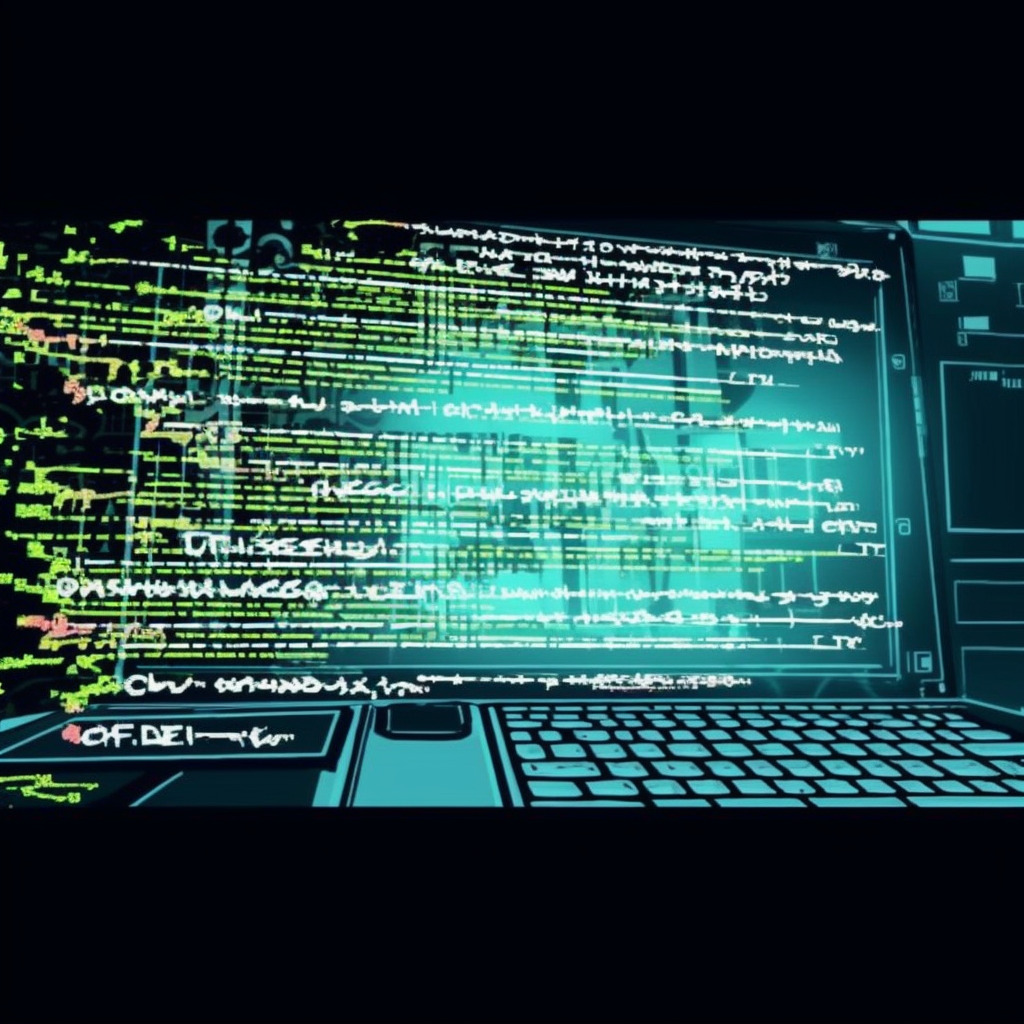
- What is GraphQL?
- What is REST?
- Key Differences between GraphQL and REST
- 1. Data Fetching
- 2. Response Structure
- 3. Versioning
- 4. Request Size
- 5. Tooling and Ecosystem
- Benefits of Using GraphQL
- Efficient Data Fetching
- Flexible API Design
- Reduced Over-Fetching and Under-Fetching
- Strong Typing
- Batched Queries
- Benefits of Using REST
- Simplicity and Ease of Learning
- Flexibility
- Statelessness
- Compatibility and Interoperability
- Tooling and Ecosystem
- When to Use GraphQL
- 1. Complex data requirements
- 2. Rapid development and iteration
- 3. Mobile and low-bandwidth applications
- 4. Microservices architecture
- When to Use REST
- 1. Simple and Stateless Operations
- 2. Caching and Performance
- 3. Wide Client Support
- 4. Compatibility with Existing Systems
- Real World Examples of GraphQL
- 1. GitHub API
- 2. Shopify API
- 3. Twitter API
- Real World Examples of REST
- Example 1: Twitter API
- Example 2: GitHub API
- Example 3: Stripe API
- Advanced Techniques for Querying with GraphQL
- 1. Aliases
- 2. Fragments
- 3. Variables
- 4. Directives
- Advanced Techniques for Requesting Data with REST
- 1. Pagination
- 2. Filtering
- 3. Sorting
- 4. Sparse Fieldsets
- 5. Caching
- 6. Batch Requests
- Handling Errors with GraphQL
- Handling Errors with REST
- Caching Strategies with GraphQL
- Cache at the network layer
- Cache at the field level
- Cache control directives
- Caching Strategies with REST
- Server-Side Caching
- Client-Side Caching
- Conditional Requests
- Security Considerations for GraphQL
- 1. Authorization and Authentication
- 2. Query Depth and Complexity
- 3. Input Validation
- 4. Rate Limiting
- 5. Secure GraphQL Endpoints
- Security Considerations for REST
- 1. Authentication and Authorization
- 2. Transport Layer Security (TLS)
- 3. Input Validation
- 4. Cross-Origin Resource Sharing (CORS)
- 5. Rate Limiting
What is GraphQL?
GraphQL is an open-source query language and runtime for APIs developed by Facebook. It was designed to overcome some of the limitations of RESTful APIs and provide a more efficient and flexible way to retrieve and manipulate data.
At its core, GraphQL allows clients to request only the data they need and specify the structure of the response. This is in contrast to RESTful APIs where the server determines the structure of the response, often resulting in over-fetching or under-fetching of data.
