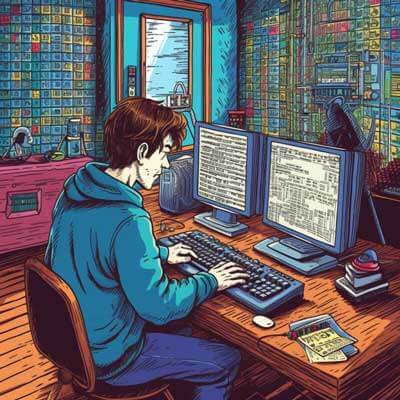
Table of Contents
What is Git Push?
Git push is a command used in Git to push or upload local changes to a remote repository. It allows you to share your changes with others who have access to the same repository. Pushing your changes to a remote repository is an essential step in the collaborative development process.
When you make changes to your files in a Git repository, those changes are initially only saved locally on your machine. Git push enables you to send those changes to a remote repository, such as one hosted on a platform like GitHub, GitLab, or Bitbucket.
Pushing your changes to a remote repository allows other developers to see and access your changes, collaborate with you, and ensure that the latest version of the codebase is always available to everyone working on the project.
In order to push your changes, you need to have write access to the remote repository. If you don't have write access, you won't be able to push your changes.
Here's a basic example of how to use the git push command:
$ git push <remote> <branch>
The <remote>
parameter specifies the remote repository that you want to push your changes to. This can be a URL, a repository name, or a remote alias defined in your Git configuration.
The <branch>
parameter specifies the branch that contains the changes you want to push. By default, Git will push the current branch you are on to the remote repository. However, you can also specify a different branch if needed.
For example, to push the changes on the current branch to the remote repository named "origin," you would use the following command:
$ git push origin master
In this example, "origin" is the remote repository's name, and "master" is the branch name. Git will push the changes on the "master" branch to the "origin" remote repository.
It's important to note that when you push changes to a remote repository, Git will only push the commits and objects that are not already present in the remote repository. This ensures that only the necessary changes are transferred, making the push operation efficient and fast.
If you encountered a conflict during the push operation, Git will notify you and require you to resolve the conflict before the push can be completed. Conflict resolution typically involves merging or rebasing your changes with the latest changes from the remote repository.
Understanding how to use the git push command is crucial for effective collaboration and version control in Git. It allows you to share your changes with others, keep the codebase up-to-date, and work seamlessly with remote repositories.
Related Article: How to Revert Multiple Git Commits
Understanding Remote Repositories
In Git, a remote repository is a version of your project that is hosted on a remote server, such as GitHub or Bitbucket. You can think of it as a way to collaborate with others or to back up your code.
When you clone a repository, Git automatically sets up a remote repository called "origin." This is typically the repository you cloned from. However, you can also add additional remote repositories if needed.
To see the list of remote repositories associated with your project, you can use the following command:
git remote -v
This will display the name and URL of each remote repository. The -v
option stands for "verbose" and provides more detailed information.
To add a new remote repository, you can use the following command:
git remote add <name> <url>
The <name>
is a convenient nickname for the remote repository, and the <url>
is the URL of the remote repository. For example, if you want to add a remote repository called "upstream" with the URL "https://github.com/upstream/repo.git", you would run the following command:
git remote add upstream https://github.com/upstream/repo.git
Once you have added a remote repository, you can use the following command to fetch the latest changes from it:
git fetch <remote>
The <remote>
is the name of the remote repository. For example, to fetch the latest changes from the "origin" repository, you would run the following command:
git fetch origin
This command downloads the latest changes from the remote repository and stores them in your local repository. However, it does not merge the changes with your current branch.
To merge the fetched changes with your branch, you can use the following command:
git merge <remote>/<branch>
The <remote>
is the name of the remote repository, and <branch>
is the name of the branch you want to merge with your current branch. For example, to merge the changes from the "origin/master" branch, you would run the following command:
git merge origin/master
Alternatively, you can use the following command to automatically fetch and merge the changes from a remote repository:
git pull <remote> <branch>
The <remote>
is the name of the remote repository, and <branch>
is the name of the branch you want to merge with your current branch. For example, to fetch and merge the changes from the "origin/master" branch, you would run the following command:
git pull origin master
These are the basic commands for working with remote repositories in Git. Understanding how to add, fetch, and merge changes from remote repositories is essential for collaborating with others and keeping your code up to date.
Setting Up a Remote Repository
To push your changes to a remote repository, you first need to set up the remote repository. A remote repository is a shared repository that can be accessed by multiple developers. It acts as a central location where everyone can push their changes.
There are several ways to set up a remote repository. One common approach is to use a hosting service like GitHub, GitLab, or Bitbucket. These services provide an easy way to create and manage remote repositories. Here's how you can set up a remote repository using GitHub as an example:
1. Sign up for a GitHub account if you don't have one already.
2. Create a new repository by clicking on the "New" button on the main page.
3. Give your repository a name and optionally add a description.
4. Choose whether you want the repository to be public or private.
5. Click on the "Create repository" button to create the repository.
Once the remote repository is set up, you need to connect it to your local repository. To do this, you'll need the URL of the remote repository. On GitHub, you can find the URL by clicking on the green "Code" button on the repository's main page.
