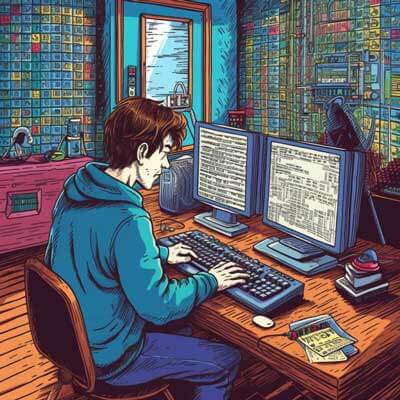
- Common JDBC Connection Issues
- 1. ClassNotFoundException
- 2. SQLException
- Troubleshooting MySQL Connection in Java
- 1. Verify MySQL Server Availability
- 2. Check Connection Parameters
- 3. Test Network Connectivity
- 4. Enable MySQL Server Logging
- 5. Use Connection Debugging Tools
- Identifying Driver Compatibility Problems
- 1. Check JDBC Driver Version
- 2. Upgrade or Downgrade the JDBC Driver
- 3. Verify MySQL Server Version
- 4. Consider Using a Driver Manager
- Handling Connection Errors and Exceptions
- 1. Handling SQLException
- 2. Handling ConnectionTimeoutException
- Testing Java-MySQL Connectivity
- 1. Using a Simple Connection Test
- 2. Using Database Connection Pooling
- Establishing a Secure Connection
- 1. Using SSL/TLS Encryption
- 2. Using SSH Tunneling
- Optimizing Java-MySQL Connection Performance
- 1. Use Connection Pooling
- 2. Use Prepared Statements
- Configuring Connection Pooling
- 1. Maximum Pool Size
- 2. Idle Timeout
- 3. Connection Timeout
- Working with Connection Timeouts
- 1. Configuring Connection Timeout
- 2. Handling Connection Timeout
- Handling Large Result Sets
- 1. Using Pagination
- 2. Using Streaming
- Implementing Batch Processing
- 1. Using Statement Batch
- 2. Using Prepared Statement Batch
- Using Prepared Statements
- 1. Creating a Prepared Statement
- 2. Using Prepared Statement with Batch Processing
- Managing Transactions
- 1. Starting a Transaction
- 2. Rolling Back a Transaction
- Logging Connection Events
- 1. Using a Logging Framework
- 2. Using System.out.println()
- Understanding Connection Parameters
- 1. JDBC URL
- 2. Username and Password
- Ensuring Data Integrity in Java-MySQL Connection
- 1. Using Transactions
- 2. Enforcing Constraints
- Securing the Connection Credentials
- 1. Use Environment Variables or Configuration Files
- 2. Encrypt the Connection Credentials
- Handling Connection Failover
- 1. Configuring Connection Failover
- 2. Using Connection Pooling Libraries
- Implementing Connection Retry Mechanism
- 1. Using a Retry Loop
- 2. Using a Retry Library
- Understanding Connection Pool Exhaustion
- 1. What is Connection Pool Exhaustion?
- 2. Handling Connection Pool Exhaustion
- Configuring Connection Encoding
- 1. Configuring Character Encoding
- 2. Handling Special Characters
- Additional Resources
Common JDBC Connection Issues
When working with Java and MySQL, it is not uncommon to encounter connection issues. These issues can arise due to various reasons, such as incorrect configuration, compatibility problems, or network connectivity. In this section, we will discuss some of the common JDBC connection issues you may encounter and how to troubleshoot them.
Related Article: How to Fix the java.lang.reflect.InvocationTargetException
1. ClassNotFoundException
One of the most common errors you may encounter when trying to establish a connection to MySQL in Java is the “ClassNotFoundException”. This error typically occurs when the MySQL JDBC driver is not found on the classpath. To resolve this issue, you will need to ensure that the MySQL JDBC driver is properly included in your project’s dependencies.
Here is an example of how to include the MySQL JDBC driver in a Maven project:
mysql mysql-connector-java 8.0.26
2. SQLException
Another common issue you may encounter is the “SQLException”. This exception is thrown when there is an error in the SQL syntax, connection failure, or other database-related errors. To troubleshoot this issue, you should carefully examine the exception message and stack trace to identify the specific cause of the error.
Here is an example of how to handle a SQLException when establishing a connection to MySQL:
try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); }
In this example, we catch the SQLException and print the error message and stack trace to help identify the cause of the connection failure.
Troubleshooting MySQL Connection in Java
Troubleshooting MySQL connection issues in Java can be a challenging task, but with the right approach, it can be resolved effectively. In this section, we will discuss some troubleshooting techniques to help you identify and resolve MySQL connection issues in your Java application.
Related Article: How to Resolve java.lang.ClassNotFoundException in Java
1. Verify MySQL Server Availability
The first step in troubleshooting MySQL connection issues is to verify the availability of the MySQL server. Ensure that the MySQL server is up and running by checking the server status or attempting to connect to it using a MySQL client tool such as MySQL Workbench.
2. Check Connection Parameters
Next, check the connection parameters used in your Java application. Verify that the hostname, port number, database name, username, and password are correct. It is common to make mistakes in these parameters, which can lead to connection failures.
