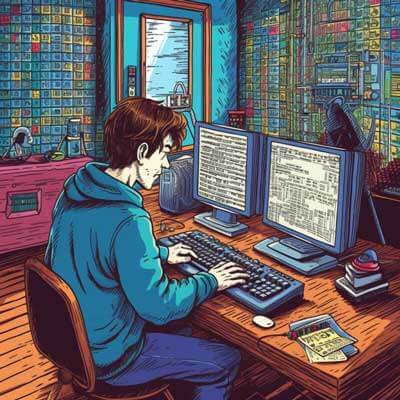
Table of Contents
Why is SSR important in Next.js?
Server-side rendering (SSR) is an essential feature in Next.js that allows rendering web pages on the server before sending them to the client. This approach has several advantages over client-side rendering (CSR) in certain scenarios.
One of the main reasons why SSR is important in Next.js is for search engine optimization (SEO). Search engines rely on the ability to crawl and index web pages to provide relevant search results. However, traditional CSR frameworks often struggle with SEO because they generate the page content dynamically on the client-side using JavaScript. This can lead to search engines indexing incomplete or empty pages, negatively impacting a website's visibility in search results.
Next.js, with its built-in SSR capabilities, solves this problem by rendering the pages on the server and sending the fully rendered HTML to the client. This ensures that search engines can easily crawl and index the content, improving SEO for Next.js applications.
Another reason why SSR is important in Next.js is for performance optimization. In CSR, the initial page load involves downloading the JavaScript bundle and rendering the components on the client-side. This can result in a delay before the user sees any content, leading to a poor user experience, especially on slower connections.
With SSR in Next.js, the server pre-renders the HTML, including the initial data, and sends it to the client. This means that the user sees the content immediately, without any delay, resulting in a faster perceived page load time. Additionally, Next.js supports incremental static regeneration, allowing you to update specific pages without rebuilding the entire application, further improving performance.
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
Example 1:
Consider the following code snippet in a Next.js application:
// pages/index.js import React from 'react'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>Server-side rendering is awesome!</p> </div> ); } export default HomePage;
In this example, the HomePage
component is rendered on the server and the resulting HTML is sent to the client. This ensures that the content is immediately visible to the user, improving the initial page load time.
Example 2:
Next.js provides a getServerSideProps
function that allows you to fetch data from an external API or perform any server-side operations before rendering the page. This is useful for scenarios where you need to fetch dynamic data for the page.
// pages/posts/[id].js import React from 'react'; function Post({ post }) { return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); } export async function getServerSideProps({ params }) { const response = await fetch(`https://api.example.com/posts/${params.id}`); const post = await response.json(); return { props: { post, }, }; } export default Post;
In this example, the getServerSideProps
function fetches the data for a specific post from an external API based on the id
parameter in the URL. The fetched data is then passed as props to the Post
component, which is rendered on the server and sent to the client.
Additional Resources
- Next.js Server Side Rendering vs Client Side Rendering