Next, open your terminal or command prompt and navigate to your local repository's directory. Use the git remote add
command to add the remote repository. Replace <remote-name>
with a name of your choice (e.g., origin) and <remote-url>
with the URL of the remote repository.
git remote add <remote-name> <remote-url>
For example, if your remote repository is hosted on GitHub and its URL is https://github.com/your-username/your-repository.git
, you would use the following command:
git remote add origin https://github.com/your-username/your-repository.git
Now your local repository is connected to the remote repository. You can push your changes to the remote repository using the git push
command. We'll cover this in more detail in the next section.
Setting up a remote repository is an essential step in collaborating with others using Git. It allows you to share your changes with others and keep track of everyone's work in a centralized location.
Pushing Changes to a Remote Repository
Once you have made changes to your local repository, the next step is to push those changes to a remote repository. This allows you to share your changes with others and collaborate on the same project.
To push changes to a remote repository, you need to use the git push
command. The basic syntax of the command is as follows:
git push <remote> <branch>
The <remote>
parameter specifies the remote repository that you want to push your changes to. This could be a URL or the name of a remote repository that you have already set up. The <branch>
parameter specifies the branch that you want to push the changes from.
For example, if you want to push your changes to a remote repository named "origin" and the branch is called "master", you would use the following command:
git push origin master
By default, the git push
command only pushes the changes from the current branch. If you have multiple branches and want to push changes from all of them, you can use the --all
flag:
git push --all <remote>
This command will push all the branches to the specified remote repository.
It's important to note that when pushing changes to a remote repository, Git will only push the commits that are not already present in the remote repository. If someone else has pushed changes to the remote repository since your last pull, you will need to perform a pull operation before you can push your changes. This ensures that you have the latest changes from others before pushing your own.
If you want to push all the changes, including the commits that are already present in the remote repository, you can use the --force
flag:
git push --force <remote> <branch>
However, be cautious when using the --force
flag as it can overwrite the work of others and cause conflicts if not used properly.
You can also push tags to a remote repository using the git push
command. Tags are useful for marking specific points in your project's history, such as release versions. To push tags, you can use the --tags
flag:
git push --tags <remote>
This command will push all the tags to the specified remote repository.
That's it! Now you know how to push your changes to a remote repository using Git. Remember to always pull the latest changes before pushing to avoid conflicts and ensure that you have the most up-to-date version of the project.
Related Article: How To Fix 'Updates Were Rejected' Error In Git
Pushing Specific Branches
In Git, you can push changes to a remote repository by specifying the branch you want to push. This allows you to selectively push changes from a specific branch without pushing changes from other branches.
To push a specific branch, you need to specify both the remote repository and the branch name. The basic syntax for pushing a branch to a remote repository is:
git push <remote> <branch>
For example, to push the changes from the local branch "feature/new-feature" to the remote repository "origin", you would use the following command:
git push origin feature/new-feature
If the branch does not already exist on the remote repository, Git will create it for you. If the branch does exist, Git will update it with the changes you are pushing.
It's important to note that when you push a specific branch, only the branch you specify will be updated on the remote repository. Other branches will not be affected.
You can also push multiple branches at once by specifying them as arguments to the push command. For example, to push both the "feature/new-feature" and "bugfix/issue-123" branches to the remote repository "origin", you would use the following command:
git push origin feature/new-feature bugfix/issue-123
By pushing specific branches, you have more control over which changes are pushed to the remote repository. This can be useful when working on different features or bug fixes in different branches and only wanting to push the changes from a specific branch.
It's important to keep in mind that pushing changes to a remote repository will make them accessible to others who have access to the repository. Therefore, it's good practice to make sure you have tested and reviewed your changes before pushing them.
In the next chapter, we will explore how to push tags to a remote repository. Stay tuned!
To learn more about pushing branches in Git, you can refer to the official documentation on Pushing Branches.
Pushing Tags
In Git, tags are used to mark specific points in the history of a project. They can be used to label significant milestones, releases, or any other important points in the development process.
To push tags to a remote repository, you can use the git push
command with the --tags
option. This will push all tags that exist in your local repository to the remote repository.
Here's an example of how to push tags to a remote repository:
git push --tags
This command will push all tags to the default remote repository. If you want to push tags to a specific remote, you can specify it as an argument to the command:
git push <remote-name> --tags
Replace <remote-name>
with the name of the remote repository you want to push the tags to.
It's important to note that pushing tags doesn't automatically push the associated commits. If you want to push both the tags and their associated commits, you can use the --follow-tags
option:
git push --follow-tags
This command will push both the tags and their associated commits to the remote repository.
