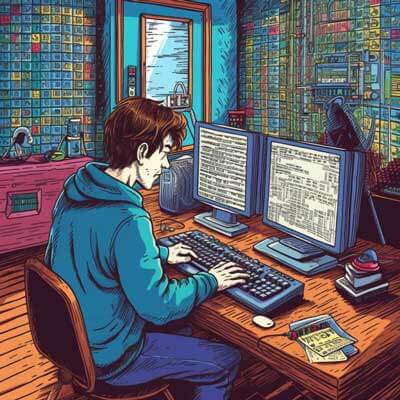
- Introduction to Database Sharding
- Use Cases for Sharding in MySQL
- Best Practices for Sharding in MySQL
- Real World Examples of Sharding in MySQL
- Performance Considerations for Sharding in MySQL
- Pros and Cons of Sharding in MySQL
- Code Snippet Ideas for Sharding in MySQL
- Error Handling in Sharded MySQL Databases
- Overview of MySQL Sharding Features
- Using Range Sharding in MySQL
- Using Hash Sharding in MySQL
- Using Key-based Sharding in MySQL
- Using Table-based Sharding in MySQL
- Using Vertical Sharding in MySQL
- Using Horizontal Sharding in MySQL
- Using Consistent Hashing for Sharding in MySQL
- Introduction to Third-Party Tools for Sharding in MySQL
- Comparing Third-Party Tools for Sharding in MySQL
- Advanced Sharding Techniques for MySQL
- Optimizing Query Performance in a Sharded MySQL Database
- Managing Data Consistency in a Sharded MySQL Database
- Replication and Failover Strategies for Sharded MySQL Databases
Introduction to Database Sharding
Database sharding is a technique used to horizontally partition a large database into smaller, more manageable shards. Each shard contains a subset of the data, allowing for better scalability and performance in distributed systems. In the context of MySQL, sharding involves distributing the data across multiple MySQL instances, or shards, based on a predefined sharding key.
Sharding offers several benefits, including improved read and write performance, increased storage capacity, and better fault tolerance. However, it also introduces complexity in terms of data distribution, query routing, and data consistency. In this chapter, we will explore the fundamentals of database sharding and how it can be implemented in MySQL.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Use Cases for Sharding in MySQL
Sharding in MySQL is commonly used in scenarios where a single database instance cannot handle the volume of data or the number of concurrent requests. Some common use cases for sharding in MySQL include:
1. Large-scale web applications: Websites with millions or billions of users generate massive amounts of data. Sharding allows the data to be distributed across multiple MySQL instances, enabling efficient data storage and retrieval.
2. E-commerce platforms: Online marketplaces often experience high traffic and require fast response times. Sharding helps distribute the load across multiple shards, allowing for better performance and scalability.
3. Social networking sites: Social media platforms deal with vast amounts of user-generated data, such as posts, comments, and likes. Sharding enables efficient storage and retrieval of this data, ensuring optimal performance.
4. IoT applications: Internet of Things (IoT) devices generate a continuous stream of data. Sharding can be used to handle the high data volume and ensure fast processing and analysis.
Best Practices for Sharding in MySQL
When implementing sharding in MySQL, it is essential to follow best practices to ensure optimal performance and data integrity. Here are some key best practices to consider:
1. Choose the right sharding key: The sharding key determines how data is distributed across shards. It should be carefully chosen to evenly distribute the data and avoid hotspots. Common sharding keys include user IDs, timestamps, or geographical locations.
2. Plan for data growth: Sharding allows for horizontal scalability, but it is crucial to plan for future data growth. Consider the potential number of shards, the distribution of data, and how the system will handle increased load.
3. Implement a shard management layer: A shard management layer helps with query routing, data distribution, and shard failover. It acts as a middleware between the application and the individual shards, abstracting the complexity of sharding.
4. Monitor and tune performance: Regularly monitor the performance of the sharded MySQL database. Use tools like MySQL’s performance schema and query analyzer to identify bottlenecks and optimize queries.
