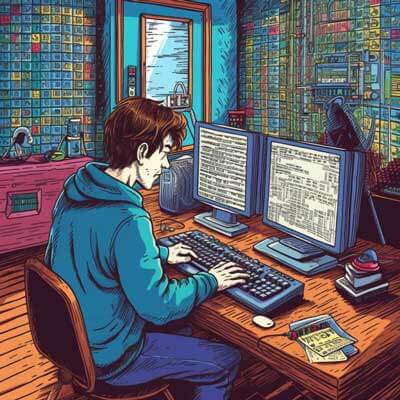
Introduction to SOLID Principles
SOLID is an acronym that stands for five principles of object-oriented design that promote software design that is easy to understand, maintain, and extend. These principles were coined by Robert C. Martin (also known as Uncle Bob) and have become fundamental concepts in software engineering.
In this chapter, we will provide an overview of each of the SOLID principles and explain their importance in object-oriented design.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Single Responsibility Principle: Detailed Overview
The Single Responsibility Principle (SRP) states that a class should have only one reason to change. In other words, a class should have only one responsibility or job.
By adhering to the SRP, we can ensure that our classes are focused and have a clear purpose. This leads to better maintainability, testability, and reusability of code.
Let’s consider an example to understand how to apply the SRP. Suppose we have a User
class that is responsible for both user authentication and user profile management. This violates the SRP because the class has multiple responsibilities.
To adhere to the SRP, we can split the User
class into two separate classes: AuthenticationService
and UserProfileService
. The AuthenticationService
class will handle user authentication, while the UserProfileService
class will handle user profile management.
Here’s an example of how the code might look:
public class AuthenticationService { public boolean authenticateUser(String username, String password) { // Code for user authentication } } public class UserProfileService { public void updateUserProfile(User user) { // Code for updating user profile } }
By separating the responsibilities, we make the code easier to understand, maintain, and test. If we need to modify the user authentication logic, we only need to make changes in the AuthenticationService
class, without affecting the UserProfileService
class.
Open-Closed Principle: Detailed Overview
The Open-Closed Principle (OCP) states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. In other words, we should be able to add new functionality without modifying existing code.
Let’s consider an example to understand how to apply the OCP. Suppose we have a Shape
class hierarchy that includes different types of shapes such as Rectangle
and Circle
. We want to calculate the area of each shape.
Instead of modifying the existing Shape
class whenever a new shape is added, we can use inheritance and create a new subclass for each shape. Each subclass will implement the calculateArea()
method according to its specific shape.
Here’s an example of how the code might look:
class Shape: def calculateArea(self): pass class Rectangle(Shape): def calculateArea(self): # Code for calculating the area of a rectangle class Circle(Shape): def calculateArea(self): # Code for calculating the area of a circle
Liskov Substitution Principle: Detailed Overview
The Liskov Substitution Principle (LSP) states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
In simpler terms, if we have a base class and multiple subclasses, we should be able to use any subclass wherever the base class is expected, without causing any unexpected behavior or violating the program’s contract.
To understand the LSP, let’s consider an example involving a Bird
base class and two subclasses, Duck
and Ostrich
. The Bird
class has a fly()
method, indicating that all birds can fly.
However, the Ostrich
class is unable to fly. If we try to substitute an instance of Ostrich
for a Bird
in a context that expects a flying ability, it would violate the LSP.
To adhere to the LSP, we can introduce an abstraction called FlyingBird
that extends the Bird
class and includes the fly()
method. The Duck
class can directly inherit from the FlyingBird
class, while the Ostrich
class can remain a subclass of Bird
without the fly()
method.
Here’s an example of how the code might look:
class Bird { // Common behavior for all birds } class FlyingBird extends Bird { public void fly() { // Code for flying behavior } } class Duck extends FlyingBird { // Additional behavior specific to ducks } class Ostrich extends Bird { // Additional behavior specific to ostriches }
By adhering to the LSP, we ensure that the code is more maintainable and extensible. It allows us to substitute objects of different subclasses without worrying about unexpected behavior or breaking the existing code.
Related Article: 16 Amazing Python Libraries You Can Use Now
Interface Segregation Principle: Detailed Overview
The Interface Segregation Principle (ISP) states that clients should not be forced to depend on interfaces they do not use. In other words, we should design fine-grained interfaces that are specific to the needs of the clients that use them.
Let’s consider an example to understand how to apply the ISP. Suppose we have an Animal
interface that includes methods such as eat()
, sleep()
, and fly()
. However, not all animals can fly.
To adhere to the ISP, we can split the Animal
interface into separate interfaces based on the specific behaviors. For example, we can create an IFlyable
interface that includes the fly()
method, and have only the relevant classes implement this interface.
Here’s an example of how the code might look:
interface IAnimal { eat(): void; sleep(): void; } interface IFlyable { fly(): void; } class Bird implements IAnimal, IFlyable { eat(): void { // Code for eating behavior } sleep(): void { // Code for sleeping behavior } fly(): void { // Code for flying behavior } } class Dog implements IAnimal { eat(): void { // Code for eating behavior } sleep(): void { // Code for sleeping behavior } }
By adhering to the ISP, we ensure that clients only depend on the specific interfaces they need. This reduces coupling and allows for more maintainable and flexible code.
Dependency Inversion Principle: Detailed Overview
The Dependency Inversion Principle (DIP) states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
In simpler terms, this principle promotes the use of interfaces or abstract classes to define the dependencies between modules. It allows for flexibility and easier testing by decoupling the high-level and low-level modules.
To understand the DIP, let’s consider an example involving a User
class that needs to send notifications to users. Instead of directly depending on a specific notification implementation, we can define an interface for the notification and have the User
class depend on the interface.
Here’s an example of how the code might look:
interface INotificationService { void sendNotification(User user, string message); } class EmailNotificationService : INotificationService { public void sendNotification(User user, string message) { // Code for sending email notification } } class SMSNotificationService : INotificationService { public void sendNotification(User user, string message) { // Code for sending SMS notification } } class User { private INotificationService notificationService; public User(INotificationService notificationService) { this.notificationService = notificationService; } public void sendMessage(string message) { // Code for sending message using the notification service notificationService.sendNotification(this, message); } }
By adhering to the DIP, we ensure that the User
class depends on an abstraction (INotificationService
) rather than a specific implementation. This allows for easy swapping of different notification services without modifying the User
class. It also enables easier testing by providing the ability to mock the notification service interface.
These are the five SOLID principles that provide guidelines for designing object-oriented systems that are flexible, maintainable, and extensible. In the next chapters, we will dive deeper into each principle and explore real-world examples, best practices, and performance considerations.