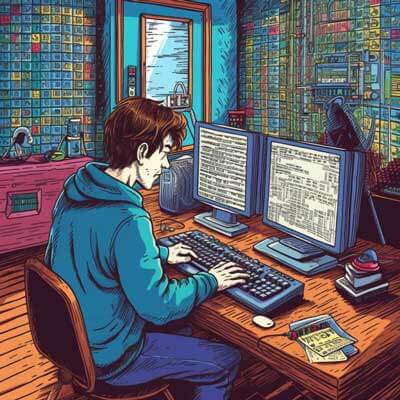
- How can I override the document in Next.js?
- What is the purpose of routing in Next.js?
- Example
- How can I fetch data from an API in Next.js?
- What are the different ways to manage state in Next.js?
- Can I use Next.js for both frontend and backend development?
- What are the advantages of using Next.js over traditional JavaScript frameworks?
- Are there any limitations or drawbacks when using Next.js?
- What is React and how does it relate to Next.js?
- Example
- How does Next.js handle server-side rendering?
- Example
- What is the difference between client-side rendering and server-side rendering?
- Example
- Additional Resources
How can I override the document in Next.js?
In Next.js, the Document
component is responsible for rendering the initial HTML document that is sent to the client. It allows you to customize the document structure, add additional meta tags, and include external scripts and stylesheets.
To override the default Document
component in Next.js, you need to create a new file named _document.js
in the pages
directory of your Next.js project. This file should export a custom Document
component that extends the default Document
provided by Next.js.
Here’s an example of how to override the Document
component in Next.js:
import Document, { Html, Head, Main, NextScript } from 'next/document'; class MyDocument extends Document { render() { return ( <Main /> ); } } export default MyDocument;
In this example, we create a new MyDocument
component that extends the default Document
component from Next.js. We override the render
method to customize the document structure. In this case, we add a meta tag for the viewport and include an external stylesheet.
Note that the MyDocument
component is only used for server-side rendering. On the client-side, Next.js automatically takes over and rehydrates the page using the JavaScript code.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
What is the purpose of routing in Next.js?
Routing is the process of determining which component should be rendered based on the URL of the web application. In a multi-page application, routing allows users to navigate between different pages by changing the URL in the browser’s address bar.
Next.js provides a built-in routing system that allows you to define and handle routes in your application. The routing system in Next.js is based on the file system. Each file in the pages
directory represents a page in the application, and the file name corresponds to the URL path.
For example, if you have a file named about.js
in the pages
directory, it will be accessible at the /about
URL path. Similarly, a file named blog/[slug].js
will be accessible at the /blog/:slug
URL path, where :slug
is a dynamic parameter that can be accessed in the page component.
Next.js also provides a Link
component that allows you to create links between pages in your application. The Link
component automatically handles client-side navigation, providing a smooth and fast user experience.
Example:
Creating a link between pages in Next.js:
import React from 'react'; import Link from 'next/link'; const HomePage = () => { return ( <div> <h1>Welcome to Next.js</h1> <a>About</a> </div> ); }; export default HomePage;
In this example, we create a link to the /about
page using the Link
component from Next.js. When the link is clicked, Next.js handles the navigation and renders the appropriate page.
How can I fetch data from an API in Next.js?
Next.js provides several methods for fetching data from APIs, depending on whether you need to fetch data on the server-side or the client-side.
To fetch data on the server-side and pass it as props to the page component, you can use the getServerSideProps
function. This function is executed on the server before rendering the page and allows you to fetch data from APIs or perform any other asynchronous operations.
Here’s an example of how to fetch data on the server-side in Next.js:
export async function getServerSideProps() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return { props: { data, }, }; } const HomePage = ({ data }) => { return ( <div> <h1>Welcome to Next.js</h1> <p>Data: {data}</p> </div> ); }; export default HomePage;
In this example, the getServerSideProps
function fetches data from an API and returns it as props to the HomePage
component. The fetched data is then rendered on the page.
To fetch data on the client-side, you can use the useEffect
hook together with the fetch
function or any other HTTP client library. This allows you to fetch data after the initial page load and update the UI dynamically.
Here’s an example of how to fetch data on the client-side in Next.js:
import React, { useState, useEffect } from 'react'; const HomePage = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => setData(data)); }, []); return ( <div> <h1>Welcome to Next.js</h1> {data ? <p>Data: {data}</p> : <p>Loading...</p>} </div> ); }; export default HomePage;
In this example, the HomePage
component uses the useState
and useEffect
hooks to fetch data from an API on the client-side. The fetched data is then rendered on the page once it is available.
Related Article: How to Use the forEach Loop with JavaScript
What are the different ways to manage state in Next.js?
Next.js is built on top of React, which provides several options for managing state in a web application. Here are some of the different ways to manage state in Next.js:
1. Local component state: React components can have their own local state using the useState
hook. This allows you to store and update state within a specific component.
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, the Counter
component uses the useState
hook to manage the local state of the count. Clicking the button increments the count and updates the UI.
