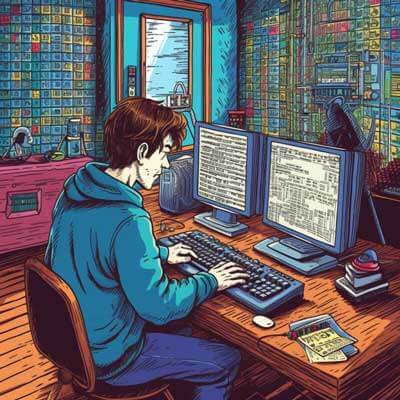
Table of Contents
Introduction to Static Methods
Related Article: How to Flatten a List of Lists in Python
Overview
Static methods are an important concept in object-oriented programming languages like Python. They provide a way to define methods that belong to a class rather than an instance of the class. Unlike instance methods, static methods do not have access to the instance or its attributes. They are primarily used for utility functions or operations that do not depend on the state of the object.
Defining Static Methods
To define a static method in Python, you need to use the @staticmethod
decorator. This decorator indicates that the method is static and does not require an instance of the class to be called. Here's an example:
class MathUtils: @staticmethod def multiply(num1, num2): return num1 * num2
In the above example, the multiply()
method is a static method that multiplies two numbers. It can be called directly on the class without creating an instance:
result = MathUtils.multiply(5, 10) print(result) # Output: 50
Static Methods vs Class Methods
Static methods and class methods are similar in that they both belong to the class rather than the instance. However, there is a subtle difference between the two. While static methods do not have access to the instance or its attributes, class methods have access to the class itself.
To define a class method in Python, you need to use the @classmethod
decorator. Class methods take the class as the first argument, conventionally named cls
. Here's an example to demonstrate the difference between static methods and class methods:
class MyClass: @staticmethod def static_method(): print("This is a static method") @classmethod def class_method(cls): print("This is a class method") # Calling the static method MyClass.static_method() # Output: This is a static method # Calling the class method MyClass.class_method() # Output: This is a class method
Related Article: Python Programming for Kids
Static Methods vs Instance Methods
Static methods and instance methods are fundamentally different. Instance methods are associated with an instance of a class and have access to the instance and its attributes. On the other hand, static methods do not have access to the instance and are not bound to a specific instance.
Here's an example that demonstrates the difference between static methods and instance methods:
class MyClass: def __init__(self, name): self.name = name def instance_method(self): print(f"Hello, {self.name}!") @staticmethod def static_method(): print("This is a static method") # Creating an instance of MyClass my_instance = MyClass("John") # Calling the instance method my_instance.instance_method() # Output: Hello, John! # Calling the static method MyClass.static_method() # Output: This is a static method
In the above example, the instance_method()
is an instance method that is called on an instance of the class, whereas the static_method()
is a static method that is called directly on the class itself.
Use Case: Optimization with Static Methods
Overview
Static methods are often used in situations where performance optimization is a concern. By using static methods, you can avoid unnecessary object creation and method invocations, resulting in improved efficiency.
Example 1: Prime Number Check
Let's consider a scenario where you need to frequently check if a given number is prime. Instead of creating an instance of a class and invoking an instance method for each number, you can define a static method that performs the prime number check. Here's an example:
class MathUtils: @staticmethod def is_prime(num): if num < 2: return False for i in range(2, int(num**0.5) + 1): if num % i == 0: return False return True # Using the static method to check prime numbers print(MathUtils.is_prime(17)) # Output: True print(MathUtils.is_prime(25)) # Output: False
In the above example, the is_prime()
static method efficiently checks if a given number is prime without the need for object instantiation.
Related Article: Python Reduce Function Explained
Example 2: Utility Function
Static methods can also be used to define utility functions that are not tied to any particular instance or class. These utility functions can be reused across different modules or projects without the need for inheritance or object creation. Here's an example:
class StringUtils: @staticmethod def reverse_string(string): return string[::-1] # Using the static method to reverse a string print(StringUtils.reverse_string("Hello, World!")) # Output: "!dlroW ,olleH"
In the above example, the reverse_string()
static method provides a utility function to reverse a given string without requiring an instance or object.
