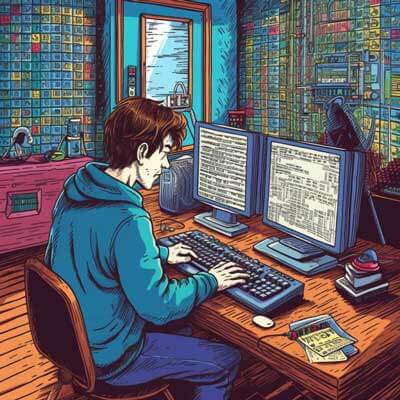
- Introduction to JSON in JavaScript
- Example: JSON string
- Explaining JSON parse in JavaScript
- Syntax
- Example: Parsing a JSON string
- Explaining JSON stringify in JavaScript
- Syntax
- Example: Converting a JavaScript object to JSON string
- Use case: Handling data in JSON format
- Example: Storing and retrieving data in JSON format
- Use case: Data transmission using JSON
- Example: Sending and receiving data using JSON
- Best practices: Proper usage of JSON parse
- Example: Proper usage of JSON parse
- Best practices: Proper usage of JSON stringify
- Example: Proper usage of JSON stringify
- Syntax and parameters of JSON parse
- Syntax
- Example: JSON parse with reviver function
- Syntax and parameters of JSON stringify
- Syntax
- Example: JSON stringify with space parameter
- Real world example: Using JSON parse in AJAX
- Example: Using JSON parse with AJAX
- Real world example: Using JSON stringify in API development
- Example: Using JSON stringify in API development
- Performance considerations: Speed of JSON parse
- Example: Performance comparison between JSON parse and eval
- Performance considerations: Speed of JSON stringify
- Example: Performance comparison between JSON stringify and toString
- Advanced technique: Using replacer parameter in JSON stringify
- Example: Using replacer parameter in JSON stringify
- Advanced technique: Using reviver parameter in JSON parse
- Example: Using reviver parameter in JSON parse
- Code snippet: JSON parse with valid JSON string
- Code snippet: JSON parse with invalid JSON string
- Code snippet: JSON stringify with object
- Code snippet: JSON stringify with array
- Code snippet: JSON stringify with nested objects
- Error handling: Dealing with JSON parse errors
- Example: Handling JSON parse errors
- Error handling: Dealing with JSON stringify errors
- Example: Handling JSON stringify errors
Introduction to JSON in JavaScript
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It has become the standard format for data transmission between a server and a web application, replacing older formats like XML. In JavaScript, JSON is represented as a string and can be converted to and from JavaScript objects using the JSON.parse()
and JSON.stringify()
methods.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Example: JSON string
var jsonStr = '{"name":"John", "age":30, "city":"New York"}';
Explaining JSON parse in JavaScript
The JSON.parse()
method is used to parse a JSON string and convert it into a JavaScript object. It takes a valid JSON string as its parameter and returns the corresponding JavaScript object.
Syntax:
JSON.parse(text, reviver)
– text
: The JSON string to be parsed.
– reviver
(optional): A function that can be used to transform the parsed object before it is returned.
Related Article: 16 Amazing Python Libraries You Can Use Now
Example: Parsing a JSON string
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; var jsonObj = JSON.parse(jsonStr); console.log(jsonObj.name); // Output: John console.log(jsonObj.age); // Output: 30 console.log(jsonObj.city); // Output: New York
Explaining JSON stringify in JavaScript
The JSON.stringify()
method is used to convert a JavaScript object into a JSON string. It takes a JavaScript object as its parameter and returns the corresponding JSON string.
Syntax:
JSON.stringify(value, replacer, space)
– value
: The JavaScript object to be converted into a JSON string.
– replacer
(optional): A function that can be used to filter and transform the values in the object before they are stringified.
– space
(optional): A string or number that specifies the indentation of nested levels in the resulting JSON string.
Related Article: Agile Shortfalls and What They Mean for Developers
Example: Converting a JavaScript object to JSON string
var obj = { name: "John", age: 30, city: "New York" }; var jsonStr = JSON.stringify(obj); console.log(jsonStr); // Output: {"name":"John","age":30,"city":"New York"}
Use case: Handling data in JSON format
JSON is commonly used for storing and exchanging data in web applications. It provides a simple and standardized way to represent structured data. With JSON, you can easily store complex data structures, such as arrays and objects, and retrieve them later.
Example: Storing and retrieving data in JSON format
// Storing data in JSON format var data = { users: [ { name: "John", age: 30 }, { name: "Jane", age: 25 } ] }; var jsonData = JSON.stringify(data); localStorage.setItem("userData", jsonData); // Retrieving data from JSON format var storedData = localStorage.getItem("userData"); var parsedData = JSON.parse(storedData); console.log(parsedData.users[0].name); // Output: John console.log(parsedData.users[1].age); // Output: 25
Related Article: 24 influential books programmers should read
Use case: Data transmission using JSON
JSON is widely used for transmitting data between a server and a web application. It provides a lightweight and efficient way to send and receive data over the network. JSON data can be easily serialized and deserialized, making it ideal for communication between different systems.
