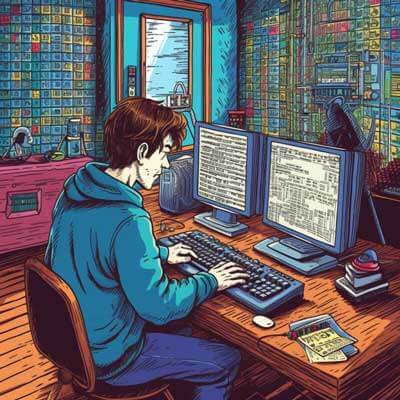
Table of Contents
Introduction to Json Server
Json Server is a lightweight and easy-to-use tool for quickly creating a RESTful API with JSON data. It allows developers to set up a mock server that responds to HTTP requests with JSON data, making it a valuable tool for front-end developers during the development and testing phases of a project. With Json Server, you can easily create a fake API that mimics the behavior of a real server, enabling you to prototype and test your application without relying on a backend server.
Related Article: Comparing Sorting Algorithms (with Java Code Snippets)
Installation and Setup of Json Server
To get started with Json Server, you need to have Node.js installed on your system. Once you have Node.js installed, you can install Json Server globally by running the following command in your terminal:
npm install -g json-server
After the installation is complete, you can create a new directory for your Json Server project and navigate to it in your terminal. Inside this directory, you can create a JSON file that will serve as your database.
For example, let's create a file named db.json
with the following content:
{ "users": [ { "id": 1, "name": "John Doe" }, { "id": 2, "name": "Jane Smith" } ] }
To start the Json Server and make it serve the db.json
file as an API, run the following command:
json-server --watch db.json
This will start the server on http://localhost:3000
by default. You can now access the API endpoints defined in your db.json
file by sending HTTP requests to this URL.
Basic Usage of Json Server
Json Server provides a set of default routes for performing CRUD operations on the data in your JSON file. By default, the routes correspond to the top-level keys in your JSON object.
For example, if you have a JSON file with a users
key, Json Server will create the following routes:
- GET /users
: Retrieves all users.
- GET /users/{id}
: Retrieves a specific user by ID.
- POST /users
: Creates a new user.
- PUT /users/{id}
: Updates an existing user.
- PATCH /users/{id}
: Partially updates an existing user.
- DELETE /users/{id}
: Deletes a user.
To retrieve all users, you can send a GET request to http://localhost:3000/users
. This will return a JSON array containing all the users in the database.
To retrieve a specific user with ID 1, you can send a GET request to http://localhost:3000/users/1
.
To create a new user, you can send a POST request to http://localhost:3000/users
with the user data in the request body.
To update an existing user with ID 1, you can send a PUT or PATCH request to http://localhost:3000/users/1
with the updated user data in the request body.
To delete a user with ID 1, you can send a DELETE request to http://localhost:3000/users/1
.
Json Server also supports filtering, sorting, and pagination. You can add query parameters to your requests to perform these operations. For example, to filter users by name, you can send a GET request to http://localhost:3000/users?name=John%20Doe
.
Creating and Configuring a Json Server
To create a new Json Server instance, you need to define a JSON file that represents your database. This file will contain the data that the server will serve as JSON responses.
You can create the JSON file manually or use a tool like Faker.js or Chance.js to generate realistic test data. For example, let's create a file named db.json
with the following content:
{ "users": [ { "id": 1, "name": "John Doe", "email": "john@example.com" }, { "id": 2, "name": "Jane Smith", "email": "jane@example.com" } ] }
Once you have your JSON file ready, you can start the Json Server by running the following command in your terminal:
json-server --watch db.json
This will start the server on http://localhost:3000
by default. You can now access the API endpoints defined in your db.json
file by sending HTTP requests to this URL.
Json Server also allows you to configure various aspects of the server, such as the port it listens on and the delay for each response. You can pass these configuration options as command-line arguments when starting the server. For example, to start the server on port 4000, you can run the following command:
json-server --watch db.json --port 4000
You can find more information about the available configuration options in the Json Server documentation.
Related Article: Agile Shortfalls and What They Mean for Developers
Use Case: Mocking an API with Json Server
Json Server is a powerful tool for mocking APIs during development and testing. By setting up a Json Server instance and defining the desired routes and responses, you can simulate the behavior of a real API and test your application without relying on the actual backend.