5. Handle data consistency: Ensuring data consistency in a sharded environment can be challenging. Consider using distributed transaction frameworks like XA transactions or two-phase commit protocols to maintain data integrity across shards.
6. Backup and recovery: Implement a robust backup and recovery strategy to protect against data loss. Regularly backup the data on each shard and test the recovery process to ensure its reliability.
Real World Examples of Sharding in MySQL
Let’s explore two real-world examples of sharding in MySQL to understand how it can be implemented in practice.
Example 1: Social Media Platform
A social media platform with millions of users decides to shard its user data to handle the increasing load. The sharding key chosen is the user ID. The platform uses a shard management layer that routes user-related queries to the appropriate shard based on the user ID.
Snippet 1: Creating a sharded user table
CREATE TABLE user ( id INT PRIMARY KEY, name VARCHAR(50), email VARCHAR(100) ) ENGINE=InnoDB;
Snippet 2: Inserting data into a sharded user table
INSERT INTO user (id, name, email) VALUES (1, 'John Doe', 'john@example.com');
Example 2: E-commerce Platform
An e-commerce platform experiences high traffic during peak shopping seasons and decides to shard its product data. The sharding key chosen is the product category. Each shard stores a specific category of products, such as electronics, clothing, or home goods.
Snippet 3: Creating a sharded product table
CREATE TABLE product ( id INT PRIMARY KEY, name VARCHAR(100), category VARCHAR(50), price DECIMAL(10,2) ) ENGINE=InnoDB;
Snippet 4: Inserting data into a sharded product table
INSERT INTO product (id, name, category, price) VALUES (1, 'iPhone 12', 'Electronics', 999.99);
These examples demonstrate how sharding can be applied to different use cases, distributing data across multiple MySQL instances for improved performance and scalability.
Related Article: How to Perform a Full Outer Join in MySQL
Performance Considerations for Sharding in MySQL
When sharding a MySQL database, it is crucial to consider the performance implications. Here are some performance considerations to keep in mind:
1. Query routing overhead: With sharding, queries need to be routed to the appropriate shards based on the sharding key. This routing overhead can add latency to query execution. Implement efficient query routing mechanisms to minimize this overhead.
2. Join operations: Joining tables across multiple shards can be challenging and can impact performance. Avoid frequent joins between sharded tables or consider denormalizing data to reduce the need for joins.
3. Load balancing: Uneven data distribution or hotspots in the sharded environment can lead to imbalanced load on shards. Implement load balancing mechanisms to evenly distribute the workload across shards and avoid performance bottlenecks.
4. Indexing strategy: Proper indexing is crucial for query performance in a sharded MySQL database. Analyze query patterns and create indexes on the sharding key and frequently queried columns to optimize query execution.
5. Connection management: Managing connections to multiple shards can impact performance. Implement connection pooling and optimize connection management to minimize overhead.
6. Caching: Implement caching mechanisms, such as Redis or Memcached, to reduce the load on the database and improve query response times.
Consider these performance considerations when designing and implementing a sharded MySQL database to ensure optimal performance and scalability.
Pros and Cons of Sharding in MySQL
Sharding in MySQL offers several advantages, but it also comes with some drawbacks. Let’s explore the pros and cons of sharding:
Pros:
– Improved scalability: Sharding allows for horizontal scalability by distributing data across multiple shards, enabling the system to handle larger data volumes and higher traffic.
– Better performance: Sharding can significantly improve read and write performance by distributing the load across multiple shards.
– Increased storage capacity: By distributing data across multiple shards, sharding increases the storage capacity of the system.
– Fault tolerance: Sharding provides fault tolerance by replicating data across multiple shards. If one shard fails, the system can continue to operate using the remaining shards.
Cons:
– Complexity: Sharding introduces complexity in terms of data distribution, query routing, and data consistency. It requires careful planning and implementation.
– Data consistency: Ensuring data consistency in a sharded environment can be challenging. Coordinating updates across multiple shards and maintaining data integrity requires additional effort.
– Joins and transactions: Joining tables across multiple shards and performing distributed transactions can be complex and impact performance.
