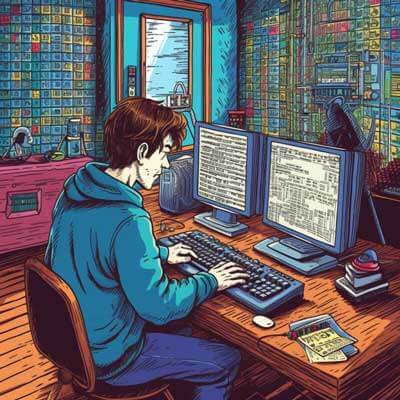
Table of Contents
To run a Docker instance from a Dockerfile, you can follow these step-by-step instructions:
Step 1: Create a Dockerfile
First, you need to create a Dockerfile. The Dockerfile is a text file that contains a set of instructions for building a Docker image. It specifies the base image to use, the dependencies to install, and the commands to run.
Here is an example of a simple Dockerfile that installs Node.js and runs a basic Node.js server:
# Use the official Node.js 14 image as the base image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy package.json and package-lock.json to the working directory COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code to the working directory COPY . . # Expose port 3000 EXPOSE 3000 # Start the Node.js server CMD [ "node", "server.js" ]
Related Article: How To Delete All Docker Images
Step 2: Build the Docker image
Once you have created the Dockerfile, you can build the Docker image using the docker build
command. Open a terminal or command prompt, navigate to the directory where the Dockerfile is located, and run the following command:
docker build -t my-node-app .
This command builds a Docker image with the tag my-node-app
using the Dockerfile in the current directory (.
). You can replace my-node-app
with any name you prefer for your image.
Step 3: Run a Docker container from the image
After building the Docker image, you can run a Docker container from it using the docker run
command. Run the following command in your terminal or command prompt:
<a href="https://www.squash.io/docker-how-to-workdir-run-cmd-env-variables/">docker run</a> -p 8080:3000 my-node-app
This command starts a Docker container from the my-node-app
image and maps port 3000 of the container to port 8080 on the host machine. You can access the Node.js server running inside the container by opening http://localhost:8080
in your web browser.
Alternative Approach: Using Docker Compose
Another way to run a Docker instance from a Dockerfile is by using Docker Compose. Docker Compose is a tool that allows you to define and run multi-container Docker applications using a YAML file.
Here is an example of a Docker Compose file (docker-compose.yml
) that defines a service based on the Dockerfile mentioned earlier:
version: "3" services: my-node-app: build: context: . dockerfile: Dockerfile ports: - "8080:3000"
To run the Docker instance using Docker Compose, navigate to the directory where the docker-compose.yml
file is located and run the following command:
docker-compose up
This command reads the docker-compose.yml
file, builds the Docker image based on the specified Dockerfile, and starts the container. The port mapping specified in the Compose file allows you to access the Node.js server running inside the container at http://localhost:8080
.
Related Article: How to Copy Files From Host to Docker Container
Best Practices
Here are some best practices to consider when running a Docker instance from a Dockerfile:
1. Use a specific version for the base image: Specify the version of the base image you are using in the Dockerfile to ensure consistency and avoid unexpected changes in future updates.
2. Minimize the number of layers: Each instruction in a Dockerfile creates a new layer in the Docker image. Minimize the number of layers by combining multiple instructions into one to reduce the overall image size and build time.
3. Cleanup unnecessary files: Remove any unnecessary files or dependencies after installing them to keep the image size small. This can be done using the RUN
instruction with appropriate cleanup commands.
4. Use .dockerignore
file: Create a .dockerignore
file in the same directory as your Dockerfile to exclude unnecessary files and directories from being copied into the Docker image. This helps to reduce the build context and improve build performance.
5. Tag and version your images: Give meaningful tags to your Docker images and consider versioning them to keep track of changes and easily roll back if needed.
These best practices can help you optimize the Docker image build process and ensure the resulting images are efficient, reliable, and easy to manage.