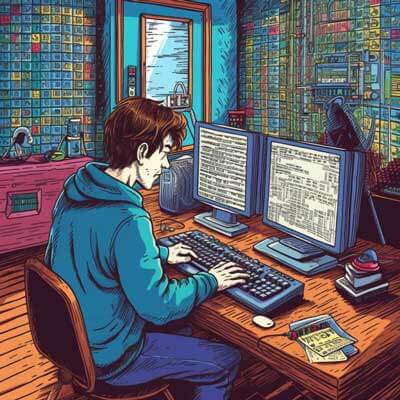
- What is Unstructured Data?
- Importance of Structuring Unstructured Data
- Challenges of Working with Unstructured Data
- 1. Data Volume
- 2. Data Variety
- 3. Data Quality
- 4. Data Complexity
- 5. Data Integration
- Methods for Structuring Unstructured Data
- 1. Data Cleaning Techniques
- 2. Data Analysis Approaches
- 3. Data Manipulation Strategies
- 4. Data Preprocessing Methods
- 5. Data Extraction Techniques
- 6. Data Transformation Approaches
- 7. Data Normalization Techniques
- 8. Common Techniques for Data Structuring
- Libraries and Packages for Structuring Unstructured Data
- Code Snippets for Structuring Unstructured Data
- Example 1: Text Preprocessing using NLTK
- Example 2: Named Entity Recognition using spaCy
- Example 3: Data Deduplication using pandas
- Step-by-Step Guide to Structuring Unstructured Data
- Step 1: Understand the Data
- Step 2: Define Objectives
- Step 3: Choose Techniques and Tools
- Step 4: Clean and Preprocess the Data
- Step 5: Perform Data Analysis
- Step 6: Manipulate and Transform the Data
- Step 7: Validate and Verify the Structured Data
- Step 8: Document the Structuring Process
- Step 9: Continuously Monitor and Update the Structured Data
- Additional Resources
What is Unstructured Data?
Unstructured data refers to any data that does not adhere to a predefined data model or format. Unlike structured data, which is organized in a tabular or hierarchical manner, unstructured data does not have a specific schema or organization. Examples of unstructured data include text documents, emails, social media posts, images, videos, audio recordings, and sensor data.
Unstructured data is typically more challenging to analyze and process compared to structured data because it lacks a consistent structure and may contain a wide variety of information. However, unstructured data often contains valuable insights and hidden patterns that can be extracted with the right techniques and tools.
Related Article: How To Convert a Python Dict To a Dataframe
Importance of Structuring Unstructured Data
Structuring unstructured data is essential for several reasons. Firstly, it enables efficient storage and retrieval of data. By organizing unstructured data into a structured format, it becomes easier to search, filter, and query the data, making it more accessible for analysis and decision-making.
Secondly, structured unstructured data can be used for various applications such as natural language processing, sentiment analysis, image recognition, recommendation systems, and predictive analytics. By structuring the data, we can leverage machine learning and data mining algorithms to uncover patterns and insights that can drive business value.
Lastly, structured unstructured data enables integration with other structured datasets, enabling cross-domain analysis and enhancing the overall understanding of the data.
Challenges of Working with Unstructured Data
Working with unstructured data poses several challenges due to its lack of structure and variability. Some common challenges include:
1. Data Volume:
Unstructured data is often voluminous, making it difficult to store and process. It requires efficient storage and processing systems to handle large volumes of data effectively.
Related Article: How To Filter Dataframe Rows Based On Column Values
2. Data Variety:
Unstructured data comes in various formats and types, such as text, images, videos, and audio. Each type requires different techniques and tools for processing and analysis.
3. Data Quality:
Unstructured data is prone to noise, errors, and inconsistencies. Data cleaning and preprocessing techniques are necessary to ensure data quality and accuracy.
4. Data Complexity:
Unstructured data can be complex and contain multiple layers of information. Extracting relevant information and identifying patterns and relationships can be challenging.
Related Article: How To Get Row Count Of Pandas Dataframe
5. Data Integration:
Integrating unstructured data with structured data sources can be challenging due to the differences in data formats and structures. Data transformation and normalization techniques are required for seamless integration.
Methods for Structuring Unstructured Data
There are several methods and techniques available for structuring unstructured data. In this section, we will explore some commonly used approaches.
1. Data Cleaning Techniques
Data cleaning is the process of identifying and correcting errors, inconsistencies, and inaccuracies in the data. It involves tasks such as removing irrelevant information, handling missing values, and standardizing data formats.
One common technique for data cleaning is text preprocessing. This involves removing special characters, punctuation, and stopwords (commonly used words like “the” or “and”) from text data. Additionally, techniques like stemming and lemmatization can be used to reduce words to their root form, improving the efficiency of text analysis algorithms.