3. Test Network Connectivity
If you are unable to establish a connection to the MySQL server from your Java application, test the network connectivity between the application server and the MySQL server. Ensure that there are no network firewalls or security groups blocking the connection.
Related Article: How To Fix the "java.lang.NoClassDefFoundError" Error
4. Enable MySQL Server Logging
Enabling logging on the MySQL server can help identify connection-related issues. Check the MySQL server logs for any error messages or warnings related to connections. The logs may provide valuable insights into the cause of the connection failures.
5. Use Connection Debugging Tools
There are several tools available that can help you debug MySQL connection issues in Java. One such tool is the MySQL Connector/J driver’s built-in connection debugging feature. By enabling this feature, you can get detailed debug information about the connection process, including the connection URL, driver version, and connection properties.
Here is an example of how to enable connection debugging with MySQL Connector/J:
import java.sql.DriverManager; import java.sql.SQLException; import java.util.Properties; public class Main { public static void main(String[] args) { try { DriverManager.setLogWriter(System.out); Properties properties = new Properties(); properties.setProperty("user", "username"); properties.setProperty("password", "password"); properties.setProperty("logger", "com.mysql.cj.log.Slf4JLogger"); properties.setProperty("profileSql", "true"); properties.setProperty("useUsageAdvisor", "true"); Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", properties); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we enable connection debugging by setting the “logger” property to “com.mysql.cj.log.Slf4JLogger” and the “profileSql” and “useUsageAdvisor” properties to “true”. This will print detailed debug information to the console.
Identifying Driver Compatibility Problems
When working with MySQL in Java, it is important to ensure that the JDBC driver you are using is compatible with the version of MySQL you are connecting to. In this section, we will discuss how to identify and resolve driver compatibility problems.
Related Article: How To Fix A NullPointerException In Java
1. Check JDBC Driver Version
The first step in identifying driver compatibility problems is to check the version of the JDBC driver you are using. The JDBC driver version should match the version of the MySQL server you are connecting to. Using an incompatible driver version can lead to connection failures or unexpected behavior.
2. Upgrade or Downgrade the JDBC Driver
If you discover that you are using an incompatible JDBC driver version, you may need to upgrade or downgrade the driver to the appropriate version. The MySQL website provides the official JDBC driver downloads, which you can use to obtain the correct version for your MySQL server.
3. Verify MySQL Server Version
In addition to checking the JDBC driver version, it is also important to verify the version of the MySQL server you are connecting to. The server version may have specific requirements or limitations that can affect the connection. Ensure that the server version is compatible with the JDBC driver you are using.
4. Consider Using a Driver Manager
If you are working with multiple databases or need to support different versions of MySQL, you may consider using a driver manager such as HikariCP or Apache Commons DBCP. These driver managers provide built-in support for managing JDBC drivers and can automatically load the appropriate driver based on the connection URL.
Here is an example of how to use HikariCP as a driver manager for MySQL:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we use the HikariConfig class to configure the connection properties and create a HikariDataSource. The HikariDataSource handles the loading and management of the JDBC driver, allowing you to focus on the database operations.
Handling Connection Errors and Exceptions
When working with MySQL in Java, it is important to handle connection errors and exceptions properly. In this section, we will discuss how to handle common connection errors and exceptions to ensure that your application handles them gracefully.
1. Handling SQLException
The SQLException class is the most common exception you will encounter when working with database connections in Java. It represents an error or warning that occurred on the database server. When handling SQLException, it is important to log the error message and stack trace for debugging purposes.
Here is an example of how to handle SQLException:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we catch the SQLException and print the error message and stack trace to the console.
2. Handling ConnectionTimeoutException
The ConnectionTimeoutException is thrown when a connection cannot be established within the specified timeout period. This exception can occur when the database server is busy or unresponsive. When handling ConnectionTimeoutException, you may want to retry the connection or display an error message to the user.
Here is an example of how to handle ConnectionTimeoutException:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { DriverManager.setLoginTimeout(5); // Set the connection timeout to 5 seconds Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { if (e instanceof ConnectionTimeoutException) { System.out.println("Connection timed out. Please try again later."); } else { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } } }
In this example, we set the connection timeout to 5 seconds using the setLoginTimeout() method. If the connection times out, we display an error message to the user.
Testing Java-MySQL Connectivity
Testing the connectivity between Java and MySQL is an essential step to ensure that your application can establish a connection to the database. In this section, we will discuss how to test Java-MySQL connectivity using various methods.
1. Using a Simple Connection Test
The simplest way to test Java-MySQL connectivity is by creating a minimal Java program that attempts to establish a connection to the database. If the connection is successful, you can assume that the connectivity is working.
Here is an example of a simple Java program to test MySQL connectivity:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); System.out.println("Connection successful!"); connection.close(); } catch (SQLException e) { System.out.println("Connection failed: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we attempt to establish a connection to the MySQL database using the getConnection() method. If the connection is successful, we print a success message to the console.
2. Using Database Connection Pooling
Another way to test Java-MySQL connectivity is by using a database connection pooling library such as HikariCP or Apache Commons DBCP. These libraries provide a built-in mechanism for testing database connectivity.