2. Context API: The Context API allows you to share state between multiple components without passing props through intermediate components. It provides a way to create and access global state within your application.
import React, { createContext, useState } from 'react'; const MyContext = createContext(); const MyProvider = ({ children }) => { const [count, setCount] = useState(0); return ( {children} ); }; const Counter = () => { const { count, setCount } = useContext(MyContext); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, we create a context using the createContext
function and a provider component using the MyProvider
component. The Counter
component accesses the count state using the useContext
hook.
3. Redux: Redux is a popular state management library for JavaScript applications. It provides a predictable state container that can be shared across components. Next.js has built-in support for integrating Redux into your application.
import { useSelector, useDispatch } from 'react-redux'; import { increment } from '../actions/counter'; const Counter = () => { const count = useSelector(state => state.counter); const dispatch = useDispatch(); const handleClick = () => { dispatch(increment()); }; return ( <div> <p>Count: {count}</p> <button>Increment</button> </div> ); }; export default Counter;
In this example, we use the useSelector
hook to access the count state from the Redux store and the useDispatch
hook to dispatch actions. The handleClick
function dispatches an increment
action, which updates the count state.
These are just a few examples of how you can manage state in Next.js. Depending on the complexity of your application, you may choose to use other state management libraries or patterns like MobX, Recoil, or custom solutions.
Can I use Next.js for both frontend and backend development?
Next.js is primarily designed for frontend development, but it also has some features that allow you to handle backend functionality within your Next.js application.
Next.js provides an API routes feature, which allows you to define serverless API endpoints within your Next.js application. These API routes can be used to handle HTTP requests and perform server-side logic, such as fetching data from a database or interacting with external APIs.
To create an API route in Next.js, you need to create a file inside the pages/api
directory. The file should export a default function that takes two arguments: req
(the incoming request) and res
(the outgoing response).
Here’s an example of an API route in Next.js:
// pages/api/hello.js export default function handler(req, res) { res.status(200).json({ message: 'Hello, World!' }); }
In this example, the API route responds with a JSON object containing a simple greeting message.
Next.js also provides support for server-side rendering and static site generation, which can be useful for generating dynamic pages on the server or pre-rendering static pages during the build process.
However, if you’re looking for a full-fledged backend framework, Next.js may not be the best choice. It is recommended to use Next.js in combination with a separate backend framework like Express, NestJS, or Django, depending on your preferred programming language.
What are the advantages of using Next.js over traditional JavaScript frameworks?
Next.js offers several advantages over traditional JavaScript frameworks for building web applications. Here are some of the key advantages:
1. Built-in server-side rendering: Next.js provides built-in support for server-side rendering, which improves the initial loading time of your web application and provides better SEO performance. It allows you to pre-render pages on the server and send fully rendered HTML to the client.
2. Static site generation: Next.js supports static site generation, which allows you to generate static HTML pages at build time. This can significantly improve the performance and scalability of your application, as static pages can be served directly from a CDN without the need for a server.
3. Automatic code splitting and lazy loading: Next.js automatically splits your JavaScript code into smaller chunks and loads them on demand, reducing the initial bundle size and improving the performance of your application. This can result in faster page loads and better user experience.
4. API routes: Next.js provides an API routes feature that allows you to define serverless API endpoints within your Next.js application. This makes it easy to handle backend functionality without the need for a separate backend server.
5. CSS-in-JS support: Next.js has built-in support for CSS-in-JS libraries like styled-components and CSS modules. This allows you to write component-scoped styles directly in your JavaScript or TypeScript files, improving the maintainability and reusability of your styles.
6. Automatic code reloading: Next.js provides a development server that automatically reloads your code whenever you make changes, making the development process faster and more efficient. It also supports hot module replacement, which allows you to update the code of a running application without losing the current application state.
7. TypeScript support: Next.js has excellent support for TypeScript, a statically-typed superset of JavaScript. TypeScript provides enhanced tooling, better code quality, and improved developer productivity, making it easier to build and maintain large-scale applications.
8. Vast ecosystem and community: Next.js is backed by a large and active community of developers, which means you can find a wide range of plugins, tools, and resources to help you build your application. The Next.js ecosystem is constantly growing, with new features and improvements being added regularly.
These are just a few of the advantages that Next.js offers over traditional JavaScript frameworks. Next.js provides a useful and flexible framework for building modern web applications, combining the best features of React with additional optimizations and server-side rendering capabilities.
Related Article: How to Use Javascript Substring, Splice, and Slice
Are there any limitations or drawbacks when using Next.js?
While Next.js offers many advantages, there are also some limitations and drawbacks that you should be aware of:
1. Learning curve: Next.js has a learning curve, especially if you’re new to React and web development. It requires an understanding of React concepts, server-side rendering, and other Next.js-specific features. However, once you get familiar with these concepts, you’ll be able to take full advantage of the framework.
2. Complexity: Next.js introduces additional complexity compared to traditional JavaScript frameworks. It requires setting up a server-side rendering environment, configuring routing, and understanding the build process. This complexity can be challenging for beginners or developers who are used to simpler frameworks.
