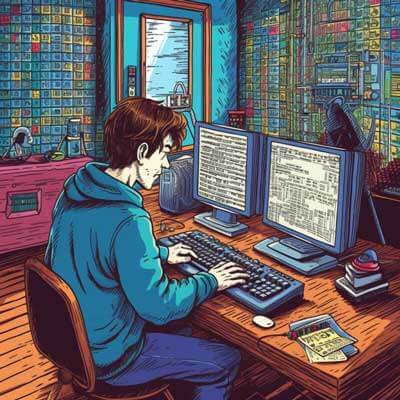
Table of Contents
Understanding Ethical Hacking
Ethical hacking, also known as penetration testing or white hat hacking, is the practice of intentionally and lawfully exploiting vulnerabilities in computer systems, networks, or software applications to identify and address security weaknesses. Unlike malicious hackers, ethical hackers have the permission and responsibility to uncover vulnerabilities and help organizations improve their overall security posture.
Related Article: OAuth 2 Tutorial: Introduction & Basics
Why Ethical Hacking is Important
In today's digital age, where cyber threats are becoming increasingly sophisticated and prevalent, organizations must proactively identify and address vulnerabilities in their software. Failure to do so can result in severe financial and reputational damage. Ethical hacking plays a crucial role in this process by enabling developers to understand and mitigate potential security risks before they can be exploited by malicious actors.
By conducting controlled attacks on their own systems or applications, developers can gain valuable insights into the vulnerabilities that exist and the potential impact they may have. This knowledge allows them to implement robust security measures and best practices, ensuring that their software is more resilient to attacks.
The Ethical Hacking Process
Ethical hacking typically follows a systematic process that involves several stages:
1. Planning and Reconnaissance: This stage involves gathering information about the target system or application. It includes activities such as understanding the system's architecture, identifying potential vulnerabilities, and developing a comprehensive testing plan.
2. Scanning: In this stage, ethical hackers use various tools and techniques to scan the target system for open ports, network services, and potential vulnerabilities. This helps them identify potential entry points for attacks.
3. Enumeration: During enumeration, hackers attempt to gather more detailed information about the target system, such as user accounts, network shares, and system configurations. This information can be valuable in planning and executing targeted attacks.
4. Vulnerability Analysis: At this stage, ethical hackers analyze the collected information to identify potential vulnerabilities. They may use automated vulnerability scanners or manually inspect the system's code and configuration to uncover weaknesses.
5. Exploitation: In this stage, ethical hackers attempt to exploit the identified vulnerabilities to gain unauthorized access or perform specific actions within the system. This helps them validate the severity and impact of the vulnerabilities.
6. Post-Exploitation: After successfully exploiting a vulnerability, ethical hackers document their findings and assess the extent of the impact. This information is crucial for organizations to understand the potential risks and prioritize remediation efforts.
7. Reporting: The final stage involves creating a detailed report that outlines the vulnerabilities discovered, the steps taken to exploit them, and recommendations for improving security. This report is shared with the organization's stakeholders, including developers, system administrators, and management.
Tools and Techniques
Ethical hackers employ a wide range of tools and techniques to identify and exploit vulnerabilities. Some commonly used tools include:
- Metasploit: A popular framework for developing, testing, and executing exploits.
- Nmap: A powerful network scanning tool used for discovering open ports, services, and potential vulnerabilities.
- Wireshark: A network protocol analyzer that captures and analyzes network traffic, helping in identifying potential security issues.
- OWASP WebGoat: An intentionally vulnerable web application used for practicing and learning about web application security.
Related Article: 24 influential books programmers should read
The Role of Developers
Developers play a critical role in the ethical hacking process. By understanding common security vulnerabilities and incorporating secure coding practices, they can prevent many potential attacks before they occur. Some essential considerations for developers include:
- Input validation: Ensuring that all user inputs are properly validated and sanitized to prevent common vulnerabilities like SQL injection or cross-site scripting (XSS).
- Secure authentication and authorization: Implementing robust authentication mechanisms and access controls to protect sensitive data and prevent unauthorized access.
- Secure configuration management: Properly configuring servers, databases, and applications to minimize the attack surface and reduce the risk of exploitation.
- Regular patching and updates: Keeping software dependencies and frameworks up-to-date to address known vulnerabilities and security patches.
By actively participating in the ethical hacking process and adopting secure coding practices, developers can contribute to building more secure software and protecting organizations from potential cyber threats.
Ethical hacking is a dynamic and evolving field, driven by the constant advancements in technology and the ever-changing threat landscape. By embracing ethical hacking principles, developers can stay ahead of the curve and create software that is more resilient to attacks.
Common Vulnerabilities in Software
In today's digital age, where software is an integral part of our lives, the security of that software is of utmost importance. As a developer, it is essential to understand the common vulnerabilities that can be exploited by attackers to gain unauthorized access, compromise data, or disrupt the functioning of a system. This chapter will explore some of the most prevalent vulnerabilities in software and provide guidance on how to prevent them.
1. Injection Attacks
Injection attacks occur when an attacker is able to insert malicious code or commands into an application's input, which is then executed by the application's interpreter or database. This can lead to severe consequences, such as unauthorized access, data breaches, or even complete system compromise.
One common example of an injection attack is SQL injection. In this type of attack, an attacker can manipulate input fields to inject malicious SQL code that can bypass security measures and gain unauthorized access to databases.
To prevent injection attacks, it is crucial to sanitize and validate all user input. Parameterized queries and prepared statements can also be used to mitigate the risk of SQL injection attacks.
Example of a vulnerable PHP code snippet susceptible to SQL injection:
$userId = $_GET['id']; $sql = "SELECT * FROM users WHERE id = " . $userId; $result = mysqli_query($conn, $sql);
To prevent SQL injection, the code can be modified as follows:
$userId = mysqli_real_escape_string($conn, $_GET['id']); $sql = "SELECT * FROM users WHERE id = " . $userId; $result = mysqli_query($conn, $sql);
2. Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. This can lead to various attacks, such as session hijacking, stealing sensitive information, or redirecting users to malicious websites.
There are three types of XSS attacks: stored XSS, reflected XSS, and DOM-based XSS. Stored XSS occurs when the malicious script is permanently stored on the target server. Reflected XSS occurs when the malicious script is embedded in a URL and is reflected back to the user. DOM-based XSS occurs when the malicious script manipulates the Document Object Model (DOM) of a web page.
To prevent XSS attacks, it is essential to properly validate and sanitize all user input, especially input that is displayed on web pages. Output encoding can also be used to ensure that user input is treated as data and not executable code.
Example of a vulnerable JavaScript code snippet susceptible to reflected XSS:
var name = window.location.hash.substring(1); document.write("Welcome, " + name + "!");
To prevent XSS, the code can be modified as follows:
var name = window.location.hash.substring(1); var sanitizedName = sanitizeHTML(name); document.getElementById("welcome-message").textContent = "Welcome, " + sanitizedName + "!";
Related Article: How to Do Sorting in C++ & Sorting Techniques
3. Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is a vulnerability that allows an attacker to trick authenticated users into performing unwanted actions on a website without their knowledge or consent. This can lead to actions such as changing passwords, making unauthorized transactions, or deleting user accounts.
To prevent CSRF attacks, it is crucial to implement measures such as using anti-CSRF tokens, checking the referer header, and enforcing strict access control.
