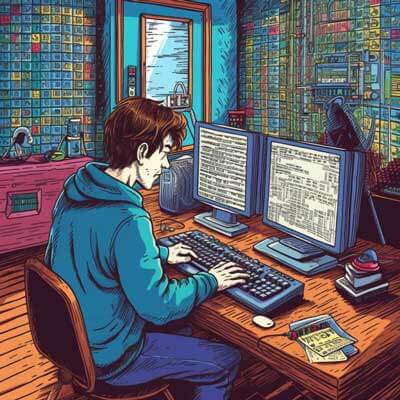
- Understanding Docker Containers: An Overview
- Containerization and Virtualization
- Container Images
- Container Networking
- Resource Management
- Container Monitoring
- Setting Up Docker on Your System: Installation Guide
- Installing Docker on Linux
- Installing Docker on macOS
- Installing Docker on Windows
- Verifying the Docker Installation
- Optimizing Docker Images: Best Practices
- Use Official Base Images
- Minimize the Number of Layers
- Use .dockerignore to Exclude Unnecessary Files
- Use Specific Tags for Base Images
- Optimize Image Size
- Managing Docker Containers: Tips and Tricks
- Use Appropriate Resource Limits
- Monitor Resource Usage
- Use Docker Volumes for Persistent Data
- Clean Up Unused Containers and Images
- Utilize Docker Compose for Complex Deployments
- Improving Docker Networking: Strategies for Efficiency
- Use Host Networking
- Optimize DNS Resolution
- Utilize Container Networking Models
- Optimize Network Traffic
- Scaling Docker Applications: Techniques for Performance
- Load Balancing
- Horizontal Scaling
- 3. Vertical Scaling
- Caching
- Monitoring and Optimization
- Monitoring Docker Containers: Tools and Examples
- Docker Stats
- cAdvisor
- Prometheus
- Grafana
- Securing Docker Containers: Best Practices
- Working with Docker Volumes: Data Persistence
- Creating a Docker Volume
- Mounting a Volume in a Container
- Sharing Volumes between Containers
- Backing Up and Restoring Volumes
- Container Orchestration with Docker
- What is Container Orchestration?
- Why Use Container Orchestration?
- Docker Swarm
- Kubernetes Integration
- Advanced Docker Performance Tuning: Tips and Techniques
- Minimize Container Size
- Optimize Image Layers
- Utilize Resource Limits
- Fine-tune Docker Networking
- Monitor and Analyze Performance
Understanding Docker Containers: An Overview
Docker has become one of the most popular technologies for containerization, enabling developers to build and deploy applications using isolated containers. A Docker container is a lightweight, standalone executable package that includes everything needed to run an application, including the code, runtime, system tools, and system libraries. Understanding the basics of Docker containers is crucial for optimizing their performance.
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
Containerization and Virtualization
Containerization is often compared to virtualization, but they are fundamentally different. Virtualization runs multiple virtual machines (VMs) on a single physical host, each with its own operating system (OS). On the other hand, containerization allows multiple containers to run on a single host, sharing the host OS kernel.
This key difference makes Docker containers faster and more lightweight than VMs. Containers start up quickly and consume fewer system resources, as they don’t require the overhead of running a full OS.
Container Images
A Docker container is created from a base image, which is a read-only template that includes the necessary dependencies and files to run an application. Images are built using a Dockerfile, a simple text file that specifies the base image, instructions to install dependencies, and commands to execute when the container starts.
To optimize container performance, it’s essential to use lightweight base images and avoid including unnecessary dependencies. For example, using a minimal Alpine Linux image instead of a full-fledged Ubuntu image can significantly reduce the container’s size and improve startup time.
Container Networking
Docker provides networking capabilities that allow containers to communicate with each other and with external systems. By default, Docker creates a bridge network for containers, enabling them to communicate with each other using IP addresses.
To optimize container networking, it’s important to consider the network architecture and choose the appropriate network driver. Docker supports different network drivers, including bridge, host, overlay, and macvlan. Each driver has its own advantages and use cases, so selecting the right one can improve network performance.