Here is an example of how to use HikariCP to test MySQL connectivity:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setMaximumPoolSize(1); // Set the maximum pool size to 1 for testing config.setInitializationFailFast(true); // Fail fast if the connection cannot be established try (HikariDataSource dataSource = new HikariDataSource(config)) { Connection connection = dataSource.getConnection(); System.out.println("Connection successful!"); connection.close(); } catch (SQLException e) { System.out.println("Connection failed: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we configure HikariCP with the necessary connection parameters and set the maximum pool size to 1 for testing purposes. We also enable the “InitializationFailFast” property to fail fast if the connection cannot be established. If the connection is successful, we print a success message to the console.
Establishing a Secure Connection
When connecting to a MySQL database in Java, it is important to establish a secure connection to protect sensitive data and prevent unauthorized access. In this section, we will discuss how to establish a secure connection between Java and MySQL.
1. Using SSL/TLS Encryption
One way to establish a secure connection between Java and MySQL is by using SSL/TLS encryption. SSL (Secure Sockets Layer) and its successor TLS (Transport Layer Security) provide a secure channel for data transmission over the network.
To use SSL/TLS encryption, you will need to configure the MySQL server to support SSL/TLS connections and provide the necessary SSL certificates. Once the server is properly configured, you can enable SSL/TLS encryption in your Java application.
Here is an example of how to establish an SSL/TLS encrypted connection to MySQL in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.Properties; public class Main { public static void main(String[] args) { try { Properties properties = new Properties(); properties.setProperty("user", "username"); properties.setProperty("password", "password"); properties.setProperty("useSSL", "true"); properties.setProperty("requireSSL", "true"); properties.setProperty("verifyServerCertificate", "true"); Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", properties); System.out.println("Secure connection established!"); connection.close(); } catch (SQLException e) { System.out.println("Connection failed: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we set the “useSSL”, “requireSSL”, and “verifyServerCertificate” properties to enable SSL/TLS encryption and verify the server’s SSL certificate. If the connection is established successfully, we print a success message to the console.
2. Using SSH Tunneling
Another way to establish a secure connection between Java and MySQL is by using SSH tunneling. SSH (Secure Shell) allows you to create an encrypted connection between your local machine and a remote server. By creating an SSH tunnel, you can securely connect to the MySQL server from your Java application.
To use SSH tunneling, you will need to have SSH access to the server hosting the MySQL database. Once you have established an SSH connection, you can configure your Java application to connect to the MySQL server through the SSH tunnel.
Here is an example of how to establish an SSH tunnel and connect to MySQL in Java:
import com.jcraft.jsch.JSch; import com.jcraft.jsch.JSchException; import com.jcraft.jsch.Session; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.Properties; public class Main { public static void main(String[] args) { try { JSch jSch = new JSch(); Session session = jSch.getSession("username", "ssh.example.com", 22); session.setPassword("password"); session.setConfig("StrictHostKeyChecking", "no"); session.connect(); int localPort = 3307; // Local port to bind the SSH tunnel int remotePort = 3306; // Remote port of the MySQL server session.setPortForwardingL(localPort, "localhost", remotePort); Properties properties = new Properties(); properties.setProperty("user", "username"); properties.setProperty("password", "password"); Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:" + localPort + "/mydatabase", properties); System.out.println("Secure connection established!"); connection.close(); session.disconnect(); } catch (JSchException | SQLException e) { System.out.println("Connection failed: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use the JSch library to create an SSH tunnel to the MySQL server. We set the SSH server hostname, username, and password, and configure the session to disable strict host key checking. We then establish the SSH tunnel by binding a local port to the remote MySQL server’s port.
Once the SSH tunnel is established, we can connect to MySQL using the local port and perform database operations. If the connection is established successfully, we print a success message to the console.
Optimizing Java-MySQL Connection Performance
Optimizing the performance of your Java-MySQL connection is essential to ensure that your application can handle a high volume of database operations efficiently. In this section, we will discuss some strategies for optimizing the performance of your Java-MySQL connection.
1. Use Connection Pooling
Using connection pooling can significantly improve the performance of your Java-MySQL connection by reusing existing connections instead of creating a new connection for every database operation. Connection pooling libraries such as HikariCP or Apache Commons DBCP provide built-in support for connection pooling.
Here is an example of how to use HikariCP for connection pooling in Java:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setMaximumPoolSize(10); // Set the maximum pool size HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we configure HikariCP with the necessary connection parameters and set the maximum pool size to 10. The connection pool will automatically manage the connections, allowing you to reuse them for multiple database operations.
2. Use Prepared Statements
Using prepared statements instead of regular statements can improve the performance of your Java-MySQL connection by reducing the overhead of query parsing and optimization. Prepared statements allow you to precompile SQL statements and reuse them with different parameter values.
Here is an example of how to use prepared statements in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { String sql = "SELECT * FROM users WHERE id = ?"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setInt(1, 1); // Set the parameter value try (ResultSet resultSet = statement.executeQuery()) { while (resultSet.next()) { String name = resultSet.getString("name"); int age = resultSet.getInt("age"); System.out.println("Name: " + name + ", Age: " + age); } } } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use a prepared statement to execute a parameterized query. We set the parameter value using the setInt() method and execute the query using the executeQuery() method. The result set is then processed to retrieve the data.
