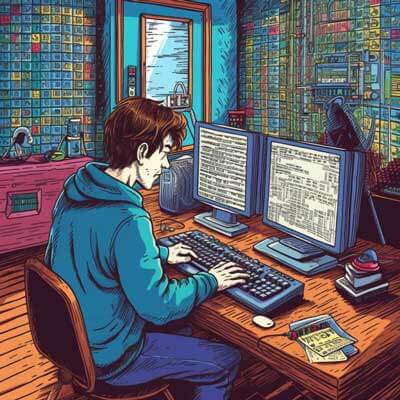
- Introduction to Min Heap Binary Trees
- Basic Concepts of Min Heap Binary Trees
- Heap Property
- Complete Binary Tree
- Building Blocks of Min Heap Binary Trees
- Array Representation
- Heapify Operation
- Structure and Properties of Min Heap
- Structure
- Properties
- Implementation of Min Heap Operations
- Insertion
- Deletion
- Code Snippet: Building a Min Heap
- Code Snippet: Heapify Operation
- Code Snippet: Extract Minimum Operation
- Use Case: Priority Queue Implementation
- Use Case: Kth Smallest Element in an Array
- Use Case: Median in a Stream of Integers
- Real World Example: Task Scheduling
- Real World Example: Network Traffic Packet Prioritization
- Best Practice: Choosing the Right Data Structures
- Best Practice: Optimal Memory Utilization
- Performance Consideration: Time Complexity of Operations
- Performance Consideration: Space Complexity of Heap
- Advanced Technique: Decrease Key Operation
- Advanced Technique: Increase Key Operation
- Advanced Technique: Extended Heapify Function
- Error Handling: Dealing with Overflow
- Error Handling: Underflow Problems
- Error Handling: Invalid Key Errors
Introduction to Min Heap Binary Trees
A Min Heap is a specialized type of binary tree that satisfies the heap property. It is a complete binary tree where each parent node has a value less than or equal to the values of its children. This property ensures that the minimum value is always at the root of the tree.
Min Heap Binary Trees are commonly used in various applications, such as priority queues, sorting algorithms, and graph algorithms. Understanding the concepts and implementation of Min Heap Binary Trees is essential for efficient problem-solving.
Related Article: How to Validate IPv4 Addresses Using Regex
Basic Concepts of Min Heap Binary Trees
To understand Min Heap Binary Trees, it is important to grasp the following basic concepts:
Heap Property
The heap property of a Min Heap Binary Tree ensures that each parent node has a value less than or equal to the values of its children. This property guarantees that the minimum value is always at the root.
Complete Binary Tree
A complete binary tree is a tree in which all levels, except possibly the last, are completely filled, and all nodes are as left as possible. Min Heap Binary Trees are complete binary trees.
Related Article: Troubleshooting 502 Bad Gateway Nginx
Building Blocks of Min Heap Binary Trees
To build a Min Heap Binary Tree, we need to understand the following building blocks:
Array Representation
A Min Heap Binary Tree can be efficiently represented using an array. The array starts from index 1, and each element at index i represents a node in the tree. The index of a node’s left child is 2i, and the index of its right child is 2i + 1.
Heapify Operation
Heapify is an operation that maintains the heap property of a binary tree. It is used during insertion, deletion, and building a Min Heap. Heapify compares the node with its children and swaps them if necessary to satisfy the heap property.
Related Article: How to Do Sorting in C++ & Sorting Techniques
Structure and Properties of Min Heap
A Min Heap Binary Tree has the following structure and properties:
Structure
A Min Heap Binary Tree is a complete binary tree where each parent node has a value less than or equal to the values of its children. The minimum value is always at the root.
Properties
– The value of a parent node is less than or equal to the values of its children.
– The minimum value is always at the root.
– The height of a Min Heap Binary Tree is logarithmic to the number of nodes.
Related Article: How to Use JSON Parse and Stringify in JavaScript
Implementation of Min Heap Operations
To implement Min Heap operations, we need to understand the following:
Insertion
Insertion in a Min Heap involves adding a new element while maintaining the heap property. The new element is inserted at the next available position in the array representation of the Min Heap. We then perform the heapify operation to maintain the heap property.
Example code snippet in Python:
def insert(heap, value): heap.append(value) i = len(heap) - 1 while i > 1 and heap[i//2] > heap[i]: heap[i//2], heap[i] = heap[i], heap[i//2] i = i//2
Deletion
Deletion in a Min Heap involves removing the minimum element, which is always at the root. We replace the root with the last element in the array representation of the Min Heap and perform the heapify operation to maintain the heap property.