Related Article: How to Install and Use Docker
Resource Management
Docker provides several features to manage and control the resources allocated to containers. By default, containers have access to the host’s resources, but this can lead to resource contention and affect performance. Docker allows you to set resource limits, such as CPU and memory constraints, to ensure fair resource allocation.
For example, you can limit a container’s CPU usage to prevent it from monopolizing the host’s resources. Similarly, you can set memory limits to prevent a container from consuming excessive memory, which can lead to out-of-memory errors.
Container Monitoring
Monitoring container performance is essential to identify bottlenecks and optimize resource allocation. Docker provides built-in monitoring tools, such as the Docker stats command, which displays real-time metrics for CPU, memory, and network usage of running containers.
Additionally, you can use third-party monitoring solutions, like Prometheus or Grafana, to collect and visualize container metrics over time. These tools can help you identify performance issues and make informed decisions to optimize container performance.
Setting Up Docker on Your System: Installation Guide
To begin optimizing Docker container performance, you first need to have Docker installed on your system. Docker provides a simple and efficient way to package, distribute, and run applications using containerization. This section will guide you through the installation process for Docker on various operating systems.
Related Article: Docker How-To: Workdir, Run Command, Env Variables
Installing Docker on Linux
Installing Docker on Linux is straightforward and can be done using the package manager of your distribution. Here’s an example of how to install Docker on Ubuntu:
1. Update the package index on your system by running the following command:
sudo apt update
2. Install the necessary packages to allow apt to use a repository over HTTPS:
sudo apt install apt-transport-https ca-certificates curl software-properties-common
3. Import Docker’s official GPG key using the following command:
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
4. Add the Docker repository to your system’s software sources:
echo "deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
5. Update the package index again:
sudo apt update
6. Install Docker by running the following command:
sudo apt install docker-ce docker-ce-cli containerd.io
7. Docker should now be installed on your Linux system. Verify the installation by running the following command:
sudo docker run hello-world
Installing Docker on macOS
To install Docker on macOS, follow these steps:
1. Download the Docker Desktop installer from the Docker website: https://www.docker.com/products/docker-desktop.
2. Double-click the downloaded package file to start the installation process.
3. Follow the instructions provided by the installer to complete the installation.
4. Once the installation is complete, Docker should be available in your Applications folder.
Installing Docker on Windows
To install Docker on Windows, follow these steps:
1. Download the Docker Desktop installer from the Docker website: https://www.docker.com/products/docker-desktop.
2. Double-click the downloaded installer to start the installation process.
3. Follow the instructions provided by the installer to complete the installation.
4. Once the installation is complete, Docker should be available in your Start menu.
Related Article: How to Secure Docker Containers
Verifying the Docker Installation
After installing Docker, it’s important to verify that the installation was successful. Run the following command in your terminal or command prompt:
docker version
This command will display the version of Docker installed on your system, along with the version of Docker Compose and other relevant information.
Now that Docker is installed and verified, you’re ready to start optimizing your Docker container performance. In the next sections, we’ll explore various tips and techniques to improve the performance of your Docker containers.
Optimizing Docker Images: Best Practices
When it comes to optimizing Docker container performance, one of the most crucial aspects is optimizing the Docker images themselves. Docker images serve as the building blocks for your containers, and any inefficiencies in the images can directly impact the performance of your containers. We will explore some best practices for optimizing Docker images.
Use Official Base Images
Official Docker base images are maintained by the Docker community and are regularly updated to include security patches and bug fixes. Using official base images ensures that you start with a solid foundation for your containers. These images are generally well-optimized and have a smaller size compared to custom-built images.
To use an official base image, specify it in your Dockerfile using the FROM
keyword. For example, to use the official Python 3.9 base image, you can use the following line in your Dockerfile:
FROM python:3.9
Related Article: Quick Docker Cheat Sheet: Simplify Containerization
Minimize the Number of Layers
Docker images are built using a layered filesystem, where each instruction in the Dockerfile creates a new layer. Each layer adds some overhead in terms of disk space and runtime performance. Therefore, it is important to minimize the number of layers in your Docker image.