If you only want to push a specific tag, you can use the git push
command followed by the tag name:
git push <remote-name> <tag-name>
Replace <tag-name>
with the name of the tag you want to push.
Additionally, you can delete tags from the remote repository by using the git push
command with the --delete
option followed by the tag name:
git push <remote-name> --delete <tag-name>
This command will delete the specified tag from the remote repository.
Inspecting Pushed Changes
Once you have pushed your changes to a remote repository using Git, it is often necessary to inspect the pushed changes to verify that they were successfully applied. Git provides several ways to inspect the changes that were pushed.
One way to inspect the pushed changes is by using the git log
command. This command allows you to view the commit history, including the commit messages and the changes made in each commit. By specifying the branch name or commit hash, you can limit the output to only the commits that were pushed.
$ git log origin/master
This command will display the commit history of the origin/master
branch. You can replace origin/master
with the name of any remote branch.
Another way to inspect the pushed changes is by using the git diff
command. This command allows you to view the differences between the commit that was pushed and its parent commit. By specifying the commit hash or branch name, you can see the changes made in that specific commit.
$ git diff origin/master^..origin/master
This command will display the differences between the commit referenced by origin/master^
(the parent commit) and the commit referenced by origin/master
(the pushed commit). Again, you can replace origin/master
with the name of any remote branch.
You can also inspect the pushed changes by using a visual Git tool, such as GitKraken or SourceTree. These tools provide a graphical interface to view the commit history, changes, and branches. They can make it easier to navigate and inspect the pushed changes, especially for complex projects with many commits.
Inspecting the pushed changes is crucial to ensure that your changes were applied correctly and as intended. By using the git log
and git diff
commands, as well as visual Git tools, you can easily review the changes made in each push and verify their correctness.
In the next chapter, we will explore how to revert or undo a push in Git. Stay tuned!
Handling Conflicts when Pushing
When working with a team on a project using Git, there may be times when conflicts arise when pushing changes to a remote repository. These conflicts occur when multiple team members make changes to the same file or lines of code, and Git is unable to automatically merge the changes.
To handle conflicts when pushing, you need to resolve these conflicts manually. Here's a step-by-step guide on how to do it:
1. Before pushing your changes, make sure to pull the latest changes from the remote repository. This will help you identify any conflicts before pushing your own changes.
2. If Git detects conflicts during the pull operation, it will mark the conflicting files and lines with special markers. These markers indicate the conflicting sections and the changes made by different team members.
$ git pull origin master
Auto-merging file.txt
CONFLICT (content): Merge conflict in file.txt
Automatic merge failed; fix conflicts and then commit the result.
3. Open the conflicting file(s) in your preferred text editor. Inside the file, you will see the conflict markers that indicate the conflicting sections. The markers typically look like this:
<<<<<<< HEAD
// Your changes
=======
// Remote changes
>>>>>>> 1234567890abcdef
The
<<<<<<< HEAD
marker represents the changes you made locally, while the>>>>>>> 1234567890abcdef
marker represents the remote changes.
4. Review the conflicting sections and decide how to resolve the conflict. You may choose to keep your changes, discard them, or merge them with the remote changes. Edit the file accordingly to resolve the conflict.
5. Once you have resolved all conflicts, save the file and stage it for commit. Use the following command to stage the file:
$ git add file.txt
6. After staging the file, you can now continue with the push operation. Use the
git push
command to push your changes to the remote repository:
$ git push origin master
If there are no further conflicts, your changes will be pushed successfully.
It's important to communicate with your team members when conflicts occur and collaborate on resolving them. Git provides powerful tools for resolving conflicts, such as merge tools and graphical interfaces. However, understanding the manual conflict resolution process is essential to effectively handle conflicts when pushing changes to a remote repository.
Related Article: How To Combine My Last N Commits In Git
Pushing to Multiple Remote Repositories
Pushing changes to a single remote repository is a common task when working with Git. However, there may be situations where you want to push your changes to multiple remote repositories simultaneously. This can be useful, for example, when collaborating with multiple teams or when deploying your code to different environments.
To push to multiple remote repositories, you can simply add multiple remote URLs to your local Git repository. You can do this using the
git remote add
command followed by the name of the remote and the URL.
Let's say you have an existing remote named "origin" that points to your main repository. To add another remote repository, you can use the following command:
git remote add <remote-name> <remote-url>
For example, if you want to add a remote named "backup" with the URL "git@github.com:user/repo.git", you would run:
git remote add backup git@github.com:user/repo.git
Once you have added the remote repositories, you can push your changes to all of them by specifying the remote name when pushing. The syntax for pushing to a remote repository is:
git push <remote> <branch>
For example, to push the changes in your local branch "master" to both the "origin" and "backup" repositories, you would run:
git push origin master git push backup master
By running these commands, Git will push the changes to both remote repositories, keeping them in sync.
It's important to note that each remote repository can have its own branch tracking configuration. This means that you can push your changes to different branches in each remote repository, if necessary.
