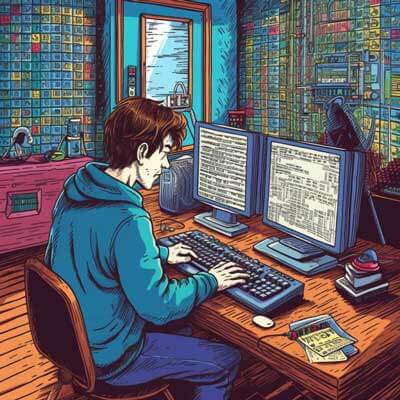
- Getting Started with Docker Containers
- What is a Docker Container?
- Installing Docker
- Working with Docker Images
- Running Your First Container
- Understanding Container Security
- 1. Isolation
- 2. Image Security
- 3. Container Hardening
- 4. Network Security
- 5. Continuous Monitoring and Auditing
- Securing Docker Images
- Best Practices for Container Configuration
- Implementing Access Controls and User Permissions
- 1. Use the principle of least privilege
- 2. Utilize Docker’s user namespace feature
- 3. Use Docker secrets for sensitive information
- 4. Implement network segmentation
- 5. Regularly update and patch Docker
- Monitoring and Logging for Container Security
- Securing Network Communication in Docker
- Protecting Container Hosts
- Securing Container Storage
- Hardening Docker for Production
- Managing Secrets in Docker Containers
- Using Security Tools and Plugins
- Docker Security Scanning
- Docker Bench for Security
- Docker Content Trust
- Third-Party Security Plugins
- Real World Examples of Docker Container Security
- Advanced Techniques for Securing Docker Containers
Getting Started with Docker Containers
Docker has quickly become the go-to solution for deploying applications in a lightweight and scalable manner. With its containerization technology, Docker allows developers to package their applications along with all their dependencies into a single, portable unit called a container. This makes it easy to run applications consistently across different environments, from development to production.
In this chapter, we will cover the basic concepts of Docker containers and provide step-by-step instructions on how to get started with Docker.
Related Article: How to Pass Environment Variables to Docker Containers
What is a Docker Container?
A Docker container is a lightweight, standalone executable package that includes everything needed to run a piece of software, including the code, runtime, system tools, and system libraries. It provides an isolated environment for running applications, ensuring that they are consistent and run the same way regardless of the underlying infrastructure.
Unlike virtual machines, which require a separate operating system for each instance, Docker containers share the host machine’s operating system kernel. This makes containers much more lightweight and faster to start and stop compared to traditional virtualization.
Installing Docker
Before you can start using Docker, you need to install it on your machine. Docker provides installers for various operating systems, including Windows, macOS, and Linux. You can find the installation instructions for your specific operating system on the official Docker website: https://www.docker.com/products/docker-desktop.
Once Docker is installed, you can verify the installation by opening a terminal or command prompt and running the following command:
docker version
This command should display the version of Docker installed on your machine, along with information about the Docker client and server.
Working with Docker Images
Docker images are the building blocks of containers. An image is a read-only template that contains all the instructions necessary to create a container. You can think of an image as a snapshot of a Docker container’s filesystem and configuration at a specific point in time.
To start using Docker, you need to pull an image from a Docker registry. Docker Hub is the default public registry for Docker images, and it hosts a vast collection of pre-built images for popular software stacks and applications.
To pull an image from Docker Hub, use the following command:
docker pull image_name:tag
For example, to pull the latest version of the official nginx image, you can run:
docker pull nginx:latest
This command will download the nginx image from Docker Hub and store it locally on your machine.
Related Article: Build a Movie Search App with GraphQL, Node & TypeScript
Running Your First Container
Once you have pulled an image, you can run a container based on that image. To start a new container, use the following command:
docker run image_name
For example, to run a new container based on the nginx image, you can run:
docker run nginx
This command will start a new container based on the nginx image and attach your terminal to its standard input, output, and error streams. You should see the nginx welcome page displayed in your terminal.
To run a container in the background, you can add the -d
flag:
docker run -d nginx
This command will start the container in detached mode, allowing you to continue using your terminal without being attached to the container’s input and output.
Understanding Container Security
Containerization has become an increasingly popular method for deploying and managing applications. Docker, one of the most widely used container platforms, offers a high level of flexibility and scalability. However, with this increased flexibility comes a need for heightened security measures to protect your applications and data.
In this chapter, we will delve into the fundamentals of container security and explore some best practices to ensure the safety of your Docker containers.
1. Isolation
One of the key aspects of container security is isolation. Docker containers provide a level of isolation by leveraging kernel namespaces and control groups. Namespaces ensure that each container has its own isolated view of the operating system, including its own network stack, process tree, and filesystem. Control groups, on the other hand, allow you to allocate resources and limit the usage of CPU, memory, and other system resources.