Using prepared statements can improve performance and also help prevent SQL injection attacks by separating the SQL logic from the parameter values.
Configuring Connection Pooling
Configuring connection pooling properly is crucial for optimizing the performance and scalability of your Java-MySQL connection. In this section, we will discuss some important configurations for connection pooling and how to set them up.
1. Maximum Pool Size
The maximum pool size determines the maximum number of connections the connection pool can hold. Setting an appropriate maximum pool size is crucial to ensure that your application can handle the expected workload efficiently.
Here is an example of how to configure the maximum pool size in HikariCP:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setMaximumPoolSize(10); // Set the maximum pool size HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we set the maximum pool size to 10 using the setMaximumPoolSize() method. This means that the connection pool can hold a maximum of 10 connections.
2. Idle Timeout
The idle timeout determines how long an idle connection can remain in the pool before it is closed and removed. Setting an appropriate idle timeout is crucial to prevent resource waste and ensure that connections are released when they are no longer needed.
Here is an example of how to configure the idle timeout in HikariCP:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setIdleTimeout(60000); // Set the idle timeout to 60 seconds HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we set the idle timeout to 60 seconds using the setIdleTimeout() method. This means that if a connection remains idle for more than 60 seconds, it will be closed and removed from the pool.
3. Connection Timeout
The connection timeout determines how long the connection pool will wait for a new connection to become available before throwing an exception. Setting an appropriate connection timeout is crucial to prevent long waiting times and ensure that your application can handle peak loads efficiently.
Here is an example of how to configure the connection timeout in HikariCP:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setConnectionTimeout(5000); // Set the connection timeout to 5 seconds HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we set the connection timeout to 5 seconds using the setConnectionTimeout() method. This means that if a connection is not available within 5 seconds, an exception will be thrown.
Working with Connection Timeouts
Working with connection timeouts is crucial to ensure that your Java application can handle unexpected delays or unresponsive database servers. In this section, we will discuss how to configure and handle connection timeouts in your Java-MySQL connection.
1. Configuring Connection Timeout
The connection timeout determines how long the connection pool will wait for a new connection to become available before throwing an exception. Configuring an appropriate connection timeout is crucial to prevent long waiting times and ensure that your application can handle peak loads efficiently.
Here is an example of how to configure the connection timeout in HikariCP:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setConnectionTimeout(5000); // Set the connection timeout to 5 seconds HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we set the connection timeout to 5 seconds using the setConnectionTimeout() method. This means that if a connection is not available within 5 seconds, an exception will be thrown.
2. Handling Connection Timeout
When a connection timeout occurs, it is important to handle it gracefully and provide appropriate feedback to the user. You can catch the SQLException and handle the connection timeout exception separately from other exceptions.
Here is an example of how to handle connection timeout in your Java application:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { DriverManager.setLoginTimeout(5); // Set the connection timeout to 5 seconds Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { if (e instanceof SQLTimeoutException) { System.out.println("Connection timed out. Please try again later."); } else { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } } }
In this example, we catch the SQLException and check if it is an instance of SQLTimeoutException, which is a subclass of SQLException specifically for connection timeouts. If a connection timeout occurs, we display an appropriate error message to the user.
Handling Large Result Sets
Handling large result sets in your Java-MySQL connection is crucial to ensure that your application can efficiently process and manipulate large amounts of data. In this section, we will discuss some strategies for handling large result sets in your Java-MySQL connection.
1. Using Pagination
One way to handle large result sets is by using pagination, which involves fetching a subset of the result set at a time. By retrieving data in smaller chunks, you can reduce memory usage and improve performance.
Here is an example of how to use pagination to handle large result sets in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { String sql = "SELECT * FROM users"; int pageSize = 100; // Number of rows per page int pageNumber = 1; // Page number try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setMaxRows(pageSize); statement.setFetchSize(pageSize); statement.setFetchDirection(ResultSet.FETCH_FORWARD); try (ResultSet resultSet = statement.executeQuery()) { resultSet.absolute((pageNumber - 1) * pageSize); while (resultSet.next()) { String name = resultSet.getString("name"); int age = resultSet.getInt("age"); System.out.println("Name: " + name + ", Age: " + age); } } } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use the setMaxRows(), setFetchSize(), and setFetchDirection() methods to configure the pagination settings. We then use the absolute() method of the ResultSet to navigate to the desired page in the result set.
2. Using Streaming
Another way to handle large result sets is by using streaming, which involves processing the result set in a streaming manner instead of loading the entire result set into memory. Streaming is particularly useful when dealing with large amounts of data.
Here is an example of how to use streaming to handle large result sets in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { Statement statement = connection.createStatement(); statement.setFetchSize(Integer.MIN_VALUE); // Enable streaming ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); while (resultSet.next()) { String name = resultSet.getString("name"); int age = resultSet.getInt("age"); System.out.println("Name: " + name + ", Age: " + age); } resultSet.close(); statement.close(); } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we set the fetch size to Integer.MIN_VALUE to enable streaming. This allows the result set to be processed in a streaming manner, fetching data from the database as needed.