With GraphQL, clients can send a single request to the server and get all the required data in a single response. This reduces the number of network requests and eliminates the need for multiple round trips to fetch related data. It also allows clients to aggregate data from different sources and combine them into a single response.
Another key feature of GraphQL is its type system. It allows developers to define a schema that describes the available data and operations in a strongly-typed manner. This schema serves as a contract between the client and the server, ensuring that both sides understand each other’s expectations.
Here’s an example of a GraphQL query:
query { user(id: "123") { name email posts { title content } } }
In this example, the client is requesting the name, email, and posts of a user with the ID “123”. The response will only contain the requested fields, making the payload more efficient.
GraphQL also supports mutations, which allow clients to modify data on the server. Mutations are similar to queries but are used to perform actions that cause side effects. For example, creating a new user or updating an existing one.
mutation { createUser(input: { name: "John Doe", email: "john@example.com" }) { id name email } }
In this mutation example, the client is creating a new user with the name “John Doe” and email “john@example.com”. The response will contain the generated ID of the newly created user along with its name and email.
Overall, GraphQL provides a more efficient and flexible approach to building APIs compared to REST. It allows clients to request exactly what they need and eliminates the problem of over-fetching or under-fetching data. The type system ensures a clear understanding between the client and server, making it easier to develop and maintain the API.
Related Article: How to Use the in Source Query Parameter in Elasticsearch
What is REST?
REST, which stands for Representational State Transfer, is an architectural style for building web services. It was introduced by Roy Fielding in his doctoral dissertation in 2000 and has since become the most common approach for designing APIs on the web.
REST is based on a few key principles:
1. Statelessness: RESTful APIs are stateless, meaning that each request from a client to a server contains all the information needed to understand and process that request. The server does not store any information about the client’s state between requests. This makes REST APIs scalable and easy to cache.
2. Resource-Based: RESTful APIs are built around resources, which are identified by URLs (Uniform Resource Locators). Each resource can be accessed and manipulated using standard HTTP methods such as GET, POST, PUT, and DELETE. For example, a blog post resource might have the URL /posts/123
, and a GET request to that URL would retrieve the details of the blog post.
3. Uniform Interface: RESTful APIs have a uniform interface that allows clients to interact with resources in a consistent way. This means that the API should follow a set of standard conventions for how resources are accessed, modified, and deleted. These conventions are typically based on HTTP methods and status codes.
4. Client-Server Architecture: RESTful APIs are based on a client-server architecture, where the client is responsible for the user interface and the server is responsible for processing requests and managing resources. This separation of concerns allows for a more scalable and decoupled system.
5. Layered System: RESTful APIs can be designed as a layered system, where each layer has a specific responsibility. This allows for flexibility and modularity, as each layer can be modified or replaced without affecting the other layers.
Here is an example of a RESTful API endpoint for retrieving a list of blog posts:
GET /posts
The server would respond with a list of blog posts in a specific format, such as JSON or XML.
REST has been widely adopted because of its simplicity, scalability, and compatibility with existing web technologies. It allows clients to access and manipulate resources using standard HTTP methods, making it easy to integrate with a wide range of programming languages and frameworks.
However, REST does have some limitations. One of the main challenges with REST is over-fetching or under-fetching of data. In some cases, a client might need to make multiple requests to retrieve all the data it needs, leading to increased latency and network overhead. This is where GraphQL, a query language for APIs, comes into play, as we will explore in the next chapter.
Key Differences between GraphQL and REST
GraphQL and REST are two popular approaches for building APIs, but they have significant differences in how they handle data retrieval and manipulation. Understanding these differences can help you choose the right approach for your project. Let’s explore the key differences between GraphQL and REST.
1. Data Fetching
In a RESTful API, each endpoint represents a specific resource, and you typically make separate HTTP requests to retrieve related data. For example, if you have a blog post with comments, you might first fetch the post and then make an additional request to fetch its comments.
GraphQL, on the other hand, allows you to fetch multiple resources in a single request. With GraphQL, you can specify the exact data you need in the query, and the server will return only that data. This reduces over-fetching and under-fetching of data, resulting in more efficient data retrieval.
Here’s an example of a GraphQL query to retrieve a blog post and its comments:
query { post(id: 123) { title content comments { text author } } }
Related Article: Detecting High-Cost Queries in Elasticsearch via Kibana
2. Response Structure
REST APIs typically have a fixed response structure defined by the server. When you request a resource, you get a predefined set of fields and relationships. If you need additional data, you have to make another request or use query parameters to include related resources.
With GraphQL, the response structure matches the structure of the query. The server returns exactly what you requested, and you can nest fields and relationships to any level. This flexibility allows clients to retrieve precisely the data they need and avoid over-fetching.
3. Versioning
In RESTful APIs, versioning is often necessary to introduce breaking changes or add new features. This is typically done by including the version number in the URL or using custom headers.
GraphQL handles versioning differently. Because the client specifies the exact data it needs, the server can introduce changes without breaking existing clients. New fields can be added, deprecated fields can be marked, and clients can choose to adopt these changes at their own pace.
4. Request Size
REST APIs often suffer from over-fetching or under-fetching of data. Clients may receive more data than they need or make multiple requests to fetch related resources. This can lead to larger response sizes and slower performance.
GraphQL addresses this issue by allowing clients to request only the required data. The client specifies the fields it needs, reducing the response size and improving performance. This is especially beneficial for mobile or low-bandwidth environments.
Related Article: Monitoring Query Performance in Elasticsearch using Kibana
5. Tooling and Ecosystem
REST has been around for a long time, and there are numerous tools and libraries available for building and consuming RESTful APIs. REST is also well-understood by developers, making it easier to onboard new team members.
GraphQL, though newer, has gained significant popularity and has a growing ecosystem of tools and libraries. GraphQL also comes with powerful developer tools that enable introspection and schema discovery. These tools make it easier to explore and understand the API without relying on external documentation.