By default, containers running on the same host share the same kernel, which means that a vulnerability in one container could potentially impact others. It is essential to regularly update your kernel and container runtime to patch any security vulnerabilities and reduce the risk of container breakout.
Related Article: Tutorial: Managing Docker Secrets
2. Image Security
When working with Docker, you often start by pulling base images from Docker Hub or other registries. It is crucial to ensure that these base images come from trusted sources and are regularly updated. A compromised or outdated base image can pose a significant security risk to your containers.
To mitigate this risk, follow these best practices:
– Only use trusted base images from reputable sources.
– Regularly update your base images to include the latest security patches.
– Scan your images for vulnerabilities using tools like Docker Security Scanning or third-party vulnerability scanners.
Here’s an example of how you can pull a trusted base image and update it regularly in your Dockerfile:
FROM alpine:latest RUN apk update && apk upgrade ...
3. Container Hardening
In addition to using trusted base images, it is essential to harden your containers to minimize the attack surface. Here are some best practices for container hardening:
– Remove unnecessary packages and dependencies from your containers.
– Use minimal and purpose-built base images to reduce the attack surface.
– Limit container privileges by running them with the principle of least privilege.
– Disable or restrict container capabilities that are not required.
– Use read-only file systems whenever possible to prevent unauthorized modifications.
4. Network Security
Proper network security is vital for protecting your Docker containers. By default, Docker containers can communicate with each other and the host system. However, you should carefully configure network access to minimize the risk of unauthorized access and data breaches.
Consider these best practices for network security:
– Use Docker’s built-in network isolation features to restrict container-to-container communication.
– Bind containers to specific host interfaces to control network access.
– Apply firewall rules and network segmentation to further secure container communication.
– Regularly monitor network traffic and use intrusion detection systems (IDS) to detect potential threats.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
5. Continuous Monitoring and Auditing
Securing your Docker containers is an ongoing process. Regularly monitoring and auditing your container environment helps detect and respond to security incidents promptly.
Consider implementing these best practices for continuous monitoring and auditing:
– Monitor container logs and system metrics to identify any suspicious activities or deviations from normal behavior.
– Use security information and event management (SIEM) tools to centralize and analyze logs for potential security incidents.
– Regularly perform vulnerability scans and penetration tests to identify and address any vulnerabilities in your container environment.
– Implement container-specific security tools, such as Docker Bench for Security, to automate security checks and ensure compliance with best practices.
In the next chapter, we will explore how to secure container images and the build pipeline. Stay tuned for more insights and practical tips on securing your Docker containers.
Securing Docker Images
When it comes to securing your Docker containers, one of the first steps is to secure the Docker images themselves. Docker images are the building blocks of containers, and ensuring their integrity is crucial to keeping your applications safe.
Here are some simple steps you can take to secure your Docker images:
1. Use Official and Trusted Base Images: When building your Docker images, it’s important to start with official and trusted base images provided by Docker or reputable organizations. These images are regularly updated and undergo thorough security testing. Avoid using unofficial or untrusted base images, as they may contain vulnerabilities or malicious code.
2. Keep Your Base Images Up to Date: Ensure that you regularly update your base images to the latest versions. New vulnerabilities are discovered all the time, and updating your base images helps protect against known security issues. You can use the docker pull
command to update your base images:
docker pull :
3. Scan for Vulnerabilities: Use vulnerability scanning tools to identify any known vulnerabilities in your Docker images. Tools like Clair, Trivy, or Anchore can scan your images for vulnerabilities and provide detailed reports. You can integrate these tools into your CI/CD pipeline to automatically scan images before deployment.
4. Minimize Image Size: Large Docker images not only take longer to build and deploy but also increase the attack surface. It’s essential to keep your images as small as possible by removing unnecessary packages, dependencies, and files. This helps reduce the potential for vulnerabilities and improves overall security.
5. Use Multi-Stage Builds: Docker supports multi-stage builds, which allow you to separate the build and runtime environments. By using multi-stage builds, you can ensure that your final image only includes the necessary components, reducing the attack surface and improving security.
Here’s an example of a Dockerfile using multi-stage builds:
# Build Stage FROM golang:1.16 as build-stage WORKDIR /app COPY . . RUN go build -o myapp # Final Stage FROM alpine:latest WORKDIR /app COPY --from=build-stage /app/myapp . CMD ["./myapp"]
6. Sign and Verify Images: Docker Content Trust (DCT) is a security feature that uses digital signatures to verify the authenticity and integrity of Docker images. Enabling DCT ensures that only signed images are pulled and run on your Docker hosts. You can enable DCT by setting the DOCKER_CONTENT_TRUST
environment variable to 1
:
export DOCKER_CONTENT_TRUST=1
7. Store Images in Private Repositories: If possible, store your Docker images in private repositories rather than public ones. Private repositories provide an additional layer of security by restricting access to authorized users only. Docker Hub offers private repositories, and you can also use private registry solutions like Harbor or Nexus.
