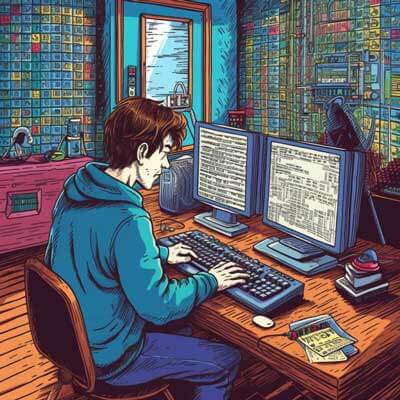
To use a regular expression (regex) to only accept numbers 0-9, you can follow these steps:
Step 1: Understand the Problem
Before diving into the solution, it’s important to understand why someone might want to use a regex to only accept numbers 0-9. There could be several reasons, including:
– Validating user input: If you have a form or an input field where you expect the user to enter a numeric value, you can use a regex to ensure that only numbers within a specific range (0-9 in this case) are accepted.
– Data cleaning or filtering: If you have a dataset that contains alphanumeric or non-numeric characters, you can use a regex to filter out or clean up the data, leaving only the numeric values.
Related Article: How to Implement a Beating Heart Loader in Pure CSS
Step 2: Define the Regex Pattern
To only accept numbers 0-9 using a regex, you can use the following pattern:
^[0-9]+$
Explanation of the pattern:
– ^
asserts the start of the string.
– [0-9]
matches any digit between 0 and 9.
– +
specifies that the previous character (the digit) can appear one or more times.
– $
asserts the end of the string.
This pattern ensures that the entire string consists of one or more digits from 0 to 9.
Step 3: Implement the Regex in Your Code
Now that you have the regex pattern, you can implement it in your code depending on the programming language or context you’re working with. Here are a few examples:
JavaScript:
const regex = /^[0-9]+$/; const input = "12345"; if (regex.test(input)) { console.log("Input is a valid number."); } else { console.log("Input is not a valid number."); }
Python:
import re regex = r"^[0-9]+$" input = "12345" if re.match(regex, input): print("Input is a valid number.") else: print("Input is not a valid number.")
Java:
import java.util.regex.Pattern; import java.util.regex.Matcher; public class RegexExample { public static void main(String[] args) { String regex = "^[0-9]+$"; String input = "12345"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(input); if (matcher.matches()) { System.out.println("Input is a valid number."); } else { System.out.println("Input is not a valid number."); } } }
These examples show how to use the regex pattern to validate a given input string and determine if it consists of only numbers 0-9.
Step 4: Additional Suggestions and Best Practices
– Anchoring the regex pattern with ^
and $
ensures that the entire string is matched from start to end. This prevents partial matches from being considered valid.
– If you want to allow an optional leading or trailing whitespace, you can modify the pattern to include \s*
before and after the digit range. For example, the pattern ^\s*[0-9]+\s*$
would allow whitespace before and after the number.
– If you want to limit the number of digits, you can use quantifiers. For example, the pattern ^[0-9]{2,4}$
would match numbers with 2 to 4 digits.
– Keep in mind that different programming languages or regex engines may have slight variations in syntax or behavior when it comes to regular expressions. Refer to the documentation of the specific language or tool you are using for more information.
Related Article: 7 Shared Traits of Ineffective Engineering Teams