Example of a vulnerable HTML code snippet susceptible to CSRF:
<form action="https://example.com/update_email" method="POST"> <input type="hidden" name="email" value="attacker@example.com"> <button type="submit">Update Email</button> </form>
To prevent CSRF, the code can be modified as follows:
<form action="https://example.com/update_email" method="POST"> <input type="hidden" name="email" value="attacker@example.com"> <input type="hidden" name="csrf_token" value="randomly_generated_token"> <button type="submit">Update Email</button> </form>
These are just a few examples of common vulnerabilities in software. As a developer, it is essential to be aware of these vulnerabilities and take appropriate measures to secure your software. Regular security assessments, code reviews, and staying up-to-date with the latest security practices are crucial in ensuring the integrity and security of your applications.
Secure Coding Best Practices
In today's digital age, software security is of utmost importance. As a developer, it is crucial to follow secure coding best practices to ensure that your software is protected against potential threats and vulnerabilities. By incorporating these practices into your development process, you can significantly reduce the risk of security breaches and data leaks.
1. Input Validation
One of the most common ways hackers exploit software is through input validation vulnerabilities. It is essential to validate and sanitize all user input to prevent attacks such as SQL injection, cross-site scripting (XSS), and command injection. Here's an example of input validation in Java:
public boolean isValidUsername(String username) { // Validate username against allowed characters and length // Return true if valid, false otherwise }
2. Password Hashing
Storing passwords securely is vital to protect user accounts. Instead of storing plain-text passwords, developers should use strong hashing algorithms to store password hashes. This way, even if the database is compromised, the passwords remain secure. Here's an example of password hashing in Python:
import hashlib password = "my_password" # Generate salt salt = os.urandom(16) # Hash password with salt hash_password = hashlib.pbkdf2_hmac('sha256', password.encode('utf-8'), salt, 100000) # Store hash and salt in the database
Related Article: 16 Amazing Python Libraries You Can Use Now
3. Secure Communication
When transmitting sensitive data over a network, it is essential to use secure communication protocols such as HTTPS. This ensures that the data is encrypted and cannot be intercepted by attackers. Here's an example of using HTTPS in Node.js:
const https = require('https'); const options = { hostname: 'example.com', port: 443, path: '/api', method: 'GET', }; const req = https.request(options, (res) => { // Handle response }); req.on('error', (error) => { // Handle error }); req.end();
4. Error Handling
Proper error handling is crucial for secure coding. Avoid displaying detailed error messages to users, as they can provide valuable information to attackers. Instead, log errors with sufficient details for debugging purposes and display generic error messages to users. Here's an example of error handling in PHP:
try { // Code that may throw an exception } catch (Exception $e) { // Log the error error_log($e->getMessage()); // Display generic error message to the user echo "An error occurred. Please try again later."; }
5. Regularly Update and Patch
Software vulnerabilities are constantly being discovered, and it is crucial to keep your software up to date with the latest security patches. Regularly update all libraries, frameworks, and dependencies used in your software to ensure that you are benefiting from the latest security enhancements. Stay informed about security advisories and apply patches promptly.
By following these secure coding best practices, you can significantly improve the security of your software and protect your users' data. Remember, security is an ongoing process, and it is essential to stay updated with the latest security trends and continuously educate yourself on new vulnerabilities and countermeasures.
Implementing Input Validation
Input validation is a crucial step in securing software applications against various types of attacks, such as SQL injection and cross-site scripting (XSS). By validating and sanitizing user input before processing it, developers can prevent malicious data from compromising the application's security.
Related Article: Top 10 AI Assistants To Optimize Your Life in 2024
Why is Input Validation Important?
Attackers often exploit software vulnerabilities by injecting malicious input into the application. Without proper input validation, this can lead to a range of security issues, including data breaches, unauthorized access, and code execution.
Implementing input validation helps ensure that data entered by users or received from external sources is safe and conforms to the expected format. By defining validation rules and sanitizing input, developers can minimize the risk of security vulnerabilities and protect the integrity of their software.
Types of Input Validation
There are several types of input validation techniques that developers can employ, depending on the nature of the input and the desired security level. Here are some commonly used validation methods:
1. White-list Validation: This approach involves defining a list of acceptable characters, patterns, or formats for input data. Any input that does not match the predefined rules is rejected. For example, if a user is expected to enter a numeric value, the input validation process can check if the input consists only of digits.
const userInput = '123abc'; if (/^\d+$/.test(userInput)) { // Process the input } else { // Reject the input }
2. Black-list Validation: In this method, developers define a list of disallowed characters, patterns, or formats. Any input that contains the blacklisted elements is rejected. However, this approach is generally less secure than white-list validation, as it relies on developers being aware of all potential threats.
user_input = "DROP TABLE users;" if "DROP TABLE" not in user_input: # Process the input else: # Reject the input
3. Regular Expression Validation: Regular expressions are powerful tools for validating complex input patterns. By defining regex patterns, developers can ensure that the input adheres to specific rules. For example, a regular expression pattern can be used to validate email addresses.
String userInput = "example@example.com"; if (userInput.matches("[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}")) { // Process the input } else { // Reject the input }
Sanitizing Input
In addition to input validation, developers should also sanitize user input to remove any potentially harmful characters or tags. Sanitizing input helps prevent cross-site scripting (XSS) attacks, where attackers inject malicious code into web pages viewed by other users.
One common approach to sanitizing input is using an HTML sanitizer library, such as OWASP Java Encoder or PHP's htmlspecialchars function. These tools can escape or remove potentially dangerous HTML tags, preventing them from being interpreted by the browser.
Here's an example of using PHP's htmlspecialchars function to sanitize user input:
$userInput = "<script>alert('XSS attack!');</script>"; $sanitizedInput = htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8'); echo $sanitizedInput; // Output: <script>alert('XSS attack!');</script>
Protecting Against Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common vulnerability that allows attackers to inject malicious scripts into webpages viewed by other users. These scripts can steal sensitive information, manipulate website content, or even redirect users to malicious websites. As a developer, it is crucial to understand and implement proper security measures to protect against XSS attacks.
1. Input Validation and Output Encoding
The first line of defense against XSS attacks is to properly validate and sanitize all user input. This includes data submitted through forms, query parameters, cookies, and any other user-controlled input. By validating input, you can ensure that it meets the expected format and type, reducing the risk of XSS vulnerabilities.
Additionally, it is essential to encode all output data before displaying it in webpages. Output encoding converts special characters to their HTML entities, preventing them from being interpreted as code by the browser. Most web frameworks provide built-in output encoding functions, such as htmlspecialchars
in PHP or escape
in JavaScript.
Here is an example of output encoding in PHP:
$name = $_GET['name']; echo htmlspecialchars($name, ENT_QUOTES, 'UTF-8');
2. Content Security Policy (CSP)
Content Security Policy (CSP) is an additional layer of defense against XSS attacks. It allows you to define the sources from which your web application can load resources, such as scripts, stylesheets, or images. By specifying trusted sources, you can prevent the execution of any malicious scripts injected via XSS.
To implement CSP, you need to set the Content-Security-Policy
HTTP header or use the <meta>
tag in your HTML. Here is an example of setting the header in PHP:
header("Content-Security-Policy: script-src 'self' https://trusted-cdn.com; default-src 'self'");
This example allows scripts to be loaded only from the current domain and the trusted CDN, while other resources must also originate from the current domain.
3. HTTP Only Cookies
XSS attacks often involve stealing users' session cookies to impersonate them. By setting the HttpOnly
flag on cookies, you can prevent client-side scripts from accessing them. This significantly reduces the risk of session hijacking through XSS.
In most web frameworks, you can set the HttpOnly
flag when creating cookies. Here is an example in JavaScript:
document.cookie = "session=123456789; HttpOnly";
It is important to note that the HttpOnly
flag only protects cookies from script access. It does not prevent interception of cookies through other means, such as network sniffing or cross-site request forgery (CSRF) attacks.
