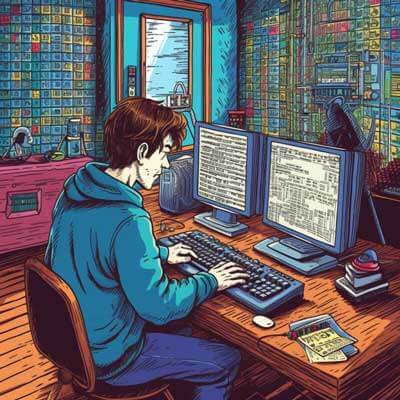
Table of Contents
To position the legend outside the plot in Matplotlib, you can use the bbox_to_anchor
parameter of the legend
function. This parameter allows you to specify the coordinates of the legend relative to the figure, rather than the axes. Here's how you can do it:
Method 1: Using the bbox_to_anchor
parameter
1. Create a plot using Matplotlib.
2. Add a legend to the plot using the legend
function.
3. Specify the bbox_to_anchor
parameter with the coordinates where you want the legend to be positioned. The coordinates are specified as a tuple of four values: (x, y, width, height)
. The x
and y
values specify the lower-left corner of the legend, and the width
and height
values specify the size of the legend. By default, the coordinates are in the range [0, 1], where (0, 0) is the lower-left corner of the plot and (1, 1) is the upper-right corner.
4. Call the plot
function to plot your data.
5. Call the show
function to display the plot.
Here's an example:
import matplotlib.pyplot as plt # Create a plot plt.plot([1, 2, 3], [4, 5, 6], label='Line 1') plt.plot([1, 2, 3], [6, 5, 4], label='Line 2') # Add a <a href="https://www.squash.io/how-to-add-a-matplotlib-legend-in-python/">legend outside the plot</a> plt.legend(bbox_to_anchor=(1.01, 1), loc='upper left') # Display the plot plt.show()
In this example, the legend is positioned slightly to the right of the plot and aligned with the upper left corner of the plot.
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
Method 2: Using the loc
parameter
Another way to position the legend outside the plot is by using the loc
parameter of the legend
function. This parameter allows you to specify the location of the legend within the plot. To position the legend outside the plot, you can set the loc
parameter to a value that is not within the plot area, such as 'upper left'
or 'upper right'
.
Here's an example:
import matplotlib.pyplot as plt # Create a plot plt.plot([1, 2, 3], [4, 5, 6], label='Line 1') plt.plot([1, 2, 3], [6, 5, 4], label='Line 2') # Add a legend outside the plot plt.legend(loc='upper left') # Display the plot plt.show()
In this example, the legend is positioned outside the plot in the upper left corner.
Additional Tips
Related Article: How to Define a Function with Optional Arguments in Python
- You can adjust the position of the legend by changing the values of the bbox_to_anchor
parameter or the loc
parameter. Experiment with different values to achieve the desired position.
- If you want to control the size of the legend, you can use the prop
parameter of the legend
function to set the font size. For example, plt.legend(prop={'size': 10})
sets the font size of the legend to 10.
- If you have multiple legends in your plot, you can specify the bbox_to_anchor
parameter or the loc
parameter for each legend separately to position them independently.