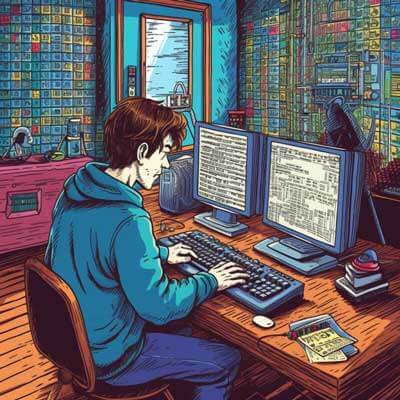
Table of Contents
Getting Started with Docker
Docker is an open-source platform that automates the deployment and management of applications inside software containers. It allows developers to package an application and all its dependencies into a standardized unit, called a container, which can be run on any operating system that supports Docker.
In this chapter, we will cover the basics of getting started with Docker. We will discuss the workdir
command, the run
command, and environment variables.
Related Article: How to Install and Use Docker
The Workdir Command
The workdir
command is used to set the working directory for the instructions that follow it in the Dockerfile. It is similar to the cd
command in a shell script. By default, Docker sets the working directory to the root directory ("/").
Here's an example of how to use the workdir
command in a Dockerfile:
FROM ubuntu WORKDIR /app COPY . .
In this example, the workdir
command sets the working directory to "/app". The copy
command will copy the contents of the current directory into the "/app" directory inside the container.
The Run Command
The run
command is used to run a command inside a container. It is one of the most commonly used commands in Docker. You can use the run
command to start a new container from an image, execute a command in a running container, or run a command in a new container and then exit.
Here's an example of how to use the run
command to start a new container from an image:
docker run -it ubuntu bash
This command will start a new container from the "ubuntu" image and run the "bash" command inside the container. The -it
flag is used to allocate a pseudo-TTY and keep the session open for interactive shell access.
Environment Variables
Environment variables are a way to pass configuration information to running containers. They are used to store values that can be accessed by processes inside the container.
You can set environment variables in Docker using the -e
flag when running the docker run
command. Here's an example:
docker run -e MY_VARIABLE=my_value ubuntu bash
In this example, the -e
flag is used to set the environment variable "MY_VARIABLE" with the value "my_value". The "ubuntu" image is used, and the "bash" command is run inside the container.
Environment variables can also be set in a Dockerfile using the env
command. Here's an example:
FROM ubuntu ENV MY_VARIABLE=my_value
In this example, the env
command sets the environment variable "MY_VARIABLE" with the value "my_value" inside the Dockerfile.
With the workdir
command, the run
command, and environment variables, you now have a solid foundation to get started with Docker. These concepts will be used extensively throughout your Docker journey.
Related Article: How to Push Changes to a Remote Repository with Git Push
Understanding the Workdir Command
The WORKDIR
command in Docker is used to set the working directory for instructions such as RUN
, CMD
, ENTRYPOINT
, COPY
, and ADD
in the Dockerfile. It allows you to specify the directory from which relative paths are evaluated.
By default, the working directory in a Docker container is set to /
. However, using the WORKDIR
command, you can change it to any directory you want.
Here's an example of how you can use the WORKDIR
command in a Dockerfile:
FROM alpine:latest
WORKDIR /app
COPY . .
RUN npm install
CMD ["npm", "start"]
In this example, we set the working directory to /app
using the WORKDIR
command. The COPY
instruction then copies the contents of the current directory into the /app
directory of the container. The RUN
command installs the required dependencies, and the CMD
command starts the application.
Using the WORKDIR
command has several benefits. Firstly, it makes your Dockerfile more readable by providing a clear and explicit path for subsequent instructions. Secondly, it simplifies the use of relative paths, as all paths specified in subsequent instructions will be relative to the WORKDIR
directory.
It's worth noting that the WORKDIR
command does not actually create the directory in the container. If the directory specified by WORKDIR
does not exist, Docker will create it when necessary. However, if the directory does exist, Docker will use it as the working directory.
To verify the working directory in a running Docker container, you can use the PWD
command inside the container's shell. You will see that the working directory is set to the one specified by the WORKDIR
command.
In conclusion, the WORKDIR
command is a useful tool in Docker to set the working directory for subsequent instructions. It helps improve the readability of your Dockerfile and simplifies the use of relative paths.
Setting the Workdir in Docker
When working with Docker, it's important to understand how to set the working directory (workdir) for your containers. The working directory is the location inside the container where your application will run and any files or directories that are created will be stored.
By default, Docker sets the workdir to the root directory ("/") of the container. However, you can change this to any directory of your choice using the WORKDIR
instruction in your Dockerfile.
The WORKDIR
instruction sets the working directory for any subsequent instructions in the Dockerfile. This means that any following RUN
, COPY
, or ADD
commands will be executed in the specified working directory.
Here's an example of how you can use the WORKDIR
instruction in a Dockerfile:
Dockerfile
:
FROM ubuntu:latest WORKDIR /app COPY . . RUN npm install CMD ["npm", "start"]
In this example, we set the working directory to "/app" using the WORKDIR
instruction. Then, we copy the current directory (where the Dockerfile is located) into the container's "/app" directory. After that, we run the npm install
command in the "/app" directory to install the necessary dependencies. Finally, we set the default command to run our application using npm start
.
Setting the working directory is especially useful when you have a complex project structure with multiple directories and files. By setting the working directory, you can simplify the paths of subsequent commands, making them more readable and less error-prone.
It's also worth noting that the WORKDIR
instruction can be used multiple times in a Dockerfile. Each subsequent use of WORKDIR
will change the working directory for the following instructions.
In conclusion, setting the workdir in Docker is a powerful technique to organize and simplify your containerized applications. It allows you to specify the location where your application will run and manage files and directories within the container.
Exploring the Run Command
The docker run
command is one of the most frequently used commands when working with Docker containers. It allows you to create and start a container from a Docker image. In this section, we will explore various options and use cases for the docker run
command.
Basic Usage
The basic syntax for the docker run
command is as follows:
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Here, OPTIONS
are the various flags that you can use to customize the behavior of the container, IMAGE
is the name of the Docker image to use, COMMAND
is the command to run inside the container, and ARG
are the arguments to pass to the command.
For example, to run a container based on the ubuntu
image and execute the echo
command inside it, you can use the following command:
docker run ubuntu echo "Hello, Docker!"