By following these simple steps, you can significantly enhance the security of your Docker images and protect your applications from potential vulnerabilities and attacks. Remember that securing Docker containers is an ongoing process, and it’s important to stay up to date with the latest security best practices.
Next, we will explore how to secure Docker containers at runtime.
Best Practices for Container Configuration
When it comes to securing Docker containers, one of the most important steps is to properly configure them. Misconfigurations can lead to vulnerabilities that can be exploited by attackers. In this chapter, we will discuss some best practices for container configuration to help you protect your applications.
1. Use a Minimal Base Image: Start with a minimal base image to reduce the attack surface. Avoid using images that come with unnecessary packages or services. Alpine Linux is a popular choice for its small size and security-focused design.
2. Update the Base Image Regularly: Keep your base image up to date with the latest security patches. Vulnerabilities in the base image can trickle down to your application. Regularly check for updates and rebuild your container with the updated image.
3. Run Containers as Non-Root: By default, Docker containers run as the root user, which can be risky. Create a non-root user inside the container and run your application as that user instead. This reduces the potential impact of a container compromise.
Here’s an example of a Dockerfile that creates a non-root user:
FROM alpine:latest RUN addgroup -S mygroup && adduser -S myuser -G mygroup USER myuser
4. Limit Container Capabilities: Docker containers inherit the capabilities of the host system by default. This means that a compromised container can potentially access sensitive resources on the host. To mitigate this, restrict the capabilities of your containers using the --cap-drop
flag when running the container.
For example, to drop the SYS_PTRACE
capability:
docker run --cap-drop=SYS_PTRACE my-container
5. Use Environment Variables for Sensitive Information: Avoid hardcoding sensitive information like passwords or API keys directly into your container image. Instead, use environment variables to pass this information at runtime. This allows for easier management and avoids exposing secrets in the image or Dockerfile.
6. Enable Content Trust: Docker Content Trust ensures the integrity and authenticity of images by using digital signatures. Enable it by setting the environment variable DOCKER_CONTENT_TRUST
to 1
. This prevents the use of unsigned or tampered images, reducing the risk of running malicious code.
export DOCKER_CONTENT_TRUST=1
7. Limit Resource Usage: Limit the resources that a container can use to prevent resource exhaustion attacks. Set resource limits such as CPU and memory usage using Docker’s resource constraints.
For example, to limit a container to 1 CPU and 512MB of memory:
docker run --cpus=1 --memory=512m my-container
By following these best practices for container configuration, you can significantly enhance the security of your Docker containers and protect your applications from potential threats. Remember to regularly review and update your configurations as new security risks emerge.
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
Implementing Access Controls and User Permissions
One of the key aspects of securing Docker containers is implementing access controls and user permissions. By properly managing user access to containers and their resources, you can significantly reduce the risk of unauthorized access and potential security breaches.
Here are some simple steps you can take to implement access controls and user permissions in your Docker environment:
1. Use the principle of least privilege
Follow the principle of least privilege when assigning user permissions. This means giving users only the minimum level of access required for them to perform their tasks. By limiting access to only what is necessary, you can reduce the potential impact of any security vulnerabilities.
For example, instead of running containers with root privileges, create dedicated user accounts with restricted permissions for each container. This way, even if a container is compromised, the attacker’s access will be limited to that specific container.
2. Utilize Docker’s user namespace feature
Docker provides a feature called user namespaces, which allows you to map container users to different user IDs (UIDs) and group IDs (GIDs) on the host system. This provides an additional layer of isolation and security.
To enable user namespaces, add the following configuration to your Docker daemon configuration file (/etc/docker/daemon.json
):
{ "userns-remap": "default" }
This will configure Docker to use the default user namespace mapping. You can also create custom mappings if needed.
Related Article: How to Force Docker for a Clean Build of an Image
3. Use Docker secrets for sensitive information
Avoid hardcoding sensitive information, such as passwords or API keys, directly into your Docker images or container configurations. Instead, use Docker secrets to securely store and manage this sensitive data.
Docker secrets are encrypted pieces of data that can be securely passed to a container during runtime. They are stored in a designated location on the host system and are only accessible by authorized containers.
To use Docker secrets, first create the secret:
$ echo "mysecretpassword" | docker secret create db_password -
Then, in your container configuration, reference the secret:
version: '3' services: db: image: mysql secrets: - db_password
This way, the sensitive password is not exposed in the container’s configuration file or environment variables.
4. Implement network segmentation
Consider implementing network segmentation to isolate containers and restrict communication between them. By separating containers into different networks, you can control the flow of network traffic and reduce the attack surface.
Docker provides network drivers that allow you to create custom networks and define specific rules for traffic between containers. For example, you can create a separate network for your database containers and only allow access from specific authorized containers.
To create a custom network, use the following Docker command:
$ docker network create mynetwork
Then, when starting a container, specify the network:
$ docker run --network=mynetwork mycontainer
5. Regularly update and patch Docker
Keeping your Docker installation up to date is crucial for security. Docker regularly releases updates that include security fixes and improvements. By regularly updating Docker, you can ensure that you have the latest security patches applied.