Example code snippet in Python:
def delete(heap): if len(heap) == 1: return None min_val = heap[1] heap[1] = heap[-1] heap.pop() i = 1 while (2*i < len(heap) and heap[i] > heap[2*i]) or (2*i+1 < len(heap) and heap[i] > heap[2*i+1]): if 2*i+1 < len(heap) and heap[2*i+1] < heap[2*i]: heap[i], heap[2*i+1] = heap[2*i+1], heap[i] i = 2*i+1 else: heap[i], heap[2*i] = heap[2*i], heap[i] i = 2*i return min_val
Related Article: How to Implement and Use Generics in Typescript
Code Snippet: Building a Min Heap
Building a Min Heap involves converting an array into a Min Heap. We start from the last non-leaf node and perform the heapify operation on each node in reverse order.
Example code snippet in Python:
def build_heap(arr): heap = [None] + arr n = len(arr) // 2 for i in range(n, 0, -1): j = i while (2*j < len(heap) and heap[j] > heap[2*j]) or (2*j+1 < len(heap) and heap[j] > heap[2*j+1]): if 2*j+1 < len(heap) and heap[2*j+1] < heap[2*j]: heap[j], heap[2*j+1] = heap[2*j+1], heap[j] j = 2*j+1 else: heap[j], heap[2*j] = heap[2*j], heap[j] j = 2*j return heap[1:]
Code Snippet: Heapify Operation
The heapify operation is a crucial part of maintaining the heap property in a Min Heap. It compares the node with its children and swaps them if necessary to satisfy the heap property.
Example code snippet in Python:
def heapify(heap, i): left = 2 * i right = 2 * i + 1 smallest = i if left < len(heap) and heap[left] < heap[i]: smallest = left if right < len(heap) and heap[right] < heap[smallest]: smallest = right if smallest != i: heap[i], heap[smallest] = heap[smallest], heap[i] heapify(heap, smallest)
Code Snippet: Extract Minimum Operation
The extract minimum operation removes the minimum element, which is always at the root of the Min Heap. It then restores the heap property by performing the heapify operation.
Example code snippet in Python:
def extract_min(heap): if len(heap) <= 1: return None min_val = heap[1] heap[1] = heap[-1] heap.pop() heapify(heap, 1) return min_val
Related Article: How to Use If-Else Statements in Shell Scripts
Use Case: Priority Queue Implementation
One of the common use cases for Min Heap Binary Trees is implementing a priority queue. A priority queue is a data structure that allows efficient insertion and retrieval of elements based on their priority.
By using a Min Heap Binary Tree as the underlying data structure, we can achieve the following time complexities:
– Insertion: O(log n)
– Retrieval of the minimum element: O(1)
This makes Min Heap Binary Trees a suitable choice for implementing a priority queue.
Use Case: Kth Smallest Element in an Array
Another use case for Min Heap Binary Trees is finding the kth smallest element in an array efficiently. By building a Min Heap from the array and extracting the minimum element k times, we can find the kth smallest element in O(n + k log n) time.
Example code snippet in Python:
def kth_smallest(arr, k): heap = build_heap(arr) for _ in range(k-1): extract_min(heap) return heap[0]
Use Case: Median in a Stream of Integers
Finding the median in a stream of integers is another use case for Min Heap Binary Trees. By using two Min Heaps, one to store the smaller half of the elements and the other to store the larger half, we can efficiently find the median.
Example code snippet in Python:
import heapq class MedianFinder: def __init__(self): self.min_heap = [] self.max_heap = [] def addNum(self, num): heapq.heappush(self.max_heap, -num) heapq.heappush(self.min_heap, -heapq.heappop(self.max_heap)) if len(self.min_heap) > len(self.max_heap): heapq.heappush(self.max_heap, -heapq.heappop(self.min_heap)) def findMedian(self): if len(self.min_heap) == len(self.max_heap): return (self.min_heap[0] - self.max_heap[0]) / 2 else: return -self.max_heap[0]
Related Article: How to Use Generics in Go
Real World Example: Task Scheduling
Min Heap Binary Trees can be used in task scheduling algorithms, where tasks have different priorities and need to be executed in a specific order. By using a Min Heap to store the tasks, we can efficiently retrieve the task with the highest priority for execution.
The tasks can be represented as objects with a priority value. Each task is inserted into the Min Heap, and the heapify operation maintains the heap property based on the priority value. The task with the highest priority can be extracted from the Min Heap.