This will create a new container based on the ubuntu
image and execute the echo
command, which will print "Hello, Docker!" to the console.
Related Article: Tutorial on Database Sharding in MySQL
Detached Mode
By default, the docker run
command runs containers in the foreground, which means that the container's output is displayed in the console. However, you can also run containers in detached mode by using the -d
or --detach
flag. This allows the container to run in the background without blocking the console.
For example, to run a container based on the nginx
image in detached mode, you can use the following command:
docker run -d nginx
This will start a new container based on the nginx
image and detach it from the console. You can use the docker ps
command to see the running containers.
Port Mapping
Another useful feature of the docker run
command is the ability to map ports between the host and the container. This is done using the -p
or --publish
flag followed by the host port and the container port.
For example, to run a container based on the nginx
image and map port 80 of the container to port 8080 on the host, you can use the following command:
docker run -p 8080:80 nginx
This will start a new container based on the nginx
image and map port 80 of the container to port 8080 on the host. You can then access the web server running inside the container by opening a browser and navigating to http://localhost:8080
.
Environment Variables
You can also pass environment variables to the container using the -e
or --env
flag. This is useful for configuring the container at runtime.
For example, to set the MYSQL_ROOT_PASSWORD
environment variable to "secretpassword" when running a container based on the mysql
image, you can use the following command:
docker run -e MYSQL_ROOT_PASSWORD=secretpassword mysql
This will start a new container based on the mysql
image and set the MYSQL_ROOT_PASSWORD
environment variable to "secretpassword".
Running Docker Containers
Once you have built a Docker image, the next step is to run a container based on that image. Running a container allows you to start an instance of the image and execute commands or run applications within it. In this chapter, we will explore different ways to run Docker containers.
1. Running a Container with the Docker Run Command
The most common way to run a Docker container is by using the docker run
command. This command creates and starts a new container from a specified image.
Here's the basic syntax:
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
For example, to run a container based on the official Ubuntu image and execute the echo
command, you can use the following command:
docker run ubuntu echo "Hello, Docker!"
This will create a new container based on the ubuntu
image and execute the echo
command within it. The output, "Hello, Docker!", will be displayed in the terminal.
2. Specifying the Working Directory
By default, the working directory inside a Docker container is the root directory (/
). However, you can specify a different working directory using the -w
or --workdir
option.
Here's an example:
docker run -w /app myimage
This command sets the working directory inside the container to /app
. Any subsequent commands or file paths will be relative to this directory.
3. Setting Environment Variables
Environment variables are a great way to configure applications running inside Docker containers. You can set environment variables using the -e
or --env
option.
Here's an example:
docker run -e MY_VAR=myvalue myimage
This command sets the environment variable MY_VAR
to myvalue
inside the container. Applications running in the container can access this variable and use it as needed.
4. Running a Container in the Background
By default, when you run a container, it runs in the foreground and attaches to your terminal. If you want to run a container in the background, you can use the -d
or --detach
option.
Here's an example:
docker run -d myimage
This command starts the container in the background and returns the container ID. You can use this ID to manage the container later.
These are just a few examples of how to run Docker containers. There are many more options and configurations available. You can explore the official Docker documentation for more information and advanced usage.
Next, we will delve into the topic of managing Docker containers and explore different commands to interact with running containers.
Related Article: Build a Movie Search App with GraphQL, Node & TypeScript
Using Environment Variables in Docker
In Docker, environment variables are a useful way to pass configuration information to your containers. They can be set at runtime and accessed by the application running inside the container. This allows for greater flexibility and easier deployment of your Dockerized applications.
To set an environment variable in a Docker container, you can use the -e
flag followed by the variable name and its value when running the docker run
command. For example, to set the DB_HOST
variable to localhost
, you would run:
docker run -e DB_HOST=localhost my_image
You can also use a file to set multiple environment variables. Create a file, let's say env_vars.txt
, with each variable on a separate line in the format VAR_NAME=value
. Then, use the --env-file
flag followed by the path to the file when running the docker run
command. For example:
docker run --env-file env_vars.txt my_image
Inside the container, you can access these environment variables in your application code just like any other environment variable. The exact method of accessing environment variables depends on the programming language and framework you are using.
For example, in Node.js, you can access environment variables using process.env
. To access the value of the DB_HOST
variable set earlier, you would use:
const dbHost = process.env.DB_HOST;
In Python, you can access environment variables using the os
module. To access the value of the DB_HOST
variable, you would use:
import os db_host = os.environ.get('DB_HOST')
Environment variables can also be used to store sensitive information such as passwords or API keys. However, it is important to handle these variables with care and not expose them in your codebase or Docker image.
To better manage environment variables, you can use a tool like Docker Compose. With Docker Compose, you can define environment variables in a separate .env
file and have them automatically loaded into your containers. This simplifies the management of multiple variables and makes it easier to share and version control your configuration.
In conclusion, environment variables are a powerful feature in Docker that allow you to configure your containers in a flexible and dynamic way. They can be set at runtime, accessed by your application code, and used to store sensitive information. By leveraging environment variables, you can make your Dockerized applications more configurable and easier to deploy.
Passing Environment Variables to Containers
When working with Docker, you often need to pass environment variables to your containers. Environment variables are useful for providing configuration values, secrets, or any other dynamic data that your application needs.
There are multiple ways to pass environment variables to Docker containers, and we will explore some of the most common methods.
1. Using the -e flag with the docker run command
One straightforward way to pass environment variables to a container is by using the -e
flag with the docker run
command. You can specify one or more environment variables using the syntax -e VARIABLE_NAME=VALUE
.
Here's an example:
docker run -e MY_VARIABLE=my_value my_image
In this example, the container will have an environment variable called MY_VARIABLE
with the value my_value
.
2. Using an environment file
Another approach is to use an environment file to define multiple environment variables at once. You can create a file with a list of variable-value pairs, with each pair separated by an equal sign.
For example, create a file named env.list
with the following contents:
VARIABLE1=value1 VARIABLE2=value2
Then, you can pass this file to Docker using the --env-file
flag:
docker run --env-file env.list my_image
In this case, the container will have two environment variables: VARIABLE1
with the value value1
and VARIABLE2
with the value value2
.
3. Defining environment variables in Dockerfile
You can also define environment variables directly in your Dockerfile using the ENV
instruction. This way, the variables will be set when the image is built and available in all containers created from that image.