– Increased operational overhead: Sharding adds operational overhead, such as managing multiple shards, backup and recovery, and monitoring performance.
Consider these pros and cons when deciding whether to implement sharding in MySQL for your specific use case.
Code Snippet Ideas for Sharding in MySQL
Here are a few code snippet ideas that demonstrate various aspects of sharding in MySQL:
Snippet 1: Query routing based on the sharding key
-- Assuming sharding key is 'user_id' SET @user_id = 123; SELECT * FROM user WHERE id = @user_id;
Snippet 2: Inserting data into a sharded table with automatic sharding key resolution
-- Assuming sharding key is 'category' INSERT INTO product (name, category, price) VALUES ('MacBook Pro', 'Electronics', 1999.99);
Snippet 3: Joining tables across shards
SELECT * FROM order JOIN user ON order.user_id = user.id WHERE order.id = 456;
Snippet 4: Performing a distributed transaction across shards
-- Assuming XA transaction support is enabled XA START 'shard1'; INSERT INTO user (id, name) VALUES (1, 'John Doe'); XA END 'shard1'; XA PREPARE 'shard1'; XA COMMIT 'shard1'; XA START 'shard2'; INSERT INTO user (id, name) VALUES (2, 'Jane Smith'); XA END 'shard2'; XA PREPARE 'shard2'; XA COMMIT 'shard2'; XA RECOVER;
These code snippets illustrate various aspects of sharding in MySQL, including query routing, data insertion, joining tables, and distributed transactions.
Related Article: How to Fix MySQL Error Code 1175 in Safe Update Mode
Error Handling in Sharded MySQL Databases
When working with sharded MySQL databases, it is essential to handle errors effectively to ensure data integrity and maintain system reliability. Here are some error handling techniques to consider:
1. Graceful error handling: Catch and handle errors at the application level to provide meaningful error messages to users and prevent system failures. Implement appropriate error logging and notification mechanisms to track and address errors promptly.
2. Retry mechanism: In a sharded environment, transient errors such as network timeouts or shard unavailability can occur. Implement a retry mechanism to handle such errors and ensure eventual consistency.
3. Rollback and recovery: In the event of an error during a distributed transaction, ensure proper rollback and recovery mechanisms are in place. Use XA transactions or similar frameworks to maintain data consistency across shards.
4. Monitoring and alerting: Set up monitoring tools to detect errors and performance issues in the sharded MySQL environment. Implement alerting mechanisms to notify administrators about critical errors or anomalies.
5. Data validation and reconciliation: Regularly validate and reconcile data across shards to identify any inconsistencies or data corruption. Implement automated data validation scripts or use third-party tools for this purpose.
6. Failover and fallback strategies: Plan for shard failures and implement failover and fallback strategies to ensure uninterrupted service. Use replication and backup mechanisms to maintain data redundancy and availability.
Overview of MySQL Sharding Features
MySQL provides several features and techniques that can be used for sharding. Let’s explore some of these features:
1. Partitioning: MySQL’s native partitioning feature allows you to divide a table into multiple partitions based on predefined criteria, such as ranges or lists of values. Partitioning can be used for sharding by distributing data across multiple partitions/shards.
2. MySQL Cluster: MySQL Cluster is a distributed, shared-nothing database architecture that provides automatic sharding and high availability. It allows for transparent sharding of data across multiple nodes, providing scalability and fault tolerance.
3. MySQL Router: MySQL Router is a middleware component that helps with query routing in a sharded MySQL environment. It acts as a proxy, routing queries to the appropriate shards based on the sharding key.
4. MySQL Fabric: MySQL Fabric is a management framework that provides tools for managing and scaling MySQL deployments. It includes features for sharding, high availability, and automated failover.
5. MySQL Proxy: MySQL Proxy is a lightweight proxy server that sits between the application and the MySQL server. It can be used for query routing, load balancing, and sharding in MySQL.
These are just a few of the features and tools available in MySQL that can be used for sharding. Depending on your specific requirements and use case, you can choose the most appropriate approach for implementing sharding in MySQL.
