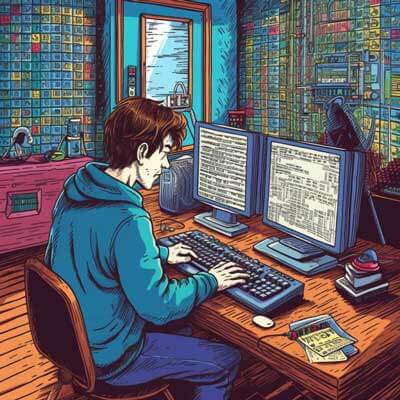
- Redux Integration with Next.js
- Understanding Redux Middleware Options
- Benefits of Using Redux with Next.js
- Recommended Approach to Integrate Redux in a Next.js Project
- Limitations and Considerations of Using Redux with Next.js
- Server-side Rendering of Redux-Connected Components in Next.js
- Popular Redux Middleware Options for Next.js
- Performance Implications of Using Redux with Next.js
- Handling State Management in Next.js without Redux
- Does Next.js Support Redux Out of the Box?
- Additional Resources
Redux Integration with Next.js
Next.js is a popular framework for building React applications with server-side rendering capabilities. Redux, on the other hand, is a state management library commonly used with React to manage application state in a predictable manner. Many developers wonder if Next.js can work seamlessly with Redux, and the answer is yes.
Integrating Redux with Next.js involves setting up the Redux store, connecting Redux to the Next.js application, and using Redux actions and reducers in the components. Let’s take a closer look at how to achieve this.
First, we need to install the necessary dependencies. Assuming you already have a Next.js project set up, you can install Redux and its related libraries using npm or yarn:
npm install redux react-redux
Next, we need to set up the Redux store. Create a new file called store.js
in your Next.js project directory and define your Redux store as follows:
// store.js import { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer); export default store;
In the above example, we import the createStore
function from the Redux library and pass our root reducer to create the Redux store. The root reducer is a combination of all the reducers in your application.
Now that we have our Redux store set up, we can connect it to our Next.js application. In the _app.js
file, which serves as the entry point for your Next.js application, we can wrap our app component with the Provider
component from the react-redux
library:
// _app.js import { Provider } from 'react-redux'; import store from './store'; function MyApp({ Component, pageProps }) { return ( ); } export default MyApp;
In the above example, we import the Provider
component from the react-redux
library and pass our Redux store as a prop. This makes the Redux store available to all components in our Next.js application.
Now that we have our Redux store connected to our Next.js application, we can start using Redux actions and reducers in our components. Let’s say we have a counter component that increments and decrements a counter value stored in the Redux store. Here’s an example implementation:
// Counter.js import { useSelector, useDispatch } from 'react-redux'; import { increment, decrement } from './actions'; function Counter() { const counter = useSelector(state => state.counter); const dispatch = useDispatch(); return ( <div> <h1>Counter: {counter}</h1> <button> dispatch(increment())}>Increment</button> <button> dispatch(decrement())}>Decrement</button> </div> ); } export default Counter;
In the above example, we use the useSelector
hook from the react-redux
library to access the counter value from the Redux store, and the useDispatch
hook to dispatch the increment
and decrement
actions to update the counter value.
That’s it! With these steps, you can integrate Redux with Next.js and start leveraging the power of Redux for state management in your Next.js applications.
Related Article: Accessing Parent State from Child Components in Next.js
Understanding Redux Middleware Options
Redux middleware is a useful feature that allows you to extend the Redux behavior with custom logic. Middleware sits between the dispatching of an action and the moment it reaches the reducers, giving you the ability to intercept, modify, or delay actions.
There are several popular Redux middleware options available, each serving a specific purpose. Let’s take a look at some of the most commonly used Redux middleware options and how they can be used with Next.js.
1. Redux Thunk: Redux Thunk is a popular middleware that allows you to write action creators that return functions instead of plain objects. This is useful for handling asynchronous actions, such as making API requests. To use Redux Thunk with Next.js, you can install it using npm or yarn:
npm install redux-thunk
To apply Redux Thunk middleware to your Redux store, you can modify the store.js
file as follows:
// store.js import { createStore, applyMiddleware } from 'redux'; import thunk from 'redux-thunk'; import rootReducer from './reducers'; const store = createStore(rootReducer, applyMiddleware(thunk)); export default store;
In the above example, we import the applyMiddleware
function from the Redux library and the thunk
middleware from the redux-thunk
library. We then pass thunk
as an argument to applyMiddleware
to apply the middleware to our Redux store.
