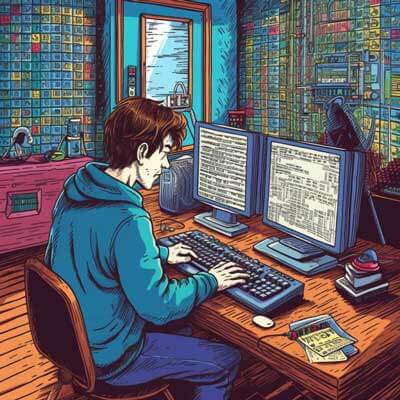
- Introduction to Database Sharding
- Example: Sharding a User Database
- Understanding Sharding with PostgreSQL
- Sharding Techniques in PostgreSQL
- Example: Partitioning a Sales Table
- List of PostgreSQL Sharding Features
- 1. Partitioning
- Example: Range Partitioning
- 2. Replication
- Example: Setting up Logical Replication
- 3. Distributed Queries
- Example: Creating a Foreign Table
- 4. Connection Pooling
- Example: Configuring pgBouncer
- Using PostgreSQL Sharding Features: Partitioning
- Example: Range Partitioning
- Example: List Partitioning
- Using PostgreSQL Sharding Features: Replication
- Logical Replication
- Example: Setting up Logical Replication
- Physical Replication
- Example: Setting up Physical Replication
- Using PostgreSQL Sharding Features: Distributed Queries
- Example: Creating a Foreign Table
- Example: Joining Tables from Different Shards
- Using PostgreSQL Sharding Features: Connection Pooling
- Example: Configuring pgBouncer
- Third-Party Tools for Sharding in PostgreSQL
- Citus
- Postgres-XL
- Pros and Cons of Sharding in PostgreSQL
- Pros of Sharding
- Cons of Sharding
- Use Cases for Sharding
- Best Practices for Sharding in PostgreSQL
- Real World Examples of Sharding in PostgreSQL
- 1. Uber
- 2. Instagram
- 3. Airbnb
- Performance Considerations for Sharding in PostgreSQL
- Query Routing Overhead
- Data Distribution and Skew
- Indexing Strategies
- Query Optimization
- Advanced Sharding Techniques for PostgreSQL
- Hash Sharding
- Consistent Hashing
- Code Snippet Ideas: Sharding in PostgreSQL – Part 1
- 1. Creating a Range-Partitioned Table
- 2. Setting up Logical Replication
- Code Snippet Ideas: Sharding in PostgreSQL – Part 2
- 1. Creating a Foreign Table
- 2. Configuring pgBouncer
- Code Snippet Ideas: Sharding in PostgreSQL – Part 3
- 1. Creating a Hash-Partitioned Table
- 2. Using Consistent Hashing for Sharding
- Code Snippet Ideas: Sharding in PostgreSQL – Part 4
- 1. Creating a Custom Sharding Function
- 2. Routing Queries to Specific Shards
- Code Snippet Ideas: Sharding in PostgreSQL – Part 5
- 1. Handling Errors in Sharded Databases
- 2. Retry Logic for Sharded Databases
Introduction to Database Sharding
Database sharding is a technique used to horizontally partition large databases into smaller, more manageable pieces called shards. Each shard contains a subset of the data and can be hosted on separate servers. Sharding is commonly employed in scenarios where there is a need to scale the database horizontally in order to handle large amounts of data or high traffic loads.
Sharding offers several benefits, including improved performance, increased storage capacity, and enhanced availability. By distributing the data across multiple shards, read and write operations can be distributed, resulting in faster query response times. Additionally, sharding allows for the allocation of more storage capacity as the database grows, as each shard can be hosted on a separate server with its own storage resources. Furthermore, sharding can improve availability by reducing the impact of hardware failures on the overall system.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Example: Sharding a User Database
To illustrate the concept of database sharding, let’s consider a scenario where we have a user database with millions of users. Instead of storing all the user records in a single database, we can shard the database based on a shard key, such as the user ID or a geographical region. Each shard would then contain a subset of the user records.