To mock an API with Json Server, you need to define the routes and responses in your JSON file. For example, let's say you are developing a frontend application that interacts with a RESTful API for managing books. You can create a JSON file named db.json
with the following content:
{ "books": [ { "id": 1, "title": "Book 1", "author": "Author 1" }, { "id": 2, "title": "Book 2", "author": "Author 2" } ] }
To simulate the behavior of the API, you can define the routes and responses in your JSON file. For example, to retrieve all books, you can add the following route:
{ "routes": { "/api/books": "/books" } }
This tells Json Server to map the route /api/books
to the /books
endpoint in your JSON file.
You can also define more complex routes and responses using JSON Server's filtering capabilities. For example, to retrieve all books by a specific author, you can add the following route:
{ "routes": { "/api/books?author=:author": "/books?author=:author" } }
This will allow you to send a GET request to /api/books?author=Author%201
to retrieve all books by "Author 1".
By defining the desired routes and responses in your JSON file, you can easily mock different API scenarios and test your frontend application without relying on the actual backend.
Use Case: Rapid Prototyping with Json Server
Json Server is an excellent tool for rapid prototyping, allowing you to quickly create a fake API and test your application's functionality without the need for a full-fledged backend.
To use Json Server for rapid prototyping, you can start by defining your data model in a JSON file. For example, let's say you are building a task management application and want to prototype the functionality for managing tasks. You can create a file named db.json
with the following content:
{ "tasks": [] }
Next, you can start the Json Server by running the following command in your terminal:
json-server --watch db.json
This will start the server on http://localhost:3000
by default. You can now access the API endpoints defined in your db.json
file.
To create a new task, you can send a POST request to http://localhost:3000/tasks
with the task data in the request body. For example:
curl -X POST -H "Content-Type: application/json" -d '{"title":"Task 1","completed":false}' http://localhost:3000/tasks
To retrieve all tasks, you can send a GET request to http://localhost:3000/tasks
.
To update an existing task, you can send a PUT or PATCH request to http://localhost:3000/tasks/{id}
with the updated task data in the request body.
To delete a task, you can send a DELETE request to http://localhost:3000/tasks/{id}
.
By using Json Server, you can quickly prototype the functionality of your application and iterate on it without the need for a backend server. This allows you to get feedback and make changes faster, accelerating the development process.
Best Practices: Structuring Json Data
When using Json Server, it's important to follow best practices for structuring your JSON data to ensure a clean and organized API.
One recommended approach is to organize your data in separate collections, each representing a different entity or resource in your application. For example, if you are building a blogging platform, you might have separate collections for users, posts, and comments.
Here's an example of how you can structure your JSON data for a blogging platform:
{ "users": [ { "id": 1, "name": "John Doe" }, { "id": 2, "name": "Jane Smith" } ], "posts": [ { "id": 1, "title": "Post 1", "content": "Lorem ipsum dolor sit amet." }, { "id": 2, "title": "Post 2", "content": "Lorem ipsum dolor sit amet." } ], "comments": [ { "id": 1, "postId": 1, "userId": 1, "content": "Great post!" }, { "id": 2, "postId": 1, "userId": 2, "content": "Thanks for sharing." } ] }
By structuring your data in this way, you can easily define routes and responses in your JSON file that correspond to the different collections. For example, you can define routes like /users
, /posts
, and /comments
to retrieve all users, posts, and comments, respectively.
This approach allows you to maintain a clear separation of concerns and easily manage your data in a structured manner.
Code Snippet: Basic CRUD Operations with Json Server
To perform CRUD operations with Json Server, you can use the following code snippets as examples:
- Retrieve all users:
fetch('http://localhost:3000/users') .then(response => response.json()) .then(users => console.log(users));
- Create a new user:
fetch('http://localhost:3000/users', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ name: 'John Doe' }), }) .then(response => response.json()) .then(user => console.log(user));
- Update an existing user:
fetch('http://localhost:3000/users/1', { method: 'PUT', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ name: 'Jane Smith' }), }) .then(response => response.json()) .then(user => console.log(user));
- Delete a user:
fetch('http://localhost:3000/users/1', { method: 'DELETE', }) .then(response => console.log('User deleted.'));
These code snippets demonstrate the basic CRUD operations with Json Server. You can adapt them to your specific use case and modify the endpoints and data as needed.
Related Article: Tutorial: Working with Stacks in C
Best Practices: Security Measures for Json Server
While Json Server is primarily used for development and testing purposes, it's important to take some security measures to protect your data and prevent unauthorized access.
One recommended practice is to secure your Json Server instance behind authentication. You can achieve this by using a reverse proxy server like Nginx or Apache, which can handle the authentication and forward requests to your Json Server instance.