Implementing Batch Processing
Batch processing allows you to execute multiple SQL statements as a batch, reducing the number of round trips between your Java application and the MySQL database. In this section, we will discuss how to implement batch processing in your Java-MySQL connection.
1. Using Statement Batch
One way to implement batch processing is by using the addBatch() and executeBatch() methods of the Statement class. The addBatch() method adds a SQL statement to the batch, and the executeBatch() method executes all the statements in the batch.
Here is an example of how to use statement batch processing in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { Statement statement = connection.createStatement(); statement.addBatch("INSERT INTO users (name, age) VALUES ('John', 30)"); statement.addBatch("INSERT INTO users (name, age) VALUES ('Jane', 25)"); int[] updateCounts = statement.executeBatch(); for (int updateCount : updateCounts) { System.out.println("Rows affected: " + updateCount); } statement.close(); } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use the addBatch() method to add two INSERT statements to the batch. We then use the executeBatch() method to execute the batch, which returns an array of update counts indicating the number of rows affected by each statement.
2. Using Prepared Statement Batch
Another way to implement batch processing is by using the addBatch() and executeBatch() methods of the PreparedStatement class. The addBatch() method adds a set of parameters to the batch, and the executeBatch() method executes all the batches.
Here is an example of how to use prepared statement batch processing in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { String sql = "INSERT INTO users (name, age) VALUES (?, ?)"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setString(1, "John"); statement.setInt(2, 30); statement.addBatch(); statement.setString(1, "Jane"); statement.setInt(2, 25); statement.addBatch(); int[] updateCounts = statement.executeBatch(); for (int updateCount : updateCounts) { System.out.println("Rows affected: " + updateCount); } } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use a prepared statement with placeholders for the values. We set the parameter values using the setString() and setInt() methods, and then add the batch using the addBatch() method. We then use the executeBatch() method to execute the batch.
Using Prepared Statements
Using prepared statements in your Java-MySQL connection is crucial to ensure the security and efficiency of your database operations. In this section, we will discuss how to use prepared statements in your Java-MySQL connection.
1. Creating a Prepared Statement
To create a prepared statement, you need to pass the SQL statement with placeholders to the prepareStatement() method of the Connection class. Placeholders are represented by question marks (?) and are used to specify the parameters.
Here is an example of how to create a prepared statement in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { String sql = "SELECT * FROM users WHERE age > ?"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setInt(1, 30); // Set the parameter value try (ResultSet resultSet = statement.executeQuery()) { while (resultSet.next()) { String name = resultSet.getString("name"); int age = resultSet.getInt("age"); System.out.println("Name: " + name + ", Age: " + age); } } } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we create a prepared statement to select all users whose age is greater than the specified parameter. We set the parameter value using the setInt() method and execute the query using the executeQuery() method. The result set is then processed to retrieve the data.
Using prepared statements helps prevent SQL injection attacks by separating the SQL logic from the parameter values.
2. Using Prepared Statement with Batch Processing
Prepared statements can also be used with batch processing to execute multiple SQL statements efficiently. By reusing the prepared statement with different parameter values, you can reduce the overhead of parsing and optimizing the SQL statements.
Here is an example of how to use prepared statement with batch processing in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { String sql = "INSERT INTO users (name, age) VALUES (?, ?)"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setString(1, "John"); statement.setInt(2, 30); statement.addBatch(); statement.setString(1, "Jane"); statement.setInt(2, 25); statement.addBatch(); int[] updateCounts = statement.executeBatch(); for (int updateCount : updateCounts) { System.out.println("Rows affected: " + updateCount); } } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we create a prepared statement to insert multiple users into the database. We set the parameter values using the setString() and setInt() methods and add the batch using the addBatch() method. We then use the executeBatch() method to execute the batch.
Using prepared statements with batch processing can improve performance and reduce the number of round trips between your Java application and the MySQL database.
Managing Transactions
Managing transactions in your Java-MySQL connection is crucial to ensure the consistency and integrity of your database operations. In this section, we will discuss how to manage transactions in your Java-MySQL connection.
1. Starting a Transaction
To start a transaction, you need to disable the auto-commit mode of the connection by calling the setAutoCommit() method with a value of false. This allows you to group multiple SQL statements into a single transaction.
Here is an example of how to start a transaction in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { connection.setAutoCommit(false); // Disable auto-commit String sql = "UPDATE users SET age = ? WHERE id = ?"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setInt(1, 30); statement.setInt(2, 1); statement.executeUpdate(); statement.setInt(1, 25); statement.setInt(2, 2); statement.executeUpdate(); connection.commit(); // Commit the transaction } catch (SQLException e) { connection.rollback(); // Rollback the transaction System.out.println("An error occurred while executing the SQL statements: " + e.getMessage()); e.printStackTrace(); } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we disable the auto-commit mode of the connection by calling the setAutoCommit() method with a value of false. We then execute multiple SQL statements within the transaction using the executeUpdate() method. Finally, we commit the transaction by calling the commit() method or rollback the transaction if an error occurs.