For example, suppose we have four shards, and we decide to shard the user database based on the user ID mod 4. The data distribution would look as follows:
– Shard 1: User IDs 0, 4, 8, 12, …
– Shard 2: User IDs 1, 5, 9, 13, …
– Shard 3: User IDs 2, 6, 10, 14, …
– Shard 4: User IDs 3, 7, 11, 15, …
Understanding Sharding with PostgreSQL
PostgreSQL, a powerful open-source relational database management system, supports sharding through various techniques and features. Understanding how sharding works in PostgreSQL is essential for effectively implementing and managing a sharded database deployment.
Sharding Techniques in PostgreSQL
PostgreSQL supports different sharding techniques, including:
– Partitioning: PostgreSQL provides native support for table partitioning, allowing you to divide a large table into smaller, more manageable pieces called partitions. Each partition can be stored on a separate tablespace or even a different server. Partitioning can be based on a range of values, a list of values, or a hash function.
– Replication: PostgreSQL supports both logical and physical replication, allowing you to replicate data across multiple database instances. Replication can be used to distribute read queries among replica nodes, improving performance and availability. However, it does not automatically distribute write queries.
– Distributed Queries: PostgreSQL’s Foreign Data Wrapper (FDW) functionality enables querying data stored in remote databases as if they were local tables. This allows you to distribute queries across multiple databases and perform joins between tables residing on different shards.
– Connection Pooling: Connection pooling is a technique to manage a pool of database connections that can be reused by multiple clients. PostgreSQL supports various connection pooling solutions that can help manage the connections to the different shards in a sharded database deployment.
Related Article: How to Check if a Table Exists in PostgreSQL
Example: Partitioning a Sales Table
Suppose we have a sales table with millions of records, and we want to partition it based on the sales date. We can create monthly partitions, where each partition contains the sales data for a specific month.
To create the partitions, we can use the native partitioning support in PostgreSQL. Here’s an example of how we can create the partitions and insert data:
-- Create the parent table CREATE TABLE sales ( id SERIAL PRIMARY KEY, product_id INTEGER, sales_date DATE, amount DECIMAL ); -- Create the partitioned table CREATE TABLE sales_partitioned ( CHECK (sales_date >= DATE '2021-01-01' AND sales_date < DATE '2022-01-01') ) INHERITS (sales); -- Create the partitions for each month CREATE TABLE sales_202101 PARTITION OF sales_partitioned FOR VALUES FROM ('2021-01-01') TO ('2021-02-01'); CREATE TABLE sales_202102 PARTITION OF sales_partitioned FOR VALUES FROM ('2021-02-01') TO ('2021-03-01'); -- ... -- Insert data into the partitioned table INSERT INTO sales_partitioned (product_id, sales_date, amount) VALUES (1, '2021-01-15', 100.0), (2, '2021-02-10', 200.0), -- ...
List of PostgreSQL Sharding Features
PostgreSQL provides a range of features that can be leveraged for sharding purposes. These features enable developers and administrators to effectively manage and scale sharded database deployments.
1. Partitioning
Partitioning allows you to divide a large table into smaller, more manageable pieces called partitions. PostgreSQL provides native support for table partitioning, allowing you to create partitions based on a range of values, a list of values, or a hash function.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
Example: Range Partitioning
To illustrate range partitioning, let’s consider a scenario where we have a sensor data table with timestamped readings. We can partition the table based on the timestamp, creating monthly partitions.
CREATE TABLE sensor_data ( id SERIAL PRIMARY KEY, sensor_id INTEGER, reading FLOAT, timestamp TIMESTAMP ) PARTITION BY RANGE (timestamp); CREATE TABLE sensor_data_202101 PARTITION OF sensor_data FOR VALUES FROM ('2021-01-01 00:00:00') TO ('2021-02-01 00:00:00'); CREATE TABLE sensor_data_202102 PARTITION OF sensor_data FOR VALUES FROM ('2021-02-01 00:00:00') TO ('2021-03-01 00:00:00'); -- ...
2. Replication
PostgreSQL supports both logical and physical replication, which can be used to replicate data across multiple database instances. Logical replication allows for selective replication of specific tables or databases, while physical replication replicates the entire database cluster.
Example: Setting up Logical Replication
To set up logical replication, you need to configure a publisher on the source database and a subscriber on the target database. Here’s an example:
-- On the source database CREATE PUBLICATION my_publication FOR TABLE my_table; -- On the target database CREATE SUBSCRIPTION my_subscription CONNECTION 'dbname=my_database host=my_host user=my_user password=my_password' PUBLICATION my_publication WITH (copy_data = false);
Once the replication is set up, any changes made to the publisher’s table will be replicated to the subscriber.