To reduce the number of layers, you can combine multiple instructions into a single RUN
instruction. For example, instead of using separate RUN
instructions for installing packages, you can combine them into a single instruction:
RUN apt-get update && apt-get install -y package1 package2 package3
Use .dockerignore to Exclude Unnecessary Files
When building a Docker image, all files in the build context directory are sent to the Docker daemon. However, not all files are required in the final image. Using a .dockerignore
file, you can specify patterns to exclude certain files and directories from being sent to the Docker daemon.
For example, you can exclude files such as development artifacts, logs, and temporary files by adding the following patterns to your .dockerignore
file:
# Exclude development artifacts *.log *.tmp # Exclude logs logs/ # Exclude temporary files tmp/
Use Specific Tags for Base Images
When specifying a base image in your Dockerfile, it is recommended to use specific tags instead of the latest
tag. The latest
tag refers to the latest version of the image, which can change over time. Using specific tags ensures that you have control over the version of the base image used in your container.
For example, instead of using FROM python:latest
, you can use a specific version like FROM python:3.9
to ensure that the version remains consistent.
Related Article: Docker CLI Tutorial and Advanced Commands
Optimize Image Size
Reducing the size of your Docker images can significantly improve the performance of your containers. Here are a few tips to optimize the image size:
– Use a minimal base image: Choose a base image that only includes the necessary dependencies for your application.
– Avoid unnecessary packages and dependencies: Only include the packages and dependencies required by your application.
– Remove unnecessary files: Clean up any unnecessary files and directories in your image.
– Use multi-stage builds: Utilize multi-stage builds to separate the build-time dependencies from the runtime dependencies, resulting in smaller final images.
By following these best practices, you can optimize your Docker images and improve the overall performance of your containers.
Remember, optimizing Docker images is an ongoing process, and you should regularly review and update your images to incorporate the latest optimizations and improvements.
Now that we have covered the best practices for optimizing Docker images, let’s explore ways to optimize container resource allocation and networking.
Managing Docker Containers: Tips and Tricks
Docker containers have revolutionized the way we develop and deploy applications. They provide a lightweight and isolated environment that can run anywhere, making them the go-to choice for many developers. However, managing Docker containers effectively is crucial to ensure optimal performance and efficiency. We will explore some tips and tricks to help you better manage your Docker containers.
Use Appropriate Resource Limits
When running Docker containers, it’s important to set appropriate resource limits to ensure that each container gets the necessary amount of CPU and memory resources. This helps prevent one container from monopolizing the resources and affecting the performance of other containers.
To set resource limits, you can use the --cpus
and --memory
options when running the docker run
command. For example, to limit a container to use only 1 CPU and 512MB of memory, you can run:
docker run --cpus=1 --memory=512m my-container
Related Article: Comparing Kubernetes vs Docker
Monitor Resource Usage
Monitoring the resource usage of your Docker containers is essential to identify bottlenecks and optimize performance. Docker provides several options to monitor resource usage, including the built-in stats
command and third-party monitoring tools like cAdvisor and Prometheus.
The docker stats
command provides real-time information about CPU, memory, and network usage of running containers. For example, to monitor resource usage of all containers on your system, you can run:
docker stats --all
Use Docker Volumes for Persistent Data
Docker volumes are a great way to manage persistent data for your containers. By using volumes, you can separate data from the container’s filesystem, making it easier to manage and backup.
To create a volume, you can use the docker volume create
command. For example, to create a volume named myvolume
, you can run:
docker volume create myvolume
Then, you can mount the volume to a container by using the -v
or --mount
option when running the docker run
command. For example, to mount the myvolume
volume to a container at the /data
directory, you can run:
docker run -v myvolume:/data my-container
Clean Up Unused Containers and Images
Over time, unused containers and images can accumulate and consume valuable disk space. It’s important to regularly clean up these unused resources to optimize disk usage.
