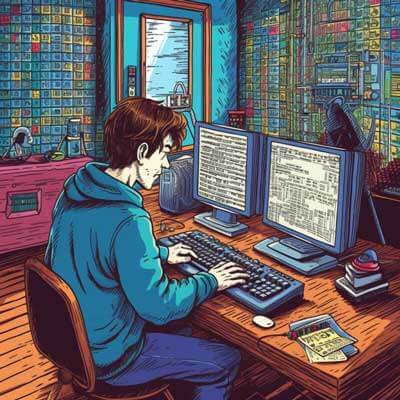
Table of Contents
Introduction to OOPs and Abstraction
Abstraction is a fundamental concept in object-oriented programming (OOP). OOP is a programming paradigm that revolves around the concept of objects, which are instances of classes. Abstraction allows us to represent real-world entities as objects in our code, while hiding unnecessary implementation details.
In OOP, abstraction is achieved through the use of abstract classes and interfaces. An abstract class is a class that cannot be instantiated and is meant to be subclassed. It can contain both abstract and non-abstract methods. An abstract method is a method that is declared but does not have an implementation. Subclasses of an abstract class must provide an implementation for all the abstract methods.
Let's take a look at a simple example in Java:
abstract class Shape { public abstract double calculateArea(); } class Rectangle extends Shape { private double length; private double width; public Rectangle(double length, double width) { this.length = length; this.width = width; } @Override public double calculateArea() { return length * width; } } class Circle extends Shape { private double radius; public Circle(double radius) { this.radius = radius; } @Override public double calculateArea() { return Math.PI * radius * radius; } } public class Main { public static void main(String[] args) { Shape rectangle = new Rectangle(5, 3); System.out.println("Rectangle area: " + rectangle.calculateArea()); Shape circle = new Circle(2.5); System.out.println("Circle area: " + circle.calculateArea()); } }
In this example, we have an abstract class Shape
that defines the abstract method calculateArea()
. The concrete classes Rectangle
and Circle
extend the Shape
class and provide their own implementation of the calculateArea()
method. We can create objects of the Rectangle
and Circle
classes and call the calculateArea()
method to calculate the areas of different shapes.
Related Article: Ethical Hacking: A Developer’s Guide to Securing Software
Defining Abstraction in OOPs Context
In the context of OOP, abstraction refers to the process of simplifying complex systems by breaking them down into smaller, more manageable components. It allows us to focus on the essential features and behaviors of an object while ignoring the implementation details that are not relevant to the current context.
Abstraction helps in reducing complexity, improving code maintainability, and promoting code reusability. By abstracting away the implementation details, we can create modular and loosely coupled code, making it easier to modify, extend, and test.
Example: Abstraction in Database Management
One common use case of abstraction in software development is in database management. When working with databases, we often use an abstraction layer such as an Object-Relational Mapping (ORM) tool to interact with the database.
Let's consider an example in Python using the popular ORM library, SQLAlchemy:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker # Database configuration engine = create_engine('sqlite:///mydatabase.db') Base = declarative_base() Session = sessionmaker(bind=engine) session = Session() # Define the model class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) email = Column(String) def __repr__(self): return f"<User(name='{self.name}', email='{self.email}')>" # Create a new user new_user = User(name='John Doe', email='john@example.com') session.add(new_user) session.commit() # Query the users users = session.query(User).all() for user in users: print(user)
In this example, we define a User
class that represents a user in our application. The class inherits from the Base
class provided by SQLAlchemy, which handles the abstraction of the underlying database. We define the table name and columns using class attributes, and SQLAlchemy takes care of mapping these attributes to the database schema.
We can create new users, query existing users, and perform various database operations using the ORM abstraction provided by SQLAlchemy. This allows us to work with the database without worrying about the specific database engine or writing raw SQL queries.