In conclusion, GraphQL and REST have fundamental differences in data fetching, response structure, versioning, request size, and tooling. Understanding these differences can help you choose the right approach for your specific use case. Whether you prefer the simplicity and familiarity of REST or the flexibility and efficiency of GraphQL, both have their strengths and can be used to build powerful APIs.
Benefits of Using GraphQL
GraphQL offers several benefits over traditional RESTful APIs, making it an attractive choice for building modern, efficient, and flexible web applications. In this chapter, we will explore some of the key benefits of using GraphQL.
Efficient Data Fetching
One of the main advantages of GraphQL is its ability to efficiently fetch data. With REST, multiple requests are often needed to retrieve all the required data from different endpoints. This can lead to over-fetching or under-fetching of data, resulting in either wasted bandwidth or additional roundtrips. With GraphQL, you can specify exactly what data you need for a particular request, reducing the amount of data transferred over the network and improving performance.
Here’s an example of a GraphQL query that fetches specific fields from multiple resources:
query { user(id: "123") { name email posts { title content } } }
Related Article: Exploring Query Passage in Spark Elasticsearch
Flexible API Design
GraphQL allows clients to request only the data they need, enabling a more flexible and efficient API design. Unlike REST, where the server defines the structure of the response, GraphQL lets the client specify the shape of the response. This decoupling between client and server enables rapid iteration and evolution of APIs without breaking existing clients.
query { user(id: "123") { name email } }
query { user(id: "123") { name posts { title content } } }
Reduced Over-Fetching and Under-Fetching
In REST APIs, endpoints often return more data than necessary, leading to over-fetching. This can result in increased bandwidth usage and slower response times. On the other hand, under-fetching occurs when an endpoint does not provide all the required data, forcing the client to make multiple requests. GraphQL solves these problems by allowing clients to specify their exact data requirements for each request, eliminating over-fetching and under-fetching.
query { user(id: "123") { name email posts { title content } } }
Strong Typing
GraphQL has a strong type system, which provides clarity and documentation for the available data and operations. By defining a schema that represents the API’s capabilities, clients can discover and understand the data they can request. This self-documenting nature of GraphQL makes it easier to develop and maintain APIs.
type User { id: ID! name: String! email: String! posts: [Post!]! } type Post { id: ID! title: String! content: String! }
Related Article: Altering Response Fields in an Elasticsearch Query
Batched Queries
GraphQL allows clients to send multiple queries in a single request, reducing the number of roundtrips to the server. This batching capability can significantly improve performance, especially in scenarios where multiple resources need to be fetched simultaneously.
query { user(id: "123") { name email } user(id: "456") { name email } }
Overall, GraphQL offers numerous benefits that make it a powerful alternative to REST. It provides efficient data fetching, flexible API design, reduced over-fetching and under-fetching, strong typing, and the ability to batch queries. These advantages make GraphQL a compelling choice for building modern web applications.
Benefits of Using REST
REST (Representational State Transfer) is a widely adopted architectural style for designing networked applications. It offers several benefits that make it a popular choice for building web services. In this chapter, we will explore some of the advantages of using REST over other alternatives, such as GraphQL.
Simplicity and Ease of Learning
One of the main benefits of using REST is its simplicity. RESTful APIs are based on a small set of well-defined principles, making it easy to understand and learn. REST leverages the existing HTTP protocol, which is widely understood and used, making it accessible to developers from various backgrounds.
Related Article: Exploring Elasticsearch Query Response Mechanisms
Flexibility
REST allows for flexibility in designing APIs. It follows a resource-oriented approach, where resources are identified by unique URLs (Uniform Resource Locators). This enables developers to model their APIs around the concept of resources, providing a consistent and intuitive way to interact with data. The flexibility of REST allows for the creation of APIs that are tailored to specific use cases and requirements.
Statelessness
REST is stateless, meaning that each request from a client to a server must contain all the necessary information to understand and process the request. The server does not store any client-related information between requests. This simplifies the server implementation and allows for easier scalability, as there is no need to maintain session state. Stateless APIs are also easier to cache, resulting in improved performance and reduced load on the server.
Compatibility and Interoperability
REST is based on the HTTP protocol, which is the backbone of the World Wide Web. This means that RESTful APIs can be easily consumed by any client or integrated with existing web technologies. RESTful APIs can be accessed using standard HTTP methods such as GET, POST, PUT, and DELETE, making it compatible with a wide range of tools and frameworks. This compatibility and interoperability make REST a popular choice for building web services that need to interact with different systems and platforms.
Related Article: Combining Match and Range Queries in Elasticsearch
Tooling and Ecosystem
REST has been around for a long time and has a mature ecosystem with a wide range of tooling and libraries available. There are numerous frameworks and libraries that make it easy to build RESTful APIs in various programming languages. This vast ecosystem provides developers with a wealth of resources and community support, making it easier to develop, test, and maintain RESTful APIs.
Overall, REST offers simplicity, flexibility, statelessness, compatibility, and a mature ecosystem, making it a compelling choice for building web services. In the next chapter, we will explore the benefits of using GraphQL and how it compares to REST.
When to Use GraphQL
GraphQL is a powerful tool that provides developers with a flexible way to query and manipulate data. While REST has been the go-to option for many years, GraphQL offers several advantages in certain scenarios. Let’s explore when it makes sense to use GraphQL over REST.
1. Complex data requirements
One of the main selling points of GraphQL is its ability to efficiently handle complex data requirements. In a REST API, you typically have multiple endpoints that return fixed data structures. This can lead to over-fetching or under-fetching of data, resulting in inefficient network requests.
With GraphQL, you can specify exactly what data you need in a single request. The server then responds with only the requested data, eliminating unnecessary round trips. This flexibility is especially valuable when dealing with deeply nested data structures or when the client needs to fetch data from multiple resources in a single request.
