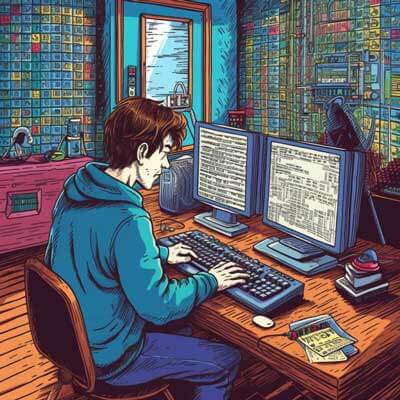
- Understanding Bundlers in Web Development
- Exploring Build Tools in Web Development
- Task Runners
- Module Bundlers
- Static Site Generators
- Understanding Server-side Rendering
- Exploring Static Site Generation
- Next.js and Bundling: A Deep Dive
- Does Next.js 13 Use Webpack as its Bundler?
- Advantages of Next.js for Web Development
- Server-side Rendering (SSR)
- Static Site Generation (SSG)
- Zero Configuration
- Automatic Code Splitting
- Hot Module Replacement (HMR)
- Rich Ecosystem
- React and TypeScript Support
Understanding Bundlers in Web Development
In web development, bundlers play a crucial role in optimizing and packaging our code for production. A bundler takes various modules, dependencies, and assets and combines them into a single output file or multiple files that can be efficiently loaded by the browser. This process helps improve the performance of web applications by reducing the number of requests and the overall file size.
One popular bundler in the JavaScript ecosystem is Webpack. Webpack is a useful module bundler that can handle not only JavaScript but also other assets such as CSS, images, and fonts. It allows us to define an entry point, analyze the dependencies of our code, and generate a bundle that can be served to the client.
Let’s take a look at an example of using Webpack to bundle a simple JavaScript application:
// index.js import { add } from './math'; console.log(add(2, 3));
// math.js export function add(a, b) { return a + b; }
To bundle this code using Webpack, we need to create a configuration file that specifies the entry point, output file, and any additional loaders or plugins we want to use. Here’s an example configuration file:
// webpack.config.js const path = require('path'); module.exports = { entry: './index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, ], }, };
In this example, we specify index.js
as the entry point and bundle.js
as the output file. We also use the Babel loader to transpile our code from ES6 to ES5, allowing us to use modern JavaScript features while ensuring compatibility with older browsers.
When we run Webpack with this configuration, it will analyze the dependencies of our code and generate a bundle that includes both index.js
and math.js
:
$ webpack --config webpack.config.js
The resulting bundle.js
file can then be included in our HTML file and served to the client:
<title>Webpack Example</title>
This is just a basic example, but Webpack can handle much more complex scenarios, such as code splitting, lazy loading, and tree shaking. It provides a wide range of plugins and loaders that can be used to customize the bundling process according to our specific needs.
Related Article: How to Build a Drop Down with a JavaScript Data Structure
Exploring Build Tools in Web Development
In addition to bundlers like Webpack, there are several other build tools commonly used in web development. These tools help automate repetitive tasks, optimize code, and improve the overall development workflow. Let’s explore some of the most popular build tools:
Task Runners
Task runners like Gulp and Grunt are build tools that allow developers to define and automate a series of tasks. These tasks can include tasks such as compiling Sass to CSS, minifying JavaScript files, optimizing images, and more.
Here’s an example of a Gulpfile.js configuration that compiles Sass to CSS:
const gulp = require('gulp'); const sass = require('gulp-sass'); gulp.task('sass', function () { return gulp.src('src/styles.scss') .pipe(sass()) .pipe(gulp.dest('dist/css')); }); gulp.task('default', gulp.series('sass'));
In this example, we define a sass
task that takes the src/styles.scss
file, compiles it to CSS using the gulp-sass
plugin, and outputs the result to the dist/css
directory.
To run this task, we can use the following command:
$ gulp sass
Module Bundlers
As we discussed earlier, module bundlers like Webpack are essential for packaging our code and its dependencies. They analyze the dependencies of our code and generate optimized bundles that can be served to the client.