Example: Abstraction in GUI Design
Another area where abstraction plays a crucial role is in graphical user interface (GUI) design. GUI frameworks provide abstractions to simplify the process of creating user interfaces and handling user interactions.
Let's consider an example in C# using the Windows Forms framework:
using System; using System.Windows.Forms; public class MainForm : Form { private Button button; public MainForm() { button = new Button(); button.Text = "Click me"; button.Click += Button_Click; Controls.Add(button); } private void Button_Click(object sender, EventArgs e) { MessageBox.Show("Button clicked!"); } public static void Main() { Application.Run(new MainForm()); } }
In this example, we create a simple Windows Forms application with a single button. We define the behavior of the button by subscribing to its Click
event and providing a callback method. When the button is clicked, a message box is displayed with the message "Button clicked!".
The Windows Forms framework abstracts away the complexities of creating and managing windows, controls, and user input. We can focus on the high-level logic of our application without getting bogged down in the low-level details of window handling and event processing.
Related Article: The issue with Monorepos
Conceptual Framework of Abstraction
At a conceptual level, abstraction can be understood as a process of creating models or representations of real-world entities in our code. These models capture the essential characteristics and behaviors of the entities while omitting unnecessary details.
Abstraction involves identifying the relevant attributes and behaviors of an entity and representing them using classes, objects, methods, and properties. It allows us to create a simplified and more manageable representation of complex systems, making it easier to reason about and work with.
The level of abstraction can vary depending on the context and the goals of the software. Higher levels of abstraction provide a more abstract and generalized view of the system, while lower levels of abstraction deal with more specific details and implementation concerns.
The Importance of Abstraction in Software Development
Abstraction plays a crucial role in software development for several reasons:
1. Simplification: Abstraction simplifies complex systems by breaking them down into manageable components. It helps in managing complexity and reduces cognitive load, making it easier to understand, modify, and maintain the code.
2. Modularity: By abstracting away implementation details, we can create modular code that is easier to reuse, test, and modify. Abstraction promotes loose coupling between components, allowing them to be developed and tested independently.
3. Code Reusability: Abstraction enables code reusability by providing a higher-level interface that can be used in different contexts. Abstract classes, interfaces, and design patterns help in creating reusable code components that can be easily adapted and extended.
4. Flexibility: Abstraction allows for flexibility in the design and implementation of software systems. It provides a level of indirection between components, making it easier to introduce changes and adapt to evolving requirements.
5. Maintainability: Abstraction improves code maintainability by isolating changes to specific components. When implementation details are abstracted away, modifications can be made to the underlying code without affecting the rest of the system.
Overall, abstraction is a powerful tool that helps in managing complexity, improving code quality, promoting reusability, and enabling flexibility in software development.
Abstraction in OOPs: Theoretical Aspects
In the context of OOP, abstraction is achieved through the use of abstract classes and interfaces. Abstract classes cannot be instantiated and are meant to be subclassed. They can contain both abstract and non-abstract methods.
Abstract methods are declared without an implementation and must be implemented by concrete subclasses. They define a contract that the subclasses must adhere to. Abstract classes provide a way to define common behavior and attributes that can be shared by multiple subclasses.
Interfaces, on the other hand, are similar to abstract classes but cannot contain any implementation. They define a set of method signatures that implementing classes must provide. Interfaces enable polymorphism and provide a way to achieve abstraction across different class hierarchies.
Abstraction allows us to create a hierarchy of classes and interfaces that represent different levels of abstraction. It provides a way to define common behaviors and attributes at higher levels of the hierarchy and specialize them at lower levels.
Practical Aspects of Abstraction in OOPs
In practice, abstraction is a fundamental building block of software development. It allows us to create modular and maintainable code by hiding unnecessary implementation details and focusing on the essential features and behaviors.
Let's explore some practical aspects of abstraction in OOP:
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
Best Practice: Abstraction in Class Design
When designing classes, it is important to identify the essential attributes and behaviors and abstract them into appropriate classes. This promotes encapsulation and allows for better organization of code.