Related Article: How to Convert Columns to Rows in PostgreSQL
3. Distributed Queries
PostgreSQL’s Foreign Data Wrapper (FDW) functionality allows you to query data stored in remote databases as if they were local tables. This enables you to distribute queries across multiple databases and perform joins between tables residing on different shards.
Example: Creating a Foreign Table
To query data from a remote database, you can create a foreign table using the postgres_fdw
extension. Here’s an example:
-- Install the postgres_fdw extension on the local database CREATE EXTENSION postgres_fdw; -- Create a foreign server CREATE SERVER remote_server FOREIGN DATA WRAPPER postgres_fdw OPTIONS (host 'remote_host', dbname 'remote_db', port '5432'); -- Create a user mapping CREATE USER MAPPING FOR current_user SERVER remote_server OPTIONS (user 'remote_user', password 'remote_password'); -- Create a foreign table CREATE FOREIGN TABLE remote_table ( id SERIAL PRIMARY KEY, data TEXT ) SERVER remote_server OPTIONS (table_name 'remote_table');
Once the foreign table is created, you can query it like a regular table in your local database.
4. Connection Pooling
Connection pooling is a technique to manage a pool of database connections that can be reused by multiple clients. PostgreSQL supports various connection pooling solutions, such as pgBouncer and Pgpool-II, which can help manage the connections to the different shards in a sharded database deployment.
Related Article: Detecting and Resolving Deadlocks in PostgreSQL Databases
Example: Configuring pgBouncer
pgBouncer is a lightweight connection pooler for PostgreSQL that can be used to manage connections to the different shards in a sharded database deployment. Here’s an example of how to configure pgBouncer:
1. Install pgBouncer on the server.
2. Create a pgbouncer.ini
configuration file with the following content:
[databases] mydb = host=shard1 port=5432 dbname=mydb mydb = host=shard2 port=5432 dbname=mydb mydb = host=shard3 port=5432 dbname=mydb [pgbouncer] listen_addr = * listen_port = 6432
3. Start pgBouncer using the configuration file:
pgbouncer pgbouncer.ini
Now you can connect to pgBouncer on port 6432 and it will manage the connections to the different shards.
Using PostgreSQL Sharding Features: Partitioning
Partitioning is a powerful feature in PostgreSQL that allows you to divide a large table into smaller, more manageable pieces called partitions. Each partition can be stored on a separate tablespace or even a different server. Partitioning can be based on a range of values, a list of values, or a hash function.
Example: Range Partitioning
To illustrate range partitioning, let’s consider a scenario where we have a sales table with millions of records, and we want to partition it based on the sales date. We can create monthly partitions, where each partition contains the sales data for a specific month.
-- Create the parent table CREATE TABLE sales ( id SERIAL PRIMARY KEY, product_id INTEGER, sales_date DATE, amount DECIMAL ); -- Create the partitioned table CREATE TABLE sales_partitioned ( CHECK (sales_date >= DATE '2021-01-01' AND sales_date < DATE '2022-01-01') ) INHERITS (sales); -- Create the partitions for each month CREATE TABLE sales_202101 PARTITION OF sales_partitioned FOR VALUES FROM ('2021-01-01') TO ('2021-02-01'); CREATE TABLE sales_202102 PARTITION OF sales_partitioned FOR VALUES FROM ('2021-02-01') TO ('2021-03-01'); -- ... -- Insert data into the partitioned table INSERT INTO sales_partitioned (product_id, sales_date, amount) VALUES (1, '2021-01-15', 100.0), (2, '2021-02-10', 200.0), -- ...
Related Article: Executing Efficient Spatial Queries in PostgreSQL
Example: List Partitioning
List partitioning is another partitioning technique available in PostgreSQL. It allows you to create partitions based on a list of predefined values.
-- Create the parent table CREATE TABLE inventory ( id SERIAL PRIMARY KEY, product_id INTEGER, quantity INTEGER ); -- Create the partitioned table CREATE TABLE inventory_partitioned ( CHECK (product_id = ANY (ARRAY[1, 2, 3])) ) INHERITS (inventory); -- Create the partitions for each product ID CREATE TABLE inventory_prod1 PARTITION OF inventory_partitioned FOR VALUES IN (1); CREATE TABLE inventory_prod2 PARTITION OF inventory_partitioned FOR VALUES IN (2); CREATE TABLE inventory_prod3 PARTITION OF inventory_partitioned FOR VALUES IN (3); -- Insert data into the partitioned table INSERT INTO inventory_partitioned (product_id, quantity) VALUES (1, 100), (2, 200), -- ...