Another security measure is to restrict access to sensitive routes or resources by implementing custom middleware in your Json Server setup. This allows you to add authentication and authorization logic to your routes, ensuring that only authenticated and authorized users can access certain endpoints.
Additionally, you should be cautious when using Json Server with sensitive data. Avoid using real or sensitive data in your development environment and be mindful of the data you include in your JSON files. If you need to test with sensitive data, consider using a local or private instance of Json Server.
It's also important to keep your Json Server instance updated with the latest version to benefit from security patches and bug fixes. Regularly check for updates and follow the recommended upgrade process to ensure the security and stability of your application.
Error Handling in Json Server
Error handling is an essential aspect of any application, including those built with Json Server. When using Json Server, you can handle errors by leveraging the built-in error handling capabilities and customizing the error responses.
Json Server provides default error responses for common HTTP status codes, such as 404 for resource not found and 500 for internal server error. These error responses include helpful information about the error, such as the status code and a brief description.
To handle errors in Json Server, you can define custom routes and responses in your JSON file. For example, you can create a route like /error
and define the desired error response. Here's an example:
{ "routes": { "/error": { "status": 500, "body": { "message": "An error occurred" } } } }
This will make Json Server respond with a 500 status code and the specified error message when a request is made to /error
.
You can also handle errors in your client-side code when interacting with the Json Server API. For example, when making requests with fetch, you can use the catch method to handle errors:
fetch('http://localhost:3000/users') .then(response => { if (!response.ok) { throw new Error('Failed to fetch users'); } return response.json(); }) .then(users => console.log(users)) .catch(error => console.error(error));
By catching errors in your client-side code, you can handle them gracefully and provide appropriate feedback to the user.
Real World Example: Implementing Json Server in a Web Application
To demonstrate how to implement Json Server in a web application, let's consider a simple task management application. This application allows users to create, update, and delete tasks.
First, set up a new Json Server instance by creating a JSON file named db.json
with the following content:
{ "tasks": [] }
Next, define the routes and responses in your JSON file to handle the CRUD operations for tasks. Here's an example:
{ "routes": { "/api/tasks": "/tasks" } }
In your web application, you can use JavaScript's fetch API to interact with the Json Server API. Here's an example of how you can implement the functionality to retrieve all tasks:
fetch('http://localhost:3000/api/tasks') .then(response => response.json()) .then(tasks => { // Display tasks in the UI console.log(tasks); }) .catch(error => { // Handle error console.error(error); });
Similarly, you can implement the functionality to create, update, and delete tasks by sending the appropriate requests to the Json Server API.
By implementing Json Server in your web application, you can quickly prototype and test the functionality without the need for a backend server. This allows you to iterate on your application faster and gather feedback early in the development process.
Real World Example: Data Visualization with Json Server
Json Server can be used in conjunction with data visualization libraries to create interactive charts and graphs based on the data in your JSON file. By retrieving the data from the Json Server API and feeding it into a data visualization library, you can create compelling visual representations of your data.
One popular data visualization library is Chart.js, which provides various chart types, including bar charts, line charts, and pie charts. Here's an example of how you can use Chart.js to visualize data from a Json Server API:
First, retrieve the data from the Json Server API using the fetch API:
fetch('http://localhost:3000/data') .then(response => response.json()) .then(data => { // Process and format the data for Chart.js const labels = data.map(item => item.label); const values = data.map(item => item.value); // Create a chart using Chart.js const ctx = document.getElementById('chart').getContext('2d'); new Chart(ctx, { type: 'bar', data: { labels, datasets: [ { label: 'Data', data: values, backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1, }, ], }, options: { responsive: true, scales: { y: { beginAtZero: true, }, }, }, }); }) .catch(error => { // Handle error console.error(error); });
In this example, the data from the Json Server API is processed and formatted to create labels and values arrays, which are then used to create a bar chart using Chart.js. The resulting chart is rendered in a canvas element with the ID chart
.
By combining Json Server with a data visualization library like Chart.js, you can create dynamic and interactive visualizations based on your JSON data.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Performance Consideration: Optimizing Json Server for Large Datasets
While Json Server is a lightweight tool, it's important to consider performance optimizations when working with large datasets to ensure a smooth and responsive experience.
One performance consideration is to limit the amount of data returned by default. By default, Json Server returns all the data in your JSON file for each request. However, if your dataset is large, this can result in slow response times and increased network traffic.
To limit the amount of data returned by default, you can use the slice
option when starting the Json Server. For example, you can use the slice
option to limit the number of items returned for each collection:
json-server --watch db.json --slice 10
This will limit the number of items returned to 10 for each collection. You can adjust the value based on your specific requirements and the size of your dataset.