Using Range Sharding in MySQL
Range sharding is a sharding technique where data is partitioned based on a specific range of values. Each shard is responsible for storing a specific range of data. Let’s explore how range sharding can be implemented in MySQL.
Snippet 1: Creating a range-sharded table
CREATE TABLE customer ( id INT PRIMARY KEY, name VARCHAR(50), age INT, city VARCHAR(50) ) ENGINE=InnoDB PARTITION BY RANGE (age) ( PARTITION p1 VALUES LESS THAN (30), PARTITION p2 VALUES LESS THAN (40), PARTITION p3 VALUES LESS THAN (50), PARTITION p4 VALUES LESS THAN MAXVALUE );
In the above example, the “customer” table is range sharded based on the “age” column. Data with an age less than 30 will be stored in partition “p1”, data with an age less than 40 (but greater than or equal to 30) will be stored in partition “p2”, and so on. The last partition “p4” will store data with ages greater than or equal to 50.
Snippet 2: Inserting data into a range-sharded table
INSERT INTO customer (id, name, age, city) VALUES (1, 'John Doe', 25, 'New York');
Snippet 3: Querying data from a range-sharded table
SELECT * FROM customer WHERE age < 40;
Range sharding allows for efficient querying of data within a specific range. It is suitable when data can be logically divided into ranges based on a specific column.
Related Article: How to Update Records in MySQL with a Select Query
Using Hash Sharding in MySQL
Hash sharding is a sharding technique where data is distributed across shards based on a hashing algorithm. The sharding key is hashed, and the resulting hash value determines which shard the data will be stored on. Let’s see how hash sharding can be implemented in MySQL.
Snippet 1: Creating a hash-sharded table
CREATE TABLE order ( id INT PRIMARY KEY, customer_id INT, product_id INT, quantity INT ) ENGINE=InnoDB PARTITION BY HASH (customer_id) PARTITIONS 4;
In the above example, the “order” table is hash sharded based on the “customer_id” column. The data is distributed across four partitions based on the hash value of the customer ID.
Snippet 2: Inserting data into a hash-sharded table
INSERT INTO order (id, customer_id, product_id, quantity) VALUES (1, 123, 456, 2);
Snippet 3: Querying data from a hash-sharded table
SELECT * FROM order WHERE customer_id = 123;
Hash sharding provides a more even distribution of data compared to range sharding. It is suitable when the distribution of data is unpredictable, and there is no logical range-based partitioning.
Using Key-based Sharding in MySQL
Key-based sharding, also known as composite sharding, is a technique where data is sharded based on a combination of multiple columns. The sharding key is composed of one or more columns, and the resulting key value determines which shard the data will be stored on. Let’s see how key-based sharding can be implemented in MySQL.
Snippet 1: Creating a key-based sharded table
CREATE TABLE product ( id INT PRIMARY KEY, category VARCHAR(50), subcategory VARCHAR(50), name VARCHAR(100), price DECIMAL(10,2) ) ENGINE=InnoDB;
In the above example, the “product” table is key-based sharded based on the combination of the “category” and “subcategory” columns. The data will be distributed across shards based on the values of these columns.
Snippet 2: Inserting data into a key-based sharded table
INSERT INTO product (id, category, subcategory, name, price) VALUES (1, 'Electronics', 'Laptops', 'MacBook Pro', 1999.99);
Snippet 3: Querying data from a key-based sharded table
SELECT * FROM product WHERE category = 'Electronics' AND subcategory = 'Laptops';
Key-based sharding allows for more granular control over the distribution of data. It is suitable when data can be logically partitioned based on multiple columns.
Using Table-based Sharding in MySQL
Table-based sharding is a technique where different tables are sharded independently based on different sharding keys. Each shard contains a subset of tables, and the data is distributed across shards based on the sharding key of each table. Let’s see how table-based sharding can be implemented in MySQL.
