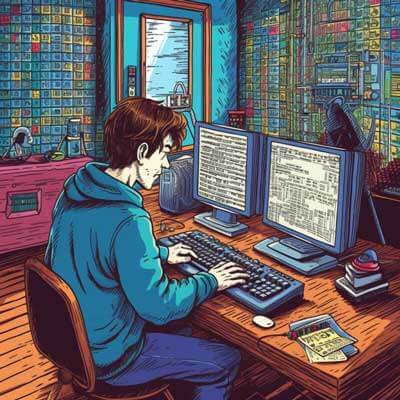
Table of Contents
Introduction to Nested Queries
Nested queries, also known as subqueries, are a powerful feature in databases that allow you to perform queries within queries. This means that you can use the result of one query as the input for another query, enabling you to retrieve or manipulate data in a more flexible and efficient way. Nested queries are commonly used when you need to filter, aggregate, compare, or manipulate data based on the results of another query.
Related Article: How to Use the WHERE Condition in SQL Joins
Syntax of Nested Queries
The syntax of nested queries varies depending on the database management system (DBMS) you are using. However, the general structure is as follows:
SELECT column1, column2, ... FROM table1 WHERE column1 IN (SELECT column1 FROM table2 WHERE condition);
In this example, the inner query (SELECT column1 FROM table2 WHERE condition)
is executed first and returns a set of values. These values are then used in the outer query SELECT column1, column2, ... FROM table1 WHERE column1 IN (...)
to filter the data from table1
.
Nested Queries in SQL: Basic Examples
Let's explore some basic examples of nested queries in SQL.
Example 1: Data Filtering
Suppose we have two tables, customers
and orders
, and we want to retrieve the names of customers who have placed at least one order. We can use a nested query to accomplish this:
SELECT name FROM customers WHERE id IN (SELECT customer_id FROM orders);
In this example, the inner query (SELECT customer_id FROM orders)
retrieves the customer_id
values from the orders
table. The outer query then uses these values to filter the name
column from the customers
table.
Related Article: How to Set Timestamps With & Without Time Zone in PostgreSQL
Example 2: Data Aggregation
Let's say we have a products
table and we want to find the average price of products in each category. We can use a nested query with the GROUP BY
clause to achieve this:
SELECT category, AVG(price) AS average_price FROM products WHERE price > (SELECT AVG(price) FROM products) GROUP BY category;
In this example, the inner query (SELECT AVG(price) FROM products)
calculates the average price of all products. The outer query then retrieves the category and calculates the average price for each category, but only for categories where the price is higher than the overall average price.
Nested Query Use Case: Data Comparison
Nested queries are often used for data comparison. Let's consider a use case where we have two tables, students
and grades
, and we want to find the students who have a higher average grade than the class average.
SELECT name FROM students WHERE average_grade > (SELECT AVG(grade) FROM grades);
In this example, the inner query (SELECT AVG(grade) FROM grades)
calculates the average grade for the entire class. The outer query then retrieves the names of students whose average grade is higher than the class average.
Nested Query Use Case: Data Manipulation
Nested queries can also be used for data manipulation. Let's say we have a products
table and we want to update the price of all products in a specific category to a certain value.
UPDATE products SET price = 10.99 WHERE category = 'Electronics' AND id IN (SELECT id FROM products WHERE category = 'Electronics');
In this example, the inner query (SELECT id FROM products WHERE category = 'Electronics')
retrieves the id
values of all products in the 'Electronics' category. The outer query then updates the price of these products to 10.99.
Nested Query Best Practice: Query Optimization
When using nested queries, it is important to optimize your queries for performance. Here are some best practices to consider:
- Minimize the use of nested queries: Instead of nesting multiple queries, try to combine them using joins or other techniques to reduce the complexity of your queries.
- Use appropriate indexes: Make sure to create indexes on columns that are frequently used in nested queries to improve query performance.
- Test and optimize query execution: Use the EXPLAIN statement (or equivalent) provided by your DBMS to analyze the query execution plan and identify potential bottlenecks. Consider rewriting your query or optimizing the database schema if necessary.
Related Article: Tutorial: PostgreSQL Array Literals
Nested Query Best Practice: Error Handling
Error handling is an important aspect when working with nested queries. Here are some best practices to follow:
- Handle NULL values: When using nested queries, be aware that the inner query may return NULL values. Make sure to handle these cases properly to avoid unexpected behavior.
- Test with different data scenarios: Test your nested queries with different data scenarios to ensure that they handle edge cases and error conditions correctly.
- Use proper error logging and reporting: Implement a robust error logging and reporting mechanism to capture and handle any errors that may occur during the execution of nested queries.