For example, consider a banking application that needs to represent different types of accounts such as savings accounts and checking accounts. We can create an abstract Account
class that defines common attributes and methods, and then subclass it to create specific account types.
abstract class Account { private String accountNumber; private double balance; public Account(String accountNumber) { this.accountNumber = accountNumber; this.balance = 0.0; } public void deposit(double amount) { balance += amount; } public abstract void withdraw(double amount); public double getBalance() { return balance; } } class SavingsAccount extends Account { private double interestRate; public SavingsAccount(String accountNumber, double interestRate) { super(accountNumber); this.interestRate = interestRate; } @Override public void withdraw(double amount) { // Implement withdrawal logic specific to savings accounts } } class CheckingAccount extends Account { private double overdraftLimit; public CheckingAccount(String accountNumber, double overdraftLimit) { super(accountNumber); this.overdraftLimit = overdraftLimit; } @Override public void withdraw(double amount) { // Implement withdrawal logic specific to checking accounts } } public class Main { public static void main(String[] args) { Account savingsAccount = new SavingsAccount("SA123456", 0.05); savingsAccount.deposit(1000); savingsAccount.withdraw(500); System.out.println("Savings account balance: " + savingsAccount.getBalance()); Account checkingAccount = new CheckingAccount("CA987654", 1000); checkingAccount.deposit(2000); checkingAccount.withdraw(3000); System.out.println("Checking account balance: " + checkingAccount.getBalance()); } }
In this example, we define an abstract Account
class that represents a generic bank account. It provides methods for depositing and withdrawing money and requires subclasses to implement the withdraw()
method according to their specific logic.
We then subclass the Account
class to create SavingsAccount
and CheckingAccount
classes, which represent different types of bank accounts. Each subclass provides its own implementation of the withdraw()
method.
By abstracting the common behaviors and attributes into the Account
class, we can easily add new account types in the future without duplicating code or modifying the existing implementation.
Best Practice: Abstraction for Code Reusability
Abstraction enables code reusability by providing a higher-level interface that can be used in different contexts. By defining abstract classes and interfaces, we can create reusable code components that can be easily adapted and extended.
For example, consider a simple application that needs to perform various mathematical operations such as addition, subtraction, multiplication, and division. We can define an abstract MathOperation
class that provides a common interface for performing these operations.
from abc import ABC, abstractmethod class MathOperation(ABC): @abstractmethod def perform_operation(self, operand1, operand2): pass class Addition(MathOperation): def perform_operation(self, operand1, operand2): return operand1 + operand2 class Subtraction(MathOperation): def perform_operation(self, operand1, operand2): return operand1 - operand2 class Multiplication(MathOperation): def perform_operation(self, operand1, operand2): return operand1 * operand2 class Division(MathOperation): def perform_operation(self, operand1, operand2): if operand2 != 0: return operand1 / operand2 else: raise ZeroDivisionError("Division by zero is not allowed") # Usage addition = Addition() result = addition.perform_operation(5, 3) print("Addition result:", result) division = Division() result = division.perform_operation(10, 2) print("Division result:", result)
In this example, the MathOperation
class defines an abstract method perform_operation()
that subclasses must implement. We then create concrete subclasses for each mathematical operation, namely Addition
, Subtraction
, Multiplication
, and Division
.
By using the common interface provided by the MathOperation
class, we can easily switch between different operations without modifying the calling code. This promotes code reusability and allows for easy addition of new operations in the future.
Real World Example: Abstraction in Framework Design
Abstraction is a key aspect of framework design. Frameworks provide a set of reusable components and abstractions that simplify the development of applications in specific domains.
One example of a framework that heavily utilizes abstraction is the Django web framework in Python. Django abstracts away many low-level details of web development, allowing developers to focus on building web applications.