To remove unused containers, you can use the docker container prune
command. For example, to remove all stopped containers, you can run:
docker container prune
To remove unused images, you can use the docker image prune
command. For example, to remove all dangling (unused) images, you can run:
docker image prune
Related Article: How To Delete All Docker Images
Utilize Docker Compose for Complex Deployments
Docker Compose is a powerful tool for managing multi-container applications. It allows you to define and run complex deployments with multiple containers, networks, and volumes using a single YAML file.
By using Docker Compose, you can easily define the relationships between different containers and manage their configuration. This simplifies the deployment process and ensures consistency across different environments.
To get started with Docker Compose, you need to create a docker-compose.yml
file in your project directory. Here’s an example of a simple docker-compose.yml
file:
version: '3' services: web: image: nginx:latest ports: - 80:80 volumes: - ./html:/usr/share/nginx/html
This example defines a service named web
based on the nginx:latest
image, maps port 80
on the host to port 80
inside the container, and mounts the ./html
directory on the host to /usr/share/nginx/html
inside the container.
Improving Docker Networking: Strategies for Efficiency
Networking is a crucial aspect of Docker container performance. Efficient networking can significantly impact the overall performance and scalability of your Dockerized applications. We will explore some strategies to optimize Docker networking and improve the efficiency of your containers.
Use Host Networking
By default, Docker containers run in an isolated network namespace, with their own IP address and network stack. However, this isolation comes with a performance overhead. To improve networking performance, you can use host networking, which allows the container to share the host network stack.
To enable host networking, use the --net=host
flag when running your container:
docker run --net=host
Using host networking eliminates the overhead of network address translation (NAT) and provides direct access to the host’s network interfaces. However, this also means that containers share the same network namespace as the host, potentially exposing them to security risks. Use host networking judiciously and consider the implications for your specific use case.
Related Article: How to Use Docker Exec for Container Commands
Optimize DNS Resolution
DNS resolution can impact the overall performance of containerized applications, especially when containers need to communicate with each other or with external services. Docker provides a built-in DNS server that resolves container names to IP addresses. However, this DNS resolution process can introduce latency.
To improve DNS resolution performance, you can configure Docker to use alternative DNS servers. You can specify the DNS server(s) using the --dns
flag when running the Docker daemon:
dockerd --dns
Alternatively, you can modify the Docker daemon configuration file (/etc/docker/daemon.json
) to set the DNS server(s):
{ "dns": [""] }
Using faster and reliable DNS servers can significantly reduce the time taken for DNS resolution, leading to improved networking performance for your Docker containers.
Utilize Container Networking Models
Docker provides different networking models that offer varying levels of isolation and performance. Understanding these networking models can help you choose the most suitable one for your application.
– **Bridge networking**: This is the default networking mode in Docker, where each container gets its own IP address on a virtual network bridge. Bridge networking provides isolation and allows containers to communicate with each other using container names.
– **Overlay networking**: Overlay networks enable containers running on different hosts to communicate with each other seamlessly. This networking model is ideal for distributed applications that span multiple hosts.
– **Macvlan networking**: Macvlan allows containers to have their own MAC addresses, making them appear as separate physical devices on the network. This mode provides the highest performance and is suitable for scenarios where containers require direct access to the network.
Choose the networking model that best suits your application’s requirements in terms of performance, scalability, and isolation.
Optimize Network Traffic
Reducing unnecessary network traffic can improve the overall performance of your Docker containers. Here are a few strategies to optimize network traffic:
– **Minimize container-to-container communication**: Reduce the number of requests between containers by optimizing your application architecture. Consider using message queues or event-driven architectures to decouple components and reduce direct communication.
– **Use efficient protocols**: Choose lightweight and efficient protocols, such as HTTP/2 or gRPC, that minimize network overhead and improve performance.
– **Enable compression**: Enable compression for network traffic to reduce the amount of data transmitted. This can be particularly beneficial for transmitting large files or payloads.