Here's an example of how to define an environment variable in a Dockerfile:
FROM my_base_image ENV MY_VARIABLE=my_value
In this example, the MY_VARIABLE
environment variable will be set to my_value
for all containers created from this image.
4. Using Docker Compose
If you are using Docker Compose to manage your containers, you can define environment variables in your docker-compose.yml
file.
Here's an example:
version: '3' services: my_service: image: my_image environment: - VARIABLE1=value1 - VARIABLE2=value2
In this example, the my_service
container will have two environment variables: VARIABLE1
with the value value1
and VARIABLE2
with the value value2
.
These are just a few examples of how you can pass environment variables to Docker containers. Depending on your use case, you may find other methods more suitable. Experiment with different approaches to find the one that works best for you.
Remember that environment variables can contain sensitive information, so make sure to handle them securely and avoid exposing them unintentionally.
Now that you know how to pass environment variables to containers, let's move on to the next chapter where we will explore the Workdir command in Docker.
Using Environment Variables in Docker Compose
Docker Compose is a powerful tool that allows you to define and manage multi-container Docker applications. One of the key features of Docker Compose is its support for environment variables. Environment variables are a convenient way to pass configuration information to your Docker containers.
When using Docker Compose, you can define environment variables in your docker-compose.yml
file. These variables can then be used within your container's configuration. Here's an example of how you can define environment variables in Docker Compose:
version: '3' services: myapp: image: myapp:latest environment: - DATABASE_HOST=db - DATABASE_USER=myuser - DATABASE_PASSWORD=mypassword
In this example, we define three environment variables: DATABASE_HOST
, DATABASE_USER
, and DATABASE_PASSWORD
. These variables can be accessed within the myapp
service container using the usual environment variable syntax, for example, $DATABASE_HOST
.
You can also use environment variables in other parts of your Docker Compose configuration, such as defining volumes or network configuration. This allows you to easily customize the behavior of your containers without modifying the underlying Docker configuration files.
Environment variables in Docker Compose can be set in multiple ways. The most common way is to define them directly in the docker-compose.yml
file, as shown in the previous example. However, you can also use external files to define your environment variables.
For example, you can create a file named .env
in the same directory as your docker-compose.yml
file, and define your environment variables there:
DATABASE_HOST=db DATABASE_USER=myuser DATABASE_PASSWORD=mypassword
Then, in your docker-compose.yml
file, you can reference these variables using the ${}
syntax:
version: '3' services: myapp: image: myapp:latest env_file: - .env
By using an external file, you can easily manage your environment variables in a separate file, which can be useful when working with multiple Docker Compose configurations or when you need to keep sensitive information out of your version control system.
Using environment variables in Docker Compose allows you to create more flexible and customizable Docker applications. They provide a convenient way to pass configuration information to your containers and make it easier to manage your application's behavior across different environments.
In the next section, we will explore another useful feature of Docker: the ability to mount volumes. Stay tuned!
Best Practices for Working with Environment Variables
When working with Docker, environment variables can be a powerful tool to configure and control your containers. They allow you to set dynamic values that can be used by your application or scripts inside the container. However, managing environment variables in a Docker environment can be challenging if not done properly. In this chapter, we will discuss some best practices for working with environment variables in Docker.
Related Article: How to Force Docker for a Clean Build of an Image
1. Use the -e flag to set environment variables
When running a Docker container, you can use the -e flag followed by the variable name and its value to set an environment variable. For example:
docker run -e MY_VARIABLE=my_value my_image
This sets the environment variable MY_VARIABLE to the value my_value inside the container. Using the -e flag is the recommended way to set environment variables when running containers.
2. Use the --env-file flag to load environment variables from a file
If you have a lot of environment variables to set, it can become cumbersome to pass them all using the -e flag. Instead, you can use the --env-file flag followed by the path to a file containing the environment variables. For example:
docker run --env-file=my_env_file my_image
The file my_env_file should contain the environment variables in the format VAR_NAME=VAR_VALUE, with each variable on a new line. This allows you to manage your environment variables separately in a file.
3. Use a .env file for local development
During local development, you may have different environment variables specific to your development environment. Instead of passing them using the -e flag or --env-file flag every time you run a container, you can use a .env file. This file should be located in the same directory as your docker-compose.yml file and should contain the environment variables in the same format as the --env-file flag.
For example, your .env file might look like this:
DB_HOST=localhost DB_PORT=5432
Then, in your docker-compose.yml file, you can use the env_file option to load the environment variables from the .env file:
services: my_service: ... env_file: - .env
This allows you to keep your environment variables separate from your code and easily manage them during local development.
4. Avoid hard-coding sensitive information in environment variables
It's important to avoid hard-coding sensitive information, such as passwords or API keys, directly in your Dockerfile or environment variables. Instead, consider using a secrets management solution, such as Docker's built-in Docker Secrets or third-party solutions like HashiCorp Vault. These solutions provide a more secure way to manage and distribute sensitive information to your containers.
Related Article: How to Improve Docker Container Performance
5. Use default values for environment variables
To make your containers more flexible, consider using default values for environment variables. This allows your container to run even if a specific environment variable is not set. You can use the syntax ${VARIABLE_NAME:-DEFAULT_VALUE} to provide a default value for an environment variable.
For example:
ENV MY_VARIABLE=${MY_VARIABLE:-default_value}
If the MY_VARIABLE environment variable is not set, it will default to default_value. This ensures that your container can still function even if certain environment variables are not provided.
By following these best practices, you can effectively manage and work with environment variables in Docker, making your containers more configurable and secure.
Real World Examples of Docker Environment Variables
Environment variables are an essential feature of Docker containers, allowing you to customize the behavior of your applications without modifying the code. In this section, we will explore some real-world examples of how you can use environment variables in Docker.
Example 1: Configuring Database Connection
One common use case for environment variables is configuring the database connection for your application. Suppose you have a Node.js application that connects to a PostgreSQL database. Instead of hardcoding the database connection details in your code, you can use environment variables to pass them dynamically.
Here's an example of how you can achieve this in a Dockerfile:
FROM node:14 ENV DB_HOST=localhost ENV DB_PORT=5432 ENV DB_USER=myuser ENV DB_PASSWORD=mypassword ENV DB_NAME=mydb # Rest of the Dockerfile
In the above example, we set the environment variables DB_HOST
, DB_PORT
, DB_USER
, DB_PASSWORD
, and DB_NAME
to their respective values. These values can then be accessed within your application code, allowing you to connect to the database without hardcoding the connection details.