4. Regular Security Updates
Keeping your software up to date is crucial for protecting against XSS attacks. Regularly update your web server, programming language, framework, and any third-party libraries you use. Security vulnerabilities are often discovered and patched in these components, and failing to update can leave your application exposed to known exploits.
Stay informed about security advisories and subscribe to relevant mailing lists or RSS feeds to receive timely updates. Promptly apply security patches to your development and production environments to ensure the latest protection against XSS vulnerabilities.
By implementing these security measures, you can significantly reduce the risk of XSS attacks in your web applications. Remember that security is an ongoing process, and it is essential to stay vigilant and keep up with the latest best practices and techniques to protect your software from evolving threats.
Related Article: SOLID Principles: Object-Oriented Design Tutorial
Preventing SQL Injection Attacks
SQL Injection attacks are one of the most common and dangerous vulnerabilities in software applications. They occur when an attacker is able to manipulate the SQL queries executed by an application, leading to unauthorized access, data breaches, or even complete system compromise. Thankfully, there are several measures developers can take to prevent SQL Injection attacks and ensure the security of their software.
1. Prepared Statements (Parameterized Queries)
One of the most effective ways to prevent SQL Injection attacks is by using prepared statements, also known as parameterized queries. Prepared statements separate the SQL code from the data, making it impossible for an attacker to inject malicious SQL code into the query.
Here's an example in PHP:
<?php $stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username AND password = :password'); $stmt->bindParam(':username', $username); $stmt->bindParam(':password', $password); $stmt->execute(); ?>
In this example, the placeholder :username
and :password
are used in the query, and the actual values are bound using bindParam()
before executing the statement. This ensures that the user input is treated as data, and not as part of the SQL code.
2. Input Validation and Sanitization
Another important step in preventing SQL Injection attacks is validating and sanitizing user input. By enforcing strict input validation, developers can ensure that only expected and safe data is used in SQL queries.
For example, if a user is expected to enter an integer value, the application should validate that the input is indeed an integer and reject any other type of input.
Additionally, it's crucial to sanitize the input data by escaping special characters or using parameterized queries. Many programming languages and frameworks provide built-in functions or libraries for input sanitization, such as mysqli_real_escape_string()
in PHP or parameter binding in Java's PreparedStatement
.
3. Least Privilege Principle
The least privilege principle states that every user or process should have the minimum privileges required to perform their tasks. Applying this principle to your database access can help mitigate the impact of SQL Injection attacks.
When connecting to the database, ensure that the user account used by the application has only the necessary permissions to execute the required queries. Avoid using a superuser account or granting unnecessary privileges to the application's database user.
Related Article: 7 Shared Traits of Ineffective Engineering Teams
4. Regularly Update and Patch
Keeping your software up to date is crucial in preventing SQL Injection attacks. Database management systems, programming languages, and frameworks often release security patches and updates to address known vulnerabilities, including those related to SQL Injection.
Make sure to regularly check for updates and patches for your software stack, and promptly apply them to ensure you're using the latest secure versions.
5. Secure Coding Best Practices
In addition to the specific measures mentioned above, following secure coding best practices is essential for preventing SQL Injection attacks. Some general guidelines to keep in mind include:
- Avoiding the use of dynamic SQL queries whenever possible.
- Implementing strong authentication and authorization mechanisms.
- Regularly conducting security audits and code reviews.
- Using strong and unique passwords for database accounts.
- Encrypting sensitive data stored in the database.
- Limiting the exposure of error messages that may reveal sensitive information.
By following these best practices, developers can significantly reduce the risk of SQL Injection attacks and enhance the overall security of their software applications.
Remember, preventing SQL Injection is an ongoing process, and it requires a combination of secure coding practices, regular updates, and constant vigilance to stay ahead of potential attackers.
Securing Authentication and Authorization
Authentication and authorization are critical aspects of securing software applications. Authentication ensures that users are who they claim to be, while authorization determines what actions a user is allowed to perform within an application. In this chapter, we will explore best practices for securing authentication and authorization to protect against common security vulnerabilities.
1. Implement Strong Password Policies
One of the simplest yet most effective ways to enhance authentication security is by implementing strong password policies. Encourage users to create complex passwords that include a combination of uppercase and lowercase letters, numbers, and special characters. Additionally, enforce minimum password length requirements and regularly prompt users to update their passwords.
# Example of a strong password policy implementation in Python import re def validate_password(password): if len(password) < 8: return False if not re.search(r"[a-z]", password): return False if not re.search(r"[A-Z]", password): return False if not re.search(r"\d", password): return False if not re.search(r"[!@#$%^&*()\-_=+{};:,<.>]", password): return False return True
Related Article: How to Use JSON Parse and Stringify in JavaScript
2. Implement Multi-Factor Authentication (MFA)
Multi-factor authentication adds an extra layer of security by requiring users to provide multiple pieces of evidence to verify their identity. This typically involves combining something the user knows (e.g., a password) with something the user has (e.g., a security token or mobile device). By implementing MFA, even if an attacker manages to obtain a user's password, they would still need the additional factor to gain unauthorized access.
// Example of implementing MFA in Java using the Google Authenticator library import com.warrenstrange.googleauth.GoogleAuthenticator; import com.warrenstrange.googleauth.GoogleAuthenticatorKey; public class MultiFactorAuthenticator { public static void main(String[] args) { GoogleAuthenticator gAuth = new GoogleAuthenticator(); GoogleAuthenticatorKey key = gAuth.createCredentials(); // Display the key to the user for setup System.out.println("Please scan the following QR code to set up MFA:"); System.out.println(key.getQRCodeUrl("MyApp", "user@example.com")); // Verify the user's code boolean isCodeValid = gAuth.authorize(key.getKey(), 123456); System.out.println("Is code valid? " + isCodeValid); } }
3. Implement Role-Based Access Control (RBAC)
Role-Based Access Control is a popular authorization model that assigns permissions to users based on their roles within an organization. By implementing RBAC, developers can ensure that users only have access to the resources and actions that are necessary for their job responsibilities. This helps to prevent unauthorized access and restricts the potential damage that a compromised account can cause.
// Example of implementing RBAC in PHP using a simple user role check function userHasPermission($userRole, $requiredRole) { $roles = [ 'admin' => ['create', 'read', 'update', 'delete'], 'editor' => ['create', 'read', 'update'], 'reader' => ['read'] ]; if (!array_key_exists($userRole, $roles)) { return false; } return in_array($requiredRole, $roles[$userRole]); }
4. Protect Against Brute-Force Attacks
Brute-force attacks involve systematically guessing passwords until the correct one is found. To protect against such attacks, developers should implement account lockouts and rate limiting. Account lockouts temporarily lock a user's account after a certain number of failed login attempts, while rate limiting restricts the number of login attempts that can be made within a specific time frame.
// Example of implementing account lockout and rate limiting in JavaScript const MAX_LOGIN_ATTEMPTS = 3; const LOCKOUT_DURATION = 300000; // 5 minutes let loginAttempts = 0; let lastLoginAttemptTime = null; function login(username, password) { if (lastLoginAttemptTime && Date.now() - lastLoginAttemptTime > LOCKOUT_DURATION) { loginAttempts = 0; } if (loginAttempts >= MAX_LOGIN_ATTEMPTS) { return "Account locked. Please try again later."; } if (authenticate(username, password)) { // Successful login loginAttempts = 0; lastLoginAttemptTime = null; return "Login successful!"; } else { // Failed login attempt loginAttempts++; lastLoginAttemptTime = Date.now(); return "Invalid username or password."; } }
By implementing these best practices, developers can significantly enhance the security of authentication and authorization mechanisms in their software applications, reducing the risk of unauthorized access and data breaches. Remember, security is an ongoing process, and it is important to regularly review and update these mechanisms to stay ahead of emerging threats.
