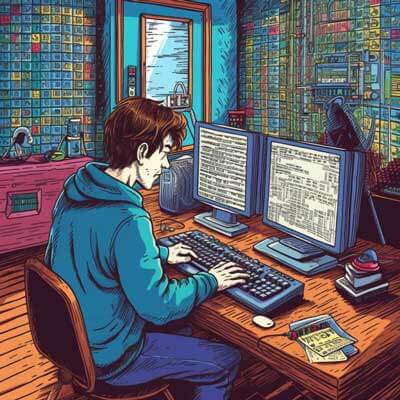
To validate IPv4 addresses using regular expressions (regex), you can use the following approaches:
Approach 1: Using a Simple Regex Pattern
One way to validate an IPv4 address is by using a simple regex pattern. Here’s an example of how you can do it in Python:
import re def is_valid_ipv4(address): pattern = r'^((([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5])\.){3}([0-9]|[1-9][0-9]|1[0-9]{2}|2[0-4][0-9]|25[0-5]))$' return re.match(pattern, address) is not None
In this example, we define a function called is_valid_ipv4
that takes an IPv4 address as input and uses the re.match
function from the re
module to check if the address matches the regex pattern. The regex pattern consists of four groups of numbers separated by dots, with each number ranging from 0 to 255.
You can use this function to validate IPv4 addresses in your code like this:
address = "192.168.0.1" if is_valid_ipv4(address): print("Valid IPv4 address") else: print("Invalid IPv4 address")
Related Article: How to Implement HTML Select Multiple As a Dropdown
Approach 2: Using the ipaddress Module
Another approach to validate IPv4 addresses is by using the ipaddress
module, which is available in Python 3. The ipaddress
module provides a convenient way to validate and manipulate IP addresses.
Here’s an example of how you can use the ipaddress
module to validate an IPv4 address in Python:
import ipaddress def is_valid_ipv4(address): try: ipaddress.IPv4Address(address) return True except ipaddress.AddressValueError: return False
In this example, we define a function called is_valid_ipv4
that takes an IPv4 address as input. We use the IPv4Address
class from the ipaddress
module to validate the address. If the address is valid, the IPv4Address
constructor will not raise an exception, and we return True
. If the address is invalid, an exception of type AddressValueError
will be raised, and we return False
.
You can use this function to validate IPv4 addresses in your code like this:
address = "192.168.0.1" if is_valid_ipv4(address): print("Valid IPv4 address") else: print("Invalid IPv4 address")
Best Practices
When validating IPv4 addresses using regex, keep the following best practices in mind:
– Use a well-tested regex pattern: The regex pattern used for validating IPv4 addresses should be well-tested and cover all possible valid cases. The pattern provided in Approach 1 is a common pattern used for this purpose.
– Consider using a dedicated library: If you are working with a programming language that provides a dedicated library for handling IP addresses, such as the ipaddress
module in Python, it is generally recommended to use that library instead of relying solely on regex. These libraries often provide more comprehensive validation and additional features for working with IP addresses.
– Be aware of address formats: IPv4 addresses can be represented in different formats, such as dotted decimal notation (e.g., “192.168.0.1”) and integer notation (e.g., 3232235521). Make sure your validation logic accounts for these different formats if necessary.
– Test thoroughly: When implementing IPv4 address validation, make sure to test your code with a variety of valid and invalid addresses to ensure its correctness.
Related Article: How to Use If-Else Statements in Shell Scripts