Consider the following example where a client needs to fetch information about a user and their associated posts:
query { user(id: "123") { name email posts { title content } } }
The server can efficiently resolve this query and return only the requested fields, reducing the payload size and improving performance.
Related Article: Tutorial: Supported Query Types in Elasticsearch
2. Rapid development and iteration
GraphQL’s introspection capabilities make it an excellent choice for rapid development and iteration. With a GraphQL schema, clients can dynamically discover and explore the available data and operations. This enables developers to build and evolve applications more quickly.
For example, imagine adding a new field to an existing API. In a REST API, clients would need to be updated to consume the new endpoint and handle the new data structure. With GraphQL, clients can query for the new field without requiring any changes on the server side.
3. Mobile and low-bandwidth applications
Mobile applications often have limited network bandwidth and face challenges with high latency. GraphQL’s ability to request only the required data can significantly improve the performance of mobile apps.
By minimizing the payload size and reducing the number of round trips, GraphQL can provide a smoother user experience on low-bandwidth connections. Additionally, GraphQL supports features like batching and caching, further optimizing network requests for mobile applications.
4. Microservices architecture
In a microservices architecture, different services handle different parts of the application’s functionality. With REST, clients often need to make multiple requests to different endpoints to fetch data from each service. This can result in tight coupling between the client and the server.
GraphQL solves this problem by allowing clients to specify their data requirements in a single request. The GraphQL server then coordinates with the necessary services to gather the required data. This decouples the client from the underlying services, making it easier to evolve and scale the system.
Related Article: Comparing Sorting Algorithms (with Java Code Snippets)
When to Use REST
REST (Representational State Transfer) is a widely adopted architectural style for designing networked applications. It has been around for many years and has proven to be effective in a variety of scenarios. Here are some situations where using REST might be the best choice:
1. Simple and Stateless Operations
REST is a good fit for applications that require simple and stateless operations. If your application involves basic CRUD (Create, Read, Update, Delete) operations on resources, REST can provide a straightforward way to expose these operations as HTTP endpoints.
For example, consider a blog application where you need to create, read, update, and delete blog posts. With REST, you can define the following endpoints:
POST /posts - Create a new blog post GET /posts - Get a list of all blog posts GET /posts/{id} - Get a specific blog post PUT /posts/{id} - Update a specific blog post DELETE /posts/{id} - Delete a specific blog post
These endpoints follow the REST principles and provide a clear and intuitive way to interact with the blog post resources.
2. Caching and Performance
RESTful APIs can take advantage of HTTP caching mechanisms to improve performance. By setting appropriate cache headers, you can enable clients to cache responses and reduce the load on your server.
For example, if you have an API endpoint that returns a list of products, you can set the appropriate cache headers to indicate that the response can be cached for a certain period of time. This can significantly improve the performance of your API by reducing the number of requests to the server.
Related Article: Defining Greedy Algorithms to Solve Optimization Problems
3. Wide Client Support
RESTful APIs are widely supported by clients and can be consumed from a variety of platforms and programming languages. This makes it easier to build applications that interact with your API.
For example, if you have a mobile application that needs to consume data from your API, REST provides a well-known and widely supported way to fetch and manipulate that data.
4. Compatibility with Existing Systems
If you have existing systems or infrastructure that are based on REST, it might make sense to continue using REST for new applications. This can help maintain consistency and interoperability across your systems.
For example, if you have an existing system that exposes a RESTful API, it would be easier to integrate a new application into that system by using REST instead of introducing a different API style like GraphQL.
Overall, REST is a solid choice for many applications, especially those that require simple and stateless operations, can benefit from caching, need wide client support, or need to integrate with existing REST-based systems.
Real World Examples of GraphQL
In order to better understand the benefits and practical applications of GraphQL, let’s explore a few real-world examples where GraphQL has been successfully implemented.
Related Article: Comparing GraphQL and Elasticsearch
1. GitHub API
GitHub, the popular code hosting platform, serves as a great example of GraphQL in action. They introduced their GraphQL API in 2016, which allows developers to query for specific data they need using a single request. This eliminates the problem of over-fetching or under-fetching data that is commonly associated with traditional REST APIs.
For instance, instead of making multiple requests to fetch information about a user’s repositories, followers, and organizations, a developer can simply make a single GraphQL query to retrieve all the required data in one go.
Here’s an example of a GraphQL query to retrieve a user’s repositories:
query { user(login: "octocat") { repositories(first: 5) { nodes { name description url } } } }
This query will return the names, descriptions, and URLs of the first five repositories owned by the user “octocat”.
2. Shopify API
Shopify, a popular e-commerce platform, also utilizes GraphQL to power its API. With GraphQL, developers can retrieve precise data about products, orders, customers, and more, all with a single request.
For example, to fetch information about a specific product, a developer can use the following GraphQL query:
query { product(id: "gid://shopify/Product/123456789") { title description price variants(first: 5) { edges { node { title price } } } } }
This query will return the title, description, and price of the product, along with the titles and prices of the first five variants.
3. Twitter API
Twitter also adopted GraphQL to enhance their API capabilities. With GraphQL, developers can build more efficient and flexible queries to fetch data from Twitter’s vast network of tweets, users, and engagements.
For instance, to retrieve information about a specific user’s tweets and their associated metadata, a developer can use the following GraphQL query:
query { user(username: "twitterdev") { tweets(count: 5) { edges { node { id text createdAt retweetCount likeCount } } } } }
This query will return the IDs, text, creation dates, retweet counts, and like counts of the five most recent tweets by the user “twitterdev”.
These examples demonstrate how GraphQL can simplify and optimize data fetching by allowing developers to request only the data they need in a single query, eliminating the need for multiple requests and reducing network overhead.
In the next chapter, we will explore the key differences between GraphQL and REST to help you make an informed decision on when to choose one over the other.
Related Article: How to Work with Async Calls in JavaScript
Real World Examples of REST
In this section, we will explore some real-world examples of how REST is used in practice.