Real World Example of Nested Query: E-commerce Data Analysis
To demonstrate the practical application of nested queries, let's consider a real-world example in the context of e-commerce data analysis. Suppose we have two tables, orders
and products
, and we want to find the top-selling products in each category.
SELECT category, name, sold_units FROM products WHERE (category, sold_units) IN ( SELECT category, MAX(sold_units) FROM products GROUP BY category );
In this example, the inner query (SELECT category, MAX(sold_units) FROM products GROUP BY category)
retrieves the maximum number of sold units for each category. The outer query then retrieves the category, name, and number of sold units for the products that match the category and maximum sold units.
Real World Example of Nested Query: Social Media Data Analysis
Another real-world example of using nested queries is in social media data analysis. Let's say we have two tables, users
and posts
, and we want to find the users who have made the most posts.
SELECT username, num_posts FROM users WHERE num_posts = (SELECT MAX(num_posts) FROM users);
In this example, the inner query (SELECT MAX(num_posts) FROM users)
retrieves the maximum number of posts made by any user. The outer query then retrieves the usernames and number of posts for the users whose number of posts matches the maximum.
Performance Consideration: Query Execution Time
When using nested queries, it is important to consider the execution time of your queries. Here are some factors that can impact query execution time:
- Data volume: The size of your tables and the amount of data being processed can significantly affect query execution time. Make sure to optimize your database schema and use appropriate indexing to improve performance.
- Query complexity: The complexity of your nested queries, including the number of subqueries and the complexity of the conditions, can impact execution time. Simplify your queries where possible to improve performance.
- Network latency: If your database is hosted on a remote server, network latency can introduce additional overhead. Consider optimizing your network infrastructure or using caching techniques to minimize this impact.
Related Article: Managing PostgreSQL Databases with PHPMyAdmin
Performance Consideration: Database Load
Nested queries can put a significant load on your database server, especially if they involve large tables or complex operations. Here are some considerations to minimize the impact on database load:
- Indexing: Use appropriate indexes on columns used in nested queries to speed up data retrieval. Be careful not to over-index, as it can also impact insert and update operations.
- Query optimization: Analyze and optimize your queries to minimize unnecessary calculations and data retrieval. Use EXPLAIN or equivalent tools to identify potential performance bottlenecks.
- Database tuning: Adjust the configuration of your database server to allocate sufficient resources for handling nested queries. This may include increasing memory, optimizing disk I/O, or adjusting query cache settings.
Performance Consideration: Data Volume
The volume of data being processed by nested queries can impact performance. Here are some strategies to manage data volume efficiently:
- Partitioning: If your tables contain a large amount of data, consider partitioning them based on a specific criterion (e.g., date range) to improve query performance.
- Data archiving: Move inactive or historical data to separate storage or archive tables to reduce the volume of data being processed by nested queries.
- Aggregation and summarization: Pre-compute and store aggregated or summarized data to reduce the amount of data processed by nested queries. This can be especially useful for frequently executed queries.
Advanced Technique: Recursive Queries
Recursive queries, also known as hierarchical queries, are a powerful extension of nested queries that allow you to traverse hierarchical or nested data structures. Recursive queries are commonly used to work with tree-like structures, such as organization charts, file systems, or forum threads.
The syntax and specifics of recursive queries vary depending on the database management system you are using. However, the general idea is to define a base case and a recursive case in the query to traverse the hierarchical structure.
Advanced Technique: Correlated Subqueries
Correlated subqueries are a special type of nested query where the inner query references columns from the outer query. This allows you to perform more complex operations and make the inner query dependent on the outer query's results. Correlated subqueries are useful when you need to perform calculations or filtering based on values in the outer query.
The syntax of correlated subqueries is similar to regular nested queries. However, instead of using a fixed value or condition in the inner query, you reference columns from the outer query.
Related Article: Exploring Left to Right SQL Joins in Databases
Code Snippet Idea: Nested Query for Data Retrieval
Here's a code snippet idea that demonstrates using a nested query for data retrieval in Python with the SQLite database.
import sqlite3 # Connect to the database conn = sqlite3.connect('example.db') cursor = conn.cursor() # Execute a nested query to retrieve data cursor.execute('SELECT name FROM customers WHERE id IN (SELECT customer_id FROM orders)') rows = cursor.fetchall() # Process the retrieved data for row in rows: print(row[0]) # Close the database connection conn.close()
In this example, the nested query SELECT customer_id FROM orders
is executed to retrieve the customer_id
values from the orders
table. The outer query then retrieves the corresponding customer names from the customers
table.