In addition to Webpack, other popular module bundlers include Rollup and Parcel. Rollup is known for its tree-shaking capabilities, which eliminate unused code from the final bundle. Parcel, on the other hand, focuses on zero-configuration bundling, making it easy to get started with minimal setup.
Related Article: Integrating JavaScript Functions in React Components
Static Site Generators
Static site generators, such as Jekyll, Hugo, and Gatsby, are build tools that generate static HTML files based on templates and data sources. These tools are commonly used for building blogs, documentation sites, and other content-heavy websites.
Gatsby, for example, allows developers to build fast and optimized websites using React and GraphQL. It analyzes the project’s source files, including Markdown files, and generates static HTML pages that can be served by a web server or deployed to a content delivery network (CDN).
Here’s an example of a Gatsby project structure:
. ├── src │ ├── pages │ │ ├── index.js │ │ └── about.js │ └── templates │ └── blog-post.js ├── content │ ├── blog │ │ ├── post-1.md │ │ └── post-2.md │ └── pages │ ├── index.md │ └── about.md └── gatsby-config.js
In this example, the src/pages
directory contains React components that represent the pages of the website. The src/templates
directory contains templates for generating dynamic pages, such as blog posts.
The content
directory contains the content for the website, including Markdown files for blog posts and pages. Gatsby uses this content to generate the corresponding HTML pages during the build process.
To build the Gatsby site, we can use the following command:
$ gatsby build
This will generate a public
directory that contains the final static HTML files, ready to be deployed to a web server or CDN.
Understanding Server-side Rendering
Server-side rendering (SSR) is a technique used in web development to generate HTML content on the server and send it to the client, instead of relying on client-side JavaScript to render the page. SSR has several advantages, including improved performance, search engine optimization (SEO), and better user experience, especially on slow or unreliable networks.
Let’s take a look at an example of server-side rendering using Node.js and Express:
// server.js const express = require('express'); const React = require('react'); const ReactDOMServer = require('react-dom/server'); const App = require('./App'); const app = express(); app.get('/', (req, res) => { const html = ReactDOMServer.renderToString(); res.send(` <title>Server-side Rendering Example</title> <div id="root">${html}</div> `); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, we define an Express server that listens for requests on port 3000. When a request is made to the root URL (/
), we use ReactDOMServer.renderToString
to render the component to a string of HTML.
We then send this HTML string as the response, along with a basic HTML template that includes the rendered HTML inside a <div>
element with the id of “root”.
When the client receives this HTML, it can immediately display the rendered content, without waiting for any client-side JavaScript to execute. This provides a faster initial load time and a better user experience.
Exploring Static Site Generation
Static site generation (SSG) is another technique used in web development to pre-generate HTML pages at build time, rather than on the server or client. SSG is commonly used for websites that have relatively static content and don’t require real-time data updates.
One popular static site generator is Next.js. Next.js is a framework for building React applications with server-side rendering (SSR) and static site generation (SSG) capabilities. It provides a streamlined development experience and optimized performance out of the box.
Let’s take a look at an example of using Next.js for static site generation:
// pages/index.js import React from 'react'; const HomePage = () => { return ( <div> <h1>Welcome to my Next.js website!</h1> <p>This is a static page generated at build time.</p> </div> ); }; export default HomePage;
In this example, we define a simple homepage component using React. This component will be rendered to HTML at build time and served as a static HTML file.
To build and export the static site using Next.js, we can use the following command:
$ next build && next export
This will generate a out
directory that contains the final static HTML files. These files can be deployed to a web server or CDN for serving to users.
Next.js also supports dynamic routes and data fetching during the build process, allowing us to generate dynamic pages with pre-rendered content. This enables us to combine the benefits of static site generation with the flexibility of server-side rendering.
Related Article: How to Group Functional Components in Reactjs
Next.js and Bundling: A Deep Dive
Next.js, as a framework for building React applications, includes a built-in bundler that handles the packaging and optimization of our code. Under the hood, Next.js uses Webpack as its default bundler.