Another performance optimization is to use pagination to load data incrementally. Instead of requesting all the data at once, you can implement pagination in your client-side code and request data in smaller chunks as needed. This can significantly improve performance, especially when dealing with large datasets.
To implement pagination with Json Server, you can use the _start
and _limit
query parameters in your requests. For example, to retrieve the first 10 items from a collection, you can send a GET request with the _start
and _limit
parameters:
fetch('http://localhost:3000/users?_start=0&_limit=10') .then(response => response.json()) .then(users => console.log(users));
This will return the first 10 items from the users
collection. You can adjust the values of _start
and _limit
to fetch different subsets of data.
By implementing these performance optimizations, you can ensure that Json Server performs well even with large datasets, providing a smooth and responsive experience for your users.
Performance Consideration: Minimizing Latency in Json Server
Minimizing latency is crucial for achieving optimal performance in any web application, including those built with Json Server. By reducing the time it takes for requests to reach the server and receive responses, you can improve the overall responsiveness of your application.
One way to minimize latency in Json Server is to enable compression for the responses. Compressing the responses reduces their size, resulting in faster transmission over the network. You can enable compression in Json Server by using a middleware like compression
. Here's an example of how to enable compression with Json Server:
First, install the compression
package:
npm install compression
Next, create a JavaScript file named server.js
with the following content:
const jsonServer = require('json-server'); const compression = require('compression'); const server = jsonServer.create(); const router = jsonServer.router('db.json'); const middlewares = jsonServer.defaults(); server.use(compression()); server.use(middlewares); server.use(router); const port = 3000; server.listen(port, () => { console.log(`Json Server is running on port ${port}`); });
This sets up a Json Server instance with compression enabled. You can start the server by running the following command:
node server.js
Another way to minimize latency is to enable caching for the responses. By caching the responses, you can serve them directly from the cache without hitting the server, reducing the round-trip time. You can enable caching in Json Server by using a caching middleware like apicache
. Here's an example of how to enable caching with Json Server:
First, install the apicache
package:
npm install apicache
Next, create a JavaScript file named server.js
with the following content:
const jsonServer = require('json-server'); const apicache = require('apicache'); const server = jsonServer.create(); const router = jsonServer.router('db.json'); const middlewares = jsonServer.defaults(); const cache = apicache.middleware; server.use(cache('5 minutes')); server.use(middlewares); server.use(router); const port = 3000; server.listen(port, () => { console.log(`Json Server is running on port ${port}`); });
This sets up a Json Server instance with caching enabled for 5 minutes. You can adjust the caching duration based on your specific requirements.
By enabling compression and caching in Json Server, you can minimize latency and improve the performance of your application, resulting in a faster and more responsive user experience.
Advanced Technique: Custom Routes in Json Server
Json Server allows you to create custom routes to handle more complex scenarios or implement specific business logic in your API. Custom routes can be useful when you need to perform additional operations that are not covered by the default routes.
To create a custom route in Json Server, you can define the desired route and response in your JSON file. For example, let's say you want to implement a custom route to retrieve all completed tasks. You can add the following route to your JSON file:
{ "routes": { "/api/tasks/completed": "/tasks?completed=true" } }
This tells Json Server to map the route /api/tasks/completed
to the /tasks
endpoint with the query parameter completed=true
.
You can also define custom routes that perform more complex operations. For example, let's say you want to implement a custom route to retrieve tasks by a specific user. You can add the following route to your JSON file:
{ "routes": { "/api/users/:userId/tasks": "/tasks?userId=:userId" } }
This allows you to send a GET request to /api/users/1/tasks
to retrieve all tasks for user with ID 1.
By defining custom routes in your JSON file, you can extend the functionality of your Json Server API and handle more complex scenarios.
Advanced Technique: Middleware in Json Server
Json Server allows you to use middleware functions to modify the behavior of the server and add additional functionality. Middleware functions are executed before the routing takes place and can be used to perform tasks such as authentication, logging, and request preprocessing.
To use middleware in Json Server, you can define a JavaScript file that exports a middleware function and use it in your server setup. Here's an example of how to use middleware in Json Server:
First, create a JavaScript file named middleware.js
with the following content:
module.exports = (req, res, next) => { // Perform some preprocessing console.log('Request received:', req.method, req.url); // Call the next middleware or route handler next(); };
Next, create another JavaScript file named server.js
with the following content:
const jsonServer = require('json-server'); const middleware = require('./middleware'); const server = jsonServer.create(); const router = jsonServer.router('db.json'); const middlewares = jsonServer.defaults(); server.use(middleware); server.use(middlewares); server.use(router); const port = 3000; server.listen(port, () => { console.log(`Json Server is running on port ${port}`); });
This sets up a Json Server instance with the custom middleware function. The middleware function is executed for every incoming request and performs some preprocessing before calling the next middleware or route handler.