3. Limited control over the build process: Next.js abstracts away much of the build process, which can be a drawback if you need fine-grained control over the configuration. While Next.js provides sensible defaults, advanced customization may require additional configuration or workarounds.
4. Performance considerations: While Next.js provides optimizations like server-side rendering and automatic code splitting, improper usage or inefficient code can still impact the performance of your application. Care should be taken to optimize your code and ensure efficient data fetching and rendering.
5. Server-side rendering limitations: Server-side rendering has its limitations, especially when it comes to interactivity and responsiveness. Some parts of the page may need to be re-rendered on the client-side, resulting in additional complexity. Care should be taken to balance server-side rendering with client-side interactivity.
6. Integration with existing projects: If you have an existing project that was not built with Next.js, integrating it with Next.js may require significant refactoring and changes to the project structure. This can be time-consuming and may introduce compatibility issues.
It’s important to consider these limitations and drawbacks when deciding whether to use Next.js for your project. While Next.js offers many benefits, it may not be the best fit for every use case. It’s recommended to carefully evaluate your project requirements and consider the trade-offs before choosing Next.js as your framework.
What is React and how does it relate to Next.js?
React is a JavaScript library for building user interfaces. It allows developers to create reusable UI components and build complex UIs by composing these components together. React introduced a declarative syntax, which makes it easier to understand and reason about the UI code.
Next.js is a framework built on top of React that provides additional features for building web applications. It adds server-side rendering, static site generation, and other optimizations to enhance the performance and user experience of React applications.
React and Next.js are closely related because Next.js leverages React for building the UI components and managing the state of the application. Next.js provides a framework for server-side rendering, routing, and other features that are not directly available in React.
Example:
React component:
import React from 'react'; const Button = ({ text }) => { return <button>{text}</button>; }; export default Button;
Next.js page:
import React from 'react'; import Button from '../components/Button'; const HomePage = () => { return ( <div> <h1>Welcome to Next.js</h1> <Button /> </div> ); }; export default HomePage;
In this example, we define a reusable Button component in React and use it in a Next.js page. Next.js allows us to build a complete application by combining React components with additional features like server-side rendering.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
How does Next.js handle server-side rendering?
Server-side rendering (SSR) is a technique that allows rendering web pages on the server before sending them to the client. This approach improves the initial loading time and provides better SEO performance compared to client-side rendering.
Next.js has built-in support for server-side rendering. When a request is made to a Next.js application, the server executes the necessary code to render the requested page on the server. This includes fetching data from APIs, processing the data, and rendering the React components into HTML.
Next.js provides a special component called getServerSideProps
that allows developers to fetch data on the server before rendering the page. This data is then passed as props to the page component, which can be used to render the initial state of the page.
Example:
Next.js page with server-side rendering:
import React from 'react'; const HomePage = ({ data }) => { return ( <div> <h1>Welcome to Next.js</h1> <p>Data: {data}</p> </div> ); }; export async function getServerSideProps() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return { props: { data, }, }; } export default HomePage;
In this example, the getServerSideProps
function is used to fetch data from an API on the server. The fetched data is then passed as a prop to the HomePage
component, which can use it to render the initial state of the page. This ensures that the page is rendered with the latest data on each request.
What is the difference between client-side rendering and server-side rendering?
Client-side rendering (CSR) and server-side rendering (SSR) are two different approaches to rendering web pages in web applications.
In client-side rendering, the initial HTML sent to the client only contains the skeleton of the page, usually a loading spinner or a blank screen. The JavaScript code running on the client’s browser then fetches the necessary data from APIs and renders the page dynamically. This approach provides a fast and interactive user experience once the page is loaded, but it may result in slower initial loading times and poor SEO performance.
On the other hand, server-side rendering renders the web page on the server before sending it to the client. The server executes the necessary code to fetch data from APIs, process the data, and render the page into HTML. This approach provides a fully rendered page to the client, resulting in faster initial loading times and better SEO performance. However, it may have limitations in terms of interactivity and responsiveness, as some parts of the page may need to be re-rendered on the client-side.
Next.js supports both client-side rendering and server-side rendering. By default, Next.js uses server-side rendering for all pages, which provides better SEO performance. However, Next.js also allows developers to opt for client-side rendering when necessary, using techniques like dynamic imports and client-side data fetching.
Related Article: JavaScript HashMap: A Complete Guide
Example:
Client-side rendering with Next.js:
import React, { useState, useEffect } from 'react'; const HomePage = () => { const [data, setData] = useState(null); useEffect(() => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => setData(data)); }, []); return ( <div> <h1>Welcome to Next.js</h1> {data ? <p>Data: {data}</p> : <p>Loading...</p>} </div> ); }; export default HomePage;
In this example, the HomePage
component fetches data from an API on the client-side using the useState
and useEffect
hooks. The page is initially rendered with a loading indicator, and once the data is fetched, it is displayed on the page. This approach provides a fast and interactive user experience, but may result in slower initial loading times compared to server-side rendering.
Additional Resources
– Next.js Documentation
– Manipulating the DOM with JavaScript
– EventTarget.addEventListener()