Mitigating Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is a type of attack that occurs when a malicious website tricks a user's browser into making an unintended request to a target website. This attack leverages the trust that a website has in a user's browser and can lead to unauthorized actions being performed on behalf of the user.
To mitigate CSRF attacks, developers need to implement proper security measures in their applications. Here are some recommended strategies:
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
1. Use Anti-CSRF Tokens
One of the most effective ways to prevent CSRF attacks is by using anti-CSRF tokens. These tokens are generated by the server and embedded in the web forms or APIs that require user interaction. When a form is submitted or an API request is made, the token is included and validated by the server.
Here's an example of how to generate and include an anti-CSRF token in a web form using PHP:
<?php session_start(); // Generate a random token $token = bin2hex(random_bytes(32)); // Store the token in the session $_SESSION['csrf_token'] = $token; ?> <form action="/process" method="post"> <input type="hidden" name="csrf_token" value="<?php echo $token; ?>"> <!-- Other form fields --> <button type="submit">Submit</button> </form>
On the server side, the token is validated before processing the request:
<?php session_start(); // Validate the CSRF token if ($_POST['csrf_token'] !== $_SESSION['csrf_token']) { // Invalid token, handle the error die('CSRF token validation failed'); } // Process the request // ... ?>
2. Implement SameSite Cookies
Another effective mitigation technique is to use SameSite cookies. SameSite is a cookie attribute that prevents the browser from sending cookies in cross-site requests, including CSRF attacks.
To set a SameSite cookie, you can use the Set-Cookie
HTTP header with the SameSite
attribute. Here's an example using Node.js and the Express framework:
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.cookie('session', '12345', { sameSite: 'strict', httpOnly: true, }); res.send('Hello World!'); }); app.listen(3000, () => { console.log('Server started on port 3000'); });
In this example, the session
cookie is set with the SameSite
attribute set to strict
, which ensures that the cookie is only sent with same-site requests.
3. Implement Reauthentication for Sensitive Actions
For sensitive actions such as changing passwords or making financial transactions, it's important to implement reauthentication. Reauthentication requires users to provide additional credentials, such as their password, before performing these actions.
By implementing reauthentication, even if a CSRF attack is successful, the attacker would still need the user's credentials to perform any sensitive action.
4. Use Time-limited Tokens
To further enhance CSRF protection, you can use time-limited tokens. These tokens have an expiration time and become invalid after a certain period. By doing so, even if an attacker manages to obtain a token, it would become useless after it expires.
When generating time-limited tokens, it's important to ensure that the expiration time is set appropriately and that tokens are invalidated after use.
Implementing these strategies can significantly reduce the risk of CSRF attacks in your applications. By combining anti-CSRF tokens, SameSite cookies, reauthentication, and time-limited tokens, developers can enhance the security of their software and protect users from potential CSRF vulnerabilities.
Related Article: Top 5 Innovative Sustainable Technology Trends in 2024
Handling Session Security
In web applications, sessions are used to maintain state and store user-specific information. However, if not properly secured, sessions can be vulnerable to attacks like session hijacking and session fixation. As a developer, it is crucial to implement robust session security measures to protect your users' sensitive information.
1. Use Secure Cookies
When a user logs into your application, a session is usually created and a session ID is stored in a cookie on the user's browser. By default, cookies are sent over both secure (HTTPS) and non-secure (HTTP) connections. To ensure session security, it is recommended to set the "Secure" attribute on session cookies, which instructs the browser to only send the cookie over secure connections. This prevents session hijacking attacks over unencrypted connections.
Here's an example in PHP:
session_set_cookie_params([ 'secure' => true, 'httponly' => true ]);
2. Protect Against Session Fixation
Session fixation is an attack where an attacker forces a victim to use a known session ID. To prevent this, it is essential to regenerate the session ID after an authentication event (e.g., login). This ensures that an attacker cannot use a previously obtained session ID to gain unauthorized access.
In PHP, you can use the session_regenerate_id()
function to regenerate the session ID:
session_regenerate_id(true);
3. Implement Session Expiry
Sessions should not last indefinitely. It is important to set an appropriate session expiry time to limit the time frame in which an attacker can exploit a session. The session expiry time should be short enough to minimize the risk but long enough to provide a seamless user experience.
In PHP, you can set the session expiry time using the session.cookie_lifetime
configuration option:
ini_set('session.cookie_lifetime', 3600); // 1 hour
Related Article: How To Distinguish Between POST And PUT In HTTP
4. Use CSRF Tokens
Cross-Site Request Forgery (CSRF) is an attack where an attacker tricks a user into performing unwanted actions in their authenticated session. To mitigate this risk, you should implement CSRF tokens. These tokens are unique per session and per request, making it difficult for an attacker to forge requests.
Here's an example of generating and verifying CSRF tokens in PHP:
// Generating CSRF token $token = bin2hex(random_bytes(32)); $_SESSION['csrf_token'] = $token; // Verifying CSRF token if (isset($_POST['csrf_token']) && $_SESSION['csrf_token'] === $_POST['csrf_token']) { // Valid token, process the request } else { // Invalid token, reject the request }
5. Secure Session Storage
The storage mechanism for sessions should be secure to prevent unauthorized access to session data. Avoid storing session data in plaintext files or databases accessible to attackers. Instead, consider using encrypted session storage or storing session data in a secure database.
6. Regularly Audit and Monitor Sessions
Regularly auditing and monitoring sessions can help identify any suspicious activities or potential security breaches. Monitor session logs, check for unusual or unexpected session behavior, and implement mechanisms to detect and prevent session hijacking.
By implementing these session security best practices, you can significantly reduce the risk of session-based attacks and ensure the confidentiality and integrity of your users' data.
Remember, session security is an ongoing concern, and it is important to stay up to date with the latest security practices and vulnerabilities.
Encrypting Data in Transit and at Rest
Data encryption is a crucial aspect of securing software applications. It ensures that sensitive information remains protected while it is being transmitted over a network or stored in a database. In this chapter, we will explore different encryption techniques that can be implemented to safeguard data in transit and at rest.
Related Article: Top 11 Emerging EdTech Companies of 2024
Encrypting Data in Transit
When data is transmitted over a network, it is vulnerable to interception and eavesdropping by malicious actors. To prevent unauthorized access, it is essential to encrypt data in transit using secure communication protocols such as HTTPS (HTTP over SSL/TLS). HTTPS encrypts the data exchanged between a client and a server, ensuring that it cannot be easily intercepted or tampered with.
To enable HTTPS in your application, you need to obtain an SSL/TLS certificate from a trusted certificate authority (CA). This certificate is used to establish a secure connection between the client and the server. Once the certificate is obtained, it needs to be installed on the server, and the application needs to be configured to use HTTPS.
Here is an example of configuring an Express.js server to use HTTPS:
const express = require('express'); const https = require('https'); const fs = require('fs'); const app = express(); const options = { key: fs.readFileSync('privateKey.key'), cert: fs.readFileSync('certificate.crt') }; https.createServer(options, app).listen(443);
In this example, we read the private key and certificate files from disk and pass them to the https.createServer
method to create an HTTPS server.
Encrypting Data at Rest
Encrypting data at rest involves protecting data when it is stored in databases, file systems, or any other storage medium. Even if an attacker gains unauthorized access to the storage, encrypted data remains unreadable without the decryption key.
One common approach to encrypting data at rest is to use database-level encryption. Many relational database management systems (RDBMS) offer built-in encryption features that allow you to encrypt specific columns or entire databases. For example, in MySQL, you can use the AES_ENCRYPT
and AES_DECRYPT
functions to encrypt and decrypt data.
