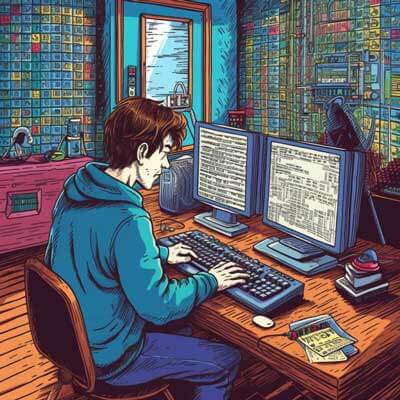
The regex not operator, also known as the negation operator, is a useful tool in programming for pattern matching and string manipulation. It allows you to match strings that do not meet a specific pattern. This can be useful in various scenarios, such as data validation, filtering, and text processing. In this article, we will explore how to use the regex not operator effectively in different programming languages.
The Basics of Regular Expressions
Before diving into the specifics of the regex not operator, it is essential to have a basic understanding of regular expressions. Regular expressions, often referred to as regex or regexp, are a sequence of characters used to define a search pattern. They are widely used in programming languages, text editors, and command-line tools for tasks like pattern matching and data extraction.
A regular expression can contain a combination of literal characters and special characters called metacharacters. Metacharacters have a special meaning in regular expressions and allow you to define more complex patterns. Some common metacharacters include:
– .
: Matches any single character except a newline.
– *
: Matches zero or more occurrences of the preceding character or group.
– +
: Matches one or more occurrences of the preceding character or group.
– ?
: Matches zero or one occurrence of the preceding character or group.
– [ ]
: Matches any single character within the brackets.
– ( )
: Groups characters together and creates a capture group.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Using the Negation Operator
The negation operator in regular expressions allows you to match patterns that do not meet a specific condition. It is represented by the ^
metacharacter when used inside a character class. A character class is defined by enclosing a set of characters within square brackets, such as [abc]
, which matches either “a”, “b”, or “c”.
To use the negation operator, you place the ^
metacharacter immediately after the opening square bracket. For example, [^abc]
matches any character that is not “a”, “b”, or “c”. Here are a few examples to illustrate how the negation operator works:
– [^0-9]
: Matches any character that is not a digit.
– [^A-Za-z]
: Matches any character that is not a letter.
– [^aeiou]
: Matches any character that is not a vowel.
It is important to note that the negation operator only works inside a character class. If you place the ^
metacharacter outside a character class, it has a different meaning, such as matching the beginning of a line.
Examples in Different Programming Languages
Now let’s see how the negation operator can be used in different programming languages.
1. Python:
In Python, you can use the re
module to work with regular expressions. Here’s an example that demonstrates the use of the negation operator in Python:
import re text = "The quick brown fox jumps over the lazy dog" matches = re.findall("[^aeiou]", text) print(matches) # Output: ['T', 'h', ' ', 'q', 'c', 'k', ' ', 'b', 'r', 'w', 'n', ' ', 'f', 'x', ' ', 'j', 'm', 'p', 's', ' ', 'v', 'r', ' ', 't', 'h', ' ', 'l', 'z', 'y', ' ', 'd', 'g']
This example uses the re.findall()
function to find all occurrences of characters that are not vowels in the given text. The resulting list contains all the matching characters.
2. JavaScript:
In JavaScript, you can use the built-in regular expression support to work with regular expressions. Here’s an example that demonstrates the use of the negation operator in JavaScript:
const text = "The quick brown fox jumps over the lazy dog"; const matches = text.match(/[^aeiou]/g); console.log(matches); // Output: ['T', 'h', ' ', 'q', 'c', 'k', ' ', 'b', 'r', 'w', 'n', ' ', 'f', 'x', ' ', 'j', 'm', 'p', 's', ' ', 'v', 'r', ' ', 't', 'h', ' ', 'l', 'z', 'y', ' ', 'd', 'g']
This example uses the match()
method with a regular expression /[^aeiou]/g
to find all occurrences of characters that are not vowels in the given text. The resulting array contains all the matching characters.
Best Practices and Tips
Here are some best practices and tips to keep in mind when using the regex not operator:
1. Be aware of case sensitivity: Regular expressions are case-sensitive by default. If you want to perform a case-insensitive search, you can use the i
flag, such as /[^aeiou]/gi
.
2. Escape special characters: If you want to match a literal metacharacter, you need to escape it with a backslash. For example, /[^\.]/
matches any character that is not a period.
3. Use character classes wisely: Character classes are useful, but they can also become complex and hard to read. Use them judiciously and consider using alternatives like negative lookaheads or lookbehinds when appropriate.
4. Test and validate your regular expressions: Regular expressions can be tricky, so it’s important to test and validate them with different inputs to ensure they produce the desired results.
5. Use online regex tools: Online regex tools like regex101.com or regexr.com can be helpful for testing and debugging your regular expressions.
Related Article: 16 Amazing Python Libraries You Can Use Now