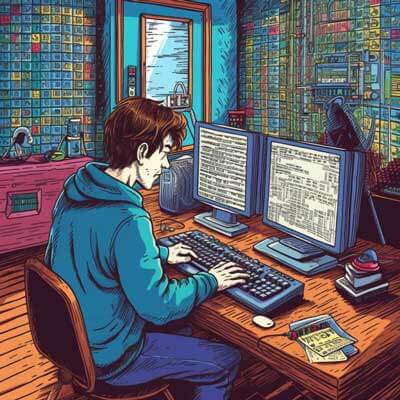
Introduction to Sorting in C++
Sorting is an essential operation in programming, allowing us to organize data in a specific order. In C++, there are various sorting techniques available that can be used to sort different types of data, such as numbers, strings, and objects. Understanding these sorting techniques and their implementation in C++ is crucial for efficient and effective programming.
Related Article: How to Implement a Beating Heart Loader in Pure CSS
Sorting Basics: Definition and Importance
Sorting refers to the process of arranging elements in a particular order, such as ascending or descending. It is a fundamental operation in computer science and has wide applications in various domains, including data analysis, database management, and algorithmic problem-solving.
In C++, sorting is important for several reasons. Firstly, sorting allows us to search for elements efficiently using techniques like binary search. Secondly, it helps in organizing data for better readability and presentation. Lastly, sorting is often a prerequisite for other operations, such as merging or removing duplicates from a dataset.
Sort Function in C++: An Overview
C++ provides a built-in sort function, which simplifies the process of sorting arrays, vectors, and other container types. The sort function is part of the C++ Standard Library and is based on the efficient and versatile Quicksort algorithm.
The syntax for using the sort function is as follows:
#include <algorithm> // Sorting a container using the sort function std::sort(container.begin(), container.end());
Here, container
refers to the data structure to be sorted, such as an array or vector. The begin()
and end()
methods are used to specify the range of elements to be sorted.
Let’s consider some examples to illustrate the usage of the sort function.
Example 1: Sorting a Vector of Integers
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {5, 2, 8, 1, 9}; std::sort(numbers.begin(), numbers.end()); for (int num : numbers) { std::cout << num << " "; } return 0; }
Output:
1 2 5 8 9
Example 2: Sorting an Array of Strings
#include <iostream> #include <algorithm> int main() { std::string names[] = {"Alice", "Charlie", "Bob", "David"}; std::sort(names, names + 4); for (const std::string& name : names) { std::cout << name << " "; } return 0; }
Output:
Alice Bob Charlie David
Different Types of Sorting Techniques in C++
In C++, there are several sorting techniques available, each with its own advantages and disadvantages. Some commonly used sorting techniques include:
– Bubble Sort
– Insertion Sort
– Quick Sort
– Merge Sort
– Heap Sort
– Radix Sort
We will explore each of these techniques in detail, along with code snippets demonstrating their implementation.
Related Article: 7 Shared Traits of Ineffective Engineering Teams
Bubble Sort: Theory and Code Snippets
Bubble sort is a simple sorting algorithm that repeatedly compares adjacent elements and swaps them if they are in the wrong order. The algorithm continues to pass through the list until the entire list is sorted.
The basic idea behind bubble sort can be summarized as follows:
1. Compare adjacent elements in the list.
2. If the two elements are out of order, swap them.
3. Repeat steps 1 and 2 until the list is sorted.
Let’s see an example of bubble sort implemented in C++.
Example 1: Bubble Sort on an Array of Integers
#include <iostream> void bubbleSort(int arr[], int size) { for (int i = 0; i < size - 1; i++) { for (int j = 0; j < size - i - 1; j++) { if (arr[j] > arr[j + 1]) { std::swap(arr[j], arr[j + 1]); } } } } int main() { int numbers[] = {5, 2, 8, 1, 9}; int size = sizeof(numbers) / sizeof(numbers[0]); bubbleSort(numbers, size); for (int i = 0; i < size; i++) { std::cout << numbers[i] << " "; } return 0; }
Output:
1 2 5 8 9
Example 2: Bubble Sort on a Vector of Strings
#include <iostream> #include <vector> void bubbleSort(std::vector<std::string>& vec) { int size = vec.size(); for (int i = 0; i < size - 1; i++) { for (int j = 0; j < size - i - 1; j++) { if (vec[j] > vec[j + 1]) { std::swap(vec[j], vec[j + 1]); } } } } int main() { std::vector<std::string> names = {"Alice", "Charlie", "Bob", "David"}; bubbleSort(names); for (const std::string& name : names) { std::cout << name << " "; } return 0; }
Output:
Alice Bob Charlie David
Insertion Sort: Theory and Code Snippets
Insertion sort is another simple sorting algorithm that builds the final sorted array one item at a time. It is based on the idea of dividing the array into a sorted and an unsorted part. Initially, the sorted part contains only the first element, and the unsorted part contains the remaining elements. The algorithm picks an element from the unsorted part and inserts it into the correct position in the sorted part.
The basic idea behind insertion sort can be summarized as follows:
1. Assume the first element in the array is sorted.
2. Take the next element from the unsorted part and insert it into the correct position in the sorted part.
3. Repeat step 2 until all elements in the unsorted part are processed.
Let’s see an example of insertion sort implemented in C++.
Example 1: Insertion Sort on an Array of Integers
#include <iostream> void insertionSort(int arr[], int size) { for (int i = 1; i < size; i++) { int key = arr[i]; int j = i - 1; while (j >= 0 && arr[j] > key) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = key; } } int main() { int numbers[] = {5, 2, 8, 1, 9}; int size = sizeof(numbers) / sizeof(numbers[0]); insertionSort(numbers, size); for (int i = 0; i < size; i++) { std::cout << numbers[i] << " "; } return 0; }
Output:
1 2 5 8 9
Example 2: Insertion Sort on a Vector of Strings
#include <iostream> #include <vector> void insertionSort(std::vector<std::string>& vec) { int size = vec.size(); for (int i = 1; i < size; i++) { std::string key = vec[i]; int j = i - 1; while (j >= 0 && vec[j] > key) { vec[j + 1] = vec[j]; j--; } vec[j + 1] = key; } } int main() { std::vector<std::string> names = {"Alice", "Charlie", "Bob", "David"}; insertionSort(names); for (const std::string& name : names) { std::cout << name << " "; } return 0; }
Output:
Alice Bob Charlie David