Best Practice: Proper Use of Static Methods
Overview
To ensure the proper use of static methods in your code, consider the following best practices:
1. Only use static methods when they are truly independent of the instance or class state.
2. Avoid using static methods for operations that rely on the instance or class attributes. Instead, use instance methods or class methods.
3. Use static methods for utility functions, helper methods, or operations that do not require access to the instance or class.
Example: Proper Use of Static Methods
Let's consider an example where we have a FileUtils
class that provides static methods for file operations. These methods are independent of the instance or class state and serve as utility functions. Here's an example:
class FileUtils: @staticmethod def read_file(file_path): with open(file_path, "r") as file: return file.read() @staticmethod def write_file(file_path, content): with open(file_path, "w") as file: file.write(content) # Using the static methods for file operations content = FileUtils.read_file("sample.txt") FileUtils.write_file("output.txt", content)
In the above example, the read_file()
and write_file()
static methods provide utility functions for reading from and writing to files. These methods do not rely on any instance or class attributes and can be used independently.
Related Article: Implementation of Data Structures in Python
Best Practice: Limitations of Static Methods
Overview
While static methods have their use cases and benefits, it's important to be aware of their limitations. Here are some limitations to consider:
1. Static methods cannot access the instance or its attributes. If you need access to instance-specific data, consider using instance methods or class methods.
2. Static methods cannot be overridden. If you require polymorphic behavior, consider using instance methods or class methods.
3. Static methods cannot make use of inheritance. If you need to inherit behavior or attributes, consider using class methods.
4. Static methods can make code less modular if they are overused. Consider using instance methods or class methods when appropriate to improve code organization and maintainability.
Understanding these limitations can help you make informed decisions when choosing between static methods, instance methods, and class methods.
Example: Limitations of Static Methods
Let's consider an example where we have a Shape
class with a static method calculate_area()
. While static methods can be used for calculations, they have limitations when it comes to polymorphism and inheritance. Here's an example:
class Shape: @staticmethod def calculate_area(): raise NotImplementedError("Method not implemented for Shape") class Circle(Shape): @staticmethod def calculate_area(): return 3.14 * radius**2 # Calling the static method Shape.calculate_area() # Output: NotImplementedError # Creating an instance of Circle radius = 5 circle = Circle() # Calling the static method on the Circle instance circle.calculate_area() # Output: NotImplementedError
In the above example, the calculate_area()
static method in the Shape
class raises a NotImplementedError
to indicate that it should be overridden in the subclasses. However, since static methods cannot be overridden, the same error is raised even when called on a Circle instance. This limitation highlights the importance of choosing the appropriate method type based on the desired behavior.
Real World Example: Static Methods in Data Analysis
Related Article: How To Find Index Of Item In Python List
Overview
Static methods can be particularly useful in the field of data analysis, where utility functions and operations that don't depend on the instance or class state are common.
Example: Data Analysis Utilities
Let's consider an example where we have a DataAnalyzer
class that provides static methods for common data analysis operations. These methods are independent of the instance or class state and can be used across different data analysis projects. Here's an example:
class DataAnalyzer: @staticmethod def calculate_average(data): return sum(data) / len(data) @staticmethod def normalize_data(data): min_value = min(data) max_value = max(data) return [(x - min_value) / (max_value - min_value) for x in data] # Using the static methods for data analysis numbers = [10, 15, 20, 25, 30] average = DataAnalyzer.calculate_average(numbers) normalized_data = DataAnalyzer.normalize_data(numbers)
In the above example, the calculate_average()
static method calculates the average of a given list of numbers, while the normalize_data()
static method normalizes the data to a range of 0 to 1. These utility functions can be used in various data analysis projects without the need for object instantiation.
Real World Example: Static Methods in System Operations
Overview
Static methods can also be valuable in system operations, where utility functions and operations that are not tied to a specific instance of a class are often needed.