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50), email VARCHAR(100), password VARBINARY(255) ); INSERT INTO users (name, email, password) VALUES ('John Doe', 'john@example.com', AES_ENCRYPT('password123', 'encryption_key'));
In this example, the password
column is encrypted using the AES_ENCRYPT
function and a secret encryption key. When retrieving the password, the AES_DECRYPT
function is used to decrypt it.
Another approach is to use application-level encryption, where data is encrypted before it is stored in the database. This provides an additional layer of security, as the encryption keys can be managed separately from the database.
const { createCipheriv, createDecipheriv } = require('crypto'); const algorithm = 'aes-256-cbc'; const key = 'encryption_key'; function encryptData(data) { const cipher = createCipheriv(algorithm, key, iv); let encrypted = cipher.update(data, 'utf8', 'hex'); encrypted += cipher.final('hex'); return encrypted; } function decryptData(encryptedData) { const decipher = createDecipheriv(algorithm, key, iv); let decrypted = decipher.update(encryptedData, 'hex', 'utf8'); decrypted += decipher.final('utf8'); return decrypted; } const data = 'sensitive information'; const encryptedData = encryptData(data); const decryptedData = decryptData(encryptedData); console.log('Encrypted Data:', encryptedData); console.log('Decrypted Data:', decryptedData);
In this example, we use the Node.js crypto
module to encrypt and decrypt data using the AES-256-CBC algorithm. The encryptData
and decryptData
functions take the data as input and return the encrypted and decrypted versions, respectively.
By implementing encryption techniques for data in transit and at rest, developers can significantly enhance the security of their software applications. It ensures that sensitive information remains protected, even if it falls into the wrong hands.
Securing APIs and Web Services
APIs and web services play a crucial role in modern software development, enabling different applications to communicate and share data. However, they also present a potential security risk if not properly secured. In this chapter, we will explore some best practices for securing APIs and web services to ensure the integrity, confidentiality, and availability of your software.
1. Authentication and Authorization
Implementing robust authentication and authorization mechanisms is essential to secure your APIs and web services. By authenticating users and verifying their access privileges, you can prevent unauthorized access to sensitive data.
One widely adopted approach is to use tokens, such as JSON Web Tokens (JWT), for authentication. JWT allows you to securely transmit user information between parties as a JSON object. Here's an example of how to generate and verify a JWT token in Node.js:
const jwt = require('jsonwebtoken'); // Generate a JWT token const token = jwt.sign({ userId: '123' }, 'secretKey', { expiresIn: '1h' }); // Verify a JWT token try { const decoded = jwt.verify(token, 'secretKey'); console.log(decoded); // { userId: '123', iat: 1632812353 } } catch (err) { console.error(err); }
Additionally, consider implementing role-based access control (RBAC) to grant users different levels of authorization based on their roles within the system.
2. Input Validation
Web services and APIs should always validate input data to prevent attacks such as SQL injection, cross-site scripting (XSS), and command injection. Input validation helps ensure that only expected and safe data is processed by your application.
For example, in a Node.js application using Express, you can use the express-validator
library to validate incoming requests. Here's an example of validating a request body parameter:
const { body, validationResult } = require('express-validator'); app.post('/api/user', [ body('email').isEmail().normalizeEmail(), body('password').isLength({ min: 8 }), ], (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Process the request });
Remember to sanitize user input to mitigate potential security vulnerabilities.
3. Secure Communication
Ensure secure communication between your APIs and web services by using encrypted protocols like HTTPS (HTTP over SSL/TLS). HTTPS encrypts the data exchanged between the client and server, preventing eavesdropping and tampering.
To enable HTTPS in a Node.js application, you can use the https
module along with SSL/TLS certificates. Here's an example:
const https = require('https'); const fs = require('fs'); const options = { key: fs.readFileSync('private-key.pem'), cert: fs.readFileSync('public-cert.pem'), }; https.createServer(options, (req, res) => { // Handle requests }).listen(443);
4. Rate Limiting
Implementing rate limiting helps protect your APIs and web services from abuse and denial-of-service attacks. By limiting the number of requests a user or IP address can make within a certain timeframe, you can prevent brute-force attacks and excessive resource consumption.
Various libraries and services exist to assist with rate limiting, such as express-rate-limit
for Express applications.
const rateLimit = require('express-rate-limit'); const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // maximum 100 requests per windowMs }); app.use(limiter);
By incorporating these best practices, you can significantly enhance the security of your APIs and web services. However, remember that security is an ongoing process, and it's vital to stay updated on the latest security threats and solutions in order to protect your software effectively.
Understanding Remote Code Execution
Remote Code Execution occurs when an attacker is able to inject and execute their own code on a target system. This can have severe consequences, ranging from unauthorized access to sensitive data to complete system compromise.
There are several common vectors through which RCE attacks can be carried out, including:
- User input: Attackers can exploit vulnerabilities in user input handling, such as insufficient input validation or sanitization, to inject malicious code.
- Deserialization: Insecure deserialization of data can allow attackers to execute arbitrary code.
- Command injection: Improper handling of user-supplied data in system commands can enable attackers to execute arbitrary commands.
- Code injection: Vulnerabilities in dynamic code execution, such as the use of eval() or other similar functions, can be exploited to execute arbitrary code.
Related Article: The issue with Monorepos
Best Practices to Protect Against Remote Code Execution
To protect against remote code execution vulnerabilities, developers should adhere to the following best practices:
1. Input Validation and Sanitization: Thoroughly validate and sanitize all user input to ensure it conforms to expected formats and does not contain any malicious code. Use whitelisting or strict input validation techniques to reject any unexpected or potentially harmful input.
2. Secure Deserialization: Implement strict controls and validation when deserializing data. Only deserialize trusted data from reliable sources and avoid using insecure deserialization frameworks or libraries.
3. Parameterized Queries: Always use parameterized queries or prepared statements when interacting with databases to prevent SQL injection attacks. Avoid concatenating user input directly into SQL queries.
4. Secure Code Execution: Avoid using dynamic code execution functions like eval() or exec() unless absolutely necessary. If unavoidable, ensure that any user input is properly validated, sanitized, and limited in scope.
5. Secure File Handling: Avoid using user-supplied input directly in file paths or names, as this can lead to path traversal attacks. Use a whitelist approach to restrict the type and location of files that can be accessed or executed.
6. Regular Security Updates: Keep all software dependencies and frameworks up to date with the latest security patches. Regularly monitor security advisories and apply updates promptly to mitigate any known vulnerabilities.
Auditing and Logging for Security
In any software application, it is crucial to have a robust logging mechanism in place to track and monitor security events. Auditing and logging play a significant role in identifying and investigating security breaches, as well as ensuring the overall integrity and security of the software.
Logging refers to the process of recording events, actions, and errors that occur within an application. It helps developers and system administrators to gain insights into the behavior of the software, detect anomalies, and troubleshoot issues. By analyzing logs, security professionals can identify potential security vulnerabilities, track user activities, and investigate any suspicious or malicious behavior.
Importance of Auditing and Logging
Auditing and logging are essential components of a comprehensive security strategy. They provide a valuable source of information for incident response, forensic analysis, and compliance requirements. Here are some key reasons why auditing and logging should be a priority for developers:
1. Threat Detection: Logs can help detect abnormal patterns or activities that may indicate a security breach or unauthorized access attempt. For example, if a user repeatedly fails authentication attempts, it may indicate a brute-force attack.
2. Forensic Analysis: When a security incident occurs, detailed logs can assist in investigating the cause, identifying the extent of the damage, and taking appropriate remedial actions. Logs can serve as crucial evidence during forensic analysis.