Example 2: Configuring API Keys
Another common use case is configuring API keys or other sensitive information. For instance, if your application interacts with third-party APIs that require an API key, you can use environment variables to securely store and pass the key to your application.
Here's an example of how you can use environment variables to configure an API key in a Dockerfile:
FROM node:14 ENV API_KEY=your-api-key # Rest of the Dockerfile
By setting the environment variable API_KEY
to your actual API key, you can access it within your application code. This approach allows you to keep your API key separate from your codebase, reducing the risk of accidental exposure.
Example 3: Controlling Application Behavior
Environment variables can also be used to control the behavior of your application. For example, you might want to enable or disable certain features based on an environment variable's value.
Here's an example of how you can use an environment variable to control a feature in a Dockerfile:
FROM node:14 ENV ENABLE_FEATURE=true # Rest of the Dockerfile
In this example, we set the environment variable ENABLE_FEATURE
to true
. Within your application code, you can check the value of this variable and enable or disable the corresponding feature accordingly.
Example 4: Injecting Configuration Files
You can also use environment variables to inject configuration files into your Docker containers. This approach allows you to easily switch between different configurations without modifying the underlying container image.
Here's an example of how you can inject a configuration file using an environment variable in a Dockerfile:
FROM nginx:latest ENV NGINX_CONF=/path/to/nginx.conf COPY $NGINX_CONF /etc/nginx/nginx.conf # Rest of the Dockerfile
In this example, the environment variable NGINX_CONF
holds the path to the desired configuration file. We then use the COPY
command to copy the configuration file into the appropriate location within the container.
Example 5: Passing Runtime Parameters
Lastly, environment variables can be used to pass runtime parameters to your Docker containers. This allows you to change the behavior of your application without rebuilding the container image.
Here's an example of how you can pass a runtime parameter using an environment variable when running a container:
docker run -e PARAMETER=value my-image
By using the -e
flag, we set the environment variable PARAMETER
to the desired value when running the container. This value can then be accessed within your application code.
Advanced Techniques for Working with Docker
In this chapter, we will explore some advanced techniques for working with Docker. These techniques will help you optimize your Docker workflow and make your containerized applications more efficient.
1. The WORKDIR Instruction
The WORKDIR
instruction in Dockerfile sets the working directory for any subsequent instructions. It helps in organizing the files and directories within your container. By setting the working directory, you can easily reference other files and directories within that directory.
Here's an example of how to use the WORKDIR
instruction in a Dockerfile:
FROM node:14 WORKDIR /app COPY . . RUN npm install CMD ["npm", "start"]
In this example, we set the working directory to /app
, and then copy all the files from the current directory to the /app
directory inside the container. This allows us to easily reference files and directories within the /app
directory.
Related Article: How to Run a Docker Instance from a Dockerfile
2. The RUN Command with Shell Form
The RUN
command in Dockerfile allows you to execute commands inside the container during the build process. By default, the command is executed using the shell form, which means it is executed in a shell environment.
Here's an example of how to use the RUN
command with the shell form:
FROM ubuntu:20.04 RUN apt-get update && apt-get install -y \ curl \ git \ unzip
In this example, we use the RUN
command to update the package lists and install some packages using the apt-get
command.
3. Environment Variables
Environment variables are a great way to configure your Docker containers. They allow you to pass configuration values to your container at runtime, without the need to hardcode them in your Dockerfile or command line.
To set an environment variable in Docker, you can use the ENV
instruction in your Dockerfile or pass them using the -e
flag when running the container.
Here's an example of setting an environment variable in a Dockerfile:
FROM python:3 ENV MY_ENV_VAR=my_value CMD echo $MY_ENV_VAR
In this example, we set the environment variable MY_ENV_VAR
to my_value
using the ENV
instruction. Then, when the container is run, the value of the environment variable is echoed.
You can also pass environment variables using the -e
flag when running the container:
docker run -e MY_ENV_VAR=my_value my_image
This will set the environment variable MY_ENV_VAR
to my_value
when running the container.
By using environment variables, you can easily configure your containers for different environments without the need to modify your Dockerfile.
These advanced techniques for working with Docker will enhance your containerized applications and improve your overall Docker workflow. Experiment with them and see how they can benefit your development process.
Building Custom Docker Images
When using Docker, you often find yourself needing a custom image that includes specific packages, configurations, or dependencies. Building your own custom Docker image allows you to create a containerized environment tailored to your needs. In this chapter, we will explore the process of building custom Docker images.
To build a custom Docker image, you need to create a Dockerfile. A Dockerfile is a text file that contains a series of instructions for building an image. These instructions specify the base image, add files or directories, install packages, set environment variables, and more.
Let's start by creating a basic Dockerfile for a custom image:
# Use an existing base image FROM ubuntu:18.04 # Set the working directory WORKDIR /app # Copy the source code into the container COPY . /app # Install any necessary packages or dependencies RUN apt-get update && apt-get install -y # Set environment variables ENV VAR_NAME=value # Specify the command to run when the container starts CMD ["python", "app.py"]
Let's go through each instruction step by step:
1. **FROM**: The FROM
instruction specifies the base image to build upon. It can be an official Docker image or one you've created previously.
2. **WORKDIR**: The WORKDIR
instruction sets the working directory for any subsequent instructions in the Dockerfile. It's a good practice to set a working directory to avoid specifying absolute paths in other instructions.
3. **COPY**: The COPY
instruction copies files or directories from the host machine to the container. In the example above, we are copying the source code into the /app
directory of the container.
4. **RUN**: The RUN
instruction allows you to execute commands inside the container during the build process. In the example, we are updating the package list and installing a package using apt-get
.
5. **ENV**: The ENV
instruction sets environment variables inside the container. This is useful for configuring the container's behavior or passing configuration values to the application.
6. **CMD**: The CMD
instruction specifies the default command to run when the container starts. In the example, we are running a Python script named app.py
.
To build the Docker image, navigate to the directory containing the Dockerfile and run the following command:
docker build -t custom-image:tag .
The -t
flag specifies the name and optional tag for the image. The .
at the end indicates that the build context is the current directory.
Once the build process completes, you can run a container using the custom image:
docker run custom-image:tag
Building custom Docker images allows you to create a reproducible and portable environment for your applications. It also enables you to share your custom images with others or use them in various deployment scenarios.