Example 1: Twitter API
Twitter provides a RESTful API that allows developers to interact with the Twitter platform. The API supports various operations such as retrieving tweets, posting new tweets, searching for tweets, and managing user accounts. Here’s an example of how you can use the Twitter API to retrieve a user’s timeline:
import requests response = requests.get("https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name=twitterapi&count=10") if response.status_code == 200: tweets = response.json() for tweet in tweets: print(tweet['text'])
Example 2: GitHub API
GitHub’s API is another popular example of a RESTful API. It allows developers to access various resources on the GitHub platform, such as repositories, issues, and pull requests. Here’s an example of how you can use the GitHub API to retrieve information about a repository:
const fetch = require('node-fetch'); fetch('https://api.github.com/repos/octocat/hello-world') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
Related Article: Visualizing Binary Search Trees: Deep Dive
Example 3: Stripe API
Stripe is a popular payment processing platform that provides a RESTful API for developers to integrate payment functionality into their applications. The API allows you to create and manage customers, process payments, and retrieve transaction data. Here’s an example of how you can use the Stripe API to create a new customer:
import com.stripe.Stripe; import com.stripe.model.Customer; import java.util.HashMap; import java.util.Map; Stripe.apiKey = "sk_test_XXXXXXXXXXXXXXXXXXXXXXXX"; Map<String, Object> params = new HashMap<>(); params.put("name", "John Doe"); params.put("email", "john.doe@example.com"); Customer customer = Customer.create(params); System.out.println(customer.getId());
These examples demonstrate the simplicity and flexibility of RESTful APIs in real-world scenarios. REST allows developers to interact with various services over HTTP using a simple and standardized approach.
Continue reading to learn about GraphQL and how it compares to REST.
To request data with REST, you typically use HTTP methods such as GET, POST, PUT, and DELETE. These methods correspond to the CRUD (Create, Read, Update, Delete) operations on resources. In a RESTful API, resources are represented by URLs, also known as endpoints.
Let’s start with a simple example. Suppose we have a RESTful API that exposes a collection of books. To retrieve all the books, we can make a GET request to the following URL:
GET /api/books
This will return a list of books in the response body. The response may also include additional information such as headers and status codes.
If we want to retrieve a specific book, we can include the book’s unique identifier in the URL:
GET /api/books/{id}
Here, {id}
represents the identifier of the book. For example, to retrieve the book with an id of 1, we would make the following request:
GET /api/books/1
To create a new book, we can use the POST method and provide the book’s details in the request body:
POST /api/books Content-Type: application/json { "title": "The Great Gatsby", "author": "F. Scott Fitzgerald", "year": 1925 }
The server will then create a new book resource and return a response with the newly created book’s information, including its unique identifier.
Updating an existing book can be done using the PUT or PATCH method. With PUT, you replace the entire resource, while with PATCH, you update only the specified fields. For example:
PUT /api/books/1 Content-Type: application/json { "title": "The Great Gatsby", "author": "F. Scott Fitzgerald", "year": 1922 }
This request would update the book with an id of 1, changing the year to 1922.
Finally, to delete a book, you can use the DELETE method:
DELETE /api/books/1
This will remove the book with an id of 1 from the collection.
In addition to the basic CRUD operations, RESTful APIs can also support filtering, sorting, and pagination through query parameters. For example, to retrieve the first 10 books sorted by title, you could use the following URL:
GET /api/books?limit=10&sort=title
In this chapter, we covered the basics of requesting data with REST. We explored how to use HTTP methods to perform CRUD operations on resources and discussed how to handle filtering, sorting, and pagination. In the next chapter, we will dive into GraphQL and compare it with REST.
Advanced Techniques for Querying with GraphQL
In the previous section, we explored the basics of querying with GraphQL and compared it to the traditional REST architecture. In this section, we will dive deeper into some advanced techniques for querying data using GraphQL.
1. Aliases
Aliases in GraphQL allow you to rename the fields returned in a query. This can be useful when you have multiple fields with the same name or when you want to give a more meaningful name to a field. Here’s an example:
query { user(id: "123") { firstName lastName friends: connections { edges { node { id name } } } } }
In this example, we alias the field “connections” to “friends” to make the query more readable and intuitive.
Related Article: How to Validate IPv4 Addresses Using Regex
2. Fragments
Fragments in GraphQL allow you to define reusable sets of fields that can be included in multiple queries. This can help reduce duplication and make your queries more modular. Here’s an example:
fragment UserInfo on User { firstName lastName } query { user(id: "123") { ...UserInfo friends { ...UserInfo } } }
In this example, we define a fragment called “UserInfo” that includes the fields “firstName” and “lastName”. We then include this fragment in both the “user” and “friends” fields to avoid repeating the same fields in multiple places.
3. Variables
Variables in GraphQL allow you to pass dynamic values into your queries. This can be useful when you need to parameterize your queries based on user input or other factors. Here’s an example:
query GetUser($id: ID!) { user(id: $id) { firstName lastName } }
In this example, we define a variable called “$id” of type “ID!” (non-null ID) and use it in the query to fetch a specific user based on the provided ID.
4. Directives
Directives in GraphQL allow you to conditionally include or exclude fields based on certain criteria. This can be useful when you want to control the shape of your response based on runtime conditions. Here’s an example:
query { user(id: "123") { firstName lastName friends @include(if: $includeFriends) { edges { node { id name } } } } }
In this example, we use the “@include” directive to conditionally include the “friends” field based on the value of the variable “$includeFriends”. This allows us to control whether or not to fetch the user’s friends along with their basic information.
These advanced techniques for querying with GraphQL can help you build more flexible and efficient APIs. By leveraging aliases, fragments, variables, and directives, you can tailor your queries to suit your specific needs and optimize your data fetching process.
Related Article: How to Use the aria-label Attribute in HTML
Advanced Techniques for Requesting Data with REST
When working with REST APIs, there are several advanced techniques that can be used to request data more efficiently and effectively. These techniques can help improve performance, reduce bandwidth usage, and provide more flexibility in retrieving the desired data.