2. Rolling Back a Transaction
If an error occurs during the execution of a transaction, you can roll back the transaction to undo the changes made by the SQL statements. To roll back a transaction, you need to call the rollback() method of the connection.
Here is an example of how to roll back a transaction in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { connection.setAutoCommit(false); // Disable auto-commit String sql = "UPDATE users SET age = ? WHERE id = ?"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setInt(1, 30); statement.setInt(2, 1); statement.executeUpdate(); statement.setInt(1, 25); statement.setInt(2, 2); statement.executeUpdate(); // Simulate an error throw new SQLException("An error occurred"); } catch (SQLException e) { connection.rollback(); // Rollback the transaction System.out.println("An error occurred while executing the SQL statements: " + e.getMessage()); e.printStackTrace(); } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we simulate an error by throwing a SQLException after executing the SQL statements. In the catch block, we call the rollback() method to roll back the transaction and undo the changes made by the SQL statements.
Logging Connection Events
Logging connection events in your Java-MySQL connection is crucial for troubleshooting and monitoring purposes. In this section, we will discuss how to log connection events in your Java-MySQL connection.
1. Using a Logging Framework
One way to log connection events in your Java-MySQL connection is by using a logging framework such as Log4j or SLF4J. These frameworks provide a standardized way to log events and can be easily integrated into your Java application.
Here is an example of how to log connection events using Log4j in Java:
import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { private static final Logger logger = LogManager.getLogger(Main.class); public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { logger.info("Connection established"); // Perform database operations } catch (SQLException e) { logger.error("An error occurred while connecting to the database: " + e.getMessage(), e); } } }
In this example, we use Log4j to log connection events. We create a logger instance using the LogManager.getLogger() method and use the info() and error() methods to log connection events.
2. Using System.out.println()
Another way to log connection events in your Java-MySQL connection is by using the System.out.println() method. Although not as sophisticated as a logging framework, this method can be useful for basic logging and debugging purposes.
Here is an example of how to log connection events using System.out.println() in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { System.out.println("Connection established"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use System.out.println() to log connection events. We print the connection established message to the console using the println() method.
Using System.out.println() can be a quick and simple way to log connection events, but it lacks the flexibility and configurability of a logging framework.
Understanding Connection Parameters
Understanding the connection parameters used in your Java-MySQL connection is crucial to ensure that your application can establish a successful connection. In this section, we will discuss some common connection parameters and their significance.
1. JDBC URL
The JDBC URL is a string that specifies the location and other properties of the MySQL database you want to connect to. It consists of a protocol, hostname, port number, database name, and optional parameters.
Here is an example of a JDBC URL:
jdbc:mysql://localhost:3306/mydatabase?useSSL=false
In this example, the JDBC URL specifies the protocol (jdbc:mysql://), hostname (localhost), port number (3306), and database name (mydatabase). The useSSL=false parameter is an optional parameter that disables SSL/TLS encryption.
The JDBC URL is a fundamental connection parameter that must be correctly configured to establish a successful connection to the MySQL database.
2. Username and Password
The username and password are used to authenticate the user with the MySQL database. The username and password must be provided in order to establish a connection.
Here is an example of how to set the username and password in a Java-MySQL connection:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we set the username and password as the second and third parameters of the getConnection() method.
The username and password are essential connection parameters that must be provided to authenticate with the MySQL database.
Ensuring Data Integrity in Java-MySQL Connection
Ensuring data integrity in your Java-MySQL connection is crucial to maintain the accuracy and consistency of your database. In this section, we will discuss some strategies for ensuring data integrity in your Java-MySQL connection.
1. Using Transactions
Using transactions is a fundamental strategy for ensuring data integrity in your Java-MySQL connection. By grouping multiple SQL statements into a single transaction, you can ensure that either all the statements are executed successfully or none of them are executed.
Here is an example of how to use transactions in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Main { public static void main(String[] args) { try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) { connection.setAutoCommit(false); // Disable auto-commit String sql = "UPDATE users SET age = ? WHERE id = ?"; try (PreparedStatement statement = connection.prepareStatement(sql)) { statement.setInt(1, 30); statement.setInt(2, 1); statement.executeUpdate(); statement.setInt(1, 25); statement.setInt(2, 2); statement.executeUpdate(); connection.commit(); // Commit the transaction } catch (SQLException e) { connection.rollback(); // Rollback the transaction System.out.println("An error occurred while executing the SQL statements: " + e.getMessage()); e.printStackTrace(); } } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we disable the auto-commit mode of the connection by calling the setAutoCommit() method with a value of false. We then execute multiple SQL statements within the transaction using the executeUpdate() method. Finally, we commit the transaction by calling the commit() method or rollback the transaction if an error occurs.
2. Enforcing Constraints
Enforcing constraints is another strategy for ensuring data integrity in your Java-MySQL connection. Constraints are rules that define the relationships and dependencies between tables and columns in the database.
Here are some common types of constraints that can be enforced in a MySQL database:
– Primary Key: Ensures that each row in a table is uniquely identified by a primary key value.