Snippet 1: Creating a table-based sharded setup
-- Shard 1 CREATE TABLE shard1.product ( id INT PRIMARY KEY, name VARCHAR(100), category VARCHAR(50) ) ENGINE=InnoDB; -- Shard 2 CREATE TABLE shard2.order ( id INT PRIMARY KEY, customer_id INT, product_id INT, quantity INT ) ENGINE=InnoDB;
In the above example, the “product” table is sharded on shard 1 based on the “id” column, while the “order” table is sharded on shard 2 based on the “customer_id” column. Each shard contains a different set of tables.
Snippet 2: Inserting data into a table-based sharded table
-- Inserting into shard 1's product table INSERT INTO shard1.product (id, name, category) VALUES (1, 'iPhone 12', 'Electronics'); -- Inserting into shard 2's order table INSERT INTO shard2.order (id, customer_id, product_id, quantity) VALUES (1, 123, 1, 2);
Snippet 3: Querying data from a table-based sharded table
-- Querying from shard 1's product table SELECT * FROM shard1.product WHERE id = 1; -- Querying from shard 2's order table SELECT * FROM shard2.order WHERE customer_id = 123;
Table-based sharding provides flexibility in choosing different sharding keys for different tables. It allows for independent distribution of data across shards based on the sharding key of each table.
Related Article: How to Use MySQL Query String Contains
Using Vertical Sharding in MySQL
Vertical sharding, also known as column-based sharding, is a technique where columns of a table are partitioned vertically across multiple shards. Each shard contains a subset of columns for the table. Let’s see how vertical sharding can be implemented in MySQL.
Snippet 1: Creating a vertically sharded table
-- Shard 1 CREATE TABLE shard1.customer ( id INT PRIMARY KEY, name VARCHAR(50), age INT ) ENGINE=InnoDB; -- Shard 2 CREATE TABLE shard2.customer ( id INT PRIMARY KEY, city VARCHAR(50), email VARCHAR(100) ) ENGINE=InnoDB;
In the above example, the “customer” table is vertically sharded. Shard 1 contains the “id”, “name”, and “age” columns, while shard 2 contains the “id”, “city”, and “email” columns.
Snippet 2: Inserting data into a vertically sharded table
-- Inserting into shard 1's customer table INSERT INTO shard1.customer (id, name, age) VALUES (1, 'John Doe', 30); -- Inserting into shard 2's customer table INSERT INTO shard2.customer (id, city, email) VALUES (2, 'New York', 'john@example.com');
Snippet 3: Querying data from a vertically sharded table
-- Querying from shard 1's customer table SELECT * FROM shard1.customer WHERE id = 1; -- Querying from shard 2's customer table SELECT * FROM shard2.customer WHERE id = 2;
Vertical sharding allows for partitioning columns vertically, which can be useful when different sets of columns have different access patterns or growth rates. It allows for efficient storage and retrieval of data based on the columns being accessed.
Using Horizontal Sharding in MySQL
Horizontal sharding, also known as row-based sharding, is a technique where rows of a table are partitioned horizontally across multiple shards. Each shard contains a subset of rows for the table. Let’s see how horizontal sharding can be implemented in MySQL.
Snippet 1: Creating a horizontally sharded table
-- Shard 1 CREATE TABLE shard1.order ( id INT PRIMARY KEY, customer_id INT, product_id INT, quantity INT ) ENGINE=InnoDB; -- Shard 2 CREATE TABLE shard2.order ( id INT PRIMARY KEY, customer_id INT, product_id INT, quantity INT ) ENGINE=InnoDB;
In the above example, the “order” table is horizontally sharded. Shard 1 contains a subset of rows for the table, while shard 2 contains another subset of rows.
Snippet 2: Inserting data into a horizontally sharded table
-- Inserting into shard 1's order table INSERT INTO shard1.order (id, customer_id, product_id, quantity) VALUES (1, 123, 456, 2); -- Inserting into shard 2's order table INSERT INTO shard2.order (id, customer_id, product_id, quantity) VALUES (2, 456, 789, 1);
Snippet 3: Querying data from a horizontally sharded table
-- Querying from shard 1's order table SELECT * FROM shard1.order WHERE id = 1; -- Querying from shard 2's order table SELECT * FROM shard2.order WHERE id = 2;
Horizontal sharding allows for partitioning rows horizontally, which can be useful when the dataset is large and needs to be distributed across multiple shards. It allows for efficient storage and retrieval of data based on the rows being accessed.