1. Pagination
Pagination is a technique used to limit the amount of data returned in a single response, especially when dealing with large datasets. By specifying the number of items to retrieve per page and the page number, the API can return only the necessary subset of data. This can be achieved by adding query parameters to the URL, such as ?page=2&limit=10
, where page
represents the page number and limit
represents the number of items per page.
2. Filtering
Sometimes, you may only need a subset of data that meets specific criteria. Filtering allows you to specify certain conditions to retrieve only the relevant data. This can be done by adding query parameters to the URL, such as ?status=active&category=electronics
, where status
and category
are the filter criteria.
Related Article: How to Restrict HTML File Input to Only PDF and XLS
3. Sorting
Sorting allows you to retrieve data in a specific order based on a particular attribute. This can be useful when you want to display the data in a specific sequence. By adding a query parameter to the URL, such as ?sort=price
, the API can return the data sorted by the specified attribute.
4. Sparse Fieldsets:
In some cases, you may only require a subset of fields from a resource rather than all of its attributes. Sparse fieldsets allow you to specify the fields you need, reducing the amount of data transferred over the network. This can be achieved by adding a fields
parameter to the URL, such as ?fields=name,price
, where name
and price
are the desired fields.
5. Caching
Caching is a technique used to store responses at various levels (client, server, or intermediate cache) to avoid making redundant requests for the same data. By including appropriate caching headers in the API responses, such as Cache-Control
and ETag
, the client or intermediary caches can cache the response and serve it directly without contacting the server, resulting in improved performance.
Related Article: How to Implement HTML Select Multiple As a Dropdown
6. Batch Requests
Batching requests allows you to combine multiple API requests into a single request, reducing the number of round trips and improving efficiency. This can be achieved by sending an array of requests to a specific endpoint, such as POST /batch
, with each request having its own URL, method, and parameters.
These advanced techniques can greatly enhance your experience when working with REST APIs. By utilizing pagination, filtering, sorting, sparse fieldsets, caching, and batch requests, you can optimize your data retrieval process and improve the overall performance of your applications.
Handling Errors with GraphQL
When it comes to error handling, GraphQL and REST take different approaches. In REST, error handling is typically done using HTTP status codes and error messages returned in the response body. On the other hand, GraphQL has a more structured approach to handling errors.
In GraphQL, errors are returned alongside the data in the response. The response always has a top-level key called “errors” that contains an array of error objects. Each error object has a message field that describes the error. This allows clients to easily parse and handle errors in a consistent manner.
Here’s an example of a GraphQL response with errors:
{ "data": { "user": null }, "errors": [ { "message": "User not found" } ] }
The errors
array can contain multiple error objects if there are multiple errors in the request. This allows GraphQL to return all the errors in a single response instead of having to make multiple requests.
In addition to the error message, GraphQL also provides other fields in the error object that can be useful for handling errors. These include locations
, which indicates where in the query the error occurred, and path
, which indicates the path to the field that caused the error.
Here’s an example of a more detailed GraphQL error response:
{ "data": { "user": null }, "errors": [ { "message": "User not found", "locations": [ { "line": 3, "column": 7 } ], "path": [ "user" ] } ] }
With this additional information, clients can provide more meaningful error messages to the user or take specific actions based on the type or location of the error.
GraphQL also allows for custom error types. You can define your own error types in the GraphQL schema and use them to categorize and handle different types of errors. This can be useful when you want to provide more specific error information to the client.
Overall, GraphQL’s approach to error handling provides a more structured and flexible way to handle errors compared to REST. By including errors directly in the response, clients can easily parse and handle errors without having to rely on HTTP status codes or parsing error messages from the response body.
Handling Errors with REST
When working with REST APIs, handling errors is an essential part of the development process. REST APIs typically return error responses using HTTP status codes and error messages in the response body.
HTTP status codes provide a standardized way to indicate the success or failure of a request. Some commonly used status codes for error handling in REST APIs include:
– 400 Bad Request: This status code is used when the client sends a malformed request, such as missing or incorrect parameters.
– 401 Unauthorized: Indicates that the client must authenticate itself to access the requested resource.
– 403 Forbidden: Similar to 401, but the client does not have the necessary permissions to access the requested resource even after authentication.
– 404 Not Found: This status code is returned when the requested resource does not exist.
– 500 Internal Server Error: Indicates a server-side error occurred while processing the request.
In addition to the status codes, REST APIs can also include error messages in the response body. These error messages can provide additional information about the cause of the error and how to resolve it.
Here’s an example of how a REST API might handle an error using JSON as the response format:
HTTP/1.1 400 Bad Request Content-Type: application/json { "error": { "code": 400, "message": "Invalid request parameters" } }
In this example, the API returns a 400 Bad Request status code along with a JSON object containing an error code and message.
It’s important to provide meaningful and informative error messages to help developers understand what went wrong. Including error codes can also help automate error handling on the client side.
When consuming REST APIs, it’s crucial to handle these error responses appropriately. Clients should check the HTTP status code and parse the response body to determine the cause of the error. Proper error handling can improve the user experience and make troubleshooting easier.
In summary, REST APIs handle errors using HTTP status codes and error messages in the response body. It’s essential for both API providers and consumers to understand how to interpret and handle these error responses to ensure robust and reliable communication between client and server.
Related Article: How to Use the Regex Negation (Not) Operator
Caching Strategies with GraphQL
Caching is an important aspect of any web application to improve performance and reduce unnecessary network requests. In this chapter, we will explore different caching strategies that can be used with GraphQL.
Cache at the network layer
One common approach to caching in GraphQL is to cache responses at the network layer. This can be achieved by utilizing HTTP caching mechanisms such as ETag and Last-Modified headers. When a client makes a request, the server can include these headers in the response, allowing the client to cache the response locally. Subsequent requests can then be served from the cache without making a round trip to the server.