By implementing these strategies, you can reduce network latency, minimize bandwidth consumption, and improve the overall efficiency of your Docker containers.
Optimizing Docker networking is essential for achieving optimal performance and scalability of your containerized applications. You can improve the efficiency of your Docker containers and enhance the overall performance of your applications.
Related Article: How to Copy Files From Host to Docker Container
Scaling Docker Applications: Techniques for Performance
Scaling Docker applications is crucial for achieving optimal performance and ensuring that your containers can handle increasing workloads. By employing various techniques and best practices, you can effectively scale your Docker applications and enhance their performance. We will explore some key techniques for scaling Docker applications.
Load Balancing
Load balancing is a vital technique for distributing incoming network traffic across multiple Docker containers. It helps in evenly distributing the workload and prevents any single container from being overwhelmed. There are several load balancing strategies, such as round-robin, least connections, and IP hash, which can be implemented using tools like Nginx, HAProxy, or Kubernetes. Let’s take a look at an example of load balancing using Nginx:
http { upstream backend { server container1:80; server container2:80; } server { listen 80; location / { proxy_pass http://backend; } } }
Horizontal Scaling
Horizontal scaling involves adding more instances of Docker containers to handle increased demand. By horizontally scaling your application, you can distribute the workload across multiple containers, which can result in improved performance and increased capacity. Tools like Docker Swarm and Kubernetes provide seamless support for horizontal scaling. Here’s an example of scaling a service using Docker Swarm:
version: '3' services: app: image: myapp:latest deploy: replicas: 5
Related Article: How to Pass Environment Variables to Docker Containers
3. Vertical Scaling
Vertical scaling refers to increasing the resources allocated to a single Docker container. It involves upgrading the CPU, memory, or disk capacity of a container to handle higher workloads. Vertical scaling is typically done by modifying the Docker host’s configuration or using tools like Amazon EC2 instances. Here’s an example of modifying the CPU and memory limits of a Docker container:
docker run --cpus=2 --memory=4g myapp:latest
Caching
Caching is an effective technique for improving the performance of Docker applications. By caching frequently accessed data or computations, you can reduce the load on your containers and provide faster responses to users. Tools like Redis or Memcached can be used for caching in Docker applications. Here’s an example of using Redis as a cache in a Docker application:
import redis redis_client = redis.Redis(host='redis', port=6379) cached_data = redis_client.get('data') if cached_data: # Use cached data else: # Compute and cache the data redis_client.set('data', computed_data)
Monitoring and Optimization
Monitoring and optimizing your Docker applications is crucial for identifying performance bottlenecks and improving overall efficiency. Tools like Prometheus, Grafana, and Docker Stats provide valuable insights into the resource utilization, container health, and performance metrics of your Docker environment. By proactively monitoring and optimizing your containers, you can ensure that they are running efficiently and delivering optimal performance.
Implementing these techniques for scaling Docker applications can significantly enhance their performance and enable them to handle increasing workloads. By combining load balancing, horizontal and vertical scaling, caching, and effective monitoring, you can create resilient and performant Docker applications.
We will explore advanced networking techniques for Docker containers.
Related Article: How to Run a Docker Instance from a Dockerfile
Monitoring Docker Containers: Tools and Examples
Monitoring your Docker containers is crucial to ensure optimal performance and troubleshoot any issues that may arise. In this section, we will explore some popular tools and examples for monitoring Docker containers.
Docker Stats
One of the simplest ways to monitor Docker containers is by using the built-in Docker Stats command. This command provides real-time statistics on CPU usage, memory consumption, network I/O, and disk I/O for each running container.
To use Docker Stats, simply run the following command:
docker stats
This will display a table with the statistics for each running container, including the container ID, name, CPU usage, memory usage, network I/O, and disk I/O.
cAdvisor
cAdvisor (Container Advisor) is an open-source monitoring tool specifically designed for Docker containers. It collects and exports detailed container resource usage and performance metrics to various monitoring systems, such as Prometheus or Graphite.