– Foreign Key: Enforces referential integrity by ensuring that values in a column of one table match values in a column of another table.
– Unique Constraint: Ensures that the values in a column or a group of columns are unique.
– Not Null Constraint: Ensures that a column does not contain null values.
– Check Constraint: Defines a condition that must be satisfied by the values in a column.
Securing the Connection Credentials
Securing the connection credentials in your Java-MySQL connection is crucial to protect sensitive information and prevent unauthorized access to your database. In this section, we will discuss some strategies for securing the connection credentials in your Java-MySQL connection.
1. Use Environment Variables or Configuration Files
One way to secure the connection credentials is by using environment variables or configuration files to store the credentials outside of your source code. Storing the credentials separately from your code helps prevent accidental exposure and makes it easier to manage and update the credentials.
Here is an example of how to use environment variables to store the connection credentials in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { String jdbcUrl = System.getenv("JDBC_URL"); String username = System.getenv("JDBC_USERNAME"); String password = System.getenv("JDBC_PASSWORD"); try { Connection connection = DriverManager.getConnection(jdbcUrl, username, password); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we use the System.getenv() method to retrieve the connection credentials from environment variables. The JDBC_URL, JDBC_USERNAME, and JDBC_PASSWORD environment variables should be set externally, outside of the Java code.
2. Encrypt the Connection Credentials
Another way to secure the connection credentials is by encrypting the credentials before storing them. Encryption ensures that even if the credentials are compromised, they cannot be easily deciphered by unauthorized individuals.
Here is an example of how to encrypt the connection credentials in Java:
import java.security.Key; import java.util.Base64; import javax.crypto.Cipher; import javax.crypto.spec.SecretKeySpec; public class Main { public static void main(String[] args) { String jdbcUrl = decrypt("encryptedJdbcUrl"); String username = decrypt("encryptedUsername"); String password = decrypt("encryptedPassword"); // ... } private static String decrypt(String encryptedValue) { try { Key key = new SecretKeySpec("encryptionKey".getBytes(), "AES"); Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding"); cipher.init(Cipher.DECRYPT_MODE, key); byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedValue)); return new String(decryptedBytes); } catch (Exception e) { e.printStackTrace(); return null; } } }
In this example, we use the AES encryption algorithm to encrypt and decrypt the connection credentials. The encryption key should be securely stored and not hard-coded in the code.
Handling Connection Failover
Handling connection failover in your Java-MySQL connection is crucial to ensure high availability and uninterrupted access to your database. In this section, we will discuss how to handle connection failover in your Java-MySQL connection.
1. Configuring Connection Failover
To configure connection failover in your Java-MySQL connection, you need to specify multiple hosts in the JDBC URL. If the primary host becomes unavailable, the connection will automatically fail over to the next available host.
Here is an example of how to configure connection failover in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://host1,host2,host3:3306/mydatabase", "username", "password"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we specify multiple hosts (host1, host2, and host3) separated by commas in the JDBC URL. If the connection to the primary host fails, the connection will automatically fail over to the next available host.
2. Using Connection Pooling Libraries
Using connection pooling libraries such as HikariCP or Apache Commons DBCP can also help with connection failover. These libraries provide built-in support for connection failover by automatically retrying failed connections and switching to the next available host.
Here is an example of how to use HikariCP with connection failover in Java:
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; import java.sql.Connection; import java.sql.SQLException; public class Main { public static void main(String[] args) { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://host1,host2,host3:3306/mydatabase"); config.setUsername("username"); config.setPassword("password"); config.setConnectionTimeout(5000); // Set the connection timeout to 5 seconds config.setAutoCommit(true); // Enable auto-commit HikariDataSource dataSource = new HikariDataSource(config); try (Connection connection = dataSource.getConnection()) { // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } finally { dataSource.close(); } } }
In this example, we configure HikariCP with the necessary connection parameters, including the multiple hosts in the JDBC URL. HikariCP will automatically handle connection failover by retrying failed connections and switching to the next available host.
Implementing Connection Retry Mechanism
Implementing a connection retry mechanism in your Java-MySQL connection is crucial to handle temporary connection failures and ensure high availability of your application. In this section, we will discuss how to implement a connection retry mechanism in your Java-MySQL connection.
1. Using a Retry Loop
One way to implement a connection retry mechanism is by using a retry loop that attempts to establish a connection multiple times with a delay between each attempt. If the connection fails, the loop will retry the connection until a successful connection is established or the maximum number of retries is reached.
Here is an example of how to implement a connection retry mechanism in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { int maxRetries = 3; int retryDelay = 5000; // 5 seconds for (int retryCount = 1; retryCount <= maxRetries; retryCount++) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password"); System.out.println("Connection established!"); // Perform database operations break; // Connection successful, exit the retry loop } catch (SQLException e) { System.out.println("Connection failed (attempt " + retryCount + "/" + maxRetries + "): " + e.getMessage()); e.printStackTrace(); if (retryCount < maxRetries) { try { Thread.sleep(retryDelay); } catch (InterruptedException ex) { Thread.currentThread().interrupt(); } } } } } }
In this example, we use a for loop to attempt the connection multiple times. If the connection fails, we display an error message and print the stack trace. We then wait for the specified delay using the Thread.sleep() method before retrying the connection.