For instance, in Django, models are defined using Python classes that inherit from the django.db.models.Model
class. This abstract base class provides a way to define database schemas using class attributes, without directly interacting with the underlying database.
from django.db import models class Book(models.Model): title = models.CharField(max_length=200) author = models.CharField(max_length=100) publication_date = models.DateField() def __str__(self): return self.title
In this example, the Book
class defines a model for a book with attributes such as title
, author
, and publication_date
. By subclassing the models.Model
class, Django takes care of creating the corresponding database table, mapping the class attributes to the table columns, and providing a high-level API for querying and manipulating the data.
Abstraction in frameworks like Django allows developers to focus on the domain-specific aspects of their applications without getting bogged down by the intricacies of low-level operations. It promotes code reuse, simplifies development, and improves productivity.
Real World Example: Abstraction in Game Development
Abstraction is also crucial in game development, where complex systems and interactions need to be managed. Game engines provide abstractions that simplify the process of creating games and handling various aspects such as graphics, physics, input handling, and audio.
One popular game engine that utilizes abstraction is Unity, which allows developers to create games for multiple platforms using a visual editor and a scripting language such as C#.
In Unity, game objects are the basic building blocks of a game scene. They can represent characters, items, obstacles, or any other element in the game. Each game object consists of components, which define its behavior and appearance.
For example, consider a simple 2D game where the player controls a character that collects coins. We can create a Player
game object with a Rigidbody2D
component for physics simulation, a SpriteRenderer
component for rendering the character sprite, and a custom PlayerController
script for handling user input and movement.
using UnityEngine; public class PlayerController : MonoBehaviour { public float speed = 5f; public int score = 0; private Rigidbody2D rb; private void Start() { rb = GetComponent<Rigidbody2D>(); } private void Update() { float horizontalInput = Input.GetAxis("Horizontal"); float verticalInput = Input.GetAxis("Vertical"); Vector2 movement = new Vector2(horizontalInput, verticalInput); rb.velocity = movement * speed; } private void OnTriggerEnter2D(Collider2D other) { if (other.CompareTag("Coin")) { Destroy(other.gameObject); score++; } } }
In this example, the PlayerController
script defines the behavior of the player character. It uses the Rigidbody2D
component to add physics simulation to the character, allowing it to move and collide with other objects. It also handles user input and collision detection with coins for scoring.
By abstracting away low-level details of physics simulation, rendering, and input handling, Unity allows developers to focus on the high-level logic and design of their games. This promotes code reusability, productivity, and enables the creation of complex and immersive gaming experiences.
Related Article: Comparing GraphQL and Elasticsearch
Performance Consideration: Abstraction and Memory Management
While abstraction provides numerous benefits in software development, it is important to consider its impact on performance, especially in resource-intensive applications.
Abstraction introduces an additional layer of indirection and can result in increased memory usage. For example, when using abstract classes or interfaces, there is often a need to create additional objects to represent the concrete implementations.
In memory-constrained environments or performance-critical applications, excessive abstraction and object creation can lead to increased memory pressure and slower execution times. It is important to strike the right balance between abstraction and performance optimization.
Optimizing abstraction-related performance issues can involve techniques such as:
- Object Pooling: Reusing objects instead of creating new ones can help reduce memory allocation overhead.
- Minimizing Indirection: Reducing unnecessary layers of abstraction and minimizing the number of method calls can improve performance.
- Data-Oriented Design: Designing code and data structures with a focus on cache efficiency can improve memory access patterns and reduce overhead.
- Profiling and Benchmarking: Profiling tools can help identify performance bottlenecks and guide optimization efforts.
It is essential to consider the specific performance requirements and constraints of your application when designing and using abstractions. While abstraction is a powerful tool for code organization and maintainability, it should not be applied blindly without considering its impact on performance.
Performance Consideration: Abstraction and Processing Time
In addition to memory management considerations, abstraction can also impact processing time, especially in performance-critical applications that require high throughput or low latency.