To use cAdvisor, you can run it as a Docker container itself:
docker run -d --name=cadvisor -p 8080:8080 \ --volume=/var/run/docker.sock:/var/run/docker.sock \ --volume=/sys:/sys:ro \ --volume=/var/lib/docker/:/var/lib/docker:ro \ google/cadvisor:latest
This command will start cAdvisor as a container and expose its web interface on port 8080. You can then access the cAdvisor dashboard by visiting http://localhost:8080
in your web browser.
Related Article: How To List Containers In Docker
Prometheus
Prometheus is a powerful monitoring and alerting system that can be used to collect and analyze metrics from Docker containers. It provides a flexible query language and a graphical interface for visualizing the collected data.
To monitor Docker containers with Prometheus, you need to configure it to scrape metrics from the cAdvisor container. Here is an example configuration:
scrape_configs: - job_name: 'cadvisor' static_configs: - targets: ['cadvisor:8080']
This configuration tells Prometheus to scrape metrics from the cAdvisor container on port 8080. Once configured, you can access the Prometheus web interface to explore and visualize the collected metrics.
Grafana
Grafana is a popular open-source visualization tool that works well with Prometheus. It allows you to create custom dashboards with graphs, charts, and other visualizations based on the collected metrics.
To use Grafana with Prometheus, you need to configure Grafana to connect to your Prometheus server. Once connected, you can create a new dashboard and add panels to display the metrics of interest.
Securing Docker Containers: Best Practices
Securing Docker containers is essential to protect your applications and data from potential threats. Docker provides several built-in security features and best practices that you can follow to enhance the security of your containers. In this section, we will explore some of the best practices for securing Docker containers.
1. Use Official Images: When building your Docker containers, it is recommended to use official images from trusted sources like Docker Hub. Official images are regularly updated and maintained by the Docker community, ensuring that any security vulnerabilities are patched promptly.
2. Update Regularly: Keeping your Docker software and images up to date is crucial for maintaining security. Docker releases regular updates with security patches and bug fixes. Make sure to regularly update your Docker software and pull the latest versions of your base images to take advantage of these updates.
3. Enable Content Trust: Docker Content Trust is a security feature that ensures the integrity and authenticity of your images. When enabled, Docker only allows the use of signed images, preventing the execution of tampered or malicious images. You can enable content trust by setting the DOCKER_CONTENT_TRUST
environment variable to 1
.
4. Limit Privileges: By default, Docker containers run with root privileges, which can be a security risk. To mitigate this risk, it is recommended to run containers with non-root users whenever possible. You can achieve this by specifying a non-root user in your Dockerfile or using the --user
flag when running containers.
5. Isolate Containers: It is a best practice to isolate your containers by running each service in a separate container. This helps contain any potential security breaches to a single container and prevents attackers from accessing other services or data on your host.
6. Implement Network Segmentation: Docker provides networking capabilities that allow you to control the communication between containers and the host. Implementing network segmentation helps isolate your containers from the host and other containers, reducing the attack surface.
7. Use Host Firewall: Configure the host firewall to restrict incoming and outgoing traffic to your containers. By only allowing necessary ports and protocols, you can prevent unauthorized access to your containers.
8. Enable AppArmor or SELinux: AppArmor and SELinux are security frameworks that provide additional protection by enforcing access control policies at the kernel level. Enable and configure one of these frameworks to further enhance the security of your Docker containers.
9. Monitor Container Activity: Docker provides various monitoring and logging options that allow you to track and analyze container activity. Monitoring container logs and events can help you identify any suspicious behavior or potential security incidents.
10. Implement Image Scanning: Regularly scan your Docker images for known security vulnerabilities using image scanning tools like Docker Security Scanning or third-party services. These tools analyze the components and dependencies of your images to identify any vulnerabilities that need to be addressed.
By following these best practices, you can significantly improve the security of your Docker containers and reduce the risk of potential attacks or data breaches. Remember, securing your containers is an ongoing process, and it is essential to stay updated with the latest security practices and patches to ensure the safety of your applications and data.