Let’s take a look at an example of text preprocessing using Python’s NLTK library:
import nltk from nltk.corpus import stopwords from nltk.stem import WordNetLemmatizer # Download stopwords and lemmatizer resources nltk.download('stopwords') nltk.download('wordnet') # Define example text text = "This is an example sentence that needs preprocessing." # Tokenize the text into individual words tokens = nltk.word_tokenize(text) # Remove stopwords stopwords = set(stopwords.words('english')) filtered_tokens = [word for word in tokens if word.lower() not in stopwords] # Lemmatize words lemmatizer = WordNetLemmatizer() lemmatized_tokens = [lemmatizer.lemmatize(word) for word in filtered_tokens] print(lemmatized_tokens)
In this example, we first download the necessary resources for stopwords and lemmatization. Then, we define an example sentence and tokenize it into individual words. We remove the stopwords using NLTK’s stopwords list and lemmatize the remaining words using the WordNetLemmatizer. The output of this code will be:
['example', 'sentence', 'need', 'preprocessing', '.']
This demonstrates a basic text preprocessing technique for structuring unstructured textual data.
Related Article: Structuring Data for Time Series Analysis with Python
2. Data Analysis Approaches
Data analysis approaches involve using statistical and machine learning techniques to uncover patterns and insights from unstructured data. These approaches can include sentiment analysis, topic modeling, clustering, and classification.
For example, sentiment analysis aims to determine the sentiment or emotion expressed in a piece of text. This can be useful for analyzing customer reviews, social media posts, or any text data that contains subjective information.
Let’s see an example of sentiment analysis using Python’s TextBlob library:
from textblob import TextBlob # Define example text text = "I love this product! It exceeded my expectations." # Perform sentiment analysis blob = TextBlob(text) sentiment = blob.sentiment print(sentiment.polarity)
In this example, we use the TextBlob library to analyze the sentiment of an example text. The sentiment
object contains the polarity and subjectivity of the text. The polarity ranges from -1 (negative sentiment) to 1 (positive sentiment), with 0 being neutral. The output of this code will be:
0.5
This indicates a positive sentiment in the example text.
These data analysis approaches help structure unstructured data by providing insights and understanding of the underlying information.
3. Data Manipulation Strategies
Data manipulation strategies involve transforming and reshaping unstructured data to fit a desired structure or format. This can include tasks such as extracting information, merging data sources, and aggregating data.
One common data manipulation strategy is the use of regular expressions for pattern matching and extraction. Regular expressions allow us to define patterns in unstructured data and extract relevant information based on those patterns.
Let’s consider an example of extracting email addresses from a text using regular expressions in Python:
import re # Define example text text = "Please contact me at john@example.com or support@example.com." # Extract email addresses using regular expressions emails = re.findall(r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b', text) print(emails)
In this example, we use the re.findall()
function from Python’s built-in re
module to extract email addresses from the example text. The regular expression pattern \b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b
matches valid email addresses. The output of this code will be:
['john@example.com', 'support@example.com']
This demonstrates how regular expressions can be used to structure unstructured data by extracting specific information.
4. Data Preprocessing Methods
Data preprocessing methods involve transforming and preparing unstructured data for analysis. This can include tasks such as data cleaning, normalization, feature extraction, and dimensionality reduction.
One common data preprocessing method is feature extraction, which involves transforming unstructured data into a structured format by extracting relevant features or characteristics. Feature extraction is commonly used in natural language processing, image recognition, and audio processing.
Let’s consider an example of feature extraction using Python’s scikit-learn library:
from sklearn.feature_extraction.text import CountVectorizer # Define example text text = ["I love this product!", "This product is amazing!", "The quality is excellent!"] # Create a CountVectorizer object vectorizer = CountVectorizer() # Perform feature extraction features = vectorizer.fit_transform(text) print(vectorizer.get_feature_names()) print(features.toarray())
In this example, we use the CountVectorizer
class from scikit-learn to perform feature extraction on a list of example text. The fit_transform()
method converts the text into a matrix of token counts, where each row represents a document and each column represents a unique word in the corpus. The get_feature_names()
method returns the vocabulary of the corpus, and the toarray()
method converts the sparse matrix representation of the features into a dense numpy array. The output of this code will be:
['amazing', 'excellent', 'is', 'love', 'product', 'quality', 'the', 'this'] [[0 0 0 1 1 0 0 1] [1 0 1 0 1 0 0 1] [0 1 0 0 0 1 1 0]]
This demonstrates how feature extraction can be used to structure unstructured text data into a numerical representation.
Related Article: How to Use Pandas Groupby for Group Statistics in Python
5. Data Extraction Techniques
Data extraction techniques involve identifying and extracting relevant information from unstructured data sources. This can include techniques such as named entity recognition, keyword extraction, and information retrieval.