Abstraction introduces additional layers of code execution and indirection, which can increase the time required to perform certain operations. Method calls, virtual function dispatching, and dynamic dispatching can all introduce overhead and impact performance.
To mitigate the impact of abstraction on processing time, consider the following techniques:
- Inline Optimization: Inlining frequently called methods or eliminating unnecessary method calls can reduce function call overhead.
- Compile-Time Polymorphism: Using templates or generics to resolve operations at compile time can eliminate dynamic dispatching and improve performance.
- Caching and Memoization: Caching expensive computations or results can help avoid unnecessary calculations and improve processing time.
- Algorithmic Optimization: Optimizing algorithms and data structures can have a significant impact on performance. It is often more beneficial to focus on algorithmic improvements rather than micro-optimizations at the abstraction level.
As with memory management considerations, it is crucial to profile and benchmark your application to identify performance bottlenecks and guide optimization efforts. Balancing the benefits of abstraction with the performance requirements of your application is essential to achieve the desired performance characteristics.
Advanced Technique: Abstraction and Inheritance
Inheritance is a powerful mechanism that enables code reuse and abstraction in object-oriented programming. It allows a class to inherit the properties and behaviors of another class, promoting code organization and modularity.
Abstract classes play a key role in inheritance-based abstraction. They serve as base classes that define common attributes and behaviors, while leaving specific implementation details to concrete subclasses.
Let's consider an example to illustrate the concept of abstraction through inheritance:
abstract class Animal { protected String name; public Animal(String name) { this.name = name; } public abstract void makeSound(); public void sleep() { System.out.println(name + " is sleeping"); } } class Dog extends Animal { public Dog(String name) { super(name); } @Override public void makeSound() { System.out.println(name + " says woof"); } } class Cat extends Animal { public Cat(String name) { super(name); } @Override public void makeSound() { System.out.println(name + " says meow"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog("Buddy"); dog.makeSound(); dog.sleep(); Animal cat = new Cat("Whiskers"); cat.makeSound(); cat.sleep(); } }
In this example, we have an abstract Animal
class that defines an abstract method makeSound()
and a non-abstract method sleep()
. The Dog
and Cat
classes inherit from the Animal
class and provide their own implementations of the makeSound()
method.
By using inheritance and abstraction, we can create a hierarchy of classes that represent different types of animals. The abstract Animal
class provides a common interface for all animals, and the concrete subclasses specify the details specific to each animal type.
Advanced Technique: Abstraction and Polymorphism
Polymorphism is another powerful aspect of abstraction in object-oriented programming. It allows objects of different types to be treated as instances of a common superclass or interface.
Polymorphism enables code to be written in a more generic and flexible manner, allowing for increased code reuse and adaptability. It is closely related to inheritance and abstraction.
Let's consider an example that demonstrates the concept of polymorphism:
class Shape: def area(self): pass class Rectangle(Shape): def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height class Circle(Shape): def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius * self.radius shapes = [Rectangle(5, 3), Circle(2.5)] for shape in shapes: print("Area:", shape.area())
In this example, we have a Shape
class that defines an abstract method area()
. The Rectangle
and Circle
classes inherit from the Shape
class and provide their own implementations of the area()
method.
We create a list of shapes that includes instances of both Rectangle
and Circle
. By iterating over the list and calling the area()
method on each shape, we can calculate and print the areas of different shapes without explicitly knowing their specific types.
Polymorphism allows us to write generic code that can operate on objects of different types, as long as they adhere to a common interface or superclass. This promotes code reuse and flexibility, as new classes can be easily added to the system without modifying the existing code.
Related Article: Altering Response Fields in an Elasticsearch Query
Code Snippet: Implementing Abstraction in Java
In Java, abstraction can be achieved using abstract classes and interfaces. Abstract classes provide a way to define common attributes and behaviors, while interfaces define a contract that implementing classes must adhere to.
