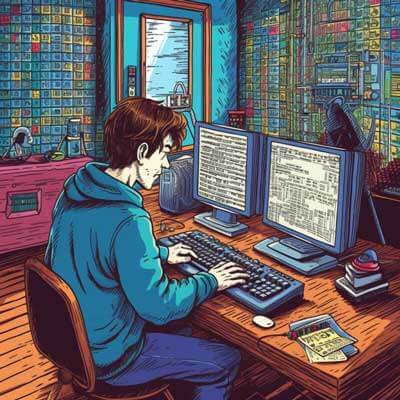
Table of Contents
What is the Vue Table Component in JavaScript?
The Vue Table Component is a reusable and customizable table component for Vue.js applications. It provides a simple way to display data in a tabular format and offers features such as sorting, filtering, pagination, and row selection.
The Vue Table Component is designed to make it easy to work with large datasets and provides a responsive and user-friendly interface for manipulating and viewing data. It allows developers to define custom templates for table cells, headers, and footers, giving them full control over the table's appearance and behavior.
The Vue Table Component is highly configurable and supports various options and callbacks for customization. It provides a flexible API for interacting with the table, making it suitable for a wide range of use cases, from simple data display to complex data manipulation.
The Vue Table Component is built on top of Vue.js and leverages its reactive system, component-based architecture, and reusability. It is widely used in Vue.js applications to present data in a tabular format and offers a convenient way to handle common table-related functionality.
Related Article: How to Check If a String is a Valid Number in JavaScript
How to resolve 'could not find a declaration file for module' error in JavaScript?
The 'could not find a declaration file for module' error occurs when you try to import a JavaScript module in a TypeScript file, but TypeScript cannot find the corresponding declaration file (typically with a '.d.ts' extension) for the module. This error indicates that TypeScript is unable to provide accurate type checking and autocompletion for the module.
To resolve this error, you can take the following steps:
1. Check if a declaration file exists for the module. First, verify if the module has an official TypeScript declaration file available. You can search for the module's declaration file on npm or the DefinitelyTyped repository. If a declaration file exists, you can install it using a package manager like npm or yarn.
2. Create a declaration file manually. If a declaration file does not exist for the module, you can create one manually. Create a new file with the '.d.ts' extension and define the types for the module using TypeScript syntax. Declare the necessary functions, classes, or objects that the module exports. Make sure to accurately define the types to ensure proper type checking and autocompletion.
3. Use an ambient module declaration. If you are importing a module that does not have native TypeScript support, you can use an ambient module declaration. Create a declaration file with the '.d.ts' extension and declare the module using the declare module
syntax. Specify the types for the module's functions, classes, or objects. This allows TypeScript to understand and provide accurate type checking for the module.
4. Disable type checking for the module. If you do not require type checking for the module, you can disable it by using the any
type for the imported module. This tells TypeScript to treat the module as an untyped JavaScript module. However, this approach should be used with caution as it can undermine the benefits of using TypeScript.
5. Use a type assertion. If you are confident in the type of the module and want to suppress the error temporarily, you can use a type assertion. Use the type assertion syntax (as
) to specify the type of the imported module. This tells TypeScript to assume that the module has the specified type, overriding the lack of a declaration file. However, be cautious when using type assertions as they can introduce type safety issues if used incorrectly.
How to use Vue Table Component in a JavaScript project?
To use the Vue Table Component in a JavaScript project, follow these steps:
1. Install Vue.js: Ensure that Vue.js is installed in your project. You can install it using npm or yarn:
npm install vue
2. Install the Vue Table Component: Install the Vue Table Component package using npm or yarn:
npm install vue-table-component
3. Import the Vue Table Component: In your JavaScript file, import the Vue Table Component using the import statement:
import VueTableComponent from 'vue-table-component';
4. Register the Vue Table Component: Register the Vue Table Component globally or locally in your Vue application:
Vue.use(VueTableComponent); // Globally // Or locally in a Vue component export default { components: { VueTableComponent, }, };
5. Use the Vue Table Component: In your Vue template, use the tag to render the table component:
<div> </div> export default { data() { return { tableData: [ { id: 1, name: 'John Doe', age: 30 }, { id: 2, name: 'Jane Smith', age: 25 }, // more data... ], tableColumns: [ { label: 'ID', field: 'id' }, { label: 'Name', field: 'name' }, { label: 'Age', field: 'age' }, ], }; }, };
In this example, the Vue Table Component is imported and registered as a global Vue plugin. It is then used in the template section of a Vue component to render a table with the provided data and columns.