2. Redux Saga: Redux Saga is another popular middleware that allows you to handle side effects, such as asynchronous API calls, using a more declarative approach. To use Redux Saga with Next.js, you can install it using npm or yarn:
npm install redux-saga
To apply Redux Saga middleware to your Redux store, you can modify the store.js
file as follows:
// store.js import { createStore, applyMiddleware } from 'redux'; import createSagaMiddleware from 'redux-saga'; import rootReducer from './reducers'; import rootSaga from './sagas'; const sagaMiddleware = createSagaMiddleware(); const store = createStore(rootReducer, applyMiddleware(sagaMiddleware)); sagaMiddleware.run(rootSaga); export default store;
In the above example, we import the createSagaMiddleware
function from the redux-saga
library. We create an instance of the saga middleware and apply it to our Redux store using applyMiddleware
. We also run the rootSaga
, which is a generator function that defines the sagas for handling different actions.
3. Redux Logger: Redux Logger is a middleware that logs all the actions and state changes in the console, making it easier to debug and understand the flow of data in your Redux application. To use Redux Logger with Next.js, you can install it using npm or yarn:
npm install redux-logger
To apply Redux Logger middleware to your Redux store, you can modify the store.js
file as follows:
// store.js import { createStore, applyMiddleware } from 'redux'; import logger from 'redux-logger'; import rootReducer from './reducers'; const store = createStore(rootReducer, applyMiddleware(logger)); export default store;
In the above example, we import the logger
middleware from the redux-logger
library and pass it as an argument to applyMiddleware
to apply the middleware to our Redux store.
These are just a few examples of the Redux middleware options available. Depending on your specific use case, you can choose the middleware that best suits your needs. The flexibility of Redux middleware allows you to extend and customize the behavior of your Redux store in a modular and reusable way.
Benefits of Using Redux with Next.js
Next.js and Redux are useful tools that, when used together, can bring numerous benefits to your web application development process. Here are some of the key benefits of using Redux with Next.js:
1. Centralized State Management: Redux provides a centralized store that holds the entire state of your application. This makes it easier to manage and access the state from any component in your Next.js application. With Redux, you can avoid prop drilling and pass data down through multiple layers of components.
2. Predictable State Updates: Redux follows a strict pattern for updating the state called “actions” and “reducers”. Actions are dispatched to describe the changes that need to be made to the state, and reducers handle these actions to update the state. This makes it easier to understand and track how the state is being updated.
3. Time Travel Debugging: Redux allows you to record and replay actions, which makes it easier to debug and reproduce issues in your Next.js application. You can step forward and backward through the state changes, inspecting the state at any given point in time.
4. Middleware Ecosystem: Redux has a rich ecosystem of middleware that can be easily integrated with Next.js. Middleware allows you to extend the behavior of Redux, such as handling asynchronous actions, logging actions, or implementing caching.
5. Server-side Rendering Support: Next.js provides server-side rendering capabilities out of the box, and Redux can be seamlessly integrated with Next.js to support server-side rendering of Redux-connected components. This allows your application to have an initial state on the server, which can improve performance and SEO.
6. Code Organization: Redux promotes a structured and organized approach to managing application state. By separating the concerns of state management from component logic, you can keep your codebase clean and maintainable.
Overall, using Redux with Next.js can improve the scalability, maintainability, and performance of your web applications. It provides a robust solution for managing complex state and enables efficient data flow between components.
Recommended Approach to Integrate Redux in a Next.js Project
Integrating Redux into a Next.js project involves several steps, including setting up the Redux store, connecting Redux to the Next.js application, and using Redux actions and reducers in components. Here’s a recommended approach to integrate Redux in a Next.js project:
1. Set up the Redux Store: Create a file called store.js
in your Next.js project directory and define your Redux store. Import the necessary dependencies from the Redux library and create the store using the createStore
function. Combine your reducers using the combineReducers
function and export the store.