Let's consider an example that demonstrates the implementation of abstraction in Java:
abstract class Animal { protected String name; public Animal(String name) { this.name = name; } public abstract void makeSound(); public void sleep() { System.out.println(name + " is sleeping"); } } class Dog extends Animal { public Dog(String name) { super(name); } @Override public void makeSound() { System.out.println(name + " says woof"); } } class Cat extends Animal { public Cat(String name) { super(name); } @Override public void makeSound() { System.out.println(name + " says meow"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog("Buddy"); dog.makeSound(); dog.sleep(); Animal cat = new Cat("Whiskers"); cat.makeSound(); cat.sleep(); } }
In this example, we have an abstract Animal
class that defines an abstract method makeSound()
and a non-abstract method sleep()
. The Dog
and Cat
classes inherit from the Animal
class and provide their own implementations of the makeSound()
method.
By using abstract classes and inheritance, we can create a hierarchy of classes that represent different types of animals. The abstract Animal
class provides a common interface for all animals, and the concrete subclasses specify the details specific to each animal type.
Code Snippet: Implementing Abstraction in Python
In Python, abstraction can be achieved using abstract base classes provided by the abc
module. Abstract base classes define abstract methods that must be implemented by subclasses.
Let's consider an example that demonstrates the implementation of abstraction in Python:
from abc import ABC, abstractmethod class Vehicle(ABC): @abstractmethod def start_engine(self): pass class Car(Vehicle): def start_engine(self): print("Car engine started") class Motorcycle(Vehicle): def start_engine(self): print("Motorcycle engine started") car = Car() car.start_engine() motorcycle = Motorcycle() motorcycle.start_engine()
In this example, we have an abstract base class Vehicle
that defines an abstract method start_engine()
. The Car
and Motorcycle
classes inherit from the Vehicle
class and provide their own implementations of the start_engine()
method.
By using abstract base classes and inheritance, we can create a hierarchy of classes that represent different types of vehicles. The abstract Vehicle
class provides a common interface for all vehicles, and the concrete subclasses specify the details specific to each vehicle type.
Code Snippet: Implementing Abstraction in C++
In C++, abstraction can be achieved using abstract classes and pure virtual functions. Abstract classes define pure virtual functions that must be implemented by derived classes.
Let's consider an example that demonstrates the implementation of abstraction in C++:
#include <iostream> class Shape { public: virtual double calculateArea() = 0; }; class Rectangle : public Shape { private: double length; double width; public: Rectangle(double length, double width) : length(length), width(width) {} double calculateArea() override { return length * width; } }; class Circle : public Shape { private: double radius; public: Circle(double radius) : radius(radius) {} double calculateArea() override { return 3.14 * radius * radius; } }; int main() { Shape* rectangle = new Rectangle(5, 3); std::cout << "Rectangle area: " << rectangle->calculateArea() << std::endl; Shape* circle = new Circle(2.5); std::cout << "Circle area: " << circle->calculateArea() << std::endl; delete rectangle; delete circle; return 0; }
In this example, we have an abstract class Shape
that defines a pure virtual function calculateArea()
. The Rectangle
and Circle
classes inherit from the Shape
class and provide their own implementations of the calculateArea()
function.
By using abstract classes and inheritance, we can create a hierarchy of classes that represent different shapes. The abstract Shape
class provides a common interface for all shapes, and the concrete subclasses specify the details specific to each shape.
Code Snippet: Implementing Abstraction in JavaScript
In JavaScript, abstraction can be achieved using constructor functions or classes and prototypal inheritance. Constructor functions define shared properties and methods using the prototype
property.