In this example, we partition the inventory table based on the product ID. Each partition contains the inventory data for a specific product. By using list partitioning, we can efficiently manage and query the inventory data for different products.
Using PostgreSQL Sharding Features: Replication
Replication is a key feature in PostgreSQL that allows you to replicate data across multiple database instances. This can be used to distribute read queries among replica nodes, improving performance and availability.
Logical Replication
PostgreSQL supports logical replication, which allows for selective replication of specific tables or databases. With logical replication, you can replicate only the necessary data to the replica nodes, reducing network traffic and storage requirements.
Related Article: Preventing Locking Queries in Read-Only PostgreSQL Databases
Example: Setting up Logical Replication
To set up logical replication, you need to configure a publisher on the source database and a subscriber on the target database. Here’s an example:
-- On the source database CREATE PUBLICATION my_publication FOR TABLE my_table; -- On the target database CREATE SUBSCRIPTION my_subscription CONNECTION 'dbname=my_database host=my_host user=my_user password=my_password' PUBLICATION my_publication WITH (copy_data = false);
Once the replication is set up, any changes made to the publisher’s table will be replicated to the subscriber.
Physical Replication
PostgreSQL also supports physical replication, which replicates the entire database cluster to the replica nodes. Physical replication provides an exact copy of the primary database, including all the tables, indexes, and data.
Example: Setting up Physical Replication
To set up physical replication, you need to configure a master-slave setup, where the master database is the primary and the slave database is the replica. Here’s an example:
1. Configure the postgresql.conf
file on the master database:
wal_level = replica max_wal_senders = 5 wal_keep_segments = 32
2. Configure the recovery.conf
file on the slave database:
standby_mode = on primary_conninfo = 'host=master_host port=5432 user=replica_user password=replica_password'
3. Start the slave database with the pg_ctl
command:
pg_ctl start -D /path/to/slave_data_directory
Now, any changes made to the master database will be replicated to the slave database, keeping them in sync.
Related Article: Passing Query Results to a SQL Function in PostgreSQL
Using PostgreSQL Sharding Features: Distributed Queries
PostgreSQL’s Foreign Data Wrapper (FDW) functionality enables querying data stored in remote databases as if they were local tables. This allows you to distribute queries across multiple databases and perform joins between tables residing on different shards.
Example: Creating a Foreign Table
To query data from a remote database, you can create a foreign table using the postgres_fdw
extension. Here’s an example:
-- Install the postgres_fdw extension on the local database CREATE EXTENSION postgres_fdw; -- Create a foreign server CREATE SERVER remote_server FOREIGN DATA WRAPPER postgres_fdw OPTIONS (host 'remote_host', dbname 'remote_db', port '5432'); -- Create a user mapping CREATE USER MAPPING FOR current_user SERVER remote_server OPTIONS (user 'remote_user', password 'remote_password'); -- Create a foreign table CREATE FOREIGN TABLE remote_table ( id SERIAL PRIMARY KEY, data TEXT ) SERVER remote_server OPTIONS (table_name 'remote_table');
Once the foreign table is created, you can query it like a regular table in your local database. For example:
SELECT * FROM remote_table;
This query will retrieve data from the remote table as if it were stored locally.
Example: Joining Tables from Different Shards
With distributed queries, you can also perform joins between tables residing on different shards. Here’s an example:
-- Create the local table CREATE TABLE local_table ( id SERIAL PRIMARY KEY, data TEXT ); -- Create the foreign table CREATE FOREIGN TABLE foreign_table ( id SERIAL PRIMARY KEY, data TEXT ) SERVER remote_server OPTIONS (table_name 'remote_table'); -- Perform a join between the local and foreign tables SELECT local_table.data, foreign_table.data FROM local_table JOIN foreign_table ON local_table.id = foreign_table.id;
This query will join data from the local table and the foreign table, which resides on a remote shard. The result will include data from both tables, as if they were stored on the same shard.
