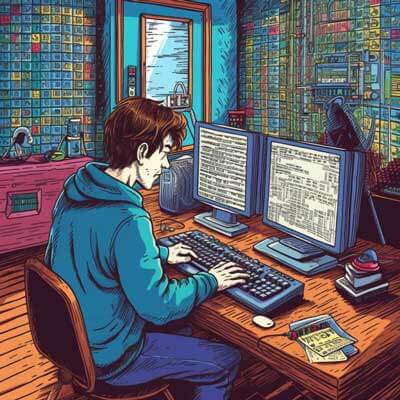
Adding a legend to a matplotlib plot in Python is a simple and effective way to provide additional information about the elements displayed in the plot. The legend can help viewers understand the meaning of different colors, markers, or line styles used in the plot. In this guide, we will explore two different methods to add a legend to a matplotlib plot in Python.
Method 1: Using the label parameter
The first method involves using the label
parameter when plotting the elements of the graph. This parameter allows you to assign a name or a description to the plotted element, which will be used in the legend.
Here is an example that demonstrates how to add a legend using the label
parameter:
import matplotlib.pyplot as plt # Generate some random data x = [1, 2, 3, 4, 5] y1 = [1, 4, 9, 16, 25] y2 = [1, 8, 27, 64, 125] # Plot the data with labels plt.plot(x, y1, label='Square') plt.plot(x, y2, label='Cube') # Add a legend plt.legend() # Display the plot plt.show()
In the above example, we first import the matplotlib.pyplot
module. Then, we generate two sets of random data y1
and y2
corresponding to the square and cube of the values in x
. We plot the data using the plot()
function and provide a label for each plotted element using the label
parameter. Finally, we call the legend()
function to add the legend to the plot.
Related Article: How To Exit Python Virtualenv
Method 2: Using the handles and labels parameters
The second method involves using the handles
and labels
parameters of the legend()
function. This method provides more flexibility and control over the legend content and appearance.
Here is an example that demonstrates how to add a legend using the handles
and labels
parameters:
import matplotlib.pyplot as plt from matplotlib.lines import Line2D # Generate some random data x = [1, 2, 3, 4, 5] y1 = [1, 4, 9, 16, 25] y2 = [1, 8, 27, 64, 125] # Plot the data without labels line1, = plt.plot(x, y1) line2, = plt.plot(x, y2) # Create custom legend handles and labels legend_handles = [Line2D([0], [0], color='blue', lw=2), Line2D([0], [0], color='red', lw=2)] legend_labels = ['Square', 'Cube'] # Add a legend with custom handles and labels plt.legend(legend_handles, legend_labels) # Display the plot plt.show()
In the above example, we first import the matplotlib.pyplot
module and the Line2D
class from the matplotlib.lines
module. Then, we generate two sets of random data y1
and y2
corresponding to the square and cube of the values in x
. We plot the data without labels using the plot()
function and assign the returned line objects to line1
and line2
. Next, we create custom legend handles using the Line2D
class and assign them to legend_handles
. We also define custom legend labels and assign them to legend_labels
. Finally, we call the legend()
function with the custom handles and labels to add the legend to the plot.
Best practices
When adding a legend to a matplotlib plot in Python, it is important to consider the following best practices:
1. Provide descriptive labels: Use labels that accurately describe the elements being plotted. This will help viewers understand the meaning of each element in the legend.
2. Position the legend appropriately: Place the legend in a position that does not obstruct important elements of the plot. Common positions include “upper right”, “upper left”, “lower right”, and “lower left”.
3. Adjust the legend font size: If the default font size is too small or too large, adjust the font size using the fontsize
parameter of the legend()
function.
4. Customize the legend appearance: Use the various parameters of the legend()
function to customize the appearance of the legend, such as the border color, background color, and transparency.
These best practices will help ensure that the legend is clear, legible, and enhances the understanding of the plot.
Related Article: How to Integrate Python with MySQL for Database Queries