Using Consistent Hashing for Sharding in MySQL
Consistent hashing is a sharding technique that provides a scalable and balanced distribution of data across shards. It minimizes the data redistribution required when adding or removing shards from the system. Let’s see how consistent hashing can be used for sharding in MySQL.
Snippet 1: Implementing consistent hashing algorithm
from hashlib import md5 class ConsistentHashing: def __init__(self, nodes, replicas=3): self.nodes = [] self.replicas = replicas for node in nodes: for i in range(replicas): key = self.hash(f"{node}-{i}") self.nodes.append((key, node)) self.nodes.sort() def hash(self, key): return int(md5(key.encode()).hexdigest(), 16) def get_node(self, key): if not self.nodes: return None hashed_key = self.hash(key) for node_key, node in self.nodes: if hashed_key <= node_key: return node return self.nodes[0][1]
In the above example, we implement a simple consistent hashing algorithm using the MD5 hash function. The algorithm distributes nodes across the hash space and maps keys to the closest node in a clockwise direction.
Snippet 2: Using consistent hashing for sharding
nodes = ["shard1", "shard2", "shard3"] hashing = ConsistentHashing(nodes) # Distribute data across shards data = ["key1", "key2", "key3"] for key in data: shard = hashing.get_node(key) print(f"Key: {key} -> Shard: {shard}")
In this example, we create three shards (“shard1”, “shard2”, “shard3”) and distribute data keys (“key1”, “key2”, “key3”) across the shards using consistent hashing. The output shows which shard each key is assigned to.
Consistent hashing provides a scalable and balanced sharding approach, ensuring minimal data redistribution when adding or removing shards from the system.
Related Article: How to Resolve Secure File Priv in MySQL
Introduction to Third-Party Tools for Sharding in MySQL
In addition to built-in features and techniques, several third-party tools and frameworks are available to simplify the implementation and management of sharding in MySQL. Let’s explore some of these tools.
1. Vitess: Vitess is an open-source database clustering system for MySQL. It provides features for automatic sharding, query routing, and horizontal scalability. Vitess is used by several large-scale applications, including YouTube.
2. ProxySQL: ProxySQL is a high-performance proxy for MySQL. It offers features like query routing, load balancing, and connection pooling. ProxySQL can be used in a sharded environment to distribute queries across shards and improve performance.
3. ScaleArc: ScaleArc is a database load balancing and caching software. It supports sharding in MySQL by providing transparent routing of queries to the appropriate shard. ScaleArc also offers features like connection pooling, query caching, and failover.
4. Shard-Query: Shard-Query is a MySQL query router and sharding library. It provides a SQL interface to query data across multiple shards and supports various sharding techniques. Shard-Query can be used to build custom sharding solutions.
5. MyCAT: MyCAT (MySQL Cluster Autonomic Tuning) is an open-source database clustering and sharding solution. It supports automatic data sharding, load balancing, and failover. MyCAT also provides features like distributed transactions and high availability.
These are just a few examples of third-party tools available for sharding in MySQL. Each tool has its own features and capabilities, so it is essential to evaluate them based on your specific requirements and use case.
Comparing Third-Party Tools for Sharding in MySQL
When choosing a third-party tool for sharding in MySQL, it is important to compare their features, performance, ease of use, and community support. Let’s compare three popular tools: Vitess, ProxySQL, and ScaleArc.
1. Vitess:
– Features: Vitess provides automatic sharding, query routing, and horizontal scalability. It offers features like connection pooling, load balancing, and high availability.
– Performance: Vitess is known for its high performance and scalability. It is used by large-scale applications like YouTube, which handle massive amounts of data and high traffic.
– Ease of use: Vitess requires some initial setup and configuration. It has a learning curve but provides comprehensive documentation and community support.