Related Article: Resolving Access Issues with Query Pg Node in PostgreSQL
Using PostgreSQL Sharding Features: Connection Pooling
Connection pooling is a technique used to manage a pool of database connections that can be reused by multiple clients. PostgreSQL supports various connection pooling solutions, such as pgBouncer and Pgpool-II, which can help manage the connections to the different shards in a sharded database deployment.
Example: Configuring pgBouncer
pgBouncer is a lightweight connection pooler for PostgreSQL that can be used to manage connections to the different shards in a sharded database deployment. Here’s an example of how to configure pgBouncer:
1. Install pgBouncer on the server.
2. Create a pgbouncer.ini
configuration file with the following content:
[databases] mydb = host=shard1 port=5432 dbname=mydb mydb = host=shard2 port=5432 dbname=mydb mydb = host=shard3 port=5432 dbname=mydb [pgbouncer] listen_addr = * listen_port = 6432
3. Start pgBouncer using the configuration file:
pgbouncer pgbouncer.ini
Now you can connect to pgBouncer on port 6432, and it will manage the connections to the different shards. Clients can request connections from pgBouncer and reuse them, reducing the overhead of establishing new connections for each query.
Third-Party Tools for Sharding in PostgreSQL
In addition to the built-in sharding features in PostgreSQL, there are several third-party tools available that can further simplify and enhance the sharding process. These tools offer additional functionality and flexibility when it comes to managing and scaling sharded database deployments.
Related Article: Does PostgreSQL Have a Maximum SQL Query Length?
Citus
Citus is an extension for PostgreSQL that provides transparent sharding and distributed query capabilities. It allows you to scale out PostgreSQL across multiple nodes by distributing the data and queries across the shards. Citus provides a SQL interface, making it easy to work with sharded data using familiar PostgreSQL syntax.
Citus also includes features such as automatic data distribution, parallel query execution, and query routing. It simplifies the process of sharding by automatically distributing data and scaling out queries, resulting in improved performance and scalability.
Postgres-XL
Postgres-XL is another PostgreSQL extension that provides sharding and distributed database capabilities. It is designed to scale out PostgreSQL across multiple nodes by partitioning the data and parallelizing query execution. Postgres-XL supports both table-based and hash-based sharding methods.
With Postgres-XL, you can distribute your data across multiple shards and execute queries in parallel across the shards, resulting in improved performance and scalability. It also provides features such as distributed transactions, global indexes, and distributed joins.
Pros and Cons of Sharding in PostgreSQL
Sharding in PostgreSQL offers several advantages, but it also comes with its own set of challenges and trade-offs. Understanding the pros and cons of sharding can help you make informed decisions when considering sharding as a solution for your database scaling needs.
Related Article: Tutorial: Dealing with Non-Existent Relations in PostgreSQL
Pros of Sharding
– Improved Performance: Sharding allows for distributing data and queries across multiple nodes, leading to improved query response times and overall system performance.
– Scalability: By dividing the data into smaller shards, sharding enables horizontal scaling, allowing you to handle larger data volumes and higher traffic loads.
– Availability: Sharding can improve availability by reducing the impact of hardware failures on the overall system. If one shard becomes unavailable, the other shards can continue to serve requests.
– Flexibility: Sharding provides the flexibility to allocate resources based on specific requirements. Each shard can be hosted on a separate server, allowing for efficient resource utilization.
Cons of Sharding
– Increased Complexity: Sharding introduces additional complexity to the database architecture and application code. It requires careful planning and implementation to ensure data consistency and maintainability.
– Data Distribution Challenges: Distributing data across shards can be challenging, especially when dealing with data that needs to be shared or joined across shards. Careful consideration is required to design efficient data distribution strategies.
– Query Coordination: Coordinating queries across multiple shards can be complex, especially for distributed queries that involve joins and aggregations. Special attention needs to be given to query planning and optimization.
– Data Consistency: Ensuring data consistency across shards can be challenging, especially for distributed transactions that span multiple shards. Careful design and implementation are required to maintain data integrity.
Use Cases for Sharding
Sharding is a technique that can be applied to various use cases where there is a need to scale the database horizontally and handle large amounts of data or high traffic loads. Some common use cases for sharding in PostgreSQL include:
– High-Volume Transactional Systems: Sharding can be used to distribute the load of high-volume transactional systems, such as e-commerce platforms or social media applications, where millions of transactions are processed daily.