The tableData
array contains the data to be displayed in the table, while the tableColumns
array defines the columns of the table. Each column object in the tableColumns
array specifies the label and field (key) of the corresponding data property.
What is a declaration file?
A declaration file is a file with the extension ".d.ts" that is used in TypeScript to provide type information for JavaScript code. It describes the shape of an existing JavaScript library or module, allowing TypeScript to understand the types and provide accurate type checking and autocompletion within the codebase.
Declaration files typically define the types of variables, functions, classes, and objects present in a JavaScript module. They provide information such as parameter and return types, property types, and method signatures. This allows developers to write TypeScript code that interacts with JavaScript modules seamlessly.
Declaration files are written in TypeScript syntax and can be manually created or generated using tools like the TypeScript Compiler (tsc) or the DefinitelyTyped project.
Related Article: How to Create Responsive Images in Next.JS
Example:
Consider a JavaScript module called "math.js" that exports a function to calculate the sum of two numbers:
// math.js exports.sum = function(a, b) { return a + b; };
To create a declaration file for this module, we would create a new file called "math.d.ts" and define the types:
// math.d.ts export function sum(a: number, b: number): number;
In this example, the declaration file specifies that the "sum" function takes two parameters of type number and returns a value of type number.
Why are declaration files important in JavaScript development?
Declaration files play a crucial role in JavaScript development, especially when using TypeScript. Here are some reasons why they are important:
1. Type Safety: JavaScript is a dynamically typed language, which means that variables can hold values of any type. This flexibility can lead to runtime errors if variables are assigned unexpected values. Declaration files allow TypeScript to enforce strong typing by providing type information for JavaScript code. This helps catch errors at compile-time and improves the overall reliability of the codebase.
2. Autocompletion and IntelliSense: IDEs and code editors that support TypeScript can leverage declaration files to provide autocompletion and IntelliSense features. These features enhance developer productivity by suggesting available properties, methods, and types while writing code. Declaration files provide the necessary information for the editor to offer accurate suggestions, making it easier to work with large codebases and third-party libraries.
3. Documentation: Declaration files serve as a form of documentation for JavaScript modules. They describe the structure and usage of the module's functions, classes, and objects, making it easier for developers to understand and use the module correctly. Declaration files can also include JSDoc comments to provide additional documentation and context for the code.
4. Interoperability: Declaration files enable the seamless integration of JavaScript code with TypeScript code. They allow TypeScript projects to consume JavaScript modules and libraries without sacrificing type safety. This is particularly useful when working with existing JavaScript codebases or when using third-party libraries that do not have native TypeScript support.
Overall, declaration files enhance the development experience by providing type safety, autocompletion, documentation, and interoperability between JavaScript and TypeScript code.
How to create a declaration file for a JavaScript module?
Creating a declaration file for a JavaScript module involves defining the types for the module's functions, classes, and objects. There are several ways to create a declaration file:
1. Manual Declaration: You can create a declaration file manually by writing TypeScript code that describes the types of the JavaScript module. This involves creating a new file with the ".d.ts" extension and using TypeScript syntax to define the types. You can then export the types using the "export" keyword.
2. TypeScript Compiler (tsc): The TypeScript Compiler (tsc) can automatically generate declaration files for JavaScript modules. When compiling TypeScript code that references JavaScript modules, the compiler can generate ".d.ts" files alongside the compiled JavaScript files. This is done by enabling the "declaration" flag in the compiler options.
3. DefinitelyTyped: DefinitelyTyped is a community-driven repository that provides declaration files for thousands of JavaScript libraries and modules. If a declaration file does not exist for a JavaScript module, you can contribute by creating one and submitting it to DefinitelyTyped. This allows other developers to benefit from the declaration file and ensures its availability for future use.
Regardless of the approach, it is important to accurately define the types in the declaration file to ensure proper type checking and autocompletion in TypeScript.