// store.js import { createStore, combineReducers } from 'redux'; import counterReducer from './reducers/counterReducer'; import userReducer from './reducers/userReducer'; const rootReducer = combineReducers({ counter: counterReducer, user: userReducer, }); const store = createStore(rootReducer); export default store;
2. Connect Redux to Next.js: In the _app.js
file, wrap your Next.js app component with the Provider
component from the react-redux
library. Import the Redux store from the store.js
file and pass it as a prop to the Provider
component.
// _app.js import { Provider } from 'react-redux'; import store from './store'; import MyApp from './MyApp'; function App({ Component, pageProps }) { return ( ); } export default App;
3. Create Redux Actions and Reducers: In the actions
directory, create action files that define the actions for your Redux store. Each action file should export an action creator function that returns an action object. In the reducers
directory, create reducer files that handle the actions and update the state accordingly.
// actions/counterActions.js export const increment = () => { return { type: 'INCREMENT', }; }; export const decrement = () => { return { type: 'DECREMENT', }; };
// reducers/counterReducer.js const counterReducer = (state = 0, action) => { switch (action.type) { case 'INCREMENT': return state + 1; case 'DECREMENT': return state - 1; default: return state; } }; export default counterReducer;
4. Connect Redux to Components: In your components, import the necessary functions from the react-redux
library to connect to the Redux store. Use the useSelector
hook to access the state from the store and the useDispatch
hook to dispatch actions to update the state.
// components/Counter.js import { useSelector, useDispatch } from 'react-redux'; import { increment, decrement } from '../actions/counterActions'; function Counter() { const counter = useSelector(state => state.counter); const dispatch = useDispatch(); return ( <div> <h1>Counter: {counter}</h1> <button> dispatch(increment())}>Increment</button> <button> dispatch(decrement())}>Decrement</button> </div> ); } export default Counter;
Related Article: Next.js Bundlers Quick Intro
Limitations and Considerations of Using Redux with Next.js
While using Redux with Next.js can bring many benefits, it’s important to consider the limitations and potential challenges that may arise. Here are some key limitations and considerations to keep in mind when using Redux with Next.js:
1. Complexity: Redux introduces additional complexity to your application. It requires setting up a store, defining actions and reducers, and connecting components to the store. This can make the codebase more complex and harder to maintain, especially for smaller projects.
2. Learning Curve: Redux has a learning curve, particularly if you are new to the library. Understanding the concepts of actions, reducers, and the Redux data flow can take time, especially for developers who are new to state management.
3. Boilerplate Code: Using Redux often involves writing boilerplate code, such as defining action types, action creators, and reducers. This can lead to more code to maintain and can make the development process slower.
4. Performance Considerations: Redux can introduce performance overhead, especially when dealing with large state trees or frequent state updates. Care should be taken to optimize the performance of Redux-connected components, as unnecessary re-renders can impact the overall performance of your Next.js application.
5. Server-side Rendering Complexity: While Next.js supports server-side rendering out of the box, integrating Redux with server-side rendering can be more complex. Special care must be taken to ensure that the initial state is correctly passed from the server to the client to maintain consistency between the server-rendered and client-rendered views.
6. Alternative State Management Options: Redux is not the only state management option available for Next.js. Depending on the complexity of your application, simpler alternatives like React’s built-in useState
or useReducer
hooks may be sufficient and easier to implement.
When deciding whether to use Redux with Next.js, carefully consider the specific needs and requirements of your project. While Redux can be a useful tool for managing complex state, it may not always be necessary or the best fit for every Next.js application.
Server-side Rendering of Redux-Connected Components in Next.js
One of the key benefits of using Next.js is its built-in support for server-side rendering (SSR). SSR allows your Next.js application to render the initial HTML on the server before sending it to the client, improving performance and SEO. When using Redux with Next.js, it’s important to ensure that Redux-connected components are properly rendered on the server.
To achieve server-side rendering of Redux-connected components in Next.js, you need to perform the following steps:
1. Set up Redux on the server: Next.js provides a special lifecycle method called getServerSideProps
that allows you to fetch data on the server before rendering the component. In the page component where your Redux-connected component is rendered, you can use getServerSideProps
to initialize the Redux store with the initial state.