Related Article: How to Mount a Host Directory as a Volume in Docker Compose
Working with Docker Volumes: Data Persistence
Docker volumes are an essential feature for ensuring data persistence in Docker containers. By default, Docker containers are ephemeral, which means that any data written inside a container is lost once the container is stopped or deleted. However, Docker volumes provide a way to store and share data between containers and the host system, ensuring that important data is not lost.
Creating a Docker Volume
To create a Docker volume, you can use the docker volume create
command followed by the volume name. For example, to create a volume named “myvolume”, you can use the following command:
$ docker volume create myvolume
Once the volume is created, you can use it in your Docker containers by referencing its name.
Mounting a Volume in a Container
To mount a volume in a container, you need to specify the volume name and the mount point inside the container. You can do this using the -v
or --volume
flag followed by the volume name and the mount point. For example:
$ docker run -v myvolume:/data myimage
In this example, the volume named “myvolume” is mounted at the “/data” directory inside the container.
Related Article: How to Use the Host Network in Docker Compose
Sharing Volumes between Containers
One of the advantages of Docker volumes is the ability to share data between containers. Multiple containers can mount the same volume, allowing them to access and modify the same data. This can be useful for scenarios where you have multiple services that need to work with the same data.
To share a volume between containers, you can simply mount the same volume in different containers using the same volume name and mount point. For example:
$ docker run -v myvolume:/shared-data container1 $ docker run -v myvolume:/shared-data container2
In this example, both “container1” and “container2” can access and modify the data inside the “myvolume” volume by using the “/shared-data” mount point.
Backing Up and Restoring Volumes
Backing up Docker volumes is crucial to ensure data integrity and recover from potential data loss. Docker provides several ways to back up and restore volumes, including using the docker cp
command to copy data from a volume to the host system.
To back up a volume, you can use the docker cp
command followed by the container ID or name and the source and destination paths. For example, to back up the “/data” directory from a container named “mycontainer” to the host system, you can use the following command:
$ docker cp mycontainer:/data /path/to/backup
To restore a volume from a backup, you can use the docker cp
command in the opposite direction, copying the data from the host system to the container’s volume.
Container Orchestration with Docker
Container orchestration plays a crucial role in managing and scaling containerized applications. Docker, being one of the most popular containerization platforms, provides powerful tools for orchestrating containers. We will introduce you to container orchestration with Docker and explore the benefits it offers.
Related Article: Copying a Directory to Another Using the Docker Add Command
What is Container Orchestration?
Container orchestration is the process of automating the deployment, management, and scaling of containers. It helps in efficiently running and coordinating multiple containers across a cluster of hosts. Orchestration platforms provide features such as service discovery, scaling, load balancing, high availability, and fault tolerance.
Why Use Container Orchestration?
Container orchestration simplifies the management of complex containerized applications by abstracting away the underlying infrastructure. Here are some key reasons to use container orchestration:
1. Scalability: Orchestration platforms enable you to easily scale your applications horizontally by adding or removing containers as per the demand.
2. High Availability: Orchestration tools monitor the health of containers and automatically restart or replace failed containers, ensuring high availability of your applications.
3. Load Balancing: Orchestration platforms distribute incoming traffic across multiple containers, optimizing resource utilization and improving performance.
4. Service Discovery: Orchestration tools provide built-in service discovery mechanisms, allowing containers to find and communicate with each other seamlessly.
Docker Swarm
Docker Swarm is Docker’s native clustering and orchestration solution. It allows you to create and manage a swarm of Docker nodes, turning them into a single virtual Docker engine. Swarm provides a simple yet powerful way to orchestrate containers using familiar Docker CLI commands.