By using middleware in Json Server, you can add additional functionality and customize the behavior of the server to suit your needs.
Related Article: How to Use Embedded JavaScript (EJS) in Node.js
Code Snippet: Pagination and Sorting with Json Server
To implement pagination and sorting with Json Server, you can use the following code snippets as examples:
- Retrieve the first 10 tasks:
fetch('http://localhost:3000/tasks?_start=0&_limit=10') .then(response => response.json()) .then(tasks => console.log(tasks));
- Retrieve the next 10 tasks:
fetch('http://localhost:3000/tasks?_start=10&_limit=10') .then(response => response.json()) .then(tasks => console.log(tasks));
- Sort tasks by title in ascending order:
fetch('http://localhost:3000/tasks?_sort=title&_order=asc') .then(response => response.json()) .then(tasks => console.log(tasks));
- Sort tasks by due date in descending order:
fetch('http://localhost:3000/tasks?_sort=dueDate&_order=desc') .then(response => response.json()) .then(tasks => console.log(tasks));
These code snippets demonstrate how to implement pagination and sorting with Json Server. You can adapt them to your specific use case and modify the query parameters as needed.
Code Snippet: Filtering and Searching with Json Server
To implement filtering and searching with Json Server, you can use the following code snippets as examples:
- Filter tasks by completed status:
fetch('http://localhost:3000/tasks?completed=true') .then(response => response.json()) .then(tasks => console.log(tasks));
- Filter tasks by due date greater than a specific date:
fetch('http://localhost:3000/tasks?dueDate_gte=2022-01-01') .then(response => response.json()) .then(tasks => console.log(tasks));
- Search tasks by title:
fetch('http://localhost:3000/tasks?q=keyword') .then(response => response.json()) .then(tasks => console.log(tasks));
These code snippets demonstrate how to implement filtering and searching with Json Server. You can adapt them to your specific use case and modify the query parameters as needed.
Code Snippet: Relationships and Embedding with Json Server
Json Server allows you to define relationships between different collections and embed related data in the responses. This can be useful when you need to retrieve related data in a single request instead of making multiple requests.
To define relationships between collections in Json Server, you can use the _embed
and _expand
query parameters. The _embed
parameter allows you to embed related data in the response, while the _expand
parameter allows you to retrieve the full related objects instead of just their IDs.
Here's an example of how to define relationships and use the _embed
and _expand
query parameters:
Assume you have two collections, users
and tasks
, where each task belongs to a user. You can define the relationship between the collections in your JSON file like this:
{ "users": [ { "id": 1, "name": "John Doe" }, { "id": 2, "name": "Jane Smith" } ], "tasks": [ { "id": 1, "title": "Task 1", "userId": 1 }, { "id": 2, "title": "Task 2", "userId": 2 } ] }
To retrieve all tasks with their associated users embedded in the response, you can send a GET request to http://localhost:3000/tasks?_embed=users
:
fetch('http://localhost:3000/tasks?_embed=users') .then(response => response.json()) .then(tasks => console.log(tasks));
This will return all tasks with their associated users embedded in the response.
To retrieve a specific task with its associated user expanded in the response, you can send a GET request to http://localhost:3000/tasks/1?_expand=user
:
fetch('http://localhost:3000/tasks/1?_expand=user') .then(response => response.json()) .then(task => console.log(task));
This will return the task with ID 1 and its associated user expanded in the response.
By defining relationships and using the _embed
and _expand
query parameters, you can easily retrieve related data in a single request and avoid making multiple requests.
Code Snippet: Customizing Id Property with Json Server
By default, Json Server uses the id
property in your JSON data as the identifier for each resource. However, you can customize the property used as the identifier by using the --id
option when starting the server.
For example, to use the uuid
property as the identifier instead of id
, you can start the Json Server with the following command:
json-server --watch db.json --id uuid
This will make Json Server use the uuid
property as the identifier for each resource.
When creating new resources, you need to provide the custom identifier property in the request body. For example, to create a new resource with a custom identifier, you can send a POST request with the custom identifier property in the request body:
curl -X POST -H "Content-Type: application/json" -d '{"uuid":"123456","name":"John Doe"}' http://localhost:3000/users