– Community support: Vitess has an active and growing community. It is backed by PlanetScale, a company focused on supporting and enhancing Vitess.
2. ProxySQL:
– Features: ProxySQL provides query routing, load balancing, and connection pooling. It is designed for high-performance query handling and can be used in a sharded environment.
– Performance: ProxySQL is known for its excellent performance and low latency. It efficiently routes queries to the appropriate shard, improving overall system performance.
– Ease of use: ProxySQL is relatively easy to set up and configure. It can be integrated into an existing MySQL infrastructure without significant changes.
– Community support: ProxySQL has an active community and is widely used in production environments. It has comprehensive documentation and community support channels.
3. ScaleArc:
– Features: ScaleArc provides database load balancing, query routing, and caching. It supports transparent sharding in MySQL and offers features like connection pooling and failover.
– Performance: ScaleArc improves query performance by caching frequently accessed data and routing queries to the appropriate shard. It is designed for high availability and scalability.
– Ease of use: ScaleArc is relatively easy to set up and configure. It provides a user-friendly GUI for managing the sharded MySQL environment.
– Community support: ScaleArc has an active community and provides comprehensive documentation and support resources.
When comparing these tools, consider factors like your specific requirements, performance needs, ease of use, and community support. Evaluate the features and capabilities of each tool to determine the best fit for your sharding implementation in MySQL.
Advanced Sharding Techniques for MySQL
In addition to the basic sharding techniques discussed earlier, there are several advanced sharding techniques that can be used to address specific requirements and challenges. Let’s explore some of these advanced sharding techniques for MySQL.
1. Cross-shard queries: Cross-shard queries involve joining or aggregating data across multiple shards. These queries can be challenging to execute efficiently due to the distributed nature of data. Techniques like scatter-gather and parallel execution can be used to optimize cross-shard queries.
2. Multi-key sharding: Multi-key sharding involves sharding data based on multiple keys. It allows for more flexible distribution of data and can be useful when data needs to be partitioned based on multiple criteria simultaneously.
3. Hybrid sharding: Hybrid sharding combines multiple sharding techniques to achieve the desired scalability and performance. For example, a system can use range sharding for one table and hash sharding for another, depending on the access patterns and data characteristics.
4. Dynamic sharding: Dynamic sharding involves dynamically redistributing data across shards based on changing access patterns or workload. It allows for adaptive scaling and load balancing in a sharded environment.
5. Transparent sharding: Transparent sharding aims to hide the complexity of sharding from the application layer. It provides a unified interface to the application, abstracting the details of data distribution and query routing.
6. Shard merging and splitting: Shard merging combines multiple shards into a single shard, reducing the number of shards in the system. Shard splitting, on the other hand, divides a shard into multiple smaller shards. These techniques can be used to optimize data distribution and manage system resources.
These advanced sharding techniques require careful planning and implementation. They can address specific challenges and provide more flexibility and scalability in a sharded MySQL environment.
Related Article: Securing MySQL Access through Bash Scripts in Linux
Optimizing Query Performance in a Sharded MySQL Database
Query performance is crucial in a sharded MySQL database to ensure fast response times and efficient data retrieval. Here are some tips for optimizing query performance in a sharded MySQL database:
1. Use appropriate indexes: Analyze query patterns and create indexes on the columns frequently used in queries. Indexes can significantly improve query performance by allowing for faster data retrieval.
2. Minimize cross-shard queries: Cross-shard queries involve joining or aggregating data across multiple shards and can be expensive in terms of performance. Minimize the need for cross-shard queries by designing the schema and access patterns accordingly.
3. Denormalize data when necessary: In a sharded environment, joining tables across shards can impact performance. Consider denormalizing data by duplicating it across shards to reduce the need for joins.
4. Optimize query execution plans: Regularly analyze query execution plans and optimize them for better performance. Use tools like MySQL’s EXPLAIN statement to understand how queries are executed and identify potential bottlenecks.
5. Implement query caching: Implement a caching mechanism, such as Redis or Memcached, to cache frequently executed queries and their results. This can significantly improve query response times and reduce the load on the database.