Let’s take a look at an example of creating a swarm with Docker Swarm mode:
# Initialize a new Docker swarm $ docker swarm init # Join additional nodes to the swarm $ docker swarm join --token :
Once your swarm is up and running, you can deploy your services as Docker stacks, which are the declarative way of defining and managing applications in Swarm. Here’s an example of deploying a stack:
version: '3' services: web: image: nginx:latest ports: - 80:80 deploy: replicas: 3
The above Docker Compose file describes a service named “web” running three replicas of the latest Nginx image, with port 80 exposed.
Related Article: How to Use Environment Variables in Docker Compose
Kubernetes Integration
While Docker Swarm provides a built-in orchestration solution, Kubernetes has emerged as a popular container orchestration platform. If you prefer using Kubernetes, Docker provides seamless integration between Docker and Kubernetes.
You can use Docker Desktop to enable Kubernetes support and run Kubernetes clusters locally. Docker Desktop also provides a Kubernetes command-line tool, allowing you to interact with Kubernetes resources using familiar Docker commands.
To start a Kubernetes cluster using Docker Desktop, simply enable Kubernetes in the Docker settings. Once enabled, you can use the kubectl
command to manage your Kubernetes cluster.
Advanced Docker Performance Tuning: Tips and Techniques
Docker provides a powerful platform for running and managing containerized applications. However, as your containerized environment grows in complexity, it becomes essential to optimize Docker container performance to ensure efficient resource utilization and optimal application performance. We will explore advanced tips and techniques for fine-tuning Docker performance.
Minimize Container Size
Reducing the size of your Docker containers can have a significant impact on performance. Smaller containers have faster startup times and require fewer resources. Here are a few techniques to minimize container size:
– Use multi-stage builds: Multi-stage builds allow you to separate the build environment from the runtime environment, resulting in smaller final images.
FROM node:14 as build # Build your application FROM node:14 as runtime # Copy the built artifacts from the build stage COPY --from=build /app /app CMD ["node", "/app/index.js"]
– Remove unnecessary dependencies: Analyze your container dependencies and remove any unnecessary packages or libraries that are not required for your application.
Related Article: How to Stop and Remove All Docker Containers
Optimize Image Layers
Docker uses a layered filesystem for container images. Optimizing the layer structure can improve Docker performance. Here are a few techniques to optimize image layers:
– Combine commands: Combine multiple commands into a single RUN
instruction to reduce the number of layers created.
RUN apt-get update && \ apt-get install -y package1 package2 && \ apt-get clean
– Use .dockerignore
: Create a .dockerignore
file in your project directory to exclude unnecessary files and directories from the build context. This reduces the size of the build context and speeds up the build process.
# .dockerignore node_modules dist
Utilize Resource Limits
Setting resource limits for your containers prevents them from consuming excessive resources and impacting overall system performance. Docker provides several options to control resource allocation:
– CPU limits: Use the --cpus
flag to limit the maximum number of CPUs a container can use.
$ docker run --cpus 2 myapp
– Memory limits: Use the -m
flag to limit the amount of memory a container can use.
$ docker run -m 512m myapp
Fine-tune Docker Networking
Networking plays a crucial role in container performance. Here are a few tips to optimize Docker networking:
– Use host networking: If your container requires direct access to the host’s network stack, use the --net=host
flag to share the host’s network namespace.
$ docker run --net=host myapp
– Optimize DNS resolution: Configure Docker to use a faster DNS server or use a DNS caching service like dnsmasq
to improve DNS resolution performance.
Related Article: How to Force Docker for a Clean Build of an Image
Monitor and Analyze Performance
Monitoring and analyzing container performance is essential to identify bottlenecks and optimize Docker performance. Here are a few tools to help you monitor and analyze Docker container performance:
– Docker Stats: Use the docker stats
command to view real-time resource usage statistics for running containers.
$ docker stats mycontainer
– cAdvisor: Container Advisor is an open-source tool for monitoring resource usage and performance of running containers.
– Prometheus and Grafana: These popular monitoring tools can be used to collect and visualize performance metrics from Docker containers.
By implementing these advanced tips and techniques, you can optimize Docker container performance and ensure efficient resource utilization for your containerized applications.