// pages/index.js import { useSelector } from 'react-redux'; import { increment, decrement } from '../actions/counterActions'; function HomePage() { const counter = useSelector(state => state.counter); return ( <div> <h1>Counter: {counter}</h1> <button>Increment</button> <button>Decrement</button> </div> ); } export async function getServerSideProps() { // Fetch initial state from an API or any other source const initialState = await fetch('https://api.example.com/state').then(res => res.json() ); return { props: { initialState } }; } export default HomePage;
In the above example, we define the getServerSideProps
function, which fetches the initial state from an API endpoint. The fetched state is then passed as a prop to the component, which can be accessed using the useSelector
hook.
2. Initialize the Redux store with the initial state: In the _app.js
file, where the Next.js app component is rendered, you can access the pageProps
and initialize the Redux store with the initial state.
// _app.js import { Provider } from 'react-redux'; import store from '../store'; function MyApp({ Component, pageProps }) { // Initialize the Redux store with the initial state store.dispatch({ type: 'INITIALIZE', payload: pageProps.initialState }); return ( ); } export default MyApp;
In the above example, we access the initialState
from the pageProps
passed to the app component and dispatch an INITIALIZE
action to update the Redux store with the initial state.
Popular Redux Middleware Options for Next.js
Next.js provides a flexible and modular architecture that allows you to integrate various Redux middleware options seamlessly. Here are some popular Redux middleware options that can be used with Next.js:
1. Redux Thunk: Redux Thunk is a popular middleware that allows you to write action creators that return functions instead of plain objects. This is useful for handling asynchronous actions, such as making API requests. Redux Thunk can be easily integrated with Next.js by applying the middleware to the Redux store.
// store.js import { createStore, applyMiddleware } from 'redux'; import thunk from 'redux-thunk'; import rootReducer from './reducers'; const store = createStore(rootReducer, applyMiddleware(thunk)); export default store;
2. Redux Saga: Redux Saga is a middleware that allows you to handle side effects, such as asynchronous API calls, using a more declarative approach. Redux Saga provides a useful way to manage complex asynchronous workflows in your Next.js application.
// store.js import { createStore, applyMiddleware } from 'redux'; import createSagaMiddleware from 'redux-saga'; import rootReducer from './reducers'; import rootSaga from './sagas'; const sagaMiddleware = createSagaMiddleware(); const store = createStore(rootReducer, applyMiddleware(sagaMiddleware)); sagaMiddleware.run(rootSaga); export default store;
3. Redux Persist: Redux Persist is a middleware that allows you to persist and rehydrate your Redux store, ensuring that the state is preserved across page reloads. Redux Persist can be used with Next.js to provide a seamless user experience and prevent data loss.
// store.js import { createStore, applyMiddleware } from 'redux'; import { persistStore, persistReducer } from 'redux-persist'; import storage from 'redux-persist/lib/storage'; import rootReducer from './reducers'; const persistConfig = { key: 'root', storage, }; const persistedReducer = persistReducer(persistConfig, rootReducer); const store = createStore(persistedReducer, applyMiddleware()); export const persistor = persistStore(store); export default store;
In the above example, we define a persistConfig
object that specifies the key and storage engine to be used for persisting the Redux store. We then create a persisted reducer using persistReducer
and pass it to the createStore
function along with the persistedReducer
.
These are just a few examples of the popular Redux middleware options that can be used with Next.js. Depending on your specific use case, you can choose the middleware that best suits your needs and integrate it seamlessly into your Next.js application.
Related Article: Integrating React Router with Next.js in JavaScript
Performance Implications of Using Redux with Next.js
When using Redux with Next.js, it’s important to consider the performance implications, as poorly optimized Redux usage can impact the overall performance of your application. Here are some performance considerations when using Redux with Next.js:
1. State Tree Size: The size of your Redux state tree can affect the performance of your application. As the state tree grows larger, the time taken to calculate the diff and update the components can increase. It’s important to keep the state tree as lean as possible and avoid storing unnecessary or derived data in the Redux store.
2. Unnecessary Re-renders: Redux-connected components are re-rendered whenever there is a change in the state they are subscribed to. If your components are subscribed to a large portion of the state tree, it can result in unnecessary re-renders and impact performance. Use the useSelector
hook with memoization to select only the required state slices in your components.