To remove a remote repository, you can use the
git remote remove
command followed by the remote name. For example, to remove the "backup" remote, you would run:
git remote remove backup
Pushing Changes to Forked Repositories
When working with open-source projects, it is common to fork a repository to make your own changes without affecting the original codebase. Once you have made the desired changes in your forked repository, you can push those changes back to the original repository. In this section, we will explore how to push changes to forked repositories using Git.
1. Adding the Original Repository as a Remote
To push changes from a forked repository, you need to add the original repository as a remote. This allows you to fetch and push changes between the two repositories. To add the original repository as a remote, you can use the following command:
git remote add upstream <original_repository_url>
For example, if the original repository's URL is "https://github.com/original/repo.git", you would run:
git remote add upstream https://github.com/original/repo.git
2. Fetching Changes from the Original Repository
Before pushing your changes, it is a good practice to fetch the latest changes from the original repository. This ensures that you have the most up-to-date codebase before merging your changes. To fetch the changes, use the following command:
git fetch upstream
This command fetches the latest changes from the upstream remote repository.
Related Article: How to Make Git Stop Tracking a File in .Gitignore
3. Merging Changes from the Original Repository
Once you have fetched the latest changes, you can merge them into your local branch. This step is essential to resolve any conflicts that may arise between your changes and the changes made in the original repository. To merge the changes, use the following command:
git merge upstream/master
This command merges the changes from the upstream remote's master branch into your current branch.
4. Pushing Changes to Your Forked Repository
After merging the changes from the original repository, you can push the changes to your forked repository. To push the changes, use the following command:
git push origin branch_name
Replace
branch_name
with the name of the branch you want to push the changes to. For example, if you want to push the changes to a branch named "feature", you would run:
git push origin feature
This command pushes the changes to the specified branch in your forked repository.
5. Creating a Pull Request
Once you have pushed the changes to your forked repository, you can create a pull request to propose the changes to the original repository. A pull request allows the original repository's maintainers to review and merge your changes. To create a pull request, navigate to your forked repository on the hosting platform (e.g., GitHub), select the branch containing your changes, and click on the "New Pull Request" button.
By following these steps, you can effectively contribute to open-source projects and collaborate with other developers to enhance software projects.
Using Git Push with SSH
Git provides several protocols for pushing changes to a remote repository, including HTTPS, SSH, and Git. In this chapter, we will focus on using Git push with SSH.
Using SSH for Git push offers several advantages, such as increased security and the ability to authenticate using public and private keys. It is a preferred method for many developers working with Git.
To use Git push with SSH, you need to have SSH keys set up on both your local machine and the remote repository server.
Related Article: How to Remove a File From the Latest Git Commit
Generating SSH keys
If you don't already have SSH keys set up, you can generate them using the following command:
$ ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command generates a new SSH key pair using the RSA algorithm with a key length of 4096 bits. Replace "your_email@example.com" with your own email address.
After running this command, you will be prompted to choose a location to save the key pair. The default location is usually fine, but you can choose a different one if desired.
Once the SSH keys are generated, you need to add the public key to your Git hosting provider. The process may vary depending on the provider, but generally, you can find an option to add SSH keys in your account settings.
Configuring SSH for Git
Next, you need to configure Git to use SSH for pushing changes. Open a terminal and run the following command:
$ git config --global remote.origin.url git@github.com:username/repository.git
Replace "username" with your Git username and "repository" with the name of your repository.
With this configuration, Git will use SSH when pushing changes to the remote repository. You can verify the remote URL by running:
$ git remote -v
If everything is set up correctly, you should see the SSH URL for the remote repository.
Pushing Changes with Git Push
Once SSH is configured, you can push your local changes to the remote repository using the Git push command. The basic syntax for Git push is:
$ git push <remote> <branch>
Replace "
" with the name of the remote repository, and " " with the name of the branch you want to push.
For example, to push changes to the "master" branch of the remote repository, you would run:
$ git push origin master
This command pushes the local changes to the "master" branch of the remote repository named "origin".
If you have already set the upstream branch, you can simply use:
$ git push
Git will push the changes to the corresponding branch on the remote repository.
Remember that when using Git push with SSH, you may be prompted to enter your SSH passphrase or provide your SSH key's password. This is normal and part of the SSH authentication process.
Using Git push with SSH provides a secure and efficient way to push changes to a remote repository. By following the steps outlined in this chapter, you can start using SSH for your Git push operations.
Using Git Push with HTTPS
When using Git to push changes to a remote repository, you have the option to use either HTTPS or SSH protocols. In this chapter, we will focus on using Git push with HTTPS.
HTTPS is the recommended protocol for most users, especially if you are new to Git or if you don't have SSH access to the remote repository. It provides a secure and encrypted connection between your local machine and the remote repository.
To push changes to a remote repository using HTTPS, you need to have the repository URL handy. This URL can be found on the repository page of your hosting provider, such as GitHub, GitLab, or Bitbucket.