Here’s an example of how you can implement caching at the network layer using the ETag header in an Express.js server:
app.get('/graphql', (req, res) => { const cacheKey = req.url; const cachedResponse = cache.get(cacheKey); if (cachedResponse && cachedResponse.etag === req.headers['if-none-match']) { res.status(304).end(); // Not Modified } else { const response = // Generate the response const etag = // Calculate the ETag cache.set(cacheKey, { etag, response }); res.set('ETag', etag); res.send(response); } });
Cache at the field level
Another interesting caching strategy in GraphQL is to cache at the field level. Since GraphQL allows clients to specify the exact data they need, we can cache the results of individual fields and reuse them across multiple queries. This can be particularly useful when dealing with complex and expensive data fetching operations.
To implement field-level caching, you can use a caching library such as Redis or Memcached. These libraries allow you to store key-value pairs in memory, making it easy to cache the results of individual fields. You can use the unique field name and arguments as the cache key and store the corresponding result as the value.
Here’s an example of how you can implement field-level caching using Redis in a GraphQL resolver:
import Redis from 'ioredis'; const redis = new Redis(); const resolvers = { Query: { user: async (_, { id }) => { const cacheKey = `user:${id}`; const cachedResult = await redis.get(cacheKey); if (cachedResult) { return JSON.parse(cachedResult); } const result = // Fetch user data from the database redis.set(cacheKey, JSON.stringify(result)); return result; }, }, };
Related Article: How to Ignore Case Sensitivity with Regex (Case Insensitive)
Cache control directives
In addition to the above caching strategies, GraphQL also provides cache control directives that allow you to control caching behavior at the schema level. These directives can be used to specify cache max age, public or private caching, and cache revalidation strategies.
The most commonly used cache control directives are @cacheControl
and @deprecated
. The @cacheControl
directive allows you to specify caching rules for a particular field, while the @deprecated
directive allows you to mark a field as deprecated and provide additional information.
Here’s an example of how you can use the @cacheControl
directive in a GraphQL schema:
type Query { user(id: ID!): User! @cacheControl(maxAge: 3600) }
This example specifies that the user
field should be cached for a maximum of 3600 seconds (1 hour).
Caching Strategies with REST
Caching is an essential aspect of building efficient and scalable web applications. It allows us to store the response of a request and reuse it instead of making the same request again. This can greatly improve the performance of our applications and reduce the load on our servers.
In RESTful architectures, there are several caching strategies that can be employed to optimize the usage of resources. Let’s take a look at some of the common strategies:
Server-Side Caching
This strategy involves caching the response on the server side before sending it back to the client. The server can use various techniques such as in-memory caching or distributed caching to store the responses. The cache can be configured to expire after a certain period or based on specific conditions.
Here’s an example of how server-side caching can be implemented using a popular Node.js caching library called “node-cache”:
const NodeCache = require('node-cache'); const cache = new NodeCache(); app.get('/api/users', (req, res) => { const cacheKey = 'users'; // Check if the response is cached const cachedResponse = cache.get(cacheKey); if (cachedResponse) { return res.json(cachedResponse); } // If not cached, fetch the data from the database const users = await User.find(); // Store the response in the cache cache.set(cacheKey, users); res.json(users); });
Related Article: How to Reverse a Linked List in Python, C and Java
Client-Side Caching
This strategy involves caching the response on the client side, usually in the browser’s cache. The client can use the “Cache-Control” header to specify how the response should be cached. This strategy is particularly useful for static resources that don’t change frequently, such as images, CSS files, or JavaScript files.
Here’s an example of how client-side caching can be implemented using the “Cache-Control” header:
app.get('/static/image.jpg', (req, res) => { const imagePath = path.join(__dirname, '/static/image.jpg'); // Set the caching headers res.setHeader('Cache-Control', 'public, max-age=3600'); // Cache for 1 hour res.sendFile(imagePath); });
Conditional Requests
This strategy involves using conditional requests to check if a resource has changed since it was last requested. This can be done using the “If-Modified-Since” or “If-None-Match” headers. If the resource has not changed, the server can respond with a “304 Not Modified” status code, indicating that the client can use its cached version.
Here’s an example of how conditional requests can be implemented:
app.get('/api/posts/:id', (req, res) => { const postId = req.params.id; // Check if the post has been modified const lastModified = new Date(post.lastModified); const ifModifiedSince = req.header('If-Modified-Since'); if (ifModifiedSince && lastModified <= new Date(ifModifiedSince)) { return res.status(304).end(); // Not modified } // If modified, send the updated post res.set('Last-Modified', lastModified.toUTCString()); res.json(post); });
These are just a few examples of caching strategies that can be used with RESTful APIs. The choice of strategy depends on the specific requirements of your application and the type of resources being served.
It’s important to note that caching can introduce some challenges, such as cache invalidation and cache coherence. It’s crucial to carefully design and test your caching strategy to ensure it works effectively and doesn’t cause any unexpected issues.
In the next chapter, we will explore how caching can be implemented with GraphQL and compare it with the RESTful approaches discussed here.
Security Considerations for GraphQL
GraphQL is a powerful query language that offers flexibility and efficiency in fetching data from a server. However, like any technology, it is important to consider security when implementing GraphQL in your applications. In this chapter, we will explore some key security considerations for GraphQL and how it compares to REST.
Related Article: Tutorial: Working with Stacks in C
1. Authorization and Authentication
One of the fundamental aspects of securing any API is implementing proper authorization and authentication mechanisms. With GraphQL, you have the flexibility to define your own authentication and authorization logic. You can use middleware or custom resolver functions to enforce access control rules based on user roles or permissions.
