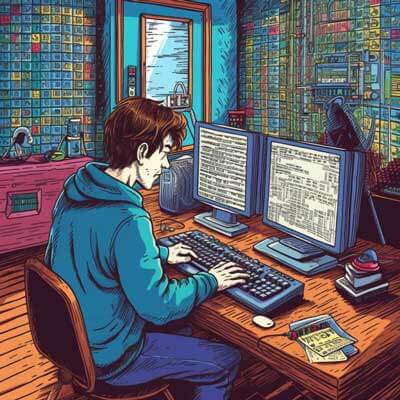
Environment variables are a fundamental concept in Docker that allow you to configure and customize your containers at runtime. They provide a way to pass information to your application without hardcoding values into your container image. In this guide, we will explore different methods to pass environment variables to Docker containers.
Method 1: Using the -e flag with the docker run command
The most common and straightforward way to pass environment variables to a Docker container is by using the -e
flag with the docker run
command. This method allows you to specify one or more environment variables and their values as command-line arguments.
Here’s the syntax for passing a single environment variable:
<a href="https://www.squash.io/how-to-run-a-docker-instance-from-a-dockerfile/">docker run</a> -e VARIABLE_NAME=variable_value image_name
And here’s an example of passing multiple environment variables:
docker run -e VAR1=value1 -e VAR2=value2 image_name
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
Method 2: Using a Docker Compose file
If you are using Docker Compose to manage your containers, you can define environment variables in the docker-compose.yml
file. This method is useful when you have multiple containers and want to manage their environment variables in a centralized configuration file.
To define an environment variable in a Docker Compose file, you can use the environment
key under the service definition. Here’s an example:
version: '3' services: myapp: image: myapp_image environment: - VAR1=value1 - VAR2=value2
In this example, the myapp
service will have two environment variables, VAR1
and VAR2
, with their respective values.
You can also use an environment file to define multiple environment variables. Create a file (e.g., env.list
) and define the variables inside it:
VAR1=value1 VAR2=value2
Then, reference the file in the Docker Compose file:
version: '3' services: myapp: image: myapp_image env_file: - ./env.list
Using a Docker Compose file provides a more structured and maintainable way to manage environment variables for your containers.
Method 3: Using the –env-file flag with the docker run command
Another way to pass environment variables to Docker containers is by using the --env-file
flag with the docker run
command. This method allows you to specify an environment file that contains a list of environment variables and their values.
Create an environment file (e.g., env.list
) and define the variables inside it:
VAR1=value1 VAR2=value2
Then, pass the file to the docker run
command using the --env-file
flag:
docker run --env-file env.list image_name
Using an environment file is useful when you have a large number of environment variables or when you want to keep them separate from the command-line arguments.
Best practices and recommendations
When working with environment variables in Docker containers, consider the following best practices:
1. Avoid sensitive information: Do not store sensitive information, such as passwords or API keys, as plain text environment variables. Instead, use a secrets management solution or a tool like Docker Secrets to securely pass sensitive data to your containers.
2. Use default values: Provide default values for your environment variables in case they are not explicitly set. This helps prevent unexpected behavior and allows your containers to work with sensible defaults.
3. Document your environment variables: Clearly document the purpose and expected values of each environment variable in your container documentation or README file. This helps other developers understand how to configure and use your container properly.
4. Use consistent naming conventions: Follow a consistent naming convention for your environment variables to make them more readable and maintainable. For example, use uppercase letters and underscores to separate words (e.g., DATABASE_HOST
, API_KEY
).
5. Avoid hardcoding values in the Dockerfile: Instead of hardcoding values directly into your Dockerfile, use environment variables to make your container image more configurable and reusable.
Related Article: How to Install and Use Docker