Related Article: Flask-Babel For Internationalization & Localization
Example: System Utilities
Let's consider an example where we have a SystemUtils
class that provides static methods for common system operations. These methods are independent of the instance or class state and can be used across different system-related tasks. Here's an example:
import os class SystemUtils: @staticmethod def get_current_directory(): return os.getcwd() @staticmethod def list_files(directory): return os.listdir(directory) # Using the static methods for system operations current_directory = SystemUtils.get_current_directory() files = SystemUtils.list_files(current_directory)
In the above example, the get_current_directory()
static method retrieves the current working directory, while the list_files()
static method lists all the files in a given directory. These utility functions can be used in various system-related operations without the need for object instantiation.
Performance Consideration: Static Methods and Memory Usage
Overview
While static methods provide benefits in terms of performance optimization, it's important to consider their impact on memory usage. As static methods are associated with the class rather than the instance, they do not require object instantiation. However, the class itself still occupies memory.
Example: Memory Usage of Static Methods
Let's consider an example where we have a MemoryAnalyzer
class that uses static methods to measure memory usage. Here's an example:
import sys class MemoryAnalyzer: @staticmethod def get_memory_usage(): return sys.getsizeof(MemoryAnalyzer) # Calling the static method memory_usage = MemoryAnalyzer.get_memory_usage() print(memory_usage) # Output: MemoryAnalyzer object size in bytes
In the above example, the get_memory_usage()
static method uses the sys.getsizeof()
function to calculate the size of the MemoryAnalyzer
class object in bytes. This demonstrates that the class itself occupies memory, even without creating an instance.
Related Article: How to Replace Strings in Python using re.sub
Performance Consideration: Static Methods and Execution Time
Overview
Static methods can provide performance benefits by avoiding unnecessary object creation and method invocations. However, it's important to consider the execution time of static methods, especially when they involve complex computations or operations.
Example: Execution Time of Static Methods
Let's consider an example where we have a TimeAnalyzer
class that uses static methods to measure the execution time of operations. Here's an example:
import time class TimeAnalyzer: @staticmethod def measure_execution_time(): start_time = time.time() # Perform some complex operation end_time = time.time() return end_time - start_time # Calling the static method execution_time = TimeAnalyzer.measure_execution_time() print(execution_time) # Output: Execution time in seconds
In the above example, the measure_execution_time()
static method uses the time.time()
function to measure the execution time of a complex operation. This demonstrates that static methods can be used to track and analyze the performance of specific operations.
Advanced Technique: Overriding Static Methods
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Overview
One of the limitations of static methods is that they cannot be overridden in subclasses. However, there are advanced techniques that can be used to achieve similar behavior by using class methods or instance methods.
Example: Dynamic Behavior with Class Methods
Let's consider an example where we have a BaseClass
with a static method static_method()
that we want to override in a subclass. Although static methods cannot be overridden, we can achieve similar behavior using class methods. Here's an example:
class BaseClass: @staticmethod def static_method(): print("BaseClass static method") class SubClass(BaseClass): @classmethod def static_method(cls): print("SubClass static method") # Calling the static method on the subclass SubClass.static_method() # Output: SubClass static method
In the above example, the SubClass
defines a class method with the same name as the static method in the BaseClass
. By using a class method, we can achieve dynamic behavior similar to overriding a static method.
Advanced Technique: Static Methods and Metaclasses
Overview
Metaclasses provide a way to customize the creation and behavior of classes. While static methods themselves are not directly related to metaclasses, they can be used in conjunction with metaclasses to add additional functionality or behavior to a class.
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
Example: Metaclass with Static Methods
Let's consider an example where we have a metaclass CustomMetaclass
that adds static methods to classes created using the metaclass. Here's an example:
class CustomMetaclass(type): def __new__(cls, name, bases, attrs): attrs['static_method'] = lambda: print("CustomMetaclass static method") return super().__new__(cls, name, bases, attrs) # Creating a class using the metaclass class MyClass(metaclass=CustomMetaclass): pass # Calling the static method on the class MyClass.static_method() # Output: CustomMetaclass static method
In the above example, the CustomMetaclass
dynamically adds a static method to any class created using the metaclass. This demonstrates how static methods can be incorporated into the metaclass process to enhance class behavior.