Let's say you have a local Git repository and you want to push your changes to a remote repository on GitHub. Here's how you can do it:
1. First, navigate to your local repository's directory using the command line or your preferred Git client.
2. Next, add the remote repository URL as a remote to your local repository using the following command:
$ git remote add origin https://github.com/username/repository.git
Make sure to replace "username" with your GitHub username and "repository" with the name of the repository you want to push to.
3. After adding the remote repository, you can confirm that it was added successfully by running the following command:
$ git remote -v
This will show you a list of all the remote repositories associated with your local repository.
4. Now, you can push your changes to the remote repository using the Git push command:
$ git push origin master
In this example, we are pushing changes to the "master" branch. Replace "master" with the name of the branch you want to push to.
5. Git will prompt you to enter your GitHub username and password to authenticate your push. Enter the required credentials and press Enter.
6. If everything goes well, Git will upload your changes to the remote repository and display a success message.
That's it! You have successfully pushed your changes to a remote repository using HTTPS. Make sure to keep your repository URL and credentials secure to protect your code.
Remember that HTTPS authentication requires entering your credentials every time you push changes. If you want to avoid entering your credentials repeatedly, you can use Git's credential caching or set up SSH authentication for your remote repository.
Using Git push with HTTPS is a convenient and secure way to collaborate and share your code with others. It provides a straightforward method for pushing your changes to a remote repository without the need for SSH access.
In the next chapter, we will explore how to use Git push with SSH, which offers a more streamlined and secure authentication process. Stay tuned!
Feel free to check out the official Git documentation on https://git-scm.com/book/en/v2 for more details on using Git push with HTTPS.
Related Article: How to Pull Latest Changes for All Git Submodules
Using Git Push with Personal Access Tokens
Git push is a command used to send local commits to a remote repository. By default, Git uses Secure Shell (SSH) to authenticate and establish a secure connection with the remote repository. However, there are scenarios where using personal access tokens (PATs) can be more convenient or necessary.
A personal access token is an alternative way to authenticate with a remote repository, particularly when using Git with services like GitHub, GitLab, or Bitbucket. These tokens act as a substitute for passwords and can provide fine-grained control over the actions a user can perform.
To use a personal access token with Git push, you need to generate the token first. The process varies depending on the remote repository service you are using. Let's take GitHub as an example:
1. Go to the GitHub website and log in to your account.
2. Click on your profile picture in the top-right corner, and then click on "Settings".
3. In the left sidebar, click on "Developer settings".
4. Select "Personal access tokens" from the options.
5. Click on the "Generate new token" button.
6. Give your token a name and select the desired scopes or permissions.
7. Click on the "Generate token" button.
8. GitHub will generate a new personal access token for you. Make sure to copy it and keep it in a safe place, as you won't be able to see it again.
Once you have your personal access token, you can use it with Git push by modifying the remote repository URL. Instead of using the SSH URL format, you will use the HTTPS format with your token embedded in the URL.
Here's an example of how to modify the remote URL for a Git repository:
$ git remote set-url origin https://<username>:<token>@github.com/<username>/<repository>.git
Replace
<username>
with your GitHub username and<token>
with your personal access token.<repository>
should be replaced with the name of the repository you want to push your changes to.
After modifying the remote URL, you can use the regular Git push command to push your commits to the remote repository:
$ git push origin <branch>
Replace
<branch>
with the name of the branch you want to push.
Using personal access tokens with Git push provides an alternative authentication method that can be useful in various situations. It allows you to securely push your changes to a remote repository without relying on SSH keys or passwords. Remember to keep your personal access tokens confidential and avoid sharing them with others.
For more information on generating personal access tokens for different services, consult their respective documentation:
- GitHub: Creating a personal access token
- GitLab: Personal access tokens
- Bitbucket: App passwords
Pushing Changes with Force
Sometimes, you may find yourself in a situation where you need to forcefully push your changes to a remote repository, even if it means overwriting existing commits. This can be useful when you want to discard or replace certain commits, or when you're collaborating with other developers and need to update the remote repository with your changes.
To force push your changes, you can use the
git push --force
command. This command allows you to update the remote repository by overwriting its commit history with your local commits. However, it's important to use this command with caution, as it can potentially cause data loss or conflicts if not used correctly.
Before force pushing, it's recommended to make sure that you've committed all your changes locally. You can use the
git status
command to check if there are any uncommitted changes. If there are, you should commit them before proceeding.
To force push your changes to a remote repository, follow these steps:
1. First, make sure you're on the branch that you want to push. You can use the
git branch
command to check your current branch and switch to a different branch if needed.
2. Next, use the
git push --force
command followed by the remote repository name and branch name. For example, if you want to force push your changes to the "master" branch of a remote repository named "origin", you would run the following command:git push --force origin master
3. Once you execute the command, Git will overwrite the remote repository's commit history with your local commits. If there are any conflicts between your local commits and the remote commits, Git will display an error message and you'll need to resolve the conflicts before proceeding.