To update Docker, use the following command:
$ sudo apt-get update && sudo apt-get upgrade docker-ce
It is also recommended to subscribe to Docker’s security announcements and follow best practices for securing your Docker environment.
By implementing these access controls and user permissions, you can enhance the security of your Docker containers and protect your applications from potential threats. Remember to always follow best practices and stay proactive in keeping your Docker environment secure.
Related Article: How to Stop and Remove All Docker Containers
Monitoring and Logging for Container Security
Monitoring and logging are essential components of container security. By actively monitoring and logging container activities, you can detect and respond to potential security threats in real-time. This chapter will explore some best practices for monitoring and logging in Docker containers.
1. Monitor Container Activity
Monitoring container activity allows you to gain visibility into the behavior of your containers. By tracking metrics such as CPU usage, memory consumption, and network traffic, you can identify abnormal patterns or potential security breaches.
Docker provides various monitoring options, including the built-in Docker Stats command and the Docker Remote API. Additionally, you can use third-party tools like Prometheus and Grafana to collect and visualize container metrics.
Here’s an example of using Docker Stats to monitor a running container:
$ docker stats
2. Enable Container Logging
Enabling container logging is crucial for capturing and analyzing container-related events. By logging container activities, you can trace and investigate potential security incidents, troubleshoot issues, and comply with regulatory requirements.
Docker supports various logging drivers, including the default JSON file driver, syslog, and third-party drivers like Fluentd and Logstash. You can specify the logging driver when starting a container using the --log-driver
flag.
Here’s an example of starting a container with the Fluentd logging driver:
$ docker run --log-driver=fluentd
3. Centralize Container Logs
To effectively manage and analyze container logs, it’s recommended to centralize them in a dedicated log management system. Centralized logging allows for easier search, analysis, and correlation of logs across multiple containers and hosts.
Popular log management tools like ELK Stack (Elasticsearch, Logstash, and Kibana) and Splunk can be used to collect, index, and visualize container logs. These tools provide powerful search capabilities, real-time alerting, and advanced analytics for container security monitoring.
Here’s an example of configuring a Docker container to send logs to an Elasticsearch instance:
$ docker run --log-driver=fluentd --log-opt fluentd-address=:
4. Implement Log Rotation
Log rotation is essential to prevent logs from consuming excessive disk space and slowing down container performance. Docker supports log rotation for the default JSON file logging driver, allowing you to limit the size and number of log files.
You can configure log rotation by specifying the maximum file size and the maximum number of log files using the --log-opt
flag when starting a container.
Here’s an example of setting the maximum file size to 10MB and the maximum number of log files to 5:
$ docker run --log-opt max-size=10m --log-opt max-file=5
5. Analyze Container Logs
Once you have collected and centralized container logs, it’s important to analyze them for security insights. By leveraging log analysis techniques, you can detect suspicious activities, identify potential vulnerabilities, and proactively respond to security incidents.
Tools like Elasticsearch, Logstash, and Kibana (ELK Stack) provide powerful log analysis capabilities. You can use their query and visualization features to search for specific log events, create dashboards for real-time monitoring, and set up alerts for security-related patterns.
In conclusion, monitoring and logging are crucial for container security. By actively monitoring container activity and enabling comprehensive logging, you can enhance the security posture of your Docker containers and quickly respond to potential threats or incidents.
Securing Network Communication in Docker
Docker containers are designed to be lightweight and isolated, allowing you to run applications in a secure environment. However, by default, containers can communicate with each other and the host system without any restrictions. This can pose a security risk, as an attacker who gains control of one container can potentially access and compromise other containers or even the host system.
To mitigate this risk, it is important to secure the network communication within your Docker environment. In this section, we will explore some simple steps you can take to protect your applications.
1. Use Bridge Networks
By default, Docker containers are connected to a default bridge network, which allows them to communicate with each other using IP addresses. However, this can expose your containers to potential attacks. To enhance security, you can create your own bridge network and isolate your containers within it.
To create a bridge network, you can use the following Docker command:
$ docker network create my-bridge-network
Then, when running your containers, you can specify the network using the --network
flag:
$ docker run --network=my-bridge-network my-container
This will ensure that your containers can only communicate with each other through the specified network.
2. Limit Network Access
Another important step in securing network communication is to limit the network access of your containers. By default, containers can access the network of the host system and other containers. However, you can restrict this access by using Docker’s built-in firewall rules.
For example, you can use the --publish
or -p
flag to specify which ports should be exposed from the container to the host system. Only the specified ports will be accessible from outside the container.
$ docker run -p 8080:80 my-container
In this example, the container’s port 80 is exposed on the host system’s port 8080. This allows external traffic to access the container’s web server, while keeping other ports inaccessible.