3. Action Dispatching Frequency: Frequent dispatching of actions can also impact performance. If your application triggers multiple actions in a short period, it can cause unnecessary re-renders and reduce the overall performance. Consider batching related actions or debouncing actions to optimize the dispatching frequency.
4. Selector Performance: When using the useSelector
hook, the performance of your selectors can also impact the overall performance of your application. Ensure that your selectors are optimized and memoized using libraries like reselect
. This can prevent unnecessary recomputation of derived data and improve performance.
5. Server-side Rendering: When using server-side rendering in Next.js, the initial state of the Redux store needs to be correctly passed from the server to the client. Ensure that the initial state is consistent between the server-rendered and client-rendered views to avoid any discrepancies.
To optimize the performance of Redux with Next.js, you can follow these best practices:
– Normalize your Redux state to keep it as flat as possible.
– Use memoization techniques, like memoizing selectors with reselect
, to avoid unnecessary recomputation.
– Optimize the dispatching of actions by batching related actions or debouncing actions when necessary.
– Profile your application using tools like Chrome DevTools to identify performance bottlenecks and optimize them accordingly.
Handling State Management in Next.js without Redux
While Redux is a popular choice for state management in Next.js applications, it’s not the only option. Next.js provides built-in features that can handle state management without the need for Redux. Here are some alternatives to Redux for state management in Next.js:
1. React Context: React Context is a built-in feature of React that allows you to share state between components without the need for prop drilling. Next.js can leverage React Context to manage state in a simple and efficient manner. You can create a context provider at the top level of your application and consume the context in any component that needs access to the state.
// StateContext.js import { createContext, useState } from 'react'; const StateContext = createContext(); export const StateProvider = ({ children }) => { const [counter, setCounter] = useState(0); const increment = () => { setCounter(prevCounter => prevCounter + 1); }; const decrement = () => { setCounter(prevCounter => prevCounter - 1); }; return ( {children} ); }; export default StateContext;
In the above example, we create a state context using createContext
and define the state and state update functions. We wrap our Next.js app component with the StateProvider
component, which provides the state and state update functions to all child components.
2. React Hooks: React Hooks, such as useState
and useReducer
, provide a simple and concise way to manage state within individual components. Next.js fully supports React Hooks, allowing you to manage state directly within your components without the need for external state management libraries.
// Counter.js import { useState } from 'react'; function Counter() { const [counter, setCounter] = useState(0); const increment = () => { setCounter(prevCounter => prevCounter + 1); }; const decrement = () => { setCounter(prevCounter => prevCounter - 1); }; return ( <div> <h1>Counter: {counter}</h1> <button>Increment</button> <button>Decrement</button> </div> ); } export default Counter;
In the above example, we use the useState
hook to manage the counter state and the setCounter
function to update the state. This allows us to manage state within the Counter
component without the need for Redux or any external state management libraries.
3. Third-Party State Management Libraries: Next.js is a flexible framework that can integrate with various third-party state management libraries, such as MobX or Zustand. These libraries provide alternative approaches to state management and can be seamlessly integrated into your Next.js application.
When considering alternatives to Redux for state management in Next.js, it’s important to evaluate the specific needs and requirements of your project. While Redux provides a useful and widely adopted solution for state management, simpler alternatives like React Context or React Hooks may be sufficient for smaller or less complex applications.
Does Next.js Support Redux Out of the Box?
Next.js does not provide built-in support for Redux out of the box. However, Next.js is designed to be highly flexible and can seamlessly integrate with Redux. By following the recommended approach outlined earlier, you can easily set up Redux in your Next.js project and leverage its useful state management capabilities.
Next.js provides a custom _app.js
file that acts as the entry point for your Next.js application. By wrapping your app component with the Provider
component from the react-redux
library, you can connect your Redux store to your Next.js application and make the Redux state available to all components.
While Next.js does not provide direct support for Redux, its server-side rendering capabilities work well with Redux. By properly setting up the initial state on the server and passing it to the client, you can achieve server-side rendering of Redux-connected components and improve performance and SEO.
Related Article: How to Create a Navbar in Next.js