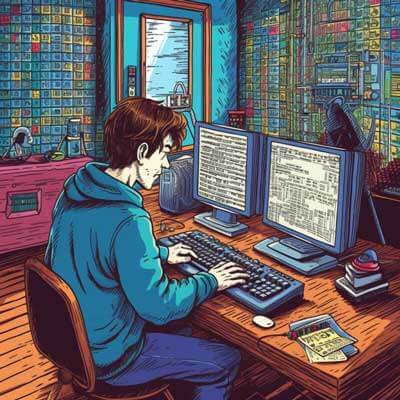
Table of Contents
To adjust the font size in a Matplotlib plot, you can use various methods provided by the Matplotlib library. Here are two possible ways to adjust the font size:
Method 1: Using the rcParams Method
One way to adjust the font size in a Matplotlib plot is by modifying the rcParams
dictionary. The rcParams
dictionary stores default settings for Matplotlib. By modifying the values in this dictionary, you can change the default font size for your plots.
Here's an example of how to adjust the font size using the rcParams
method:
import matplotlib.pyplot as plt # Set the font size plt.rcParams['font.size'] = 12 # Create a simple plot x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] plt.plot(x, y) # Display the plot plt.show()
In the example above, the font size is set to 12 using plt.rcParams['font.size'] = 12
. You can adjust the value to your desired font size. By modifying the font.size
value in the rcParams
dictionary, you can change the font size for all elements in your plot, including the title, axis labels, tick labels, and legends.
Related Article: How to Use Different Python Versions With Virtualenv
Method 2: Using the fontsize
Parameter
Another way to adjust the font size in a Matplotlib plot is by using the fontsize
parameter in the various plotting functions. This method allows you to set the font size for specific elements in your plot.
Here's an example of how to adjust the font size using the fontsize
parameter:
import matplotlib.pyplot as plt # Create a simple plot x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] plt.plot(x, y) # Set the font size for the title plt.title('My Plot', fontsize=14) # Set the font size for the x and y axis labels plt.xlabel('X-axis', fontsize=12) plt.ylabel('Y-axis', fontsize=12) # Set the font size for the tick labels plt.xticks(fontsize=10) plt.yticks(fontsize=10) # Display the plot plt.show()
In the example above, the fontsize
parameter is used to set the font size for different elements of the plot. The fontsize
parameter is specified within the various plotting functions like plt.title
, plt.xlabel
, plt.ylabel
, plt.xticks
, and plt.yticks
. By adjusting the value of fontsize
, you can set the desired font size for each element.
Additional Suggestions
Related Article: How to Check for an Empty String in Python
- You can also adjust the font size for specific text elements in your plot, such as legends, annotations, and text labels, by using the fontsize
parameter in the respective functions.
- If you want to change the font size for a specific plot without affecting the default settings, you can create a separate style sheet using the matplotlib.style
module. This allows you to define custom styles for your plots, including font size.
- When adjusting the font size, it is important to consider the readability and aesthetics of your plot. Choosing an appropriate font size can enhance the visual appeal and clarity of your plot.