It's important to note that force pushing can have serious consequences, especially when working in a team. If other developers have pulled the previous commits that you're overwriting, they may encounter issues when trying to merge their changes with the updated commit history. It's crucial to communicate with your team and make sure they're aware of the force push you're planning to perform.
Force pushing should generally be used as a last resort when other options, such as merging or rebasing, are not feasible. It's always a good practice to discuss with your team and follow any established guidelines or workflows before force pushing.
Pushing Changes with Dry Run
When working with Git, it's important to understand the consequences of pushing your changes to a remote repository. One way to ensure that you are pushing the correct changes without actually modifying the remote repository is by using the "dry run" option.
The dry run option allows you to simulate a push operation without actually pushing any changes. This can be useful when you want to verify that your local repository is in sync with the remote repository before pushing your changes.
To perform a dry run, you can use the following command:
git push --dry-run
Running this command will display a list of the commits that would be pushed if the dry run was not enabled. This allows you to review the changes that are about to be pushed without modifying the remote repository.
Here's an example of the output you can expect from the dry run command:
To https://github.com/username/repository.git 3de4f56..7a8b9c0 branch-name -> branch-name
In this example, the output shows that the commits between the local branch and the remote branch will be pushed if the dry run was not enabled. The commit range is displayed as
3de4f56..7a8b9c0
, indicating the starting and ending commit hashes.
By reviewing the output of the dry run, you can ensure that you are only pushing the intended changes and avoid accidentally pushing unwanted changes.
It's important to note that the dry run option does not modify any files or branches in your local or remote repository. It's purely a simulation to provide you with information about the changes that would be pushed.
In addition to the
--dry-run
option, you can also use the--dry-run=check
option. This option performs additional checks, such as verifying that the local branch is up to date with the remote branch, before performing the dry run. This can be useful in scenarios where you want to ensure that your local repository is in sync with the remote repository.
To summarize, using the dry run option with the
git push
command allows you to simulate a push operation without actually modifying the remote repository. This can help you verify that you are pushing the correct changes before actually pushing them.
Pushing Changes with Verbose Output
When pushing changes to a remote repository using Git, you can use the
--verbose
or-v
option to get more detailed output about the push operation. This can be helpful for understanding what exactly is happening during the push and for troubleshooting any issues that may arise.
The verbose output provides information such as the objects being sent, the references being updated, and the progress of the push. It can help you identify any conflicts or errors that occur during the push.
To push changes with verbose output, you can use the following command:
git push --verbose
or
git push -v
This will display a detailed output of the push operation, showing each object being sent and each reference being updated. For example:
Pushing to https://github.com/username/repository.git Counting objects: 5, done. Delta compression using up to 4 threads. Compressing objects: 100% (3/3), done. Writing objects: 100% (3/3), 291 bytes | 291.00 KiB/s, done. Total 3 (delta 1), reused 0 (delta 0), pack-reused 0 To https://github.com/username/repository.git abc123..def456 main -> main
In this example, the push operation is pushing 3 objects and updating the
main
branch. The verbose output provides information about the progress and status of each step in the push.
Using the verbose output can be especially useful when troubleshooting issues with the push. For example, if you encounter any conflicts during the push, the verbose output will show you which files are causing the conflicts and provide more information about the conflicts themselves.
By default, Git only displays a summary of the push operation. However, using the
--verbose
or-v
option allows you to get a more detailed view of what is happening behind the scenes.
Next, we will explore how to push specific branches to a remote repository.
Related Article: How to Stash Untracked Files in Git
Using Hooks with Git Push
Git hooks are scripts that can be triggered at various points in Git's workflow. They allow you to customize and automate certain actions before or after specific Git commands. Hooks can be a powerful tool to enforce code quality, run tests, or perform other custom actions.
When it comes to the
git push
command, Git provides two types of hooks that can be useful: the pre-push hook and the post-push hook.
The Pre-Push Hook
The pre-push hook is executed before the remote repository is updated with your changes. It allows you to verify the changes you are about to push and prevent the push if certain conditions are not met. This can be useful to enforce code standards, validate tests, or perform any other checks you deem necessary.
To use the pre-push hook, you need to create an executable script file named
pre-push
inside the.git/hooks
directory of your local repository. Here's an example of a pre-push hook written in Bash:
#!/bin/bash # Run tests before pushing npm test # Prevent push if tests fail if [ $? -ne 0 ]; then echo "Tests failed. Push aborted." exit 1 fi
In this example, the pre-push hook runs the
npm test
command to execute the tests. If the tests fail (indicated by a non-zero exit code), the hook displays an error message and aborts the push by exiting with a non-zero status code.
The Post-Push Hook
The post-push hook, as the name suggests, is executed after the remote repository has been updated with your changes. It can be used to trigger additional actions or notifications based on the pushed changes. For example, you could notify a team chat channel about the new changes or trigger a deployment process.