3. Compliance: Many industries and regulatory frameworks require organizations to maintain comprehensive logs for auditing and compliance purposes. Logging security events helps meet these requirements and provides an audit trail for accountability.
Best Practices for Auditing and Logging
To ensure effective auditing and logging, consider implementing the following best practices:
1. Define Clear Objectives: Clearly define what events and activities should be logged based on your application's security requirements. Focus on logging events that are relevant to security monitoring, threat detection, and compliance.
2. Log Sensitive Data Carefully: Be cautious when logging sensitive data such as personally identifiable information (PII) or authentication credentials. Ensure that sensitive information is properly anonymized or encrypted to protect user privacy.
3. Log Sufficient Information: Include enough contextual information in the logs to aid in troubleshooting and forensic analysis. Log relevant details such as timestamps, user IDs, IP addresses, input parameters, and relevant business context.
4. Implement Log Integrity: Ensure that logs are tamper-resistant and cannot be modified or deleted without appropriate controls. Consider using cryptographic hashes or digital signatures to verify the integrity of log files.
5. Centralized Logging: Establish a centralized logging system to aggregate logs from multiple sources. This helps in real-time monitoring, correlation, and analysis of security events across the entire software ecosystem.
6. Regular Log Review: Regularly review and analyze logs to identify potential security incidents or anomalies. Implement automated log analysis tools or scripts to assist in identifying patterns or abnormal behaviors.
Here's an example of how logging can be implemented in a Python Flask application:
import logging from flask import Flask app = Flask(__name__) @app.route('/') def hello(): app.logger.info('Hello endpoint accessed') return 'Hello, World!' if __name__ == '__main__': app.logger.setLevel(logging.INFO) app.run()
In the above example, the Flask application logs an information message whenever the '/' endpoint is accessed. The log messages can be stored in a file or sent to a centralized logging server for further analysis.
Related Article: The very best software testing tools
Performing Security Testing
Security testing is a crucial step in the software development lifecycle. It helps identify vulnerabilities and weaknesses in your codebase, allowing you to address them before they can be exploited by malicious attackers. In this chapter, we will explore different techniques and tools that can be used to perform security testing on your software.
1. Manual Code Review
One of the most effective ways to identify security flaws is through manual code review. By carefully examining your codebase line by line, you can uncover potential vulnerabilities such as SQL injection, cross-site scripting (XSS), or insecure data handling.
Here's an example of a code snippet where a SQL injection vulnerability can be found:
def login(username, password): query = "SELECT * FROM users WHERE username = '" + username + "' AND password = '" + password + "'" result = execute_query(query) if result: return "Login successful" else: return "Invalid credentials"
In this snippet, the username and password values are directly concatenated into the SQL query, making it susceptible to SQL injection attacks. To fix this, you should use parameterized queries or prepared statements to ensure that user input is properly sanitized.
2. Automated Static Analysis
Automated static analysis tools can greatly assist in identifying potential security vulnerabilities in your codebase. These tools analyze the source code without actually executing it, looking for patterns that may indicate security issues.
For example, you can use a tool like SonarQube to scan your codebase for common security flaws such as buffer overflows, insecure cryptographic algorithms, or improper input validation.
3. Dynamic Analysis
Dynamic analysis involves testing your software in a runtime environment to identify security vulnerabilities that may not be visible in the source code. This can be done through techniques like penetration testing or fuzz testing.
Penetration testing involves simulating real-world attacks on your software to identify potential weaknesses. This can be done manually or through the use of specialized tools like Metasploit.
Fuzz testing, on the other hand, involves feeding your software with invalid or unexpected inputs to see how it behaves. This can help uncover vulnerabilities such as buffer overflows or input validation issues.
Related Article: Monitoring Query Performance in Elasticsearch using Kibana
4. Third-party Security Testing
In addition to manual and automated testing, it is also advisable to have your software tested by third-party security experts. These experts can provide an unbiased perspective and help identify vulnerabilities that may have been missed during internal testing.
There are several companies that offer security testing services, such as Synopsys, WhiteHat Security, or Cobalt. These services typically involve a combination of automated scanning, manual testing, and code review to ensure comprehensive coverage.
Building a Secure Development Workflow
Developing software with security in mind is crucial in today's digital landscape. To create secure software, developers must integrate security practices into their development workflow. This chapter will guide you through building a secure development workflow that ensures the software you create is robust and protected against potential vulnerabilities.
1. Establishing a Secure Development Environment
The first step in building a secure development workflow is to establish a secure development environment. This includes the tools and practices you use during the development process. Here are some key considerations:
- Use version control systems like Git to track changes and collaborate with your team. This helps in identifying and reverting any malicious code introduced by unauthorized users.
- Regularly update and patch your development tools, libraries, and frameworks to ensure you're using the latest secure versions.
- Enable secure coding practices by enforcing code reviews, static code analysis, and coding standards. Tools like SonarQube and ESLint can help identify security vulnerabilities and coding errors.
2. Secure Coding Practices
Secure coding practices are essential for building secure software. Here are some best practices to follow:
- Validate all user inputs to prevent common vulnerabilities like SQL injection and Cross-Site Scripting (XSS). Use techniques like parameterized queries and input validation functions.
- Implement secure authentication and authorization mechanisms. Use strong password hashing algorithms like bcrypt or Argon2 and consider using multi-factor authentication.
- Sanitize and escape user-generated content to prevent XSS attacks. Utilize libraries like OWASP Java Encoder or PHP's htmlspecialchars function to sanitize user inputs.
Related Article: Top 12 AI Content Creation Tools of 2024
3. Testing and Vulnerability Assessments
Regular testing and vulnerability assessments are crucial for identifying and fixing security issues. Here are some techniques to consider:
- Conduct regular penetration testing to identify vulnerabilities and validate the effectiveness of your security controls. Tools like Burp Suite and OWASP ZAP can assist in performing these tests.
- Implement automated security testing into your continuous integration/continuous deployment (CI/CD) pipeline. Tools like Snyk and OWASP Dependency Check can scan your dependencies for known vulnerabilities.
- Perform threat modeling to identify potential security risks and prioritize security mitigations. Tools like Microsoft's Threat Modeling Tool can assist in this process.
4. Secure Deployment and Monitoring
A secure development workflow doesn't end with coding and testing. It's essential to ensure secure deployment and continuous monitoring. Consider the following:
- Implement secure deployment practices, such as using secure protocols (e.g., HTTPS) and secure configurations for your web servers and databases. Automate deployment processes to reduce the risk of human error.
- Implement logging and monitoring mechanisms to detect and respond to security incidents. Tools like ELK Stack (Elasticsearch, Logstash, Kibana) can assist in centralizing and analyzing logs.
- Regularly update and patch your production environment, including your operating system, web server, and database, to address any newly discovered vulnerabilities.
By incorporating these practices into your development workflow, you can significantly enhance the security of your software. Remember, security is an ongoing process, and it's crucial to stay up-to-date with the latest security best practices and emerging threats.
Securing Mobile Applications
Mobile applications have become an integral part of our lives, offering convenience and accessibility on the go. However, the increasing popularity of mobile apps has also made them a prime target for hackers. As a developer, it is crucial to understand the potential vulnerabilities in mobile applications and take steps to secure them. In this chapter, we will explore various techniques to secure mobile applications against common security threats.
1. Secure Data Storage
One of the first steps in securing a mobile application is to ensure that sensitive data is stored securely. This includes user credentials, personal information, and any other sensitive data collected by the app. Storing data in an encrypted format, both at rest and in transit, can help protect it from unauthorized access.