For more advanced use cases, you can explore other Dockerfile instructions and techniques such as multi-stage builds, caching, and layer optimization. Docker documentation provides detailed information on these topics.
In the next chapter, we will delve into the topic of managing container data with Docker volumes. Stay tuned!
Optimizing Docker Performance
When using Docker, optimizing performance is crucial to ensure efficient and smooth operations. In this chapter, we will explore some key strategies to optimize the performance of your Docker containers.
Related Article: How to Use Environment Variables in Docker Compose
1. Use the Workdir Directive
The WORKDIR
directive in a Dockerfile sets the working directory for the instructions that follow it. It is recommended to use the WORKDIR
directive to specify a working directory inside the container. This can improve performance by reducing the need to specify absolute paths in subsequent instructions.
For example, consider the following Dockerfile:
FROM node:14 WORKDIR /app COPY . . RUN npm install CMD ["npm", "start"]
In this example, the WORKDIR /app
directive sets the working directory to /app
. This means that subsequent instructions such as COPY
and RUN
will be relative to this directory, improving performance and readability.
2. Optimize the Run Command
The docker run
command is used to run a Docker container based on an image. Optimizing the run command can significantly improve performance.
One important optimization is to use the --rm
flag to automatically remove the container when it exits. This helps to prevent unused containers from cluttering up your system.
Additionally, you can use the --detach
or -d
flag to run containers in the background. This allows you to continue working in the same terminal session without the container's output cluttering your screen.
Another useful option is to limit the container's resource usage. For example, you can use the --cpus
flag to limit the number of CPUs the container can use. This can prevent the container from consuming excessive resources and impacting the performance of other applications running on the host machine.
3. Leverage Environment Variables
Environment variables are a powerful feature in Docker that allow you to configure your containers at runtime. Leveraging environment variables can greatly enhance the performance and flexibility of your Docker deployments.
By using environment variables, you can easily modify container behavior without modifying the underlying image. This makes it easier to reuse the same image in different environments or with different configurations.
To set an environment variable in a Dockerfile, use the ENV
directive. For example, the following Dockerfile sets the environment variable NODE_ENV
to "production":
FROM node:14 ENV NODE_ENV=production
To pass environment variables to a container at runtime, you can use the -e
flag with the docker run
command. For example, to pass the value "123" to an environment variable named MY_VAR
, you can run:
docker run -e MY_VAR=123 my-image
Using environment variables can improve the performance of your Docker containers by allowing you to easily configure and customize their behavior without modifying the image itself.
These are just a few strategies to optimize the performance of your Docker containers. By utilizing the WORKDIR
directive, optimizing the run command, and leveraging environment variables, you can achieve better performance and efficiency in your Docker environment.
Troubleshooting Docker Issues
Docker is a powerful tool for managing and running containerized applications, but like any technology, it can sometimes encounter issues. In this chapter, we will discuss common Docker issues and how to troubleshoot them effectively.
Related Article: Tutorial: Managing Docker Secrets
1. Container Fails to Start
One of the most common issues when using Docker is a container that fails to start. This can happen for various reasons, such as incorrect configurations or missing dependencies. To troubleshoot this issue, you can follow these steps:
1. Check the container logs: Use the docker logs
command followed by the container ID or name to view the logs generated by the container. This can provide valuable information about why the container failed to start.
2. Inspect the container configuration: Run the docker inspect
command followed by the container ID or name to get detailed information about the container's configuration. Check for any misconfigured settings or missing dependencies.
3. Verify image availability: Ensure that the Docker image required to start the container is available locally or in a remote registry. You can use the docker images
command to list all the images available on your system.
2. Networking Issues
Docker containers rely on networking to communicate with each other and the outside world. If you encounter networking issues with your containers, here are some troubleshooting steps:
1. Check container connectivity: Use the docker exec
command followed by the container ID or name and a network troubleshooting tool like ping
or curl
to verify if the container can reach other hosts or external services.
2. Inspect network settings: Run the docker network inspect
command followed by the network ID or name to examine the network configuration. Make sure that the containers are connected to the correct network and have the appropriate IP addresses.
3. Check firewall rules: If your host system has a firewall enabled, ensure that it allows traffic to and from Docker containers. Docker uses various ports for communication, so make sure the necessary ports are open.
3. Resource Constraints
Docker containers consume system resources such as CPU, memory, and disk space. If you experience performance issues or resource limitations, consider the following troubleshooting steps:
1. Monitor resource usage: Utilize tools like docker stats
or a container orchestration platform to monitor resource utilization of running containers. Identify any containers that are consuming excessive resources and optimize their configurations or resource limits.
2. Adjust resource limits: Docker allows you to set limits on CPU, memory, and other resources using the --cpus
, --memory
, and related flags. Ensure that your containers have appropriate limits set to prevent resource contention.
3. Check disk space: If your host system runs out of disk space, it can cause issues with Docker. Use the docker system df
command to check the Docker disk usage and clean up any unnecessary images or containers.
Remember, troubleshooting Docker issues often requires a systematic approach. Always start by gathering relevant information such as container logs, configuration details, and network settings. By following the steps outlined in this chapter, you'll be able to diagnose and resolve common Docker issues effectively.
Scaling Docker Applications
Scaling Docker applications is an essential aspect of managing containerized environments. By effectively scaling your Docker applications, you can ensure high availability, improve performance, and accommodate increased traffic or workload demands. In this chapter, we will explore some useful tips and techniques for scaling Docker applications.
Related Article: Tutorial on Installing and Using redis-cli with Redis
1. Horizontal Scaling
One of the most common scaling techniques is horizontal scaling, which involves adding more instances of your application to handle increased traffic or workload. Docker makes horizontal scaling easy by allowing you to create multiple replicas of your containers and distribute the load across them.
To scale your Docker application horizontally, you can use Docker Compose or an orchestration tool like Docker Swarm or Kubernetes. For example, with Docker Compose, you can define the desired number of replicas for your services in the docker-compose.yml
file:
version: '3' services: web: image: myapp:latest scale: 3
In this example, the web
service will be scaled to three replicas, distributing the workload across the three instances.
2. Load Balancing
Load balancing is another important aspect of scaling Docker applications. It allows you to distribute incoming requests across multiple containers to ensure optimal performance and avoid overloading a single instance.