Let's consider an example that demonstrates the implementation of abstraction in JavaScript:
function Animal(name) { this.name = name; } Animal.prototype.makeSound = function() { throw new Error('makeSound() must be implemented'); }; Animal.prototype.sleep = function() { console.log(this.name + ' is sleeping'); }; function Dog(name) { Animal.call(this, name); } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; Dog.prototype.makeSound = function() { console.log(this.name + ' says woof'); }; function Cat(name) { Animal.call(this, name); } Cat.prototype = Object.create(Animal.prototype); Cat.prototype.constructor = Cat; Cat.prototype.makeSound = function() { console.log(this.name + ' says meow'); }; const dog = new Dog('Buddy'); dog.makeSound(); dog.sleep(); const cat = new Cat('Whiskers'); cat.makeSound(); cat.sleep();
In this example, we have a constructor function Animal
that defines shared properties and methods using the prototype
object. The Dog
and Cat
constructor functions inherit from the Animal
constructor function using Object.create()
and set their own prototype
objects. They provide their own implementations of the makeSound()
method.
By using constructor functions and prototypal inheritance, we can create a hierarchy of objects that represent different types of animals. The Animal
constructor function provides a common interface for all animals, and the Dog
and Cat
constructor functions specify the details specific to each animal type.
Related Article: OAuth 2 Tutorial: Introduction & Basics
Code Snippet: Implementing Abstraction in C#
In C#, abstraction can be achieved using abstract classes and interfaces. Abstract classes provide a way to define common attributes and behaviors, while interfaces define a contract that implementing classes must adhere to.
Let's consider an example that demonstrates the implementation of abstraction in C#:
using System; abstract class Animal { protected string name; public Animal(string name) { this.name = name; } public abstract void MakeSound(); public void Sleep() { Console.WriteLine(name + " is sleeping"); } } class Dog : Animal { public Dog(string name) : base(name) { } public override void MakeSound() { Console.WriteLine(name + " says woof"); } } class Cat : Animal { public Cat(string name) : base(name) { } public override void MakeSound() { Console.WriteLine(name + " says meow"); } } class Program { static void Main(string[] args) { Animal dog = new Dog("Buddy"); dog.MakeSound(); dog.Sleep(); Animal cat = new Cat("Whiskers"); cat.MakeSound(); cat.Sleep(); } }
In this example, we have an abstract class Animal
that defines an abstract method MakeSound()
and a non-abstract method Sleep()
. The Dog
and Cat
classes inherit from the Animal
class and provide their own implementations of the MakeSound()
method.
By using abstract classes and inheritance, we can create a hierarchy of classes that represent different types of animals. The abstract Animal
class provides a common interface for all animals, and the concrete subclasses specify the details specific to each animal type.
Error Handling and Abstraction
When working with abstractions, error handling can be challenging due to the separation of concerns and the lack of direct control over the concrete implementation. However, there are several strategies that can be employed to handle errors effectively in an abstracted environment.
1. Exception Propagation: One way to handle errors is to propagate exceptions up the call stack. When a method encounters an error, it can throw an exception that is caught by a higher-level component responsible for error handling. This allows for centralized error handling and separation of concerns.
2. Error Codes or Status Flags: Another approach is to use error codes or status flags to indicate the occurrence of an error. Methods can return a special value or set a flag to indicate the success or failure of the operation. The calling code can then check the status and take appropriate action.
3. Error Events or Callbacks: In event-driven systems, error events or callbacks can be used to notify listeners or subscribers about errors. Components can register listeners or provide callback functions to handle specific types of errors. This approach allows for asynchronous error handling and decoupling of error handling logic.
4. Error Handling Frameworks: Many programming languages and frameworks provide error handling mechanisms and frameworks that can be leveraged to handle errors in an abstracted environment. These frameworks often provide features such as exception handling, error logging, and error recovery strategies.
It is important to choose an error handling strategy that aligns with the abstraction level and requirements of your application. Proper error handling ensures that errors are handled gracefully, promotes robustness, and improves the overall quality of the software.
References
- Java Tutorials: Abstract Methods and Classes
- Python Documentation: abc - Abstract Base Classes
- C++ Reference: Abstract classes and pure virtual functions