– Analytics and Reporting: Sharding can be beneficial for analytical workloads that involve processing large volumes of data. By distributing the data across multiple shards, queries can be parallelized, and the overall system performance can be improved.
– Geographically Distributed Applications: Sharding can be useful for applications that need to serve users across different geographical regions. By partitioning the data based on the user’s location, queries can be routed to the appropriate shard, reducing latency and improving performance.
– Multi-Tenant Applications: Sharding can be applied to multi-tenant applications where each tenant’s data is stored in a separate shard. This approach allows for efficient resource utilization and isolation between tenants.
Related Article: How to Use PostgreSQL SELECT INTO TEMP Table
Best Practices for Sharding in PostgreSQL
When implementing sharding in PostgreSQL, it is important to follow best practices to ensure the success and maintainability of your sharded database deployment. Here are some best practices to consider:
– Plan for Growth: Consider the future growth of your database and design your sharding strategy accordingly. Choose a sharding key that allows for balanced data distribution and scalability.
– Maintain Data Consistency: Ensure data consistency across shards by carefully designing data distribution strategies and implementing mechanisms for distributed transactions and data synchronization.
– Monitor and Tune Performance: Regularly monitor the performance of your sharded database and tune it as needed. Pay attention to query execution plans, indexes, and resource utilization to optimize performance.
– Backup and Recovery: Implement a robust backup and recovery strategy for your sharded database. Consider the impact of failures on individual shards and design appropriate backup and recovery procedures.
– Test and Validate: Thoroughly test your sharded database deployment to ensure its reliability and performance. Use realistic workloads and simulate various failure scenarios to validate the behavior of your system.
Real World Examples of Sharding in PostgreSQL
Sharding is widely adopted by organizations to scale their PostgreSQL databases and handle large volumes of data. Here are some real-world examples of companies that have successfully implemented sharding in PostgreSQL:
1. Uber
Uber, the global ride-sharing platform, relies on sharding to handle the massive amount of data generated by its operations. Uber’s PostgreSQL-based database is sharded based on the city and region, allowing for efficient data distribution and scalability. Sharding enables Uber to process millions of ride requests and driver updates in real-time, providing a seamless experience to its users.
Related Article: Exploring Natural Join in PostgreSQL Databases
2. Instagram
Instagram, the popular photo-sharing platform, utilizes sharding to manage its vast amount of user-generated content. By sharding the database based on user IDs, Instagram ensures efficient data distribution and scalability. Sharding allows Instagram to handle billions of photos and videos, enabling users to seamlessly upload, share, and explore content.
3. Airbnb
Airbnb, the online marketplace for vacation rentals, employs sharding to handle the massive volume of booking and accommodation data. By sharding the database based on geographical regions, Airbnb ensures efficient data distribution and scalability. Sharding allows Airbnb to serve millions of users worldwide, providing real-time availability and booking information.
These examples highlight the effectiveness of sharding in PostgreSQL for handling large-scale applications and managing high volumes of data.
Performance Considerations for Sharding in PostgreSQL
While sharding offers scalability and performance benefits, there are certain considerations to keep in mind when it comes to performance in a sharded PostgreSQL database deployment.
Related Article: Tutorial: Inserting Multiple Rows in PostgreSQL
Query Routing Overhead
In a sharded database, queries need to be routed to the appropriate shard based on the sharding key. This routing overhead can introduce latency, especially for queries that involve joins or aggregations across multiple shards. Careful query planning and optimization are required to minimize the impact of query routing overhead.
Data Distribution and Skew
Efficient data distribution is crucial for optimal performance in a sharded database. Uneven data distribution or data skew can lead to performance issues, as some shards may become hotspots with higher query loads. Monitoring and rebalancing the data distribution can help alleviate data skew and ensure balanced query execution across all shards.
Indexing Strategies
Choosing the right indexing strategy is important for efficient query execution in a sharded database. Indexes need to be carefully designed to support the sharding key and the common query patterns. Consideration should be given to the trade-off between query performance and the overhead of maintaining indexes across multiple shards.
Related Article: How to Extract Data from PostgreSQL Databases: PSQL ETL
Query Optimization
Query optimization becomes more complex in a sharded database, as queries may involve multiple shards and distributed data. Understanding the query execution plans and optimizing queries for distributed execution can significantly improve performance. Techniques such as query rewriting, parallel execution, and intelligent query routing can be employed to optimize query performance.