There are several ways to implement load balancing with Docker. One common approach is to use a reverse proxy server like NGINX or HAProxy. These servers act as a single entry point for incoming requests and distribute them to the available containers based on predefined rules.
Here's an example of using NGINX as a reverse proxy for load balancing Docker containers:
http { upstream myapp { server app1:80; server app2:80; server app3:80; } server { listen 80; location / { proxy_pass http://myapp; } } }
In this example, NGINX is configured to proxy requests to three instances of the myapp
application running on different containers.
3. Service Discovery
When scaling Docker applications, it's crucial to have a mechanism for service discovery. Service discovery allows containers to find and communicate with each other, even as they scale up or down.
Docker provides built-in service discovery features through its integrated DNS server. Containers within the same Docker network can refer to each other using their service names as hostnames. For example, if you have a service named myapp
, other containers can access it using the hostname myapp
.
Service discovery can also be accomplished using external tools like Consul or etcd. These tools provide additional features such as health checks and dynamic configuration updates, making them suitable for more complex scaling scenarios.
4. Monitoring and Logging
To effectively scale Docker applications, it's crucial to have proper monitoring and logging in place. Monitoring allows you to identify performance bottlenecks, resource utilization, and potential issues. Logging helps you track application behavior, troubleshoot problems, and analyze historical data.
Docker provides various monitoring and logging options, including built-in functionality and integration with third-party tools. Some popular choices for monitoring Docker applications are Prometheus, Grafana, and cAdvisor. For logging, tools like ELK Stack (Elasticsearch, Logstash, and Kibana) or Splunk can be used to collect, analyze, and visualize log data.
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
5. Auto Scaling
Auto scaling is an advanced technique that allows your Docker application to automatically adjust its capacity based on predefined rules or metrics. With auto scaling, you can dynamically add or remove instances of your containers to match the current workload or traffic patterns.
Docker Swarm and Kubernetes both provide built-in support for auto scaling based on CPU usage, memory utilization, or custom metrics. These platforms monitor the application's resource consumption and scale the number of replicas accordingly.
For example, in Kubernetes, you can define an auto scaling policy using the Horizontal Pod Autoscaler (HPA) feature:
apiVersion: autoscaling/v2beta2 kind: HorizontalPodAutoscaler metadata: name: myapp-autoscaler spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: myapp minReplicas: 2 maxReplicas: 10 metrics: - type: Resource resource: name: cpu target: type: Utilization averageUtilization: 70
In this example, the autoscaler will adjust the number of replicas for the myapp
deployment based on the average CPU utilization, maintaining a minimum of 2 replicas and a maximum of 10.
Scaling Docker applications is a critical aspect of managing containerized environments. By understanding and implementing the techniques discussed in this chapter, you can ensure the scalability and performance of your Docker applications.
Security Considerations for Docker
When using Docker, it is important to consider security measures to protect your applications and data. Docker provides some built-in security features, but there are additional steps you can take to enhance the security of your Docker containers.
1. Keep Docker Up to Date
Regularly updating Docker is crucial to ensure you have the latest security patches and bug fixes. Docker releases updates frequently, addressing vulnerabilities and improving security. To update Docker, you can use the following command:
$ docker update
2. Use Official and Verified Images
It is highly recommended to use official Docker images from trusted sources. Official images are regularly maintained and updated to address security concerns. Additionally, always verify the authenticity and integrity of the images you use. Docker provides a verification process to check the integrity of an image before pulling it. You can verify an image using the following command:
$ docker trust inspect
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
3. Limit Container Privileges
By default, Docker containers run with root privileges, which can be a security risk. It is best practice to run containers with non-root users whenever possible. You can specify a non-root user using the USER
instruction in your Dockerfile. For example:
FROM ubuntu USER myuser
4. Restrict Container Capabilities
Docker containers inherit the host system's kernel capabilities by default. It is recommended to restrict container capabilities to reduce the potential attack surface. The --cap-drop
and --cap-add
flags can be used with the docker run
command to drop or add specific capabilities to a container. For example:
$ docker run --cap-drop=NET_RAW --cap-add=NET_ADMIN my-container
5. Secure Docker Daemon
The Docker daemon should be secured to prevent unauthorized access to the host system. By default, the Docker daemon listens on a Unix socket, which is accessible only by the root user. You can also configure the Docker daemon to listen on a specific IP and port, enabling remote management. However, this should only be done with proper authentication and encryption. Refer to the Docker documentation for more information on securing the Docker daemon.
6. Use Docker Secrets for Sensitive Data
Sensitive data such as passwords, API keys, and certificates should not be stored directly in Docker images or environment variables. Docker provides a feature called Docker Secrets that allows you to securely manage and distribute sensitive data to containers. Secrets are encrypted and only accessible to the services that need them. You can create a secret using the following command:
$ echo "mysecretpassword" | docker secret create mysecret -
Related Article: How to Use the Host Network in Docker Compose
7. Enable AppArmor or SELinux
AppArmor and SELinux are security modules that provide mandatory access control for Docker containers. They enforce security policies and restrict the actions containers can take. Enabling either AppArmor or SELinux can provide an additional layer of protection. Refer to the Docker documentation for instructions on enabling and configuring these security modules.
8. Regularly Monitor and Audit Containers
Monitoring and auditing containers can help detect and prevent security breaches. Use container monitoring tools to track container behavior, resource usage, and network activity. Additionally, regularly review container logs and system logs for any suspicious activity. Docker provides logging drivers that allow you to forward container logs to external systems for centralized monitoring and analysis.
By following these security considerations, you can enhance the security of your Docker containers and protect your applications and data from potential vulnerabilities and attacks. Always stay updated with the latest security best practices and guidelines provided by Docker and the community.
Deploying Docker in Production Environments
Deploying Docker in production environments requires careful consideration and planning to ensure a smooth and reliable deployment. Here are some tips to help you deploy Docker containers effectively in a production environment.
1. Use a proper base image:
Selecting the right base image is crucial for the security and stability of your Docker containers. Choose an image that is regularly updated, maintained by the community, and has a small attack surface. Avoid using images that are outdated or have known security vulnerabilities.
2. Optimize container size:
Reducing the size of your Docker containers can lead to faster deployment times and lower resource consumption. Use multi-stage builds to minimize the number of layers and remove unnecessary files and dependencies. Additionally, consider using Alpine-based images, as they are known for their small size.