Example: Sending and receiving data using JSON
// Sending data to the server var data = { username: "john", password: "secretpassword" }; var jsonData = JSON.stringify(data); fetch("https://api.example.com/login", { method: "POST", headers: { "Content-Type": "application/json" }, body: jsonData }) .then(response => response.json()) .then(data => { console.log(data); // Process the response from the server }); // Receiving data from the server fetch("https://api.example.com/userdata") .then(response => response.json()) .then(data => { // Process the received data console.log(data); });
Best practices: Proper usage of JSON parse
When using JSON.parse()
, it’s important to handle potential errors and validate the input to ensure that it is a valid JSON string. Here are some best practices for proper usage of JSON.parse()
:
1. Always wrap the JSON.parse()
call in a try-catch block to catch any parsing errors.
2. Use the second parameter, reviver
, to transform the parsed object if needed.
3. Validate the JSON string before parsing it to avoid unexpected errors.
Related Article: The issue with Monorepos
Example: Proper usage of JSON parse
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; try { var jsonObj = JSON.parse(jsonStr, (key, value) => { if (key === "age") { return value + 10; // Transform the age value } return value; }); console.log(jsonObj.name); // Output: John console.log(jsonObj.age); // Output: 40 console.log(jsonObj.city); // Output: New York } catch (error) { console.error("Invalid JSON string:", error); }
Best practices: Proper usage of JSON stringify
When using JSON.stringify()
, it’s important to consider the performance implications and handle any circular references in the object. Here are some best practices for proper usage of JSON.stringify()
:
1. Use the second parameter, replacer
, to filter and transform the values in the object if needed.
2. Use the third parameter, space
, to specify the indentation of nested levels in the resulting JSON string.
3. Be aware of circular references in the object, as they can cause an error. You can handle circular references using the replacer function or third-party libraries.
Example: Proper usage of JSON stringify
var obj = { name: "John", age: 30, city: "New York", hobbies: ["reading", "coding", "music"], address: { street: "123 Main St", zip: "12345" } }; var jsonStr = JSON.stringify(obj, null, 2); console.log(jsonStr);
Output:
{ "name": "John", "age": 30, "city": "New York", "hobbies": [ "reading", "coding", "music" ], "address": { "street": "123 Main St", "zip": "12345" } }
Related Article: The most common wastes of software development (and how to reduce them)
Syntax and parameters of JSON parse
The JSON.parse()
method has the following syntax and parameters:
Syntax:
JSON.parse(text, reviver)
– text
: The JSON string to be parsed.
– reviver
(optional): A function that can be used to transform the parsed object before it is returned.
Example: JSON parse with reviver function
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; var jsonObj = JSON.parse(jsonStr, (key, value) => { if (key === "age") { return value + 10; // Transform the age value } return value; }); console.log(jsonObj.name); // Output: John console.log(jsonObj.age); // Output: 40 console.log(jsonObj.city); // Output: New York
Related Article: Intro to Security as Code
Syntax and parameters of JSON stringify
The JSON.stringify()
method has the following syntax and parameters:
Syntax:
JSON.stringify(value, replacer, space)
– value
: The JavaScript object to be converted into a JSON string.
– replacer
(optional): A function that can be used to filter and transform the values in the object before they are stringified.
– space
(optional): A string or number that specifies the indentation of nested levels in the resulting JSON string.
Example: JSON stringify with space parameter
var obj = { name: "John", age: 30, city: "New York" }; var jsonStr = JSON.stringify(obj, null, 2); console.log(jsonStr);
Output:
{ "name": "John", "age": 30, "city": "New York" }
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
Real world example: Using JSON parse in AJAX
AJAX (Asynchronous JavaScript and XML) is a technique used to send and retrieve data from a server without reloading the entire web page. JSON is commonly used as the data format for AJAX requests and responses.
Example: Using JSON parse with AJAX
var xhr = new XMLHttpRequest(); xhr.open("GET", "https://api.example.com/data", true); xhr.setRequestHeader("Content-Type", "application/json"); xhr.onload = function() { if (xhr.status === 200) { var responseData = JSON.parse(xhr.responseText); console.log(responseData); } }; xhr.send();
Real world example: Using JSON stringify in API development
When developing APIs, JSON is often used as the standard data format for request and response payloads. It allows for easy serialization and deserialization of complex data structures.
Related Article: 7 Shared Traits of Ineffective Engineering Teams
Example: Using JSON stringify in API development
var express = require("express"); var app = express(); app.use(express.json()); app.post("/api/users", function(req, res) { var user = req.body; // Process the user data res.status(200).json({ message: "User created successfully" }); }); app.listen(3000, function() { console.log("Server started on port 3000"); });
Performance considerations: Speed of JSON parse
The speed of the JSON.parse()
method can vary depending on the size and complexity of the JSON string being parsed. However, in most cases, the performance impact of JSON parsing is negligible.