To use the post-push hook, you need to create an executable script file named
post-push
inside the.git/hooks
directory of your local repository. Here's an example of a post-push hook written in Python:
#!/usr/bin/env python import requests # Send a notification to a webhook def send_notification(): webhook_url = "https://example.com/webhook" payload = { "text": "New changes pushed to the repository." } response = requests.post(webhook_url, json=payload) if response.status_code != 200: print("Failed to send notification.") # Trigger the notification send_notification()
In this example, the post-push hook uses the
requests
library to send a notification to a webhook URL. You can customize the payload and the destination URL to fit your needs.
Remember to make the hook script executable by running
chmod +x .git/hooks/post-push
(orpre-push
) in your terminal.
Hooks can greatly enhance your Git workflow by automating repetitive tasks or ensuring code quality. However, it's important to use them responsibly and avoid excessive or unnecessary checks that could slow down the development process.
Now that you understand how to use hooks with
git push
, you can take advantage of this feature to streamline your workflow and enforce best practices.
Pushing Changes in a Team Environment
In a team environment, collaborating on a project requires seamless integration of code changes made by different team members. Git provides a reliable and efficient way to push changes to a remote repository, allowing team members to share their work and keep the project up to date.
When working on a team project, it is essential to follow a consistent workflow to avoid conflicts and ensure smooth collaboration. Here are some best practices for pushing changes in a team environment:
1. Pull Before Push: Before pushing your changes to the remote repository, it is recommended to pull the latest changes from the remote repository. This ensures that your local copy is up to date and reduces the chances of conflicts when pushing your changes. You can use the following command to pull the latest changes:
git pull2. Resolve Conflicts: If there are conflicts between your local changes and the changes in the remote repository, Git will prompt you to resolve them before pushing. Conflicts occur when both you and another team member have made changes to the same lines of code. It is crucial to carefully review and resolve these conflicts to maintain code integrity. Git provides tools to help you resolve conflicts, such as merging or using a visual merge tool.
3. Create a New Branch: In a team environment, it is good practice to create a new branch for each feature or bug fix. This allows team members to work on different parts of the project simultaneously without interfering with each other's work. To create a new branch, use the following command:
git checkout -b new-branch4. Push to the Remote Repository: Once you have resolved any conflicts and are ready to push your changes to the remote repository, use the following command:
git push origin branch-name
Replace
branch-name
with the name of the branch you want to push. If you are pushing to the default branch, typicallymaster
ormain
, you can omit the branch name.5. Review and Merge Pull Requests: In a team environment, it is common to use pull requests as a way to review and merge changes into the main branch. Pull requests allow team members to review the changes made by others and provide feedback before merging. This helps maintain code quality and ensures that the project remains stable. Tools like GitHub and Bitbucket provide a user-friendly interface for creating and managing pull requests.
By following these best practices, you can effectively push changes in a team environment and collaborate seamlessly with your team members. Remember to communicate with your team and follow the established guidelines to ensure a smooth workflow.
Related Article: How to Undo Pushed Commits Using Git
Best Practices for Git Push
Pushing changes to a remote repository in Git is an essential part of the development workflow. It allows you to share your changes with other team members and collaborate effectively. However, it is important to follow some best practices to ensure a smooth and error-free push process. In this chapter, we will discuss some of these best practices.
1. Commit and Push Frequently
It is a good practice to commit your changes frequently and push them to the remote repository. This helps in keeping a track of your work and ensures that your changes are backed up. By committing frequently, you can easily revert to a previous state if needed. Pushing regularly also reduces the chances of merge conflicts when collaborating with others.
To commit and push your changes, you can use the following commands:
git add . git commit -m "Your commit message" git push
2. Review Your Changes Before Pushing
Before pushing your changes, it is important to review them carefully. Use the
git status
command to see which files have been modified and are ready to be pushed. You can also usegit diff
to see the exact changes made to each file.
git status git diff
Reviewing your changes allows you to catch any mistakes or unintended modifications before they are pushed to the remote repository.
3. Pull Before Pushing
Before pushing your changes, it is a good practice to pull the latest changes from the remote repository. This ensures that you are working on the most up-to-date version of the codebase and reduces the chances of conflicts.
To pull the latest changes, you can use the following command:
git pull
If there are any conflicts, Git will prompt you to resolve them before you can push your changes.
Related Article: How to Implement Database Sharding in PostgreSQL
4. Use Descriptive Commit Messages
When committing your changes, it is important to use descriptive commit messages that explain the purpose of the changes. This helps in understanding the context of the changes and makes it easier to track the history of the codebase.
A good commit message should be concise yet informative. It should start with a capitalized verb in the present tense, followed by a brief description. For example:
git commit -m "Fix issue with login validation"
Avoid using generic or vague commit messages like "Update code" or "Bug fixes" as they don't provide much information.
5. Push Only What is Necessary
When pushing your changes, it is a best practice to only push what is necessary. Avoid pushing unrelated or incomplete changes that are still work in progress. This keeps the commit history clean and makes it easier to understand the purpose of each commit.