Webpack is a popular choice for Next.js due to its flexibility, extensibility, and wide range of features. It allows Next.js to optimize and bundle our code, handle dependencies, and provide features like code splitting and hot module replacement.
Next.js leverages Webpack’s configuration system to customize the bundling process according to our needs. It provides a next.config.js
file where we can define additional configurations and plugins for Webpack.
For example, we can use the next.config.js
file to add a custom Webpack loader:
// next.config.js module.exports = { webpack: (config, { dev, isServer }) => { // Add a custom loader config.module.rules.push({ test: /\.txt$/, use: 'raw-loader', }); return config; }, };
In this example, we add a custom loader that allows us to import and handle .txt
files using the raw-loader
. This is just one of the many ways we can extend and customize Next.js’s bundling process using Webpack.
Next.js also provides several optimization features out of the box, such as automatic code splitting and tree shaking. These features help reduce the initial load time of our application by only including the necessary code for each page.
Additionally, Next.js supports server-side rendering (SSR), allowing us to generate dynamic content on the server and send it to the client. This can be particularly useful for improving search engine optimization (SEO) and providing a better user experience.
Does Next.js 13 Use Webpack as its Bundler?
Yes, Next.js 13 uses Webpack as its default bundler. Webpack is a useful and widely adopted bundler in the JavaScript ecosystem, providing Next.js with the necessary features for optimizing and packaging our code.
Using Webpack as the default bundler allows Next.js to take advantage of its extensive configuration system, plugins, and loaders. It enables Next.js to handle dependencies, optimize code splitting, and provide a seamless development experience.
However, it’s important to note that Next.js is a highly modular and configurable framework. While Webpack is the default bundler, Next.js allows developers to customize and extend its bundling process using the next.config.js
file. This flexibility allows us to leverage other bundlers or add additional optimizations as needed.
Advantages of Next.js for Web Development
Next.js offers several advantages for web development, making it a popular choice among developers. Here are some of the key advantages of using Next.js:
Related Article: Executing JavaScript Post UI Component Rendering
Server-side Rendering (SSR)
Next.js provides built-in support for server-side rendering (SSR), allowing us to generate HTML on the server and send it to the client. SSR provides several benefits, including improved performance, better search engine optimization (SEO), and enhanced user experience, especially on slow networks.
Static Site Generation (SSG)
In addition to SSR, Next.js supports static site generation (SSG), which allows us to pre-render pages at build time. SSG is ideal for websites with relatively static content and can significantly improve performance and reduce the load on the server.
Zero Configuration
Next.js aims to provide a zero-configuration experience, allowing developers to get started quickly without spending time on complex setup. It provides sensible defaults and automatic optimizations out of the box, reducing the need for manual configuration.
Related Article: Resolving Declaration File Issue for Vue-Table-Component
Automatic Code Splitting
Next.js automatically splits our code into smaller chunks, allowing for faster page loads and better performance. It only loads the necessary JavaScript for each page, reducing the initial load time and improving the overall user experience.
Hot Module Replacement (HMR)
Next.js supports hot module replacement (HMR), which allows us to make changes to our code and see the updates reflected in the browser without a full page reload. This speeds up the development process and improves productivity.
Rich Ecosystem
Next.js benefits from a vibrant and active ecosystem, with a wide range of plugins, extensions, and community support. This makes it easy to find solutions to common problems, share knowledge, and leverage existing tools and libraries.
Related Article: Tutorial: Role of JavaScript in AJAX Technology
React and TypeScript Support
Next.js has excellent support for React, making it a natural choice for React developers. It also provides strong TypeScript integration, allowing us to build type-safe applications and catch potential errors early in the development process.
Overall, Next.js combines the power of server-side rendering, static site generation, and a rich ecosystem to provide a versatile and efficient framework for building modern web applications. Whether you’re building a small blog or a large-scale e-commerce platform, Next.js offers the tools and features to meet your needs.