Example:
Let's consider an example where we want to create a declaration file for a JavaScript module called "math.js" that exports a function to calculate the sum of two numbers:
// math.js exports.sum = function(a, b) { return a + b; };
To create the declaration file "math.d.ts" manually, we would define the types as follows:
// math.d.ts export function sum(a: number, b: number): number;
In this example, the declaration file specifies that the "sum" function takes two parameters of type number and returns a value of type number.
Related Article: Javascript Template Literals: A String Interpolation Guide
What is the purpose of a declaration file in JavaScript?
The purpose of a declaration file in JavaScript is to provide type information for JavaScript code, enabling better tooling support and improved developer experience. Declaration files serve the following purposes:
1. Type Checking: JavaScript is a dynamically typed language, which means that variables can hold values of any type. This flexibility can lead to runtime errors if variables are assigned unexpected values. Declaration files allow developers to annotate JavaScript code with type information, enabling static type checking by tools like TypeScript. This helps catch errors at compile-time and improves the overall reliability of the codebase.
2. Autocompletion and IntelliSense: IDEs and code editors that support TypeScript can leverage declaration files to provide autocompletion and IntelliSense features. These features enhance developer productivity by suggesting available properties, methods, and types while writing code. Declaration files provide the necessary information for the editor to offer accurate suggestions, making it easier to work with large codebases and third-party libraries.
3. Documentation: Declaration files serve as a form of documentation for JavaScript modules. They describe the structure and usage of the module's functions, classes, and objects, making it easier for developers to understand and use the module correctly. Declaration files can also include JSDoc comments to provide additional documentation and context for the code.
4. Interoperability: Declaration files enable the seamless integration of JavaScript code with TypeScript code. They allow TypeScript projects to consume JavaScript modules and libraries without sacrificing type safety. This is particularly useful when working with existing JavaScript codebases or when using third-party libraries that do not have native TypeScript support.
How to import a module in Vue.js?
In Vue.js, modules can be imported using the ES6 import syntax. The import statement allows you to bring in functionality from other JavaScript files or modules and use them within your Vue component.
To import a module in Vue.js, follow these steps:
1. Identify the module you want to import. This could be a third-party library or a custom module that you have created.
2. Determine the path to the module file. This can be a relative or absolute path, depending on the file structure of your project.
3. Use the import statement to import the desired module. The import statement follows the syntax: import { module } from 'path/to/module'
. If the module exports a default value, you can import it using import module from 'path/to/module'
.
4. Once the module is imported, you can use its functionality within your Vue component.
Example:
Let's say you have a custom module called "utils.js" that exports a utility function to capitalize a string:
// utils.js export function capitalize(str) { return str.charAt(0).toUpperCase() + str.slice(1); }
To import this module and use the capitalize
function in a Vue component, you would do the following:
// MyComponent.vue <div> <p>{{ capitalizedText }}</p> </div> import { capitalize } from './utils.js'; export default { data() { return { text: 'hello world', }; }, computed: { capitalizedText() { return capitalize(this.text); }, }, };
In this example, the capitalize
function from the "utils.js" module is imported using the ES6 import syntax. It is then used within the computed property capitalizedText
to capitalize the text
data property.
What is the difference between module and component in JavaScript?
In JavaScript, both modules and components are used to organize and encapsulate code. However, they serve different purposes and have different characteristics.
A module is a self-contained unit of code that encapsulates related functionality. It typically consists of a collection of variables, functions, classes, or objects that work together to perform a specific task or provide a specific feature. Modules are designed to promote code reusability, modularity, and maintainability. They can be imported and used by other parts of the application, making it easier to manage dependencies and share code across different files.
On the other hand, a component is a reusable and self-contained piece of code that defines the structure, behavior, and appearance of a user interface (UI) element. Components are typically used in the context of front-end development, where they represent individual parts of a web page or application. Components encapsulate both the visual and behavioral aspects of the UI element, making it easier to reuse, test, and maintain UI code. Components can have their own internal state, lifecycle hooks, and event handling capabilities.
Related Article: How to Reverse an Array in Javascript Without Libraries
How to install typings for a JavaScript module?
Typings, also known as TypeScript declaration files, provide type information for JavaScript modules. They enable TypeScript to understand the types and provide accurate type checking and autocompletion within the codebase. Here's how to install typings for a JavaScript module:
1. Identify the JavaScript module for which you want to install typings. This could be a third-party library or a custom module.
