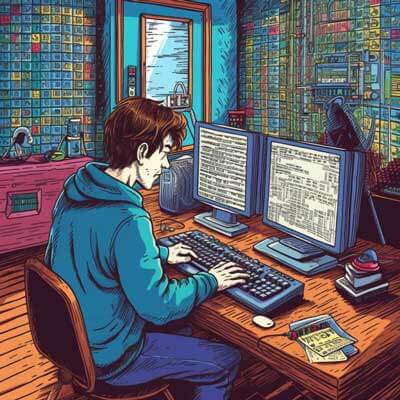
- Introduction to EJS
- Setting Up the Development Environment
- Creating Your First EJS Template
- Interpolating Data with EJS
- Implementing Conditional Statements in EJS
- Utilizing Loops in EJS
- Best Practices when Working with EJS
- Common Use Cases for EJS
- Code Snippet: Displaying a List of Items with EJS
- Code Snippet: Form Submission with EJS
- Code Snippet: User Authentication with EJS
- Code Snippet: Creating a Shopping Cart with EJS
- Code Snippet: Building a Weather App with EJS
- Error Handling in EJS
- Performance Considerations for EJS
- Advanced Techniques: Partial Views in EJS
- Advanced Techniques: Layouts in EJS
- Advanced Techniques: Custom Filters in EJS
- Real World Example: Building a Blog Site with EJS
- Real World Example: Developing an E-commerce Site with EJS
Introduction to EJS
EJS (Embedded JavaScript) is a popular templating engine for Node.js applications. It allows you to create dynamic HTML pages by embedding JavaScript code directly into your HTML templates. With EJS, you can easily generate dynamic content, render data from your server, and reuse template components.
Related Article: How To Update Node.Js
Setting Up the Development Environment
Before you can start using EJS in your Node.js application, you need to set up your development environment. Here are the steps to get started:
1. Create a new directory for your project and navigate to it in your terminal.
2. Initialize a new Node.js project by running the command: npm init -y
.
3. Install EJS as a dependency by running the command: npm install ejs
.
4. Create a new file called app.js
and require the EJS module at the top of the file: const ejs = require('ejs');
.
Creating Your First EJS Template
To create your first EJS template, follow these steps:
1. Create a new file called index.ejs
in the root directory of your project.
2. Open the index.ejs
file and add the following code:
<!DOCTYPE html> <html> <head> <title>My First EJS Template</title> </head> <body> <h1>Welcome to my EJS template!</h1> <p>Today's date is <%= new Date().toLocaleDateString() %>.</p> </body> </html>
3. In your app.js
file, add the following code to render the index.ejs
template:
ejs.renderFile('index.ejs', {}, (err, html) => { if (err) { console.error(err); } else { console.log(html); } });
4. Run your Node.js application by executing the command: node app.js
.
5. Open your web browser and navigate to http://localhost:3000
to see the rendered EJS template.
Interpolating Data with EJS
Interpolating data into your EJS templates is a common practice. It allows you to dynamically display data from your server in your HTML pages. Here’s an example of how to interpolate data with EJS:
1. Modify your index.ejs
file to include a variable to be interpolated:
<!DOCTYPE html> <html> <head> <title>My First EJS Template</title> </head> <body> <h1>Welcome to my EJS template, <%= name %>!</h1> </body> </html>
2. In your app.js
file, update the render function to pass the name
variable:
ejs.renderFile('index.ejs', { name: 'John' }, (err, html) => { if (err) { console.error(err); } else { console.log(html); } });
3. Restart your Node.js application and refresh your browser. You should now see the greeting personalized with the name “John”.
Related Article: How to Uninstall npm Modules in Node.js
Implementing Conditional Statements in EJS
EJS allows you to implement conditional statements directly in your templates. This enables you to conditionally render HTML elements based on certain conditions. Here’s an example:
1. Modify your index.ejs
file to include a conditional statement:
<!DOCTYPE html> <html> <head> <title>My First EJS Template</title> </head> <body> <% if (isLoggedIn) { %> <h1>Welcome back, <%= username %>!</h1> <% } else { %> <h1>Welcome, guest!</h1> <% } %> </body> </html>
2. In your app.js
file, update the render function to pass the isLoggedIn
and username
variables:
ejs.renderFile('index.ejs', { isLoggedIn: true, username: 'John' }, (err, html) => { if (err) { console.error(err); } else { console.log(html); } });
3. Restart your Node.js application and refresh your browser. You should see a personalized greeting based on the value of the isLoggedIn
variable.
