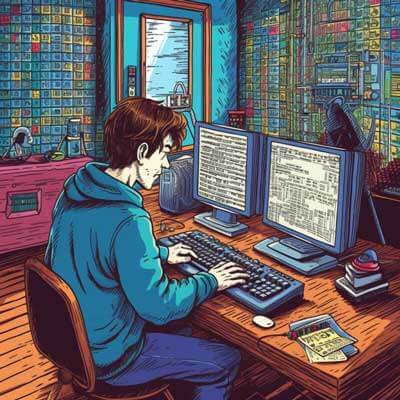
Table of Contents
HTTP (Hypertext Transfer Protocol) is the foundation of data communication on the web. It enables the exchange of information between a client (such as a web browser) and a server. When working with HTTP, it's important to understand the differences between various methods, such as POST and PUT.
Why is the distinction between POST and PUT important?
The question of how to distinguish between POST and PUT in HTTP arises because these two methods have different purposes and behaviors. Understanding the differences is crucial for building robust and well-designed web applications.
When a client makes an HTTP request, it specifies the method to be used. The server then interprets the method and performs the appropriate action. In the case of POST and PUT, the server needs to handle the requests differently based on their semantics.
Related Article: How To Display Local Image In Markdown
POST method
The POST method is used to submit data to be processed by the identified resource. It is commonly used for creating new resources on the server or submitting data to update an existing resource. Some key characteristics of the POST method include:
- It is not idempotent, meaning that sending the same request multiple times may result in different outcomes.
- The request payload typically contains the data to be processed by the server.
- The server may respond with a status code indicating the success or failure of the operation, along with any relevant data.
Here's an example of a POST request in HTTP:
POST /api/users HTTP/1.1 Host: example.com Content-Type: application/json { "name": "John Doe", "email": "john@example.com" }
In this example, the client is submitting data to create a new user. The server will process the request and respond with a success status code (e.g., 201 Created) if the user is successfully created.
PUT method
The PUT method is used to update or replace an existing resource on the server. It is idempotent, meaning that sending the same request multiple times will have the same effect as sending it once. Key characteristics of the PUT method include:
- It requires the client to send the entire representation of the resource in the request payload.
- If the resource already exists, the server will update it with the provided data. If the resource does not exist, the server may create a new resource based on the provided data.
- The server may respond with a success status code (e.g., 200 OK) or a status code indicating the result of the operation.
Here's an example of a PUT request in HTTP:
PUT /api/users/123 HTTP/1.1 Host: example.com Content-Type: application/json { "name": "John Doe", "email": "john@example.com" }
In this example, the client is updating the user with the ID 123. The server will update the user's information based on the provided data and respond with a success status code if the update is successful.
How to distinguish between POST and PUT
To distinguish between POST and PUT in HTTP, consider the following guidelines:
1. Semantics: Understand the purpose and semantics of the HTTP methods. POST is used for creating resources or submitting data to update existing resources, while PUT is used to update or replace existing resources.
2. Idempotency: Consider whether the operation should be idempotent. If sending the same request multiple times should have the same effect, use the PUT method. If the operation is not idempotent, use the POST method.
3. Payload: Determine the nature of the data being sent. POST requests typically include a payload containing the data to be processed, while PUT requests require the entire representation of the resource being updated.
4. Resource identification: Consider how the resource is identified in the request URL. POST requests typically target a collection or a resource creation endpoint, while PUT requests target a specific resource identified by its unique identifier.
5. Response codes: Pay attention to the response codes returned by the server. POST requests may result in a variety of status codes, depending on the outcome of the operation, while PUT requests typically result in a success status code if the update is successful.
Related Article: Comparing GraphQL vs REST & How To Manage APIs
Best practices and considerations
When working with POST and PUT in HTTP, it's important to follow best practices to ensure the reliability and maintainability of your web applications. Consider the following tips:
- Use appropriate status codes: Return the appropriate HTTP status codes to indicate the result of the operation. HTTP status codes provide valuable information to clients and can help with error handling and troubleshooting.
- Validate input: Perform validation on the input data to ensure it meets the required criteria. This helps prevent invalid or malicious data from being processed or stored.
- Protect sensitive data: If the data being submitted includes sensitive information, consider using secure connections (HTTPS) to protect the confidentiality and integrity of the data during transit.
- Handle errors gracefully: Implement error handling mechanisms to handle cases where the server encounters an error during processing. Provide meaningful error messages to clients to assist with troubleshooting.
- Consider using frameworks and libraries: When developing web applications, consider using frameworks or libraries that provide abstractions for handling HTTP requests and methods. These tools can simplify the process of distinguishing between POST and PUT and help enforce best practices.
Alternative ideas
While POST and PUT are commonly used methods for creating and updating resources, there are alternative approaches in some scenarios:
- PATCH method: The PATCH method can be used to partially update a resource, rather than requiring the entire representation to be sent. This can be useful when updating only specific fields or properties of a resource.
- Custom methods: In some cases, it may be appropriate to define custom HTTP methods to handle specific operations that don't fit the semantics of POST or PUT. However, this approach should be used judiciously and adhere to established conventions and standards.