3. Set the working directory:
Specify the working directory inside your Docker container using the WORKDIR
instruction in your Dockerfile. This ensures that any relative file paths used in your container are resolved correctly, making your container more portable and easier to manage.
WORKDIR /app
4. Configure environment variables:
Use environment variables to configure your Docker containers. This allows you to separate configuration from code and makes your containers more flexible and easier to manage. Environment variables can be set using the -e
flag when running the docker run
command or by using a .env
file.
docker run -e MY_VARIABLE=value my-image
5. Manage secrets securely:
When deploying Docker containers in production, it's important to handle sensitive information, such as passwords or API keys, securely. Avoid hardcoding secrets in your Dockerfile or environment variables. Instead, consider using a secrets management tool like Docker Secrets or storing secrets in a secure key vault.
6. Monitor container health:
Implement container health checks to ensure the availability and reliability of your Docker containers. Docker provides a built-in health check mechanism that allows you to define a command or HTTP endpoint to periodically check the container's health status.
HEALTHCHECK --interval=5m --timeout=3s \ CMD curl -f http://localhost/ || exit 1
7. Use orchestration tools:
When deploying Docker containers in a production environment, consider using orchestration tools like Kubernetes or Docker Swarm. These tools provide advanced features for managing and scaling containerized applications, including load balancing, service discovery, and automatic container recovery.
8. Implement logging and monitoring:
Enable logging and monitoring for your Docker containers to gain insights into their performance and troubleshoot issues. Use tools like ELK Stack (Elasticsearch, Logstash, and Kibana) or Prometheus and Grafana to collect and visualize container logs and metrics.
By following these tips, you can ensure a smooth and reliable deployment of Docker containers in production environments. Remember to regularly update your container images, monitor their health, and implement proper security measures to ensure the stability and security of your application.
Monitoring and Logging for Docker
Monitoring and logging are essential for managing and troubleshooting Docker containers. They provide insights into the health, performance, and behavior of your containers and help you identify and fix issues quickly. In this chapter, we will explore some useful tips and best practices for monitoring and logging in Docker.
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
1. Monitoring Docker Containers
Monitoring Docker containers allows you to track their resource usage, network activity, and overall performance. There are several tools available for monitoring Docker containers, including:
- Prometheus: A powerful open-source monitoring and alerting toolkit that provides a wide range of metrics and visualization options for Docker containers. You can configure Prometheus to scrape metrics from the Docker daemon and containerized applications.
- Grafana: An open-source analytics and monitoring platform that works seamlessly with Prometheus. Grafana allows you to create custom dashboards to visualize and analyze the metrics collected by Prometheus.
- cAdvisor: A lightweight container monitoring tool developed by Google. cAdvisor collects and exports metrics about running containers, such as CPU, memory, and network usage. It provides a web interface for viewing container stats and can be integrated with other monitoring systems.
2. Logging Docker Containers
Logging is crucial for capturing container events, errors, and application output. Docker provides various logging drivers that allow you to control how container logs are collected and stored. Some popular logging drivers include:
- json-file: The default logging driver in Docker, which writes container logs as JSON files on the host machine. You can configure the maximum size and number of log files to retain.
- syslog: Sends container logs to the syslog daemon on the host machine. This can be useful for centralized logging and integration with existing logging infrastructure.
- fluentd: A popular open-source data collector that can aggregate container logs and forward them to multiple destinations, such as Elasticsearch, Kafka, or cloud-based log analysis services.
To enable a specific logging driver for a container, you can use the --log-driver
option when running the container:
$ docker run --log-driver=json-file my-container
You can also configure the logging driver in a Docker Compose file:
version: '3' services: my-service: image: my-container logging: driver: json-file
3. Monitoring and Logging Tips
Here are some tips and best practices for monitoring and logging in Docker:
- Use container labels: Assigning labels to your containers can help you organize and categorize them. You can use labels to filter and aggregate metrics and logs in monitoring and logging systems.
- Monitor container health: Docker provides a health check mechanism that allows you to define a command or script to periodically check the health of your containers. Monitoring the health of your containers can help you detect and handle failures early.
- Monitor container resource usage: Keep an eye on the resource consumption of your containers, such as CPU, memory, and disk usage. Monitoring resource usage can help you identify performance bottlenecks and optimize container configurations.
- Monitor container network traffic: Track the network activity of your containers, such as incoming and outgoing connections, bandwidth usage, and latency. Monitoring network traffic can help you identify security issues and optimize network configurations.
- Centralize logs: Consider centralizing your container logs in a dedicated logging system. Centralized logging provides a unified view of your container logs and simplifies log analysis and troubleshooting.
- Implement log rotation: Configure log rotation to prevent log files from consuming excessive disk space. Regularly rotate and compress log files to ensure efficient log storage and retrieval.
In the next chapter, we will explore more advanced tips and techniques for working with Docker, including managing container networking and security.
Backup and Recovery Strategies for Docker
Backing up and recovering your Docker containers and images is essential to ensure the safety and availability of your applications. In this chapter, we will explore some useful strategies for backing up and recovering Docker environments.
Related Article: How to Implement Database Sharding in MongoDB
Backing up Docker Containers
To back up a Docker container, you can use the Docker commit command to create a new image from the container's current state. This image can then be saved to a tar file and stored as a backup. Here's an example of how you can back up a running container:
$ docker commit $ docker save -o .tar
This will create a tar file containing the backup image.
Backing up Docker Volumes
In addition to backing up the container itself, you may also want to back up any data volumes associated with the container. Docker provides a command called docker volume
that allows you to create a backup of a volume.
$ docker run --rm -v :/data -v :/backup busybox \ tar -czvf /backup/.tar.gz /data
This command mounts the volume into a temporary container and uses the tar
command to create a compressed backup file.
Backing up Docker Images
To back up Docker images, you can use the Docker save command to export an image to a tar file. Here's an example:
$ docker save -o .tar
This will save the image as a tar file, which can be stored as a backup.
Recovering Docker Containers
To recover a Docker container from a backup, you can use the Docker run command with the --volumes-from
flag to mount the volumes from the backup container. Here's an example:
$ docker run --volumes-from -d --name
This command creates a new container and mounts the volumes from the backup container.
Related Article: How to Use Nested Queries in Databases
Recovering Docker Images
To recover a Docker image from a backup, you can use the Docker load command to import the image from the tar file. Here's an example:
$ docker load -i .tar
This will load the image into Docker, making it available for use.