Utilizing Loops in EJS
Loops are another powerful feature of EJS that allow you to iterate over arrays or objects and generate repetitive HTML content. Here’s an example of how to use loops in EJS:
1. Modify your index.ejs
file to include a loop:
<!DOCTYPE html> <html> <head> <title>My First EJS Template</title> </head> <body> <ul> <% for (let i = 0; i < fruits.length; i++) { %> <li><%= fruits[i] %></li> <% } %> </ul> </body> </html>
2. In your app.js
file, update the render function to pass the fruits
array:
ejs.renderFile('index.ejs', { fruits: ['Apple', 'Banana', 'Orange'] }, (err, html) => { if (err) { console.error(err); } else { console.log(html); } });
3. Restart your Node.js application and refresh your browser. You should see a list of fruits rendered based on the contents of the fruits
array.
Best Practices when Working with EJS
When working with EJS, it’s important to follow best practices to ensure clean and maintainable code. Here are some best practices to keep in mind:
1. Separate your EJS templates into separate files for better organization and reusability.
2. Use partials and layouts to modularize your templates and avoid code duplication.
3. Keep your templates as simple as possible by moving complex logic to your server-side code.
4. Sanitize user input to prevent XSS (Cross-Site Scripting) attacks.
5. Use descriptive variable names to improve code readability.
6. Comment your code to make it easier for other developers (and yourself) to understand.
Related Article: How to Use Force and Legacy Peer Deps in Npm
Common Use Cases for EJS
EJS can be used in a variety of scenarios in your Node.js applications. Here are some common use cases:
1. Rendering dynamic web pages with data retrieved from a database.
2. Generating HTML email templates with personalized content.
3. Creating PDF documents with dynamic content.
4. Building server-side rendered applications with frameworks like Express.js.
5. Implementing view components and reusable UI elements in your application.
Code Snippet: Displaying a List of Items with EJS
To display a list of items using EJS, you can utilize the power of loops. Here’s an example:
<ul> <% items.forEach((item) => { %> <li><%= item.name %></li> <% }) %> </ul>
This code snippet demonstrates how to iterate over an array of items and dynamically generate an HTML list.
Code Snippet: Form Submission with EJS
EJS can handle form submissions and process user input. Here’s an example of how to handle a form submission using EJS:
<form action="/submit" method="POST"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <button type="submit">Submit</button> </form>
In your server-side code, you can retrieve the submitted form data and process it accordingly.
Related Article: How to Fix "nvm command not found" with Node Version Manager
Code Snippet: User Authentication with EJS
User authentication is a common requirement in web applications. With EJS, you can implement user authentication flows easily. Here’s an example:
<% if (isLoggedIn) { %> <p>Welcome, <%= user.username %>!</p> <a href="/logout">Logout</a> <% } else { %> <a href="/login">Login</a> <a href="/register">Register</a> <% } %>
This code snippet demonstrates how to conditionally render different content based on whether a user is logged in or not.
Code Snippet: Creating a Shopping Cart with EJS
EJS is well-suited for building e-commerce applications and handling shopping cart functionality. Here’s an example of how to create a shopping cart using EJS:
<% if (cart.length > 0) { %> <ul> <% cart.forEach((item) => { %> <li><%= item.name %> - $<%= item.price %></li> <% }) %> </ul> <% } else { %> <p>Your cart is empty.</p> <% } %>
This code snippet demonstrates how to conditionally render a shopping cart based on its contents.
Code Snippet: Building a Weather App with EJS
EJS can be used to display real-time data in your application, such as weather information. Here’s an example of how to build a weather app with EJS:
<h1>Current Weather: <%= weather.temperature %>°C</h1> <p>Conditions: <%= weather.conditions %></p>
In your server-side code, you can retrieve weather data from an API and pass it to the EJS template for rendering.
Related Article: How to Set the Default Node Version Using Nvm
Error Handling in EJS
Error handling is an important aspect of any application. When working with EJS, you may encounter errors related to template rendering or data processing. Here are some best practices for error handling in EJS:
1. Wrap your EJS rendering code in a try-catch block to catch any errors that occur during rendering.
2. Use the err
parameter in the callback function to handle template rendering errors.