2. Determine if typings are available for the module. Typings are typically distributed as separate packages and can be installed using package managers like npm or yarn.
3. Search for the typings package on npm or the DefinitelyTyped repository. The DefinitelyTyped repository is a community-driven project that provides typings for thousands of JavaScript libraries and modules.
4. Install the typings package using your preferred package manager. If the typings package is available on npm, you can install it using the following command:
npm install @types/module
Replace "module" with the name of the module for which you want to install typings.
5. Once the typings package is installed, TypeScript will automatically include the typings when compiling your code. You can now use the module with accurate type checking and autocompletion in your TypeScript codebase.
Example:
Let's say you want to install typings for the "lodash" JavaScript library. To install the typings, you would do the following:
1. Search for the "lodash" typings package on npm or the DefinitelyTyped repository. In this case, the typings package is available on DefinitelyTyped.
2. Install the typings package using npm:
npm install @types/lodash
3. Once the typings package is installed, TypeScript will automatically include the typings when compiling your code. You can now use the "lodash" library with accurate type checking and autocompletion in your TypeScript codebase.
It's important to note that not all JavaScript modules have typings available. In such cases, you can create your own declaration file or contribute to the DefinitelyTyped repository to provide typings for the module.
What is an ambient module in JavaScript?
An ambient module in JavaScript is a declaration file that provides type information for existing JavaScript libraries or modules that do not have native TypeScript support. Ambient modules are used to describe the shape of external modules and allow TypeScript to understand and provide accurate type checking for them.
Ambient modules are typically used for third-party libraries or modules that are not written in TypeScript but need to be used in TypeScript codebases. They provide type information for the functions, classes, and objects exposed by the external module, enabling TypeScript to provide autocompletion, type checking, and documentation for the module.
Ambient modules are declared using the declare
keyword in TypeScript declaration files. They can be manually created or installed using a typings package from the DefinitelyTyped repository.
Example:
Let's say you want to use the "axios" library, which is a popular JavaScript library for making HTTP requests, in your TypeScript codebase. However, the "axios" library does not have native TypeScript support. In this case, you can create an ambient module declaration file to provide type information for "axios".
Create a file called "axios.d.ts" and declare the ambient module using the declare
keyword as follows:
// axios.d.ts declare module 'axios' { export function get(url: string): Promise; export function post(url: string, data: any): Promise; // additional methods and types... }
In this example, the ambient module declaration file provides type information for the "axios" library. It declares the get
and post
functions and their respective parameter and return types. This allows TypeScript to provide accurate type checking and autocompletion when using the "axios" library in your codebase.
Once the ambient module declaration file is created, you can import and use the "axios" library in your TypeScript code as if it had native TypeScript support.
Related Article: How To Sum An Array Of Numbers In Javascript
How to declare types for Vue.js components?
In Vue.js, types for components can be declared using TypeScript. TypeScript allows you to define the shape and types of the props, data, computed properties, methods, and lifecycle hooks of a Vue component.
To declare types for Vue.js components, follow these steps:
1. Install the necessary dependencies. You need to have TypeScript and the Vue.js typings installed in your project. You can install them using npm or yarn:
npm install typescript vue @types/vue
2. Create a new .vue
file to define your Vue component. This file will contain the template, script, and style sections of your component.
3. In the script section of your Vue component, use TypeScript syntax to define the types of your component's properties, data, computed properties, methods, and lifecycle hooks.
4. Use the defined types within your component to ensure type safety and enable autocompletion in your IDE.
Example:
Consider a simple Vue component called HelloWorld
that takes a name
prop and displays a greeting message:
<div> <p>{{ greeting }}</p> </div> import { Vue, Prop, Component } from 'vue-property-decorator'; @Component export default class HelloWorld extends Vue { @Prop({ type: String, required: true }) name!: string; get greeting(): string { return `Hello, ${this.name}!`; } } /* styles for the component */
In this example, the @Prop
decorator from the vue-property-decorator
package is used to define the type of the name
prop. The name
prop is defined as a required string.
The greeting
computed property is defined with the return type of string
. This ensures that the computed property always returns a string value.
Additional Resources
- Creating Declaration Files