For example, let’s say we have a GraphQL resolver that fetches a user’s email address:
type Query { getUserEmail: String! }
To protect this resolver, you can add a middleware function that checks if the user making the request is authenticated and has the necessary permissions to access the email address:
const getUserEmailResolver = async (root, args, context) => { if (!context.user) { throw new Error("Unauthorized"); } // Check user permissions // Fetch and return the email address };
2. Query Depth and Complexity
GraphQL allows clients to request nested data structures, which can potentially lead to complex and expensive queries. This can be abused by malicious users to perform denial-of-service attacks or overload your server with resource-intensive queries.
To mitigate this risk, it is recommended to set limits on the query depth and complexity. You can use tools like graphql-depth-limit
or graphql-cost-analysis
to enforce these limits.
For example, using graphql-depth-limit
, you can set a maximum depth of 10 for queries:
const depthLimit = require("graphql-depth-limit"); app.use( "/graphql", expressGraphQL({ schema, validationRules: [depthLimit(10)], }) );
3. Input Validation
Input validation is crucial to prevent attacks like SQL injection or arbitrary code execution. With GraphQL, you can define input types and use validation libraries to ensure the data sent by clients is safe.
For example, let’s say we have a mutation that creates a new user:
type Mutation { createUser(input: CreateUserInput!): User! } input CreateUserInput { username: String! password: String! }
To validate the input, you can use a library like joi
:
const Joi = require("joi"); const createUserResolver = async (root, args) => { const { input } = args; const schema = Joi.object({ username: Joi.string().required(), password: Joi.string().required(), }); const { error } = schema.validate(input); if (error) { throw new Error("Invalid input"); } // Create the user };
Related Article: How to Use Generics in Go
4. Rate Limiting
To protect your server from abuse or unexpected traffic spikes, it is important to implement rate limiting. Rate limiting prevents clients from making an excessive number of requests within a given time frame.
You can use libraries like express-rate-limit
or services like AWS API Gateway to enforce rate limits on your GraphQL API.
For example, using express-rate-limit
, you can limit clients to 100 requests per hour:
const rateLimit = require("express-rate-limit"); const limiter = rateLimit({ windowMs: 60 * 60 * 1000, // 1 hour max: 100, }); app.use("/graphql", limiter);
5. Secure GraphQL Endpoints
When deploying your GraphQL API, it is crucial to secure the endpoints to prevent unauthorized access or tampering of data. Here are some best practices to follow:
– Use HTTPS to encrypt data transmitted between clients and the server.
– Implement CORS (Cross-Origin Resource Sharing) to restrict access from unauthorized domains.
– Disable GraphQL Playground or GraphiQL in production to prevent exposing sensitive information.
By following these security considerations, you can ensure that your GraphQL API is secure and protected against common vulnerabilities.
In the next chapter, we will explore the performance aspects of GraphQL and how it compares to REST.
Security Considerations for REST
When building an API, security is a crucial aspect to consider. RESTful APIs have been around for a long time and have well-established security practices. However, it’s important to be aware of certain security considerations when using REST.
Related Article: How to Use If-Else Statements in Shell Scripts
1. Authentication and Authorization
Authentication ensures that users are who they claim to be, while authorization determines what actions they are allowed to perform. REST APIs commonly use token-based authentication, where a token is issued by the server upon successful login and is then sent with each subsequent request to authenticate the user.
Here’s an example of how token-based authentication can be implemented using JSON Web Tokens (JWT) in a REST API:
// server.js const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); app.post('/login', (req, res) => { // Authenticate user and generate token const user = { id: 1, username: 'john.doe' }; const token = jwt.sign(user, 'secret_key'); res.json({ token }); }); app.get('/protected', (req, res) => { // Verify token and authorize user const token = req.headers.authorization.split(' ')[1]; jwt.verify(token, 'secret_key', (err, decoded) => { if (err) { res.sendStatus(403); } else { res.json({ message: 'Access granted!' }); } }); }); app.listen(3000, () => { console.log('Server started on port 3000'); });
2. Transport Layer Security (TLS)
To ensure secure communication between the client and server, it’s recommended to use Transport Layer Security (TLS) or its predecessor, Secure Sockets Layer (SSL). This provides encryption and authentication, preventing eavesdropping and tampering of data in transit.
Implementing TLS requires obtaining an SSL certificate and configuring the server to use it. Let’s Encrypt (https://letsencrypt.org/) is a widely used certificate authority that provides free SSL certificates.
3. Input Validation
One common security vulnerability is the lack of input validation, which can lead to injection attacks such as SQL injection or cross-site scripting (XSS). It’s essential to validate and sanitize all user input to prevent these types of attacks.
Here’s an example of input validation using regular expressions in a Node.js REST API:
// server.js const express = require('express'); const app = express(); app.post('/users', (req, res) => { const username = req.body.username; // Validate username format if (!/^[a-zA-Z0-9]+$/.test(username)) { res.status(400).json({ error: 'Invalid username format' }); } else { // Save user to the database res.json({ message: 'User created successfully' }); } }); app.listen(3000, () => { console.log('Server started on port 3000'); });
Related Article: How to Implement and Use Generics in Typescript
4. Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) is a security mechanism that restricts cross-origin requests. By default, browsers enforce the same-origin policy, which prevents requests from different origins. However, when building APIs, it’s often necessary to allow cross-origin requests from specific origins.
In a Node.js application, you can enable CORS using the ‘cors’ middleware:
// server.js const express = require('express'); const cors = require('cors'); const app = express(); app.use(cors()); // Define API routes... app.listen(3000, () => { console.log('Server started on port 3000'); });
5. Rate Limiting
To protect your API from abuse or denial-of-service attacks, it’s recommended to implement rate limiting. Rate limiting restricts the number of requests a client can make within a certain time frame.
There are various rate limiting strategies available, such as limiting requests per IP address or per user account. Tools like ‘express-rate-limit’ (https://www.npmjs.com/package/express-rate-limit) can help implement rate limiting in a Node.js application.
These are just a few security considerations to keep in mind when using REST. It’s important to stay updated with the latest security practices and regularly audit your API for vulnerabilities. Remember, security is an ongoing process that requires continuous attention.