Code Snippet Idea: Nested Query for Data Update
Here's a code snippet idea that demonstrates using a nested query for data update in Java with JDBC and MySQL.
import java.sql.*; public class NestedQueryExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try (Connection conn = DriverManager.getConnection(url, username, password); Statement stmt = conn.createStatement()) { // Execute a nested query to update data String query = "UPDATE products SET price = 10.99 WHERE category = 'Electronics' AND id IN (SELECT id FROM products WHERE category = 'Electronics')"; int rowsAffected = stmt.executeUpdate(query); // Print the number of rows affected System.out.println("Rows affected: " + rowsAffected); } catch (SQLException e) { e.printStackTrace(); } } }
In this example, the nested query SELECT id FROM products WHERE category = 'Electronics'
is executed to retrieve the id
values of products in the 'Electronics' category. The outer query then updates the price of these products to 10.99.
Code Snippet Idea: Nested Query for Data Deletion
Here's a code snippet idea that demonstrates using a nested query for data deletion in C# with ADO.NET and SQL Server.
using System; using System.Data.SqlClient; namespace NestedQueryExample { class Program { static void Main(string[] args) { string connectionString = "Data Source=(local);Initial Catalog=mydatabase;Integrated Security=True"; using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // Execute a nested query to delete data <a href="https://www.squash.io/how-to-use-mysql-query-string-contains/">string query</a> = "DELETE FROM products WHERE category = 'Electronics' AND id IN (SELECT id FROM products WHERE category = 'Electronics')"; using (SqlCommand command = new SqlCommand(query, connection)) { int rowsAffected = command.ExecuteNonQuery(); // Print the number of rows affected Console.WriteLine("Rows affected: " + rowsAffected); } } } } }
In this example, the nested query SELECT id FROM products WHERE category = 'Electronics'
is executed to retrieve the id
values of products in the 'Electronics' category. The outer query then deletes these products from the products
table.
Code Snippet Idea: Nested Query for Data Insertion
Here's a code snippet idea that demonstrates using a nested query for data insertion in PHP with PDO and MySQL.
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a nested query to insert data $query = "INSERT INTO orders (customer_id, order_date) SELECT id, NOW() FROM customers WHERE name = :name"; $stmt = $conn->prepare($query); $stmt->bindParam(':name', $name); // Set the parameter value and execute the query $name = 'John Doe'; $stmt->execute(); // Print the number of rows affected echo "Rows affected: " . $stmt->rowCount(); } catch (PDOException $e) { echo "Error: " . $e->getMessage(); } $conn = null; ?>
In this example, the nested query SELECT id, NOW() FROM customers WHERE name = :name
is executed to retrieve the id
values of customers with the specified name and the current date. The outer query then inserts these values into the orders
table.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Code Snippet Idea: Nested Query for Data Validation
Here's a code snippet idea that demonstrates using a nested query for data validation in Ruby with ActiveRecord and PostgreSQL.
class Order < ActiveRecord::Base belongs_to :customer validate :validate_customer_name private def validate_customer_name customer = Customer.find_by(name: customer_name) unless customer errors.add(:customer_name, 'does not exist') return end unless customer.orders.empty? errors.add(:customer_name, 'already has an order') end end end
In this example, a nested query is used within a validation method in the Order
model. The nested query Customer.find_by(name: customer_name)
retrieves the customer with the specified name. The outer query then validates the presence of the customer and checks if they already have an order.
Error Handling: Debugging Syntax Errors
When working with nested queries, it is common to encounter syntax errors. Here are some tips for debugging syntax errors:
- Check the query syntax: Carefully review the syntax of your nested query, including the placement of parentheses, commas, and quotation marks. Make sure the query is well-formed according to the syntax rules of your database management system.
- Use error messages: When a syntax error occurs, the database management system usually provides an error message that describes the issue. Pay attention to these messages and try to identify the specific part of the query that is causing the error.
- Use logging and debugging tools: Enable logging and debugging tools provided by your database management system to get more detailed information about the syntax error. These tools can help you trace the execution flow and identify the root cause of the error.
Error Handling: Managing Runtime Errors
In addition to syntax errors, you may also encounter runtime errors when working with nested queries. Here are some best practices for managing runtime errors:
- Use proper error handling techniques: Implement error handling mechanisms, such as try-catch blocks or exception handling, to handle and manage runtime errors gracefully. Proper error handling can prevent application crashes and provide meaningful feedback to users.
- Validate input data: Validate the input data before executing nested queries to avoid potential runtime errors caused by invalid or unexpected values. Perform data validation and sanitization to ensure the input data meets the required criteria.
- Test with different scenarios: Test your nested queries with different scenarios and edge cases to identify and handle potential runtime errors. Consider using automated tests to cover a wide range of input data and conditions.