One common data extraction technique is named entity recognition (NER), which aims to identify and classify named entities (e.g., person names, organizations, locations) in unstructured text data.
Let’s consider an example of named entity recognition using the spaCy library in Python:
import spacy # Load the English language model nlp = spacy.load('en_core_web_sm') # Define example text text = "Apple Inc. is an American multinational technology company headquartered in Cupertino, California." # Perform named entity recognition doc = nlp(text) # Extract named entities entities = [(entity.text, entity.label_) for entity in doc.ents] print(entities)
In this example, we use the spaCy library to perform named entity recognition on an example text. The en_core_web_sm
model is loaded, and the text is processed using the nlp()
function. The resulting doc
object contains the identified named entities, which we extract and print. The output of this code will be:
[('Apple Inc.', 'ORG'), ('American', 'NORP'), ('Cupertino', 'GPE'), ('California', 'GPE')]
This demonstrates how named entity recognition can be used to extract specific information from unstructured text data.
6. Data Transformation Approaches
Data transformation approaches involve converting unstructured data into a structured format through various techniques such as parsing, normalization, and encoding.
One common data transformation approach is the use of parsing techniques to extract structured information from unstructured data sources. Parsing involves analyzing the syntax and structure of the data to extract meaningful information.
Let’s consider an example of parsing XML data in Python using the ElementTree library:
import xml.etree.ElementTree as ET # Define example XML data data = ''' <title>Harry Potter and the Philosopher's Stone</title> J.K. Rowling 1997 <title>Thinking, Fast and Slow</title> Daniel Kahneman 2011 ''' # Parse the XML data root = ET.fromstring(data) # Extract information from the parsed data books = [] for book in root.findall('book'): title = book.find('title').text author = book.find('author').text year = book.find('year').text category = book.get('category') books.append({'title': title, 'author': author, 'year': year, 'category': category}) print(books)
In this example, we define an example XML data representing a bookstore with books of different categories. We use the ET.fromstring()
function from the ElementTree library to parse the XML data. We then extract the relevant information from the parsed data using the find()
and get()
methods. The extracted information is stored in a list of dictionaries representing each book. The output of this code will be:
[{'title': "Harry Potter and the Philosopher's Stone", 'author': 'J.K. Rowling', 'year': '1997', 'category': 'Fiction'}, {'title': 'Thinking, Fast and Slow', 'author': 'Daniel Kahneman', 'year': '2011', 'category': 'Non-Fiction'}]
This demonstrates how parsing can be used to transform unstructured XML data into a structured format.
7. Data Normalization Techniques
Data normalization techniques involve transforming unstructured data into a standardized format to eliminate redundancies and inconsistencies. Normalization can include tasks such as data deduplication, standardizing data formats, and resolving inconsistencies.
One common data normalization technique is data deduplication, which aims to identify and remove duplicate records or information from unstructured data sources.
Let’s consider an example of data deduplication using Python’s pandas library:
import pandas as pd # Define example dataset data = {'Name': ['John Smith', 'Jane Doe', 'John Smith', 'John Doe', 'Jane Doe'], 'Age': [25, 30, 25, 35, 30], 'City': ['New York', 'San Francisco', 'New York', 'Chicago', 'San Francisco']} df = pd.DataFrame(data) # Perform data deduplication deduplicated_df = df.drop_duplicates() print(deduplicated_df)
In this example, we define an example dataset with columns for name, age, and city. We create a pandas DataFrame from the data and use the drop_duplicates()
method to remove duplicate records from the DataFrame. The output of this code will be:
Name Age City 0 John Smith 25 New York 1 Jane Doe 30 San Francisco 3 John Doe 35 Chicago
This demonstrates how data deduplication can be used to normalize unstructured data by removing duplicate information.
Related Article: How to Change Column Type in Pandas
8. Common Techniques for Data Structuring
There are several common techniques for structuring unstructured data, including:
– Text parsing and tokenization: Breaking down unstructured text into smaller units (tokens) for further analysis.
– Entity recognition: Identifying and classifying named entities in unstructured text data.
– Feature extraction: Transforming unstructured data into a structured format by extracting relevant features or characteristics.
– Data deduplication: Identifying and removing duplicate records or information from unstructured data sources.
– Normalization: Transforming unstructured data into a standardized format to eliminate redundancies and inconsistencies.
– Data integration: Combining unstructured data with structured data sources to enable cross-domain analysis.
– Data transformation: Converting unstructured data into a structured format through parsing, normalization, and encoding techniques.
These techniques help in structuring unstructured data and making it more amenable to analysis and processing.
Libraries and Packages for Structuring Unstructured Data
There are several libraries and packages available in Python that facilitate the structuring of unstructured data. These libraries provide a wide range of functionalities for data cleaning, analysis, manipulation, preprocessing, extraction, transformation, and normalization.
