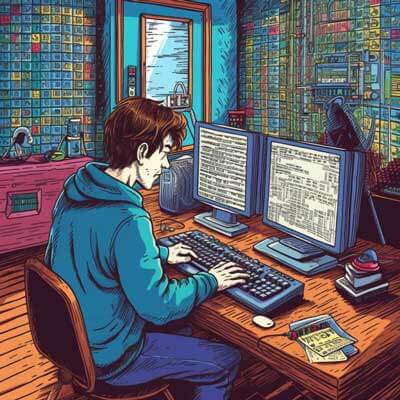
The Purpose of a Property in an Object
In JavaScript, an object is a data structure that allows you to store multiple values as key-value pairs. Each value in an object is called a property. Properties can be of any data type, including strings, numbers, booleans, arrays, functions, or even other objects.
The purpose of a property in an object is to represent a characteristic or attribute of the object. For example, if you have an object that represents a person, you might have properties such as “name”, “age”, and “gender”. These properties provide information about the person object.
To define properties in JavaScript objects, you can use either dot notation or bracket notation. Here are examples of both:
// Using dot notation const person = {}; person.name = "John"; person.age = 30; person.gender = "male"; // Using bracket notation const person = {}; person["name"] = "John"; person["age"] = 30; person["gender"] = "male";
You can also access the properties of an object using either dot notation or bracket notation. Here are examples of both:
// Using dot notation console.log(person.name); // Output: John console.log(person.age); // Output: 30 console.log(person.gender); // Output: male // Using bracket notation console.log(person["name"]); // Output: John console.log(person["age"]); // Output: 30 console.log(person["gender"]); // Output: male
Properties in JavaScript objects can also be modified or deleted. To modify a property, you can simply assign a new value to it. To delete a property, you can use the delete
keyword. Here are examples of modifying and deleting properties:
person.age = 40; // Modifying the age property console.log(person.age); // Output: 40 delete person.gender; // Deleting the gender property console.log(person.gender); // Output: undefined
In addition to the regular properties, JavaScript objects can also have special properties called methods. Methods are functions that are associated with an object and can be called on that object. The next section will discuss defining methods in JavaScript objects.
Related Article: How to Compare Arrays in Javascript
Defining Methods in JavaScript Objects
In JavaScript, methods are functions that are associated with objects. They allow you to define behavior for an object and perform actions related to that object. To define a method in a JavaScript object, you can assign a function to a property of the object. Here’s an example:
const person = { name: "John", age: 30, sayHello: function() { console.log("Hello, my name is " + this.name); } }; person.sayHello(); // Output: Hello, my name is John
In the example above, the sayHello
property is assigned a function that logs a greeting message. The this
keyword refers to the object itself, so this.name
retrieves the value of the name
property of the person
object.
Methods can also accept arguments, just like regular functions. Here’s an example that demonstrates how to define a method with arguments:
const calculator = { add: function(a, b) { return a + b; }, subtract: function(a, b) { return a - b; } }; console.log(calculator.add(5, 3)); // Output: 8 console.log(calculator.subtract(5, 3)); // Output: 2
In the example above, the add
and subtract
methods of the calculator
object accept two arguments and perform the corresponding mathematical operations.
It’s important to note that when defining methods in JavaScript objects, you should use regular function syntax instead of arrow functions. Arrow functions do not bind their own this
value, which means that this
inside an arrow function refers to the surrounding scope instead of the object itself.
const person = { name: "John", sayHello: () => { console.log("Hello, my name is " + this.name); } }; person.sayHello(); // Output: Hello, my name is undefined
In the example above, the arrow function used to define the sayHello
method does not have access to the name
property of the person
object because this
refers to the global scope, where name
is not defined.
To summarize, methods in JavaScript objects allow you to define behavior for an object and perform actions related to that object. They are defined by assigning a function to a property of the object and can accept arguments just like regular functions. Remember to use regular function syntax instead of arrow functions when defining methods to ensure that this
refers to the object itself.
Constructors in JavaScript Objects
In JavaScript, constructors are special functions that are used to create and initialize objects. They are typically used in conjunction with the new
keyword to create instances of a particular object type. Constructors are named with a capital letter by convention to distinguish them from regular functions.
To define a constructor function, you can use the function
keyword followed by the name of the constructor. Inside the constructor function, you can define properties and methods for the object by using the this
keyword. Here’s an example that demonstrates how to define a constructor function:
function Person(name, age) { this.name = name; this.age = age; this.sayHello = function() { console.log("Hello, my name is " + this.name); }; } const person1 = new Person("John", 30); const person2 = new Person("Jane", 25); person1.sayHello(); // Output: Hello, my name is John person2.sayHello(); // Output: Hello, my name is Jane
In the example above, the Person
constructor function takes two parameters, name
and age
, and assigns them to the respective properties of the object being created using the this
keyword. It also defines a sayHello
method that logs a greeting message.
To create instances of the Person
object, you can use the new
keyword followed by the name of the constructor function, passing any required arguments. This creates a new object and invokes the constructor function to initialize its properties.
Constructors can also have prototype properties and methods, which will be discussed in the next section. Using prototype properties and methods can help optimize memory usage by sharing common properties and methods among all instances of an object type.
Prototypes in JavaScript Objects
In JavaScript, prototypes play a crucial role in object-oriented programming. They provide a way to share properties and methods among all instances of an object type, reducing memory usage and improving performance.
Every JavaScript object has an internal property called [[Prototype]]
, which can be accessed using the __proto__
property. The [[Prototype]]
property is a reference to another object, known as the prototype object. When you access a property or method on an object, JavaScript first checks if the object itself has that property or method. If not, it looks up the prototype chain until it finds the property or method or reaches the end of the chain.
To better understand prototypes, let’s consider an example:
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.sayHello = function() { console.log("Hello, my name is " + this.name); }; const person1 = new Person("John", 30); const person2 = new Person("Jane", 25); person1.sayHello(); // Output: Hello, my name is John person2.sayHello(); // Output: Hello, my name is Jane
In the example above, the sayHello
method is defined on the prototype object of the Person
constructor function using the Person.prototype
syntax. This means that all instances of the Person
object share the same sayHello
method, instead of having their own copy.
You can also modify the properties and methods of the prototype object at runtime, and the changes will be reflected in all instances of the object type. Here’s an example that demonstrates how to modify the prototype object:
Person.prototype.sayHello = function() { console.log("Hola, mi nombre es " + this.name); }; person1.sayHello(); // Output: Hola, mi nombre es John person2.sayHello(); // Output: Hola, mi nombre es Jane
In the example above, we modified the sayHello
method of the prototype object to log a message in Spanish instead of English. This change affects all instances of the Person
object.
Prototypes also allow for inheritance in JavaScript, which will be discussed in more detail in the next section.
Related Article: How to Create a Countdown Timer with Javascript
Additional Resources
– MDN Web Docs – Working with objects
– W3Schools – JavaScript Objects
– JavaScript.info – Objects