6. Monitor and tune performance: Regularly monitor the performance of the sharded MySQL database using tools like MySQL’s performance schema or third-party monitoring tools. Identify performance bottlenecks and tune the database configuration, query execution plans, or indexing strategies accordingly.
7. Consider data locality: When designing the sharding strategy, consider the data locality principle. Place data that is frequently accessed together on the same shard to reduce the need for cross-shard queries.
8. Use connection pooling: Implement connection pooling to minimize the overhead of establishing database connections. Connection pooling allows for reusing existing connections, reducing the connection establishment time.
Managing Data Consistency in a Sharded MySQL Database
Ensuring data consistency in a sharded MySQL database can be challenging due to the distributed nature of data. However, there are strategies and techniques that can help manage data consistency effectively. Here are some approaches to consider:
1. Distributed transactions: Use distributed transaction frameworks like XA transactions or two-phase commit protocols to maintain data consistency across multiple shards. These frameworks coordinate the commit or rollback of transactions across all participating shards, ensuring that data remains consistent.
2. Eventual consistency: Consider relaxing immediate consistency requirements and adopting an eventual consistency model. In this model, updates to different shards are applied asynchronously, and consistency is achieved over time. This approach can improve system performance and scalability.
3. Data replication: Replicate data across multiple shards to ensure redundancy and availability. MySQL’s built-in replication features can be used to replicate data between primary and replica shards, providing failover and data consistency.
4. Conflict resolution: Define conflict resolution strategies to handle conflicts that may arise when updating data across multiple shards. Techniques like last write wins or conflict detection mechanisms can be used to resolve conflicts and maintain data integrity.
5. Change data capture: Use change data capture mechanisms to capture and propagate changes made to data across multiple shards. This allows other shards to be notified and update their data accordingly, ensuring data consistency.
6. Data validation and reconciliation: Regularly validate and reconcile data across shards to identify any inconsistencies or data corruption. Implement automated data validation scripts or use third-party tools that compare data across shards and detect discrepancies.
7. Monitoring and alerting: Set up monitoring tools to detect data consistency issues and anomalies. Implement alerting mechanisms to notify administrators when data inconsistencies are detected, allowing for timely resolution.
Implementing these strategies and techniques can help manage data consistency effectively in a sharded MySQL database. It is essential to carefully design the sharding strategy and choose the appropriate tools and frameworks to ensure data integrity and consistency.
Replication and Failover Strategies for Sharded MySQL Databases
Replication and failover strategies are crucial for ensuring high availability and fault tolerance in a sharded MySQL database. Let’s explore some strategies for replication and failover in a sharded MySQL environment.
1. Shard-level replication: Implement replication at the shard level by configuring primary and replica shards. Each shard can have one or more replica shards that replicate data from the primary shard. This provides redundancy and failover capabilities at the shard level.
2. Global replication: In addition to shard-level replication, implement global replication to replicate data across all shards. This provides an additional layer of redundancy and ensures that data is available even if an entire shard fails.
3. Automatic failover: Implement automatic failover mechanisms to detect and handle shard failures. When a primary shard fails, the failover mechanism should promote one of the replica shards as the new primary shard. This ensures uninterrupted service and minimal downtime.
4. Load balancing: Use load balancing mechanisms to distribute requests across available shards. Load balancers can monitor the health of shards and route requests to healthy shards. This helps distribute the workload and maintain high availability.
5. Monitoring and alerting: Set up monitoring tools to detect shard failures and performance issues. Implement alerting mechanisms to notify administrators when a shard fails or experiences abnormal behavior. This allows for prompt action and resolution.
6. Data redundancy: Ensure that data is replicated across multiple shards to provide redundancy. Replication can be synchronous or asynchronous, depending on the requirements. Synchronous replication ensures data consistency but may impact performance, while asynchronous replication provides better performance but may introduce some data lag.
7. Regular backups: Implement regular backups of the data on each shard. Backups should be stored in a separate location to ensure data availability even in the event of a catastrophic failure. Regularly test the backup and restore process to ensure its reliability.