Real World Example: Network Traffic Packet Prioritization
In network systems, packets often have different priorities based on their importance or time sensitivity. Min Heap Binary Trees can be used to prioritize the processing and transmission of network packets.
By using a Min Heap to store the packets, we can efficiently retrieve the packet with the highest priority for processing or transmission. The packets can be represented as objects with a priority value, and the Min Heap ensures that the packet with the highest priority is always at the root.
Best Practice: Choosing the Right Data Structures
When implementing algorithms or solving problems that involve sorting, prioritization, or efficient retrieval of elements, considering the use of Min Heap Binary Trees is a best practice. Min Heap Binary Trees provide a suitable data structure that guarantees efficient operations such as insertion, deletion, and retrieval of the minimum element.
However, it is important to analyze the problem requirements and constraints before choosing the right data structure. Depending on the specific use case, other data structures such as balanced binary search trees or hash tables may provide better performance or additional features.
Related Article: Tutorial: Working with Stacks in C
Best Practice: Optimal Memory Utilization
To optimize memory utilization when implementing Min Heap Binary Trees, it is recommended to use an array-based representation. This representation eliminates the need for additional pointers or memory allocations, resulting in more efficient memory utilization.
The array-based representation also allows for better cache locality and reduces the overhead of pointer traversal. However, it is important to consider the potential dynamic resizing of the array when the number of elements exceeds the initial capacity.
Performance Consideration: Time Complexity of Operations
The time complexity of operations in Min Heap Binary Trees is an important performance consideration:
– Insertion: O(log n)
– Deletion: O(log n)
– Retrieval of the minimum element: O(1)
– Building a Min Heap: O(n)
These time complexities make Min Heap Binary Trees suitable for applications that require efficient insertion, deletion, and retrieval of the minimum element.
Performance Consideration: Space Complexity of Heap
The space complexity of a Min Heap Binary Tree depends on the number of elements stored in the heap. In the array-based representation, the space complexity is O(n), where n is the number of elements in the heap.
It is important to consider the space requirements when working with large datasets or in memory-constrained environments. Additionally, the space complexity can be influenced by any additional data associated with each element in the heap.
Related Article: How to Reverse a Linked List in Python, C and Java
Advanced Technique: Decrease Key Operation
The decrease key operation is an advanced technique that allows modifying the value of a key in a Min Heap and maintaining the heap property. This operation is useful in scenarios where the priority or value of an element needs to be updated.
Example code snippet in Python:
def decrease_key(heap, i, new_value): if i < 1 or i >= len(heap): return heap[i] = new_value while i > 1 and heap[i//2] > heap[i]: heap[i//2], heap[i] = heap[i], heap[i//2] i = i//2
Advanced Technique: Increase Key Operation
The increase key operation is another advanced technique that allows modifying the value of a key in a Min Heap and maintaining the heap property. This operation is useful in scenarios where the priority or value of an element needs to be updated.
Example code snippet in Python:
def increase_key(heap, i, new_value): if i < 1 or i >= len(heap): return heap[i] = new_value heapify(heap, i)
Advanced Technique: Extended Heapify Function
The extended heapify function is an advanced technique that allows heapifying a specific range of elements in a Min Heap. This operation can be useful in scenarios where only a portion of the heap needs to be maintained or modified.
Example code snippet in Python:
def extended_heapify(heap, start, end): for i in range(end, start - 1, -1): heapify(heap, i)
Related Article: How to Ignore Case Sensitivity with Regex (Case Insensitive)
Error Handling: Dealing with Overflow
When implementing Min Heap Binary Trees, it is important to consider potential overflow scenarios. Overflow can occur when the number of elements exceeds the capacity of the underlying data structure, such as an array.
To handle overflow, it is recommended to dynamically resize the array or use a resizable data structure that can accommodate a larger number of elements. Additionally, considering the memory constraints and available resources is crucial to avoid performance degradation or system failures.
Error Handling: Underflow Problems
Underflow problems can occur when attempting to extract the minimum element from an empty Min Heap. To handle underflow, it is important to check if the heap is empty before performing the extraction operation. Proper error handling or exception mechanisms should be in place to handle such scenarios and prevent unexpected program behavior.
Error Handling: Invalid Key Errors
Invalid key errors can occur when attempting to access or modify elements in a Min Heap using an invalid index or key. To handle invalid key errors, it is recommended to perform proper bounds checking and validate the input before accessing or modifying the heap. Implementing appropriate error handling mechanisms helps ensure the correctness and reliability of the code.