3. Encrypt Network Traffic
To further enhance the security of your Docker environment, you can encrypt the network traffic between containers and the host system. This prevents attackers from intercepting and tampering with sensitive data.
One way to achieve this is by using Docker’s built-in support for Transport Layer Security (TLS). By enabling TLS, you can secure the communication between Docker clients and the Docker daemon.
To enable TLS, you need to generate TLS certificates and configure Docker to use them. The process may vary depending on your operating system and Docker version. Docker provides detailed documentation on how to generate and configure TLS certificates for different platforms, which you can find here.
Once TLS is enabled, all communication between Docker clients and the Docker daemon will be encrypted, providing an extra layer of security.
4. Regularly Update Docker
Finally, it is crucial to keep your Docker installation up to date with the latest security patches and updates. Docker regularly releases new versions that address security vulnerabilities and improve the overall security of the platform.
You can update Docker by running the following command:
$ sudo apt update && sudo apt upgrade docker-ce
By regularly updating Docker, you ensure that your containers are protected against the latest security threats.
In this section, we explored several simple steps you can take to secure the network communication within your Docker environment. By creating bridge networks, limiting network access, encrypting network traffic, and keeping Docker up to date, you can significantly enhance the security of your Docker containers and protect your applications from potential attacks.
Protecting Container Hosts
When working with Docker containers, it is crucial to ensure the security of the host system that runs these containers. A compromised host can lead to unauthorized access, data breaches, and potentially the compromise of all containers running on that host. This chapter will discuss some simple steps you can take to protect your container hosts.
1. Regularly Update the Host Operating System
Keeping your host operating system up to date is one of the most important steps to ensure the security of your container environment. Regularly applying security patches and updates helps protect against known vulnerabilities. Consider setting up automatic updates or implementing a regular update schedule to ensure you are always running the latest security patches.
2. Enable and Configure Firewalls
Firewalls play a crucial role in securing your container host. By controlling network traffic, firewalls can prevent unauthorized access to your host and container environments. Configure your firewall to only allow necessary incoming and outgoing connections on the required ports, and block all other traffic. Additionally, consider using network segmentation to isolate your container host from other parts of your network.
3. Implement Strong User Authentication and Access Controls
Proper user authentication and access controls are vital to securing your container host. Use strong passwords or, preferably, implement SSH key-based authentication to prevent brute-force attacks on user accounts. Disable root login via SSH and create separate user accounts with limited privileges for managing your container environment. Additionally, regularly review and update user access controls to ensure only authorized individuals have access to your container host.
4. Isolate Containers
Isolating containers from each other and the host system adds an extra layer of security to your container environment. Docker provides several ways to achieve this, such as using separate user namespaces, limiting container capabilities, and implementing resource constraints. By isolating containers, you minimize the impact of a compromised container on the host system and other containers.
5. Monitor and Log Activities
Implementing proper monitoring and logging practices helps you identify and respond to potential security incidents. Monitor your container host for any suspicious activities, such as unauthorized access attempts or unusual resource usage. Enable logging for both host and container activities, and regularly review logs to detect any anomalies. Consider using tools like the ELK stack (Elasticsearch, Logstash, and Kibana) to centralize and analyze logs from multiple sources.
6. Regularly Backup and Test
Regularly backing up your container host and associated data is essential to protect against data loss or system compromise. Ensure your backups are encrypted and securely stored. Additionally, regularly test your backup and restoration processes to ensure they are working correctly in case of a disaster.
By following these simple steps, you can enhance the security of your container hosts and protect your applications running within them. However, it is important to note that security is an ongoing process, and it is crucial to stay up to date with the latest security practices and vulnerabilities in order to adapt and improve your security measures.
Related Article: How to Use Environment Variables in Docker Compose
Securing Container Storage
Securing container storage is an important consideration when it comes to protecting your applications. Docker provides several features and best practices to help you secure your container storage effectively.
1. Use Volume Encryption: Docker allows you to encrypt your container volumes to protect sensitive data. By using encrypted volumes, you can ensure that even if someone gains unauthorized access to the storage, they won’t be able to read the data without the encryption key.
To create an encrypted volume, you can use the --opt encrypted
flag when creating a volume. For example:
docker volume create --driver local --opt encrypted my-encrypted-volume
2. Limit Access to Volumes: It is essential to restrict access to container volumes to only authorized users or containers. By default, Docker volumes are mounted in a way that allows all containers on the host to access them. To prevent unauthorized access, you should explicitly specify the containers that are allowed to access the volumes.
For example, you can create a volume and specify the allowed containers using labels:
docker volume create --driver local --label com.example.allowed-containers=my-container my-volume
3. Use Persistent Volumes: Docker provides a feature called Persistent Volumes that allows you to manage storage for your containers separately from the container lifecycle. Persistent Volumes are not automatically deleted when containers are removed, ensuring that your data is preserved even if a container is recreated or deleted.
