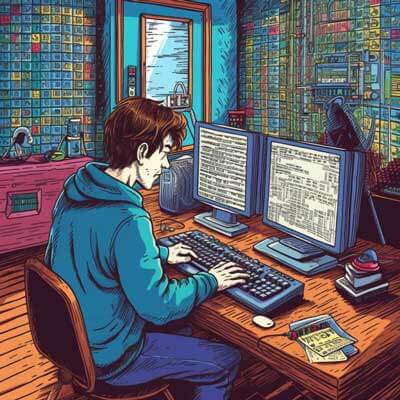
In Python, the truth value of a series can sometimes be ambiguous. This means that when evaluating the truthiness of a series, Python may not be able to determine whether the series is considered True or False. To handle this ambiguity, there are several methods available in the pandas library that can be used. These methods include a.empty
, a.bool()
, a.item()
, a.any()
, and a.all()
. In this answer, we will explore each of these methods and discuss when and how to use them.
Background
Before we dive into the methods for handling ambiguous truth values in a Python series, let’s briefly discuss why this issue arises in the first place. In Python, truthiness is determined by the bool()
function, which converts an object into a boolean value. By default, most objects in Python are considered True, unless they are empty or have a custom __bool__()
or __len__()
method that returns False.
However, when working with pandas series, the truth value of the entire series is ambiguous because it is not clear how to evaluate the truthiness of multiple elements. For example, consider the following series:
import pandas as pd s = pd.Series([1, 2, 3])
What should be the truth value of s
? Should it be True because the series is not empty? Or should it be False because it contains multiple elements? This ambiguity can lead to unexpected behavior and errors in your code if not handled properly.
To address this issue, pandas provides several methods that allow you to explicitly handle the truth value of a series. Let’s explore each of these methods in detail.
Related Article: How To Create Pandas Dataframe From Variables - Valueerror
a.empty
The a.empty
method returns True if the series is empty, and False otherwise. This method can be used to check if a series contains any elements or if it is completely empty.
Here’s an example:
import pandas as pd # Create an empty series s1 = pd.Series([]) # Create a non-empty series s2 = pd.Series([1, 2, 3]) # Check if the series is empty print(s1.empty) # Output: True print(s2.empty) # Output: False
In this example, s1.empty
returns True because the series s1
is empty, while s2.empty
returns False because the series s2
contains elements.
a.bool()
The a.bool()
method returns a boolean value representing the truthiness of the series. If the series is empty or contains only elements that evaluate to False, a.bool()
will return False. Otherwise, it will return True.
Here’s an example:
import pandas as pd # Create an empty series s1 = pd.Series([]) # Create a series with elements that evaluate to False s2 = pd.Series([0, '', False]) # Create a series with elements that evaluate to True s3 = pd.Series([1, 'hello', True]) # Check the truth value of the series print(s1.bool()) # Output: False print(s2.bool()) # Output: False print(s3.bool()) # Output: True
In this example, s1.bool()
returns False because the series s1
is empty. s2.bool()
also returns False because all elements in s2
evaluate to False. On the other hand, s3.bool()
returns True because at least one element in s3
evaluates to True.
a.item()
The a.item()
method returns the single value contained in the series. This method can only be used if the series contains exactly one element. If the series is empty or contains more than one element, a ValueError
will be raised.
Here’s an example:
import pandas as pd # Create a series with a single element s1 = pd.Series([42]) # Create an empty series s2 = pd.Series([]) # Create a series with multiple elements s3 = pd.Series([1, 2, 3]) # Get the single value from the series print(s1.item()) # Output: 42 # Trying to get the single value from an empty series will raise an error print(s2.item()) # Raises ValueError: can only convert an <a href="https://www.squash.io/how-to-work-with-lists-and-arrays-in-python/">array of size 1 to a Python</a> scalar # Trying to get the single value from a series with multiple elements will also raise an error print(s3.item()) # Raises ValueError: can only convert an array of size 1 to a Python scalar
In this example, s1.item()
returns the single value 42
because s1
contains only one element. However, trying to call item()
on an empty series or a series with multiple elements will raise a ValueError
.
Related Article: How to Sort a Pandas Dataframe by One Column in Python
a.any()
The a.any()
method returns True if any element in the series is True, and False otherwise. If the series is empty, a.any()
will return False.
Here’s an example:
import pandas as pd # Create an empty series s1 = pd.Series([]) # Create a series with elements that evaluate to False s2 = pd.Series([0, '', False]) # Create a series with elements that evaluate to True s3 = pd.Series([1, 'hello', True]) # Check if any element in the series is True print(s1.any()) # Output: False print(s2.any()) # Output: False print(s3.any()) # Output: True
In this example, s1.any()
returns False because the series s1
is empty. s2.any()
also returns False because all elements in s2
evaluate to False. However, s3.any()
returns True because at least one element in s3
evaluates to True.
a.all()
The a.all()
method returns True if all elements in the series are True, and False otherwise. If the series is empty, a.all()
will return True.
Here’s an example:
import pandas as pd # Create an empty series s1 = pd.Series([]) # Create a series with elements that evaluate to False s2 = pd.Series([0, '', False]) # Create a series with elements that evaluate to True s3 = pd.Series([1, 'hello', True]) # Check if all elements in the series are True print(s1.all()) # Output: True print(s2.all()) # Output: False print(s3.all()) # Output: True
In this example, s1.all()
returns True because the series s1
is empty. s2.all()
returns False because at least one element in s2
evaluates to False. However, s3.all()
returns True because all elements in s3
evaluate to True.