Advanced Sharding Techniques for PostgreSQL
In addition to the basic sharding techniques discussed earlier, there are advanced sharding techniques that can further enhance the scalability and performance of PostgreSQL in a sharded database deployment.
Hash Sharding
Hash sharding is a technique where the sharding key is hashed to determine the shard where the data should be stored. This approach ensures an even distribution of data across shards and eliminates the need for range or list-based partitioning. Hash sharding can simplify the sharding process and provide a more balanced distribution of data.
Consistent Hashing
Consistent hashing is a technique used to distribute data across shards in a way that minimizes the need for data movement when adding or removing shards. It provides a way to map the data to shards in a distributed and scalable manner. Consistent hashing is particularly useful in dynamic environments where the number of shards can change frequently.
Code Snippet Ideas: Sharding in PostgreSQL – Part 1
Here are some code snippet ideas to help you get started with sharding in PostgreSQL:
1. Creating a Range-Partitioned Table
CREATE TABLE sensor_data ( id SERIAL PRIMARY KEY, sensor_id INTEGER, reading FLOAT, timestamp TIMESTAMP ) PARTITION BY RANGE (timestamp); CREATE TABLE sensor_data_202101 PARTITION OF sensor_data FOR VALUES FROM ('2021-01-01 00:00:00') TO ('2021-02-01 00:00:00'); CREATE TABLE sensor_data_202102 PARTITION OF sensor_data FOR VALUES FROM ('2021-02-01 00:00:00') TO ('2021-03-01 00:00:00'); -- ...
This code snippet demonstrates how to create a range-partitioned table in PostgreSQL. The sensor_data
table is partitioned based on the timestamp
column, with separate partitions for each month.
2. Setting up Logical Replication
-- On the source database CREATE PUBLICATION my_publication FOR TABLE my_table; -- On the target database CREATE SUBSCRIPTION my_subscription CONNECTION 'dbname=my_database host=my_host user=my_user password=my_password' PUBLICATION my_publication WITH (copy_data = false);
This code snippet shows how to set up logical replication in PostgreSQL. The my_publication
publication is created on the source database, and the my_subscription
subscription is created on the target database, specifying the connection details.
Code Snippet Ideas: Sharding in PostgreSQL – Part 2
Here are some more code snippet ideas to help you with sharding in PostgreSQL:
1. Creating a Foreign Table
-- Install the postgres_fdw extension on the local database CREATE EXTENSION postgres_fdw; -- Create a foreign server CREATE SERVER remote_server FOREIGN DATA WRAPPER postgres_fdw OPTIONS (host 'remote_host', dbname 'remote_db', port '5432'); -- Create a user mapping CREATE USER MAPPING FOR current_user SERVER remote_server OPTIONS (user 'remote_user', password 'remote_password'); -- Create a foreign table CREATE FOREIGN TABLE remote_table ( id SERIAL PRIMARY KEY, data TEXT ) SERVER remote_server OPTIONS (table_name 'remote_table');
This code snippet demonstrates how to create a foreign table in PostgreSQL using the postgres_fdw
extension. The foreign table remote_table
is created, which represents a table in a remote database accessed through the remote_server
foreign server.
2. Configuring pgBouncer
[databases] mydb = host=shard1 port=5432 dbname=mydb mydb = host=shard2 port=5432 dbname=mydb mydb = host=shard3 port=5432 dbname=mydb [pgbouncer] listen_addr = * listen_port = 6432
This code snippet shows an example configuration file for pgBouncer, a connection pooler for PostgreSQL. The file specifies the databases to connect to and the listening address and port for pgBouncer.
Code Snippet Ideas: Sharding in PostgreSQL – Part 3
Here are some more code snippet ideas to help you with sharding in PostgreSQL:
1. Creating a Hash-Partitioned Table
CREATE TABLE sensor_data ( id SERIAL PRIMARY KEY, sensor_id INTEGER, reading FLOAT, timestamp TIMESTAMP ) PARTITION BY HASH (sensor_id); CREATE TABLE sensor_data_1 PARTITION OF sensor_data FOR VALUES WITH (MODULUS 4, REMAINDER 0); CREATE