Here's an example of how to encrypt data using Android's SecurePreferences library:
// Encrypt data SecurePreferences preferences = new SecurePreferences(context, "my-preferences", "my-password", true); preferences.put("key", "value"); // Decrypt data String value = preferences.getString("key");
Related Article: Agile Shortfalls and What They Mean for Developers
2. Secure Network Communication
Mobile applications often communicate with remote servers to fetch data or perform actions. It is essential to secure these network communications to prevent eavesdropping, tampering, or man-in-the-middle attacks. Using secure protocols such as HTTPS and SSL/TLS can ensure the confidentiality and integrity of the data being transmitted.
Here's an example of how to establish a secure network connection in a Swift-based iOS application:
// Create a secure session configuration let sessionConfig = URLSessionConfiguration.default sessionConfig.httpAdditionalHeaders = ["Content-Type": "application/json"] // Configure SSL/TLS settings sessionConfig.urlSessionSecurityLevel = .strict // Create a URLSession with the secure configuration let session = URLSession(configuration: sessionConfig) // Make a secure network request let task = session.dataTask(with: request) { (data, response, error) in // Handle the response } task.resume()
3. Input Validation and Sanitization
Mobile applications receive input from various sources, including user interaction, network requests, and external data sources. It is crucial to validate and sanitize input to prevent common security vulnerabilities such as SQL injection, cross-site scripting (XSS), or remote code execution (RCE). Input validation should be performed both on the client-side and server-side.
Here's an example of input validation in a React Native application:
import React, { useState } from 'react'; import { TextInput, Button } from 'react-native'; const App = () => { const [email, setEmail] = useState(''); const handleEmailChange = (text) => { // Validate email input if (/^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(text)) { setEmail(text); } }; return ( <> <TextInput placeholder="Enter your email" onChangeText={handleEmailChange} value={email} /> <Button title="Submit" onPress={handleSubmit} /> </> ); };
4. Code Obfuscation and Anti-Tampering
Mobile applications are susceptible to reverse engineering, which can expose sensitive information and enable attackers to modify the code. Code obfuscation techniques can make it harder for hackers to understand the application's logic and extract valuable information. Additionally, anti-tampering mechanisms can detect and prevent unauthorized modifications to the application's code.
Here's an example of how to obfuscate code using ProGuard in an Android application:
# Keep application code and entry point -keep class com.example.app.** { *; } -keepclassmembers class com.example.app.** { *; } # Obfuscate everything else -keep,allowobfuscation class * { *; }
5. Regular Updates and Security Patches
New security vulnerabilities are discovered regularly, and it is essential to keep mobile applications up to date with the latest security patches. Regularly updating the application's dependencies, libraries, and frameworks can help address known vulnerabilities and protect against emerging threats. Additionally, monitoring security bulletins and staying informed about potential risks can ensure timely remediation.
In this chapter, we explored various techniques to secure mobile applications against common security threats. By incorporating these techniques into the development process, developers can enhance the security of their mobile applications and protect user data from unauthorized access. Remember, securing mobile applications is an ongoing process that requires continuous monitoring and improvement.
Related Article: Comparing GraphQL vs REST & How To Manage APIs
Defending Against DDoS Attacks
Distributed Denial of Service (DDoS) attacks are a common threat that can disrupt the availability of online services, causing significant financial and reputational damage to organizations. In this chapter, we will explore various techniques and strategies to defend against DDoS attacks and ensure the resilience of your software.
Understanding DDoS Attacks
DDoS attacks involve overwhelming a target system or network with a massive volume of traffic, rendering it unable to respond to legitimate requests. Attackers typically achieve this by leveraging a large number of compromised machines, forming a botnet, to flood the target with traffic.
There are several types of DDoS attacks, including:
1. **Volumetric Attacks**: These attacks aim to consume the target's network bandwidth by flooding it with a high volume of data packets. Examples include UDP floods and ICMP floods.
2. **Application Layer Attacks**: These attacks exploit vulnerabilities in the target's application layer, such as HTTP floods. By overwhelming the web server, attackers can exhaust its resources and impair its functionality.
3. **Protocol Attacks**: These attacks exploit weaknesses in network protocols, such as SYN floods or DNS amplification attacks. By exploiting the target's resources, attackers can disrupt its normal operation.
Implementing DDoS Mitigation Techniques
To defend against DDoS attacks, it is essential to implement proper mitigation techniques. Here are a few strategies you can employ:
1. **Traffic Filtering**: Implementing traffic filtering mechanisms, such as firewalls and intrusion prevention systems (IPS), can help identify and block malicious traffic. These systems can be configured to detect and drop traffic from suspicious or known malicious sources.
Example of traffic filtering using iptables in a Linux environment:
# Block all traffic from a specific IP address
iptables -A INPUT -s 123.45.67.89 -j DROP
2. **Rate Limiting**: Setting limits on the number of requests a server can handle per second or per minute can help prevent service overload. This can be achieved by implementing rate-limiting mechanisms at the network or application layer.
Example of rate limiting with Nginx:
http {
...
limit_req_zone $binary_remote_addr zone=one:10m rate=10r/s;
...
server {
...
location / {
limit_req zone=one burst=5;
...
}
...
}
}
3. **Load Balancing**: Distributing traffic across multiple servers using load balancers can help mitigate the impact of DDoS attacks. By spreading the load, the chances of overwhelming a single server are reduced.
Example of load balancing with HAProxy:
frontend my_frontend
bind *:80
mode http
default_backend my_backend
backend my_backend
mode http
balance roundrobin
server server1 192.168.0.1:80 check
server server2 192.168.0.2:80 check
Monitoring and Incident Response
Implementing proactive monitoring and incident response plans can help detect and mitigate DDoS attacks effectively. Monitoring network traffic, analyzing logs, and setting up alerts can provide early warning signs of an ongoing attack.
In the event of a DDoS attack, it is crucial to have a well-defined incident response plan. This plan should include procedures to isolate affected systems, divert traffic, and collaborate with network service providers to mitigate the attack.
Related Article: How to Start Your Data Science Journey with Kaggle in 2024
Exploring Cryptography Concepts
Cryptography is a fundamental component of secure software development. It involves the use of mathematical algorithms to encode data and protect it from unauthorized access. In this chapter, we will explore some important concepts related to cryptography and how they can be applied to secure software.
Symmetric Encryption
Symmetric encryption is a type of encryption where the same key is used for both encryption and decryption. This means that both the sender and the receiver of the encrypted data must possess the same secret key. One commonly used symmetric encryption algorithm is the Advanced Encryption Standard (AES).
Here is an example of how to use AES encryption in Python:
import os from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes from cryptography.hazmat.backends import default_backend def encrypt(plaintext, key): backend = default_backend() iv = os.urandom(16) cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend) encryptor = cipher.encryptor() ciphertext = encryptor.update(plaintext) + encryptor.finalize() return iv + ciphertext def decrypt(ciphertext, key): backend = default_backend() iv = ciphertext[:16] ciphertext = ciphertext[16:] cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend) decryptor = cipher.decryptor() plaintext = decryptor.update(ciphertext) + decryptor.finalize() return plaintext key = os.urandom(32) plaintext = b"Hello, world!" ciphertext = encrypt(plaintext, key) decrypted_text = decrypt(ciphertext, key)
In this example, we generate a random initialization vector (IV) and use it along with the key to encrypt the plaintext using AES in CBC mode. The IV is prepended to the ciphertext for later decryption. The same key and IV are used for decryption to retrieve the original plaintext.
Asymmetric Encryption
Asymmetric encryption, also known as public-key encryption, uses a pair of keys: a public key and a private key. The public key is used to encrypt data, while the private key is used to decrypt it. This enables secure communication between two parties without the need to exchange a shared secret key.
