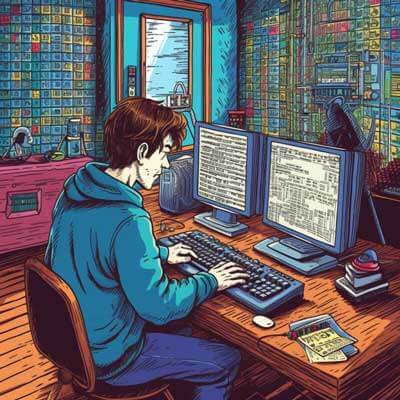
Table of Contents
Chapter 1: Defining JSON
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and for machines to parse and generate. It is widely used for transmitting data between a server and a web application, as well as for storing and exchanging data.
JSON is based on a subset of the JavaScript Programming Language, specifically the object literal syntax. It provides a simple and flexible way to represent structured data in a text format.
JSON data is organized into key-value pairs, where the key is always a string enclosed in double quotes, and the value can be any valid JSON data type, including objects, arrays, numbers, strings, booleans, and null.
Here's an example of a JSON object:
{ "name": "John Doe", "age": 30, "email": "john.doe@example.com"}
In this example, "name", "age", and "email" are the keys, and "John Doe", 30, and "john.doe@example.com" are the corresponding values.
Related Article: How to Use Embedded JavaScript (EJS) in Node.js
Example: Creating a JSON Object
To create a JSON object in JavaScript, you can define a JavaScript object literal and then use the JSON.stringify()
method to convert it to a JSON string:
const person = { name: "John Doe", age: 30, email: "john.doe@example.com"};const jsonString = JSON.stringify(person);console.log(jsonString);
Output:
{"name":"John Doe","age":30,"email":"john.doe@example.com"}
Example: Parsing a JSON String
To parse a JSON string and convert it into a JavaScript object, you can use the JSON.parse()
method:
const jsonString = '{"name":"John Doe","age":30,"email":"john.doe@example.com"}';const person = JSON.parse(jsonString);console.log(person.name); // Output: John Doeconsole.log(person.age); // Output: 30console.log(person.email); // Output: john.doe@example.com
In this example, the JSON string jsonString
is parsed using JSON.parse()
and assigned to the person
variable. You can then access the individual properties of the person object using dot notation.
Chapter 2: JSON Syntax
The JSON syntax is simple and easy to understand. It follows a set of rules that define how data should be structured and represented in a JSON format.
Here are some key syntax rules:
- Data is represented in name/value pairs.
- Names (keys) must be enclosed in double quotes.
- Values can be strings, numbers, booleans, arrays, objects, or null.
- Multiple name/value pairs are separated by commas.
- Curly braces {}
define objects.
- Square brackets []
define arrays.
Here's an example that demonstrates the JSON syntax:
{ "name": "John Doe", "age": 30, "email": "john.doe@example.com", "hobbies": ["reading", "traveling"], "address": { "street": "123 Main St", "city": "New York" }, "isActive": true, "score": null}
In this example, the JSON object contains various data types, including strings, numbers, arrays, objects, booleans, and null.
Related Article: How To Use Enctype Multipart Form Data
Example: Serializing JSON to a String
To convert a JavaScript object to a JSON string, you can use the JSON.stringify()
method:
const person = { name: "John Doe", age: 30, email: "john.doe@example.com"};const jsonString = JSON.stringify(person);console.log(jsonString);
Output:
{"name":"John Doe","age":30,"email":"john.doe@example.com"}
In this example, the person
object is serialized to a JSON string using JSON.stringify()
. The resulting JSON string can then be transmitted or stored as needed.
Example: Accessing JSON Data
To access data in a JSON object, you can use dot notation or square bracket notation:
const jsonString = '{"name":"John Doe","age":30,"email":"john.doe@example.com"}';const person = JSON.parse(jsonString);console.log(person.name); // Output: John Doeconsole.log(person["age"]); // Output: 30
In this example, the person
object is accessed using dot notation (person.name
) and square bracket notation (person["age"]
) to retrieve the corresponding values.
This allows you to extract specific data from a JSON object for further processing or manipulation.