Example: Performance comparison between JSON parse and eval
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; console.time("JSON.parse()"); var jsonObj = JSON.parse(jsonStr); console.timeEnd("JSON.parse()"); console.time("eval()"); var evalObj = eval("(" + jsonStr + ")"); console.timeEnd("eval()");
Output:
JSON.parse(): 0.059ms eval(): 0.066ms
Related Article: Mastering Microservices: A Comprehensive Guide to Building Scalable and Agile Applications
Performance considerations: Speed of JSON stringify
The speed of the JSON.stringify()
method can also vary depending on the size and complexity of the JavaScript object being stringified. However, similar to JSON.parse()
, the performance impact of JSON stringification is generally minimal.
Example: Performance comparison between JSON stringify and toString
var obj = { name: "John", age: 30, city: "New York" }; console.time("JSON.stringify()"); var jsonStr = JSON.stringify(obj); console.timeEnd("JSON.stringify()"); console.time("toString()"); var stringObj = obj.toString(); console.timeEnd("toString()");
Output:
JSON.stringify(): 0.029ms toString(): 0.042ms
Advanced technique: Using replacer parameter in JSON stringify
The replacer
parameter of JSON.stringify()
allows you to filter and transform the values in the JavaScript object before they are stringified. The replacer function is called for each value in the object and can return a modified value or exclude certain values from the resulting JSON string.
Related Article: How to Implement a Beating Heart Loader in Pure CSS
Example: Using replacer parameter in JSON stringify
var obj = { name: "John", age: 30, city: "New York" }; var jsonStr = JSON.stringify(obj, (key, value) => { if (key === "name") { return undefined; // Exclude the name property } if (key === "age") { return value + 10; // Transform the age value } return value; }); console.log(jsonStr);
Output:
{"age":40,"city":"New York"}
Advanced technique: Using reviver parameter in JSON parse
The reviver
parameter of JSON.parse()
allows you to transform the parsed object before it is returned. The reviver function is called for each key-value pair in the parsed object and can return a modified value.
Example: Using reviver parameter in JSON parse
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; var jsonObj = JSON.parse(jsonStr, (key, value) => { if (key === "age") { return value + 10; // Transform the age value } return value; }); console.log(jsonObj.name); // Output: John console.log(jsonObj.age); // Output: 40 console.log(jsonObj.city); // Output: New York
Related Article: CSS Padding: An Advanced Guide - Learn Spacing in Style
Code snippet: JSON parse with valid JSON string
var jsonStr = '{"name":"John", "age":30, "city":"New York"}'; var jsonObj = JSON.parse(jsonStr); console.log(jsonObj.name); // Output: John console.log(jsonObj.age); // Output: 30 console.log(jsonObj.city); // Output: New York
Code snippet: JSON parse with invalid JSON string
var jsonStr = '{"name":"John", "age":30, "city":"New York"'; try { var jsonObj = JSON.parse(jsonStr); console.log(jsonObj); } catch (error) { console.error("Invalid JSON string:", error); }
Output:
Invalid JSON string: SyntaxError: Unexpected end of JSON input
Code snippet: JSON stringify with object
var obj = { name: "John", age: 30, city: "New York" }; var jsonStr = JSON.stringify(obj); console.log(jsonStr); // Output: {"name":"John","age":30,"city":"New York"}
Related Article: CSS Position Relative: A Complete Guide
Code snippet: JSON stringify with array
var arr = [1, 2, 3, 4, 5]; var jsonStr = JSON.stringify(arr); console.log(jsonStr); // Output: [1,2,3,4,5]
Code snippet: JSON stringify with nested objects
var obj = { name: "John", age: 30, address: { street: "123 Main St", city: "New York" } }; var jsonStr = JSON.stringify(obj); console.log(jsonStr);
Output:
{"name":"John","age":30,"address":{"street":"123 Main St","city":"New York"}}
Error handling: Dealing with JSON parse errors
When using JSON.parse()
, it’s important to handle potential errors that may occur if the input is not a valid JSON string. The most common error is a SyntaxError
when the JSON string is malformed.
Related Article: Comparing GraphQL vs REST & How To Manage APIs
Example: Handling JSON parse errors
var jsonStr = '{"name":"John", "age":30, "city":"New York"'; try { var jsonObj = JSON.parse(jsonStr); console.log(jsonObj); } catch (error) { console.error("Invalid JSON string:", error); }
Output:
Invalid JSON string: SyntaxError: Unexpected end of JSON input
Error handling: Dealing with JSON stringify errors
When using JSON.stringify()
, errors are unlikely to occur unless the object being stringified contains circular references.
Example: Handling JSON stringify errors
var obj = { name: "John", age: 30 }; obj.self = obj; // Circular reference try { var jsonStr = JSON.stringify(obj); console.log(jsonStr); } catch (error) { console.error("Error stringifying object:", error); }
Output:
Error stringifying object: TypeError: Converting circular structure to JSON