One widely used asymmetric encryption algorithm is the RSA algorithm. Here is an example of how to use RSA encryption in Java:
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import javax.crypto.Cipher; public class RSAExample { public static void main(String[] args) throws Exception { KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA"); keyPairGenerator.initialize(2048); KeyPair keyPair = keyPairGenerator.generateKeyPair(); PublicKey publicKey = keyPair.getPublic(); PrivateKey privateKey = keyPair.getPrivate(); Cipher cipher = Cipher.getInstance("RSA"); cipher.init(Cipher.ENCRYPT_MODE, publicKey); byte[] encryptedBytes = cipher.doFinal("Hello, world!".getBytes()); cipher.init(Cipher.DECRYPT_MODE, privateKey); byte[] decryptedBytes = cipher.doFinal(encryptedBytes); String decryptedText = new String(decryptedBytes); } }
In this example, we generate a key pair using the RSA algorithm with a key size of 2048 bits. We then use the public key to encrypt the plaintext and the private key to decrypt the ciphertext. The decrypted text should match the original plaintext.
Hash Functions
Hash functions are mathematical algorithms that convert input data into a fixed-size string of bytes, known as a hash value or hash code. One important property of hash functions is that even a small change in the input data should produce a significantly different hash value. This property makes hash functions useful for verifying the integrity of data and password storage.
Here is an example of how to calculate the SHA-256 hash of a string in C#:
using System; using System.Security.Cryptography; using System.Text; public class HashExample { public static string CalculateHash(string input) { using (SHA256 sha256 = SHA256.Create()) { byte[] inputBytes = Encoding.UTF8.GetBytes(input); byte[] hashBytes = sha256.ComputeHash(inputBytes); StringBuilder builder = new StringBuilder(); for (int i = 0; i < hashBytes.Length; i++) { builder.Append(hashBytes[i].ToString("x2")); } return builder.ToString(); } } public static void Main(string[] args) { string input = "Hello, world!"; string hash = CalculateHash(input); } }
In this example, we use the SHA-256 hash algorithm to calculate the hash value of a string. The hash value is then converted to a hexadecimal string representation for readability.
Related Article: Top 10 AI Assistants For Journalists in 2024
Understanding Social Engineering
Social engineering is a technique used by hackers to manipulate people into revealing sensitive information or performing certain actions that can compromise the security of a system. It relies on the manipulation of human psychology rather than exploiting technical vulnerabilities. In this chapter, we will explore different types of social engineering attacks and how developers can protect their software from such threats.
Types of Social Engineering Attacks
1. Phishing: Phishing is the most common type of social engineering attack. It involves sending deceptive emails, messages, or websites that appear to be from a trusted source, tricking users into providing sensitive information such as passwords, credit card details, or social security numbers. Developers should educate their users about the importance of verifying the authenticity of emails and websites before sharing any personal information.
2. Pretexting: Pretexting involves creating a fictional scenario or pretext to trick individuals into revealing information or performing actions they wouldn't typically do. For example, a hacker might pretend to be a co-worker or IT support personnel and ask for login credentials or access to sensitive data. Developers should encourage users to follow strict protocols for verifying the identity of individuals before sharing any sensitive information or granting access.
3. Baiting: Baiting involves offering something enticing, such as free software, movie downloads, or USB drives infected with malware, to entice users to take actions that compromise security. Developers should remind users to be cautious when accepting any external devices or downloading files from untrusted sources.
4. Tailgating: Tailgating occurs when an unauthorized individual gains physical access to restricted areas by following an authorized person. This can lead to unauthorized access to computer systems or sensitive information. Developers should ensure that physical security measures are in place to prevent unauthorized individuals from gaining access to restricted areas.
Protecting Against Social Engineering Attacks
Developers can take several steps to protect their software and users from social engineering attacks:
1. User Education: It is essential to educate users about social engineering attacks and how to identify and respond to them. Regular training sessions, email reminders, and informative materials can help users recognize and report suspicious activities.
2. Strong Authentication: Implementing strong authentication mechanisms, such as two-factor authentication, can significantly reduce the risk of unauthorized access to user accounts and sensitive data.
3. Vulnerability Assessments: Conduct regular vulnerability assessments and penetration tests to identify and address any potential security weaknesses in the software. This can help uncover vulnerabilities that social engineers may exploit.
4. Monitoring and Incident Response: Implement robust monitoring systems to detect and respond to suspicious activities. Regularly review logs and establish protocols for incident response to mitigate the impact of social engineering attacks.
5. Security Awareness: Foster a culture of security awareness within the development team and the organization as a whole. Encourage open communication about security concerns and provide channels for reporting potential threats.
By understanding social engineering tactics and implementing appropriate security measures, developers can protect their software and users from falling victim to social engineering attacks. It is crucial to remain vigilant and stay updated on emerging social engineering techniques to stay one step ahead of potential threats.
Securing IoT Devices
The rise of Internet of Things (IoT) devices has brought about numerous benefits, but it has also introduced new security challenges. These devices, ranging from smart home appliances to industrial sensors, often lack basic security measures, making them prime targets for hackers. In this chapter, we will explore some best practices for securing IoT devices and protecting them from potential vulnerabilities.
Related Article: Top 7 Healthcare Technologies for Mental Wellness in 2024
1. Implement Strong Authentication
One of the first steps in securing IoT devices is to implement strong authentication mechanisms. By requiring users to provide a unique username and password, you can ensure that only authorized individuals can access the device's functionalities. Additionally, consider implementing two-factor authentication (2FA) for an extra layer of security.
Here's an example of how to implement strong authentication in Python using Flask:
from flask import Flask, request, jsonify from werkzeug.security import generate_password_hash, check_password_hash app = Flask(__name__) users = { "admin": generate_password_hash("admin_password"), "user1": generate_password_hash("user1_password") } @app.route("/login", methods=["POST"]) def login(): username = request.json.get("username") password = request.json.get("password") if username not in users or not check_password_hash(users[username], password): return jsonify({"error": "Invalid credentials"}), 401 # Continue with login process return jsonify({"message": "Login successful"}), 200 if __name__ == "__main__": app.run()
2. Encrypt Communication
IoT devices often communicate with other devices or servers over networks. It is crucial to encrypt these communications to prevent unauthorized access or data interception. Transport Layer Security (TLS) or Secure Sockets Layer (SSL) protocols can be used to establish secure connections.
Here's an example of how to enable SSL/TLS in a Node.js application using the Express framework:
const express = require("express"); const https = require("https"); const fs = require("fs"); const app = express(); const options = { key: fs.readFileSync("private.key"), cert: fs.readFileSync("certificate.crt") }; app.get("/", (req, res) => { res.send("Hello, secure world!"); }); https.createServer(options, app).listen(443);
3. Regularly Update Firmware
IoT devices often receive firmware updates to fix bugs, add new features, and address security vulnerabilities. It is essential to keep these devices up to date by regularly checking for firmware updates and applying them promptly. Manufacturers typically release these updates through their websites or over-the-air (OTA) updates.
4. Follow the Principle of Least Privilege
To minimize the potential impact of a compromised IoT device, it is crucial to follow the principle of least privilege. Each device should only have the necessary permissions and access rights to perform its intended functions. Restricting unnecessary privileges reduces the attack surface and limits the potential damage a hacker can cause.
Related Article: Comparing Sorting Algorithms (with Java Code Snippets)
5. Implement Secure Software Development Practices
Secure software development practices play a vital role in building robust and secure IoT devices. Follow secure coding guidelines, perform regular code reviews, and conduct thorough security testing during the development process. Additionally, consider implementing static code analysis tools to identify potential vulnerabilities early on.