3. Provide informative error messages to help identify and debug issues.
4. Implement error logging to record errors for later analysis.
Performance Considerations for EJS
While EJS is a powerful templating engine, it’s important to consider performance when using it in your applications. Here are some tips to optimize EJS performance:
1. Minimize the use of complex logic in your templates.
2. Avoid unnecessary template re-rendering by caching rendered templates.
3. Optimize your server-side code to reduce data processing time.
4. Use partials and layouts to modularize your templates and improve rendering speed.
5. Enable template compression for faster delivery of HTML content to clients.
Advanced Techniques: Partial Views in EJS
Partial views are reusable components that can be included in multiple templates. They allow you to modularize your code and avoid code duplication. Here’s an example of how to use partial views in EJS:
1. Create a new file called header.ejs
and add the following code:
<header> <h1>My Website</h1> <nav> <a href="/">Home</a> <a href="/about">About</a> <a href="/contact">Contact</a> </nav> </header>
2. In your main template file, include the partial view using the include
directive:
<!DOCTYPE html> <html> <head> <title>My Website</title> </head> <body> <% include header.ejs %> <h1>Welcome to my website!</h1> <p>Content goes here...</p> </body> </html>
This code snippet demonstrates how to include a partial view in your main template using the include
directive.
Related Article: How to Fix the "getaddrinfo ENOTFOUND" Error in Node.js
Advanced Techniques: Layouts in EJS
Layouts provide a way to define a common structure for multiple pages in your application. They allow you to define a base layout with shared HTML elements and inject dynamic content into specific sections. Here’s an example of how to use layouts in EJS:
1. Create a new file called layout.ejs
and add the following code:
<!DOCTYPE html> <html> <head> <title><%= title %></title> </head> <body> <header> <h1>My Website</h1> <nav> <a href="/">Home</a> <a href="/about">About</a> <a href="/contact">Contact</a> </nav> </header> <main> <%= content %> </main> <footer> © 2022 My Website </footer> </body> </html>
2. In your individual page templates, specify the layout using the layout
directive:
<% layout('layout.ejs') %> <% title = 'About' %> <h1>About</h1> <p>This is the about page content...</p>
This code snippet demonstrates how to define a layout template and use it in individual page templates.
Advanced Techniques: Custom Filters in EJS
Custom filters allow you to extend the functionality of EJS by creating your own reusable filters. Filters can be used to transform data before rendering it in your templates. Here’s an example of how to create a custom filter in EJS:
1. Define your custom filter function in your server-side code:
const ejs = require('ejs'); ejs.filters.uppercase = function (input) { return input.toUpperCase(); };
2. In your EJS template, use the custom filter on a variable:
<p><%= message | uppercase %></p>
This code snippet demonstrates how to create a custom filter to convert a string to uppercase in an EJS template.
Real World Example: Building a Blog Site with EJS
To illustrate the use of EJS in a real-world scenario, let’s consider building a blog site. Here are some key features you can implement using EJS:
1. Displaying a list of blog posts with their titles, dates, and excerpts.
2. Rendering individual blog post pages with full content and comments.
3. Implementing a search functionality to find blog posts based on keywords.
4. Allowing users to leave comments on blog posts.
5. Creating an admin dashboard to manage blog posts, comments, and user accounts.
By combining EJS with a Node.js web framework like Express.js, you can build a fully functional blog site with dynamic content and a great user experience.
Related Article: How to Fix "Npm Err! Code Elifecycle" in Node.js
Real World Example: Developing an E-commerce Site with EJS
E-commerce sites often require dynamic content rendering and complex data processing. EJS can be a great choice for building e-commerce applications. Here are some key features you can implement using EJS:
1. Displaying product listings with images, prices, and descriptions.
2. Implementing a shopping cart functionality for users to add and remove items.
3. Integrating with payment gateways to process online transactions.
4. Allowing users to write reviews and rate products.
5. Building an admin panel to manage products, orders, and customer data.
With EJS and a robust backend framework like Express.js, you can develop a feature-rich e-commerce site that provides a seamless shopping experience for your customers.