Code Snippet: Basic Static Method
Overview
A basic static method in Python is a method that belongs to the class rather than an instance of the class. It does not require object instantiation and can be called directly on the class itself.
Code Snippet
class MathUtils: @staticmethod def multiply(num1, num2): return num1 * num2 # Using the static method result = MathUtils.multiply(5, 10) print(result) # Output: 50
In the above code snippet, the multiply()
method is a static method defined in the MathUtils
class. It takes two numbers as arguments and returns their product. The static method can be called directly on the class without creating an instance.
Related Article: How To Create Pandas Dataframe From Variables - Valueerror
Code Snippet: Static Method with Arguments
Overview
Static methods in Python can accept arguments just like any other method. These arguments can be used within the static method to perform specific operations.
Code Snippet
class StringUtils: @staticmethod def capitalize(text): return text.capitalize() # Using the static method with arguments result = StringUtils.capitalize("hello, world!") print(result) # Output: "Hello, world!"
In the above code snippet, the capitalize()
static method in the StringUtils
class accepts a text
argument and returns the capitalized version of the text. The static method can be called directly on the class and passed the required argument.
Code Snippet: Static Method in Inheritance
Related Article: How To Check If Key Exists In Python Dictionary
Overview
Static methods in Python cannot be overridden in subclasses. However, you can achieve similar behavior by using class methods or instance methods.
Code Snippet
class BaseClass: @staticmethod def static_method(): print("BaseClass static method") class SubClass(BaseClass): @staticmethod def static_method(): print("SubClass static method") # Calling the static method on the subclass SubClass.static_method() # Output: SubClass static method
In the above code snippet, the SubClass
defines a static method with the same name as the static method in the BaseClass
. Although the static method cannot be overridden, the subclass's static method will be called when invoked on the subclass.
Code Snippet: Static Method and Decorators
Overview
Static methods in Python can also be used in conjunction with decorators to add additional functionality or behavior to the methods.
Related Article: How to Calculate the Square Root in Python
Code Snippet
class Logger: @staticmethod def log_method(func): def wrapper(*args, **kwargs): print(f"Calling method: {func.__name__}") return func(*args, **kwargs) return wrapper class MathUtils: @staticmethod @Logger.log_method def multiply(num1, num2): return num1 * num2 # Using the static method with the decorator result = MathUtils.multiply(5, 10) print(result) # Output: 50
In the above code snippet, the Logger
class defines a static method log_method()
that adds logging functionality to methods. The MathUtils
class uses the @Logger.log_method
decorator to apply the logging behavior to the multiply()
static method.
Code Snippet: Static Method and Exception Handling
Overview
Static methods in Python can handle exceptions just like any other method. You can use try-except blocks within static methods to catch and handle specific exceptions.
Code Snippet
class MathUtils: @staticmethod def divide(num1, num2): try: result = num1 / num2 except ZeroDivisionError: print("Cannot divide by zero") return None else: return result # Using the static method with exception handling result = MathUtils.divide(10, 0) print(result) # Output: None
In the above code snippet, the divide()
static method in the MathUtils
class attempts to divide two numbers. If a ZeroDivisionError
occurs, it catches the exception, prints a message, and returns None
. Otherwise, it returns the result of the division.
Related Article: How to Use Python dotenv
Error Handling with Static Methods
Overview
Static methods in Python can handle errors and exceptions just like any other method. You can use try-except blocks within static methods to catch and handle specific errors or exceptions.
Code Snippet
class MathUtils: @staticmethod def divide(num1, num2): try: result = num1 / num2 except ZeroDivisionError: print("Cannot divide by zero") return None except Exception as e: print(f"An error occurred: {str(e)}") return None else: return result # Using the static method with error handling result = MathUtils.divide(10, 0) print(result) # Output: None
In the above code snippet, the divide()
static method in the MathUtils
class attempts to divide two numbers. It uses try-except blocks to catch specific errors or exceptions. If a ZeroDivisionError
occurs, it catches the exception, prints a message, and returns None
. If any other exception occurs, it catches the exception, prints the error message, and returns None
. Otherwise, it returns the result of the division.