Docker Networking: Bridged Networks
In Docker, networking is a crucial aspect that allows containers to communicate with each other and the outside world. By default, Docker creates a bridge network, known as the bridge
network, for containers to connect to. This network isolates containers from the host machine and other networks.
When a container is launched without specifying a network, it is automatically connected to the bridge network. This allows the container to communicate with other containers on the same network, but not with the host machine or other networks.
To list the available networks in Docker, you can use the following command:
$ docker network ls
By default, you should see the bridge
network listed. This network uses the docker0
interface on the host machine to provide connectivity for containers.
To create a new bridged network, you can use the docker network create
command followed by the desired network name. For example, to create a network called my-network
, you would run:
$ docker network create my-network
This command will create a new bridged network named my-network
. You can then connect containers to this network by specifying the network name when launching the containers.
$ docker run --network=my-network my-container
In addition to the bridge network, Docker provides other types of networks such as host
and overlay
. The host
network allows containers to share the host's network stack, while the overlay
network enables communication between containers across multiple Docker hosts in a swarm.
To learn more about Docker networking and the different network types, you can refer to the official Docker documentation on networking: https://docs.docker.com/network/.
Bridged networks in Docker provide a convenient way to connect containers together and enable communication between them. By understanding how Docker networking works, you can effectively design and manage your containerized applications.
Docker Networking: Host Networks
In Docker, by default, each container runs in its own isolated network namespace. This means that the container has its own IP address and its own network interfaces. However, there are cases when you might want to run a container in the host network, where the container shares the network namespace with the host machine.
Using the host network mode can be useful in situations where the container needs to bind to a specific network interface or when you want the container to have direct access to the host's network stack.
To run a container in host network mode, you can use the --network=host
option when starting the container:
docker run --network=host
By doing this, the container uses the host's network stack, and thus, the container's network interfaces are the same as the host's network interfaces. This means that the container can listen on any port that is available on the host machine, without the need to publish or map ports.
However, running a container in host network mode comes with some trade-offs. One of the main trade-offs is that the container no longer has its own isolated network namespace. This means that the container's networking is not isolated from the host machine, and any network security measures applied on the host will directly affect the container.
Another trade-off is that running a container in host network mode can lead to port conflicts if multiple containers are running on the same host machine and trying to bind to the same port.
If you need to access a specific port on the host machine from within a container running in host network mode, you can simply use localhost
as the target address, as the container shares the network stack with the host.
In conclusion, using host network mode in Docker can be beneficial in certain scenarios, such as when you need the container to have direct access to the host's network stack or when you want the container to bind to a specific network interface. However, it's important to consider the trade-offs, such as the lack of network isolation and potential port conflicts.
Docker Networking: Overlay Networks
Docker provides a powerful networking feature called Overlay Networks that allows containers to communicate with each other across multiple Docker hosts. This is particularly useful when working with distributed applications that are spread across multiple machines.
Overlay Networks are created using the Docker Swarm mode, which allows you to create a cluster of Docker nodes and manage them as a single entity. To use Overlay Networks, you need to have Docker Swarm mode enabled on your Docker hosts.
To create an Overlay Network, you can use the following command:
docker network create --driver overlay my-network
This command creates an Overlay Network named "my-network" using the default overlay network driver. You can choose a different driver by specifying the --driver
option.
Once the Overlay Network is created, you can attach containers to it using the --network
option when running a container:
docker run -d --network=my-network --name=my-container my-image
In this example, the container named "my-container" is attached to the "my-network" Overlay Network. You can now communicate with other containers in the same network using their container names as hostnames.
Overlay Networks also support service discovery, which allows you to access containers by their service names instead of their container names. To enable service discovery, you need to create a service using the --name
option:
docker service create --network=my-network --name=my-service my-image
Now, you can access the containers in the Overlay Network using the service name as the hostname.
Overlay Networks provide a secure and scalable way to connect containers across multiple Docker hosts. They are particularly useful for deploying distributed applications that require communication between different components running on different machines.
To learn more about Overlay Networks and Docker Swarm mode, you can refer to the official Docker documentation:
https://docs.docker.com/engine/swarm/networking/
Related Article: Copying a Directory to Another Using the Docker Add Command
Exploring Docker Network Drivers
Docker networks are a powerful feature that allow containers to communicate with each other and with the outside world. Docker provides several built-in network drivers that can be used to create and manage these networks. In this chapter, we will explore some of the most commonly used Docker network drivers.
1. Bridge Network Driver
The bridge network driver is the default network driver used by Docker. It creates a private network for the containers on a single host and provides each container with a unique IP address. Containers connected to the same bridge network can communicate with each other using these IP addresses.
To create a bridge network, you can use the following command:
docker network create mybridge
This will create a new bridge network named "mybridge". You can then connect containers to this network using the --network
flag when running a container.
2. Host Network Driver
The host network driver allows a container to use the network stack of the host machine. This means that the container shares the same network interface as the host and does not have its own IP address. As a result, containers using the host network driver can access services running on the host directly.
To run a container using the host network driver, you can use the following command:
docker run --network host myimage
This will run a container using the host network driver and the image "myimage".
3. Overlay Network Driver
The overlay network driver allows you to create multi-host networks that span multiple Docker hosts. This is useful for deploying applications across multiple machines or for connecting containers running on different hosts.
To create an overlay network, you can use the following command:
docker network create --driver overlay myoverlay
This will create a new overlay network named "myoverlay". You can then connect containers to this network using the --network
flag when running a container.
4. Macvlan Network Driver
The macvlan network driver allows you to assign a MAC address to a container, making it appear as a physical device on the network. This is useful in scenarios where you need to assign a specific IP address to a container or when you want to bridge Docker containers with physical devices on the network.
To create a macvlan network, you can use the following command:
docker network create -d macvlan --subnet=192.168.1.0/24 --gateway=192.168.1.1 -o parent=eth0 mymacvlan
This will create a new macvlan network named "mymacvlan" with the specified subnet, gateway, and parent interface.
5. Null Network Driver
The null network driver is a special network driver that isolates a container from any network access. This is useful in scenarios where you want to run a container without any network capabilities.
To run a container using the null network driver, you can use the following command:
docker run --network none myimage
This will run a container using the null network driver and the image "myimage".