subscriptions, or perform other side effects.
2. Updating: This phase occurs when a component’s props or state change. The following methods are invoked in the updating phase:
– render: This method is called to re-render the component’s UI when its props or state change.
– componentDidUpdate: This method is called after the component has been re-rendered due to a prop or state change. It is commonly used to perform side effects, such as fetching updated data from an API based on the new props or state.
3. Unmounting: This phase occurs when a component is being removed from the DOM. The following method is invoked in the unmounting phase:
– componentWillUnmount: This method is called just before the component is unmounted and destroyed. It is commonly used to clean up resources, such as canceling API requests or unsubscribing from event listeners.
In addition to these main phases, Next.js also provides other lifecycle methods that can be used for specific purposes, such as handling errors or preventing unnecessary renders:
– shouldComponentUpdate: This method is called before the component is re-rendered and allows you to control whether the re-render should occur. It can be used to optimize performance by preventing unnecessary renders.
– getDerivedStateFromProps: This method is called when the component is about to re-render due to a prop change. It allows you to update the component’s state based on the new props.
– componentDidCatch: This method is called when an error occurs during rendering, in a lifecycle method, or in the constructor of any child component. It allows you to handle and log errors within the component.