Here are some commonly used libraries and packages for structuring unstructured data in Python:
– Natural Language Toolkit (NLTK): Provides a suite of libraries and programs for natural language processing, including text preprocessing, tokenization, stemming, lemmatization, and named entity recognition.
– spaCy: A Python library for industrial-strength natural language processing, featuring pre-trained models for named entity recognition, part-of-speech tagging, dependency parsing, and more.
– scikit-learn: A popular machine learning library that provides various tools for data analysis and preprocessing, including feature extraction, dimensionality reduction, and data normalization techniques.
– pandas: A useful data manipulation and analysis library that provides data structures and functions for cleaning, transforming, and organizing data, including handling missing values, data deduplication, and data integration.
– Beautiful Soup: A Python library for web scraping that allows you to extract data from HTML and XML documents, making it useful for parsing and extracting structured information from unstructured web data.
– TensorFlow: An open-source machine learning framework that provides tools for building and training machine learning models, including deep learning models for tasks such as image recognition and natural language processing.
These libraries and packages provide a wide range of functionalities and can be combined to structure and analyze unstructured data effectively.
Code Snippets for Structuring Unstructured Data
Here are some code snippets that demonstrate the usage of various libraries and techniques for structuring unstructured data:
Related Article: How to Implement Data Science and Data Engineering Projects with Python
Example 1: Text Preprocessing using NLTK
import nltk from nltk.corpus import stopwords from nltk.stem import WordNetLemmatizer # Download stopwords and lemmatizer resources nltk.download('stopwords') nltk.download('wordnet') # Define example text text = "This is an example sentence that needs preprocessing." # Tokenize the text into individual words tokens = nltk.word_tokenize(text) # Remove stopwords stopwords = set(stopwords.words('english')) filtered_tokens = [word for word in tokens if word.lower() not in stopwords] # Lemmatize words lemmatizer = WordNetLemmatizer() lemmatized_tokens = [lemmatizer.lemmatize(word) for word in filtered_tokens] print(lemmatized_tokens)
Example 2: Named Entity Recognition using spaCy
import spacy # Load the English language model nlp = spacy.load('en_core_web_sm') # Define example text text = "Apple Inc. is an American multinational technology company headquartered in Cupertino, California." # Perform named entity recognition doc = nlp(text) # Extract named entities entities = [(entity.text, entity.label_) for entity in doc.ents] print(entities)
Example 3: Data Deduplication using pandas
import pandas as pd # Define example dataset data = {'Name': ['John Smith', 'Jane Doe', 'John Smith', 'John Doe', 'Jane Doe'], 'Age': [25, 30, 25, 35, 30], 'City': ['New York', 'San Francisco', 'New York', 'Chicago', 'San Francisco']} df = pd.DataFrame(data) # Perform data deduplication deduplicated_df = df.drop_duplicates() print(deduplicated_df)
These code snippets demonstrate the usage of different libraries and techniques for structuring unstructured data.
Related Article: How to Delete a Column from a Pandas Dataframe
Step-by-Step Guide to Structuring Unstructured Data
Here is a step-by-step guide to structuring unstructured data:
Step 1: Understand the Data:
Thoroughly analyze and understand the unstructured data. Consider its volume, variety, and quality, as well as any potential biases or limitations.
Step 2: Define Objectives:
Clearly define your objectives and goals for structuring the unstructured data. Understand what insights or patterns you want to extract from the data.
Related Article: How to Rename Column Names in Pandas
Step 3: Choose Techniques and Tools:
Select the appropriate techniques and tools for structuring the unstructured data based on its characteristics and your objectives.
Step 4: Clean and Preprocess the Data:
Clean and preprocess the unstructured data to remove noise, errors, and inconsistencies. Use techniques such as text preprocessing, data cleaning, and normalization.
Step 5: Perform Data Analysis:
Apply data analysis approaches to uncover patterns and insights from the structured data. This can include techniques such as sentiment analysis, topic modeling, and clustering.
Related Article: How to Use Pandas Dataframe Apply in Python
Step 6: Manipulate and Transform the Data:
Manipulate and transform the structured data to fit desired formats or structures. This can include tasks such as data extraction, merging, and aggregation.
Step 7: Validate and Verify the Structured Data:
Validate and verify the structured data to ensure its accuracy and reliability. Compare it against the original unstructured data and perform data quality checks.
Step 8: Document the Structuring Process:
Document the steps and techniques used to structure the unstructured data. This will help with reproducibility and enable others to understand and validate the structured data.
Related Article: How To Reorder Columns In Python Pandas Dataframe
Step 9: Continuously Monitor and Update the Structured Data:
Regularly monitor and update the structured data to ensure its relevance and accuracy. Adjust the structuring approach as needed to accommodate changes in the unstructured data sources.
Additional Resources
– NLTK documentation
– Scikit-learn documentation
– Pandas documentation