To use Persistent Volumes, you need to define a Persistent Volume Claim (PVC) and attach it to your containers. Here’s an example of a PVC definition:
apiVersion: v1 kind: PersistentVolumeClaim metadata: name: my-pvc spec: accessModes: - ReadWriteOnce resources: requests: storage: 1Gi
4. Implement Role-Based Access Control (RBAC): RBAC allows you to define fine-grained access controls for Docker volumes. By using RBAC, you can specify which users or groups have read or write access to specific volumes.
Docker provides an RBAC plugin called docker-authz-plugin
that you can use to implement RBAC for container storage. You can find more information about the plugin and how to use it in the Docker documentation.
5. Regularly Update and Patch: Keeping your Docker installation up to date with the latest security patches is crucial for securing container storage. Make sure to regularly update Docker and its dependencies to benefit from the latest security improvements and bug fixes.
Additionally, regularly monitor Docker security advisories and apply any relevant patches or updates promptly.
By following these best practices and using Docker’s built-in features, you can significantly enhance the security of your container storage and protect your applications from unauthorized access or data breaches. Remember that securing container storage is just one aspect of overall container security, and it’s important to implement a comprehensive security strategy to ensure the safety of your applications.
Hardening Docker for Production
Docker provides a secure containerization solution, but to ensure the highest level of security for your production environment, it is important to harden your Docker setup. By following these steps, you can minimize potential vulnerabilities and protect your applications from attacks.
1. Use Official Images
When building your Docker images, it is recommended to use official images from the Docker Hub or other trusted sources. Official images are regularly maintained, have undergone security reviews, and are less likely to contain vulnerabilities. Always check the Docker Hub repository for the official image before using a third-party one.
FROM node:14-alpine
2. Update Regularly
Docker releases updates frequently, and these updates often include important security fixes. To ensure you are running the latest secure version, regularly update both Docker and your base images.
$ docker pull node:14-alpine
3. Limit Privileges
By default, Docker containers run with root privileges, which can be risky. To minimize the impact of a potential attack, it is recommended to run containers with non-root users and limit their privileges.
Within your Dockerfile, create a new user and switch to it:
RUN adduser -D myuser USER myuser
4. Enable Docker Content Trust
Docker Content Trust (DCT) is a security feature that provides cryptographic verification of the authenticity and integrity of Docker images. When enabled, only signed images can be pulled and run on your Docker host. Enable DCT by setting the DOCKER_CONTENT_TRUST
environment variable to 1
.
$ export DOCKER_CONTENT_TRUST=1
5. Restrict Container Capabilities
Docker containers inherit all capabilities from the host kernel by default. To minimize the risk of privilege escalation attacks, it is advisable to restrict the capabilities available to containers. Use the --cap-drop
flag to drop specific capabilities when running containers.
$ docker run --cap-drop=ALL ...
6. Implement Resource Constraints
To prevent containers from abusing system resources, it is important to implement resource constraints. Use Docker’s resource management features to limit CPU, memory, and other resources for each container.
$ docker run --cpus=1 --memory=512m ...
7. Secure Docker Daemon Socket
The Docker daemon socket provides an API for interacting with Docker. By default, it listens on a Unix socket and can be accessed by root and members of the Docker group. To enhance security, consider using a Unix socket with restricted permissions or a TLS-secured TCP socket.
8. Enable AppArmor or SELinux
AppArmor and SELinux are security modules that can provide an additional layer of protection by enforcing access control policies on containers. Enable and configure either AppArmor or SELinux to restrict container processes and actions.
9. Monitor and Audit Docker Activity
Implement centralized logging and monitoring of Docker activity to detect and respond to potential security incidents. Tools like the ELK stack (Elasticsearch, Logstash, and Kibana) or Splunk can help you collect and analyze Docker logs.
10. Regularly Scan Images for Vulnerabilities
Regularly scan your Docker images for vulnerabilities using tools like Clair, Trivy, or Anchore. These tools analyze the contents of your images and provide information about any known vulnerabilities present.
By following these steps, you can significantly harden your Docker setup and enhance the security of your production environment. Remember, security is an ongoing process, so stay vigilant and keep up with the latest security best practices and updates in the Docker ecosystem.
Managing Secrets in Docker Containers
When working with Docker containers, it’s important to handle sensitive information, such as passwords, API keys, and certificates, securely. Docker provides several mechanisms to manage secrets within containers, ensuring that these sensitive details are protected.
One common approach to managing secrets in Docker is to use environment variables. Environment variables are a simple way to pass sensitive information to a container at runtime. However, this approach has some limitations. For example, environment variables can be easily accessed from within the container, making it less secure for storing highly sensitive data.
To address these limitations, Docker introduced the concept of secrets. Secrets are encrypted files or objects that are only accessible to the services that need them. Docker Swarm mode provides built-in support for managing secrets, making it easier to handle sensitive information in a cluster.