2. Using a Retry Library
Another way to implement a connection retry mechanism is by using a retry library such as Resilience4j or Failsafe. These libraries provide built-in support for implementing retries with various configurable options, such as maximum number of retries, delay between retries, and exponential backoff.
Here is an example of how to use Resilience4j to implement a connection retry mechanism in Java:
import io.github.resilience4j.retry.Retry; import io.github.resilience4j.retry.RetryConfig; import io.github.resilience4j.retry.RetryRegistry; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.time.Duration; public class Main { public static void main(String[] args) { RetryConfig config = RetryConfig.custom() .maxAttempts(3) .waitDuration(Duration.ofSeconds(5)) .build(); RetryRegistry registry = RetryRegistry.of(config); Retry retry = registry.retry("myRetry"); try { Connection connection = retry.executeCheckedSupplier(() -> DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password") ); System.out.println("Connection established!"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we configure the RetryConfig with the maximum number of retries (3) and the wait duration between retries (5 seconds). We then create a RetryRegistry and a Retry instance with the specified configuration. Finally, we use the retry.executeCheckedSupplier() method to execute the connection code within the retry mechanism.
Understanding Connection Pool Exhaustion
Understanding connection pool exhaustion in your Java-MySQL connection is crucial to ensure that your application can handle a high volume of database operations efficiently. In this section, we will discuss connection pool exhaustion and how to handle it in your Java-MySQL connection.
1. What is Connection Pool Exhaustion?
Connection pool exhaustion occurs when all the connections in the connection pool are in use and no more connections are available for new requests. This can happen when the number of concurrent requests exceeds the maximum pool size or when connections are not released properly after use.
When connection pool exhaustion occurs, new requests for connections may be queued or rejected, leading to delays or errors in your application.
2. Handling Connection Pool Exhaustion
To handle connection pool exhaustion in your Java-MySQL connection, you can take the following steps:
– Increase the maximum pool size: If you frequently experience connection pool exhaustion, you may need to increase the maximum pool size to handle the increased load. However, be cautious as increasing the pool size can put more strain on the database server and may not always be the most efficient solution.
– Optimize database operations: Review your database operations and optimize them to minimize the time spent on each operation. This can help reduce the number of connections needed and improve overall performance.
– Use connection timeout: Configure a connection timeout to limit the time a connection can be held by a request. This can help prevent connections from being held for too long and improve overall connection availability.
– Implement connection monitoring: Implement connection monitoring to track the usage and availability of connections in the pool. This can help identify any patterns or issues that may lead to connection pool exhaustion.
– Handle connection leaks: Ensure that connections are properly released after use to prevent connection leaks. Connection leaks occur when connections are not closed or returned to the pool, leading to a gradual depletion of available connections.
– Implement connection eviction: Implement connection eviction to remove idle or expired connections from the pool. This can help free up resources and improve overall connection availability.
Configuring Connection Encoding
Configuring connection encoding in your Java-MySQL connection is crucial to ensure that your application can handle different character encodings and properly handle special characters. In this section, we will discuss how to configure connection encoding in your Java-MySQL connection.
1. Configuring Character Encoding
To configure character encoding in your Java-MySQL connection, you need to set the characterEncoding parameter in the JDBC URL to the desired encoding. The characterEncoding parameter specifies the character encoding used for sending and receiving data between the Java application and the MySQL database.
Here is an example of how to configure character encoding in Java:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class Main { public static void main(String[] args) { try { Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase?characterEncoding=UTF-8", "username", "password"); // Perform database operations } catch (SQLException e) { System.out.println("An error occurred while connecting to the database: " + e.getMessage()); e.printStackTrace(); } } }
In this example, we set the characterEncoding parameter to UTF-8, which is a common encoding for Unicode characters. This ensures that the Java application and the MySQL database can properly handle special characters and different languages.
2. Handling Special Characters
When handling special characters in your Java-MySQL connection, it is important to ensure that the character encoding is properly configured, both in the Java application and the MySQL database.
Here are some best practices for handling special characters in your Java-MySQL connection:
– Set the character encoding consistently: Ensure that the character encoding is set consistently in both the Java application and the MySQL database. Inconsistent character encoding can lead to data corruption and display issues.
– Use parameterized queries: When using SQL statements with user input, always use parameterized queries to prevent SQL injection attacks and properly handle special characters. Parameterized queries separate the SQL logic from the parameter values, ensuring that special characters are handled correctly.
– Validate and sanitize user input: Validate and sanitize user input to ensure that it conforms to the expected character encoding and prevent unexpected behavior. Reject or sanitize user input that contains special characters or non-ASCII characters, depending on your application’s requirements.
– Use proper data types: Use the appropriate data types in your MySQL database to store special characters. For example, use VARCHAR or TEXT for strings that may contain special characters, and use proper data types for numeric or date/time values to ensure correct encoding and storage.
Additional Resources
– How to Connect to a MySQL Database in Java
– Java MySQL Connection Example
– Common Exceptions in JDBC