Before pushing, you can use the
git diff --cached
command to see the changes that are staged for commit. This helps in ensuring that you are only pushing the intended changes.
git diff --cached
By following these best practices, you can ensure a smooth and efficient Git push process. Commit and push frequently, review your changes before pushing, pull before pushing, use descriptive commit messages, and push only what is necessary. These practices will help you collaborate effectively with your team and maintain a clean and organized codebase.
Real World Examples of Git Push
In this chapter, we will explore some real-world examples of using the
git push
command to push changes to a remote repository. These examples will help you understand how to effectively use this command in different scenarios.Example 1: Pushing changes to a shared repository
Let's say you are working on a team project where multiple developers have access to a shared remote repository. After making some changes to your local repository, you need to share these changes with your teammates. Here's how you can do it:
$ git add . $ git commit -m "Implement new feature" $ git push origin master
In this example, we assume that the remote repository is named "origin" and the branch you want to push to is "master". The
git push
command will push your local changes to the remote repository, allowing your teammates to access and review your code.Example 2: Pushing changes to a specific branch
Sometimes, you may want to push your changes to a branch other than the default branch (usually "master"). Here's an example of how you can achieve that:
$ git add . $ git commit -m "Fix bug" $ git push origin feature-branch
In this example, we assume that you are working on a feature branch named "feature-branch". By specifying the branch name after the
git push
command, you can push your changes to that specific branch on the remote repository.Example 3: Pushing tags to a remote repository
Tags are useful for marking specific points in your project's history, such as releases or important milestones. To push tags to a remote repository, you can use the
git push
command with the--tags
option. Here's an example:
$ git tag v1.0.0 $ git push origin --tags
In this example, we first create a tag named "v1.0.0" using the
git tag
command. Then, we usegit push
with the--tags
option to push the tag to the remote repository named "origin". This allows other users to see and access the tagged version of the code.Example 4: Pushing changes to multiple remote repositories
In some cases, you may need to push your changes to multiple remote repositories, such as when you have a backup repository or when collaborating with developers from different organizations. Here's an example:
$ git add . $ git commit -m "Update documentation" $ git push origin master $ git push backup-repo master
In this example, we assume that you have two remote repositories: "origin" and "backup-repo". By executing the
git push
command multiple times, specifying different remote repositories, you can push your changes to each of them.
These real-world examples should give you a better understanding of how to use the
git push
command in different scenarios. Experiment with these examples in your own projects to become comfortable with pushing changes to remote repositories.
Advanced Techniques for Git Push
Git push is a powerful command that allows you to send your local commits to a remote repository. While the basic usage of git push is straightforward, there are additional techniques that can enhance your workflow and help you manage your code more effectively.
Related Article: How to Obtain the Current Branch Name in Git
1. Pushing Specific Branches
By default, git push sends all local branches to the remote repository. However, you can specify a particular branch to push by using the following syntax:
git push <remote> <branch>
For example, to push the changes from your local "feature" branch to the "origin" remote repository, you would use:
git push origin feature
This can be particularly useful when you are working on multiple branches simultaneously and only want to push changes from a specific branch.
2. Force Pushing
Sometimes, you may need to overwrite the history of a remote branch with your local changes. This can be done using the
--force
flag:
git push --force <remote> <branch>
However, be cautious when using this command, as it can potentially discard other developers' work if they have based their changes on the previous version of the branch.
3. Pushing Tags
Git tags are used to mark specific points in your commit history, such as releases or major milestones. To push tags to a remote repository, you can use the
--tags
flag:
git push --tags <remote>
This command pushes all local tags to the specified remote repository.
4. Configuring Push Behaviors
Git provides several configuration options that allow you to customize the behavior of the push command. Two commonly used options are:
push.default
: This option determines the default behavior when pushing branches. It can be set tosimple
,matching
, orcurrent
. For most cases, thesimple
option is recommended as it pushes the current branch to the remote branch with the same name.push.followTags
: When set totrue
, this option ensures that tags are pushed along with the branch they are associated with. This can be useful when you want to share tags with the remote repository.
You can set these options using the git config command:
git config --global push.default simple git config --global push.followTags true
Related Article: How to Force Overwrite During Git Merge
5. Pushing to Multiple Remote Repositories
Git allows you to push your changes to multiple remote repositories at once. To achieve this, you can configure multiple push URLs for a remote using the git remote
command.
git remote set-url --add --push <remote> <url>
For example, to add a second remote repository called "upstream" with the URL "https://github.com/upstream/repository.git", you would use:
git remote set-url --add --push origin https://github.com/origin/repository.git git remote set-url --add --push upstream https://github.com/upstream/repository.git
After configuring multiple push URLs, you can use the regular git push
command to push your changes to all configured remotes simultaneously.
These advanced techniques for git push can help you tailor the command to fit your specific needs and make your Git workflow more efficient and flexible. Experiment with these techniques and explore other git push options to further enhance your version control capabilities.