To create a secret in Docker Swarm, you can use the docker secret
command. For example, to create a secret from a file, you can use the following command:
$ echo "mysecretpassword" | docker secret create my_secret_password -
In this example, we create a secret called my_secret_password
and populate it with the contents of the file mysecretpassword
. The dash (-
) at the end indicates that the secret should be read from the standard input.
Once the secret is created, you can attach it to a service. For example, if you have a service called myapp
that requires the secret, you can specify the secret using the --secret
flag when creating or updating the service:
$ docker service create --name myapp --secret my_secret_password my_image
Docker Swarm automatically mounts the secret as a file in the container’s filesystem, making it accessible to the service. The secret is stored securely and can only be accessed by the specified service.
In addition to Docker Swarm secrets, you can also use external key-value stores, such as HashiCorp Vault or Amazon S3, to manage secrets in Docker containers. These tools provide more advanced features for secret management, such as access control and auditing.
When using external key-value stores, you can retrieve secrets at runtime by making API calls to the store. For example, if you’re using HashiCorp Vault, you can use the Vault API to fetch secrets and inject them into your containers using environment variables or configuration files.
Overall, managing secrets in Docker containers is crucial for ensuring the security of your applications. Whether you choose to use Docker Swarm secrets or external key-value stores, it’s important to adopt a secure approach to handle sensitive information effectively.
Related Article: Copying a Directory to Another Using the Docker Add Command
Using Security Tools and Plugins
Securing Docker containers is crucial to protect your applications from potential vulnerabilities and attacks. Docker provides a variety of security tools and plugins that can help you enhance the security of your containers. In this chapter, we will explore some of these tools and plugins and discuss how to effectively use them.
Docker Security Scanning
One of the built-in security tools provided by Docker is Docker Security Scanning. It allows you to scan your container images for known vulnerabilities and provides detailed vulnerability reports. By scanning your images before deploying them, you can identify and address potential security issues early in the development process.
To use Docker Security Scanning, you need to have a subscription to Docker Hub. Once you have a subscription, you can enable security scanning for your container images. Here’s an example of how to enable security scanning for a Docker image using the Docker CLI:
$ docker scan myimage:tag
This command will trigger a security scan for the specified image and provide you with a detailed report on any vulnerabilities found.
Docker Bench for Security
Docker Bench for Security is an open-source project that provides a script to check your Docker installation against industry-standard best practices for security. It scans your Docker host and container configurations, and provides recommendations for improving security.
To use Docker Bench for Security, you can clone the project’s GitHub repository and run the script on your Docker host. Here’s an example of how to use Docker Bench for Security:
$ git clone https://github.com/docker/docker-bench-security.git $ cd docker-bench-security $ ./docker-bench-security.sh
Running this script will perform a series of tests on your Docker installation and provide a report with recommendations for securing your setup.
Related Article: How to Use the Host Network in Docker Compose
Docker Content Trust
Docker Content Trust is a feature that ensures the authenticity and integrity of your container images. By enabling Docker Content Trust, you can verify that the images you pull from remote registries are signed and have not been tampered with.
To enable Docker Content Trust, you need to set the DOCKER_CONTENT_TRUST
environment variable to 1
before pulling or pushing images. Here’s an example of how to enable Docker Content Trust:
$ export DOCKER_CONTENT_TRUST=1
With Docker Content Trust enabled, Docker will only pull and run images that are signed and have a valid signature.
Third-Party Security Plugins
In addition to the built-in security tools, Docker has a rich ecosystem of third-party security plugins that can further enhance the security of your containers. These plugins provide additional security features, such as advanced access control, network isolation, and runtime protection.
You can find a wide range of security plugins on the Docker documentation and Docker Hub. Depending on your specific security requirements, you can choose and integrate the appropriate plugins into your Docker environment.
Using these security tools and plugins, you can significantly strengthen the security of your Docker containers and protect your applications from potential threats and vulnerabilities. However, it’s important to regularly update and monitor these tools to stay ahead of emerging security risks.
Real World Examples of Docker Container Security
As the popularity of Docker containers continues to rise, so does the need for robust security measures to protect the applications running within them. In this section, we will explore some real-world examples of Docker container security and the steps you can take to secure your own containers.
1. Limiting Privileges
One of the first steps you should take to secure your Docker containers is to limit the privileges of the containers themselves. By default, Docker containers run with root privileges, which can be a significant security risk. Instead, you should run containers with non-root users whenever possible.
To do this, you can specify a user in the Dockerfile using the USER
instruction. For example:
FROM alpine:latest USER myuser
This ensures that the container is running with the specified user and not with root privileges. It is also important to ensure that the user you are running the container as has the minimum necessary permissions to perform its intended tasks.
2. Using Image Vulnerability Scanning
Another important aspect of Docker container security is to regularly scan the container images for vulnerabilities. Vulnerabilities in container images can be a gateway for attackers to compromise your entire system.
There are several tools available that can help you scan your container images for vulnerabilities. One popular tool is Trivy, which is an open-source vulnerability scanner specifically designed for containers. Trivy can be easily integrated into your CI/CD pipeline to automatically scan your container images for known vulnerabilities.
3. Implementing Network Segmentation
Network segmentation is an essential practice to limit the impact of a potential breach within a Docker container. By separating containers into different networks or subnets, you can prevent an attacker from easily moving laterally within your containerized environment.
Docker provides several networking options that allow you to implement network segmentation, such as bridge networks, overlay networks, and MACVLAN networks. You can choose the most appropriate network type based on your specific requirements.
For example, to create a bridge network, you can use the following Docker command:
docker network create mynetwork
4. Enforcing Resource Limits
It is important to enforce resource limits on your Docker containers to prevent resource exhaustion attacks and ensure fair allocation of resources among containers. Docker provides several mechanisms to set resource limits, such as CPU and memory limits.
To set CPU limits, you can use the --cpus
flag when running a container:
docker run --cpus 1 mycontainer
To set memory limits, you can use the --memory
flag:
docker run --memory 1g mycontainer
By setting appropriate resource limits, you can ensure that a misbehaving container does not consume excessive resources and impact the performance of other containers.
5. Regularly Updating Container Images
Keeping your container images up to date is crucial for maintaining the security of your Docker environment. Just like any other software, container images can contain vulnerabilities that are discovered over time. Therefore, it is essential to regularly update your container images to include the latest security patches and fixes.
You can update the base image of your Dockerfile to pull the latest version of the image. For example:
FROM alpine:latest
By regularly updating your container images, you can minimize the risk of known vulnerabilities being exploited.
These real-world examples provide a starting point for securing your Docker containers. However, container security is an ongoing process, and it is important to stay updated with the latest best practices and security tools in the Docker ecosystem.
Related Article: How to Mount a Host Directory as a Volume in Docker Compose
Advanced Techniques for Securing Docker Containers
In the previous chapter, we discussed the basic steps to secure Docker containers. In this chapter, we will explore some advanced techniques that can further enhance the security of your Dockerized applications.
1. Implementing Image Vulnerability Scanning:
One crucial aspect of securing Docker containers is identifying and addressing vulnerabilities in the base images used by your application. To achieve this, you can employ image vulnerability scanning tools that analyze the images for potential security issues. These tools scan the image layers, dependencies, and libraries to identify known vulnerabilities.
One popular tool for image vulnerability scanning is Aqua Security. It provides a comprehensive vulnerability scanning solution specifically designed for Docker containers. By regularly scanning your images, you can detect vulnerabilities and take appropriate actions to mitigate them.
2. Utilizing Docker Content Trust:
Docker Content Trust (DCT) is a security feature that ensures the integrity and authenticity of Docker images. It uses digital signatures to verify that the images you pull and run are from trusted sources and haven’t been tampered with.
To enable Docker Content Trust, you need to set the DOCKER_CONTENT_TRUST
environment variable to 1
before performing any Docker operations. This ensures that only signed images are used, preventing the execution of potentially malicious or altered images. For example, in a Linux environment, you can set the environment variable using the command:
export DOCKER_CONTENT_TRUST=1
3. Enforcing Resource Limitations:
By default, Docker containers have access to the host system’s resources, which can potentially lead to resource exhaustion attacks. To mitigate this risk, you can enforce resource limitations on your containers.
Docker provides several resource constraints that you can apply to containers, such as CPU limits, memory limits, and I/O restrictions. These constraints can prevent a single container from monopolizing the host’s resources and ensure fair allocation among all running containers.
For example, you can limit the CPU usage of a container to 50% by setting the --cpus
flag when running the container:
docker run --cpus=0.5 myapp
4. Implementing Network Segmentation:
Network segmentation is a technique that involves isolating containers into separate networks to minimize the potential attack surface. By default, Docker containers share the same network namespace, allowing direct communication between containers on the same host.
To implement network segmentation, you can create multiple Docker networks and assign specific containers to each network based on their security requirements. This way, even if one container is compromised, the attacker’s access is limited to the containers within the same network.
For example, you can create a custom bridge network and attach containers to it using the --network
flag during container creation:
docker network create mynetwork docker run --network=mynetwork myapp
5. Regularly Updating Docker and Images:
Keeping Docker and your images up to date is vital for maintaining container security. Docker regularly releases security patches and updates that address vulnerabilities and improve overall security.
To update Docker, you can use the package manager specific to your operating system. For example, on Ubuntu, you can run the following commands to update Docker:
sudo apt-get update sudo apt-get upgrade docker-ce
Additionally, ensure that you regularly update the base images used by your application. This ensures that you are using the most secure and up-to-date versions of the software.
By implementing these advanced techniques, you can significantly enhance the security of your Docker containers and protect your applications from potential threats. Remember to regularly review and update your security measures to adapt to evolving security risks.