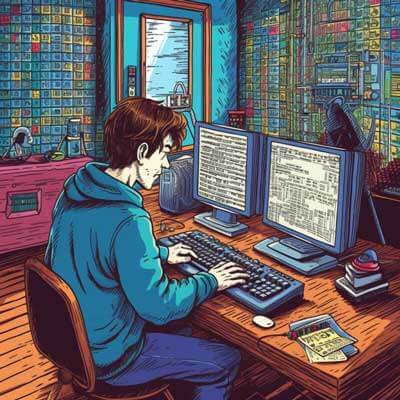
Table of Contents
Introduction to SQL Data Types in MySQL
SQL data types in MySQL are used to define the type of data that can be stored in a column of a database table. These data types help ensure data integrity and provide a way to enforce constraints on the values that can be stored. MySQL supports a wide range of data types, each with its own unique characteristics and purposes.
Related Article: How to Select Specific Columns in SQL Join Operations
List of SQL Types Supported by MySQL
MySQL supports various SQL data types, including numeric types, string types, date and time types, boolean types, binary types, spatial types, and more. Here is a list of some commonly used SQL data types in MySQL:
- INT: Used for storing integers.
- VARCHAR: Used for storing variable-length alphanumeric data.
- DATE: Used for storing dates.
- TIME: Used for storing times.
- BOOLEAN: Used for storing boolean values (true or false).
- BLOB: Used for storing binary large objects.
- GEOMETRY: Used for storing spatial data.
Working with Numeric Data Types in MySQL
Numeric data types in MySQL allow you to store numeric values, such as integers, decimals, and floating-point numbers. Some commonly used numeric data types in MySQL include INT, DECIMAL, FLOAT, and DOUBLE. Here are a few examples:
Example 1: Creating a table with an INT column:
CREATE TABLE employees ( id INT, name VARCHAR(100), age INT );
Example 2: Inserting data into the table:
INSERT INTO employees (id, name, age) VALUES (1, 'John', 25);
Working with String Data Types in MySQL
String data types in MySQL are used to store character data, such as names, addresses, and text. MySQL provides several string data types, including VARCHAR, CHAR, TEXT, and ENUM. Here are a couple of examples:
Example 1: Creating a table with a VARCHAR column:
CREATE TABLE customers ( id INT, name VARCHAR(100), email VARCHAR(100) );
Example 2: Inserting data into the table:
INSERT INTO customers (id, name, email) VALUES (1, 'Alice', 'alice@example.com');
Related Article: Updating JSONB Columns in PostgreSQL
Working with Date and Time Data Types in MySQL
Date and time data types in MySQL allow you to store and manipulate dates, times, and timestamps. Some commonly used date and time data types in MySQL include DATE, TIME, DATETIME, and TIMESTAMP. Here are a few examples:
Example 1: Creating a table with a DATE column:
CREATE TABLE orders ( id INT, order_date DATE, total_amount DECIMAL(10,2) );
Example 2: Inserting data into the table:
INSERT INTO orders (id, order_date, total_amount) VALUES (1, '2022-01-01', 100.50);
Working with Boolean Data Types in MySQL
Boolean data types in MySQL are used to store boolean values, which can be either true or false. In MySQL, boolean values are represented using the TINYINT(1) data type, where 1 represents true and 0 represents false. Here is an example:
Example: Creating a table with a BOOLEAN column:
CREATE TABLE students ( id INT, name VARCHAR(100), is_active TINYINT(1) );
Example: Inserting data into the table:
INSERT INTO students (id, name, is_active) VALUES (1, 'Mike', 1);
Working with Binary Data Types in MySQL
Binary data types in MySQL are used to store binary data, such as images, files, and serialized objects. MySQL provides several binary data types, including BLOB and VARBINARY. Here is an example:
Example: Creating a table with a BLOB column:
CREATE TABLE documents ( id INT, title VARCHAR(100), content BLOB );
Example: Inserting data into the table:
INSERT INTO documents (id, title, content) VALUES (1, 'Document 1', 'Binary data');
Working with Spatial Data Types in MySQL
Spatial data types in MySQL are used to store and manipulate geometric and geographic data. MySQL supports various spatial data types, such as POINT, LINESTRING, POLYGON, and GEOMETRY. Here is an example:
Example: Creating a table with a GEOMETRY column:
CREATE TABLE locations ( id INT, name VARCHAR(100), location GEOMETRY );
Example: Inserting data into the table:
INSERT INTO locations (id, name, location) VALUES (1, 'Location 1', ST_GeomFromText('POINT(10 20)'));
Related Article: How to Perform a Full Outer Join in MySQL
Using the UUID Data Type in MySQL
The UUID data type in MySQL allows you to store universally unique identifiers (UUIDs). UUIDs are 128-bit values that are unique across all devices and time. Here is an example:
Example: Creating a table with a UUID column:
CREATE TABLE users ( id UUID, name VARCHAR(100), email VARCHAR(100) );
Example: Inserting data into the table:
INSERT INTO users (id, name, email) VALUES (UUID(), 'John', 'john@example.com');
Using the XML Data Type in MySQL
The XML data type in MySQL allows you to store XML data. MySQL provides various functions and operators to manipulate and query XML data. Here is an example:
Example: Creating a table with an XML column:
CREATE TABLE books ( id INT, title VARCHAR(100), xml_data XML );
Example: Inserting data into the table:
INSERT INTO books (id, title, xml_data) VALUES (1, 'Book 1', '<book><author>John Doe</author></book>');
Using the JSON Data Type in MySQL
The JSON data type in MySQL allows you to store and manipulate JSON (JavaScript Object Notation) data. MySQL provides various functions and operators to work with JSON data. Here is an example:
Example: Creating a table with a JSON column:
CREATE TABLE products ( id INT, name VARCHAR(100), attributes JSON );
Example: Inserting data into the table:
INSERT INTO products (id, name, attributes) VALUES (1, 'Product 1', '{"color": "red", "size": "small"}');
MySQL Extensions for Additional Data Types
MySQL provides extensions for additional data types beyond the standard SQL data types. These extensions include data types for spatial data, range data, and more. Here are a few examples:
Example 1: Using the ENUM data type:
CREATE TABLE colors ( id INT, name ENUM('red', 'green', 'blue') );
Example 2: Using the SET data type:
CREATE TABLE toppings ( id INT, name SET('cheese', 'tomato', 'onion', 'mushroom') );
Related Article: How to Use the WHERE Condition in SQL Joins
Working with Geometric Data Types in MySQL
In addition to the basic spatial data types, MySQL also provides geometric data types for representing and manipulating geometric shapes. These geometric data types include POINT, LINESTRING, POLYGON, and more. Here is an example:
Example: Creating a table with a POINT column:
CREATE TABLE locations ( id INT, name VARCHAR(100), point POINT );
Example: Inserting data into the table:
INSERT INTO locations (id, name, point) VALUES (1, 'Location 1', POINT(10, 20));
Working with Range Data Types in MySQL
Range data types in MySQL allow you to store and query ranges of values. MySQL provides several range data types, such as INT ranges, DATE ranges, and more. Here is an example:
Example: Creating a table with an INT range column:
CREATE TABLE prices ( id INT, product VARCHAR(100), price INT RANGE );
Example: Inserting data into the table:
INSERT INTO prices (id, product, price) VALUES (1, 'Product 1', 10 TO 20);
Advanced Techniques for MySQL Data Types
MySQL supports various advanced techniques for working with data types, including type casting, type conversion, and type-specific functions. These techniques can be used to perform calculations, comparisons, and transformations on data. Here are a couple of examples:
Example 1: Type casting:
SELECT CAST('123' AS INT);
Example 2: Type conversion:
SELECT CONVERT('2022-01-01', DATE);
Code Snippet Ideas for MySQL Data Types
Here are a few code snippets that demonstrate some common operations and manipulations with MySQL data types:
Example 1: Retrieving the length of a string:
SELECT LENGTH('Hello, world!');
Example 2: Converting a string to uppercase:
SELECT UPPER('hello');
Related Article: Merging Two Result Values in SQL
Best Practices for Working with MySQL Data Types
When working with MySQL data types, it is important to follow these best practices to ensure data integrity and optimize performance:
1. Choose the appropriate data type for each column based on the nature of the data.
2. Avoid using excessively large data types for columns to conserve storage space.
3. Use the appropriate string length for VARCHAR columns to avoid wasted space.
4. Use the appropriate numeric type for numeric values to ensure accurate calculations.
5. Use the appropriate date and time type for storing date and time values.
6. Avoid using deprecated data types and features.
7. Regularly analyze and optimize the database schema to improve performance.
Real World Examples of MySQL Data Types
MySQL data types are widely used in various real-world applications. Here are a few examples of how MySQL data types can be used:
1. E-commerce websites use numeric data types to store product prices and quantities.
2. Social media platforms use string data types to store user names and messages.
3. Financial applications use date and time data types to store transaction timestamps.
4. Media storage platforms use binary data types to store multimedia files.
5. Mapping applications use spatial data types to store geographic coordinates.
Performance Considerations for MySQL Data Types
When designing a database schema in MySQL, it is important to consider the performance implications of the chosen data types. Here are a few performance considerations for MySQL data types:
1. Smaller data types generally have better performance and consume less storage space.
2. Avoid using excessive precision for numeric data types if it is not necessary.
3. Be cautious when using string data types with large maximum lengths, as they can impact performance and storage requirements.
4. Consider the indexing requirements of the data types used in the schema to optimize query performance.
5. Regularly analyze and optimize the database schema to improve performance.
Error Handling for MySQL Data Types
When working with MySQL data types, it is important to handle errors that may occur during data manipulation and query execution. Here are a few error handling techniques for MySQL data types:
1. Use appropriate error handling mechanisms, such as try-catch blocks, to handle exceptions in application code.
2. Validate user input to ensure it matches the expected data type before performing any database operations.
3. Use proper error reporting and logging mechanisms to identify and troubleshoot data type-related issues.
4. Familiarize yourself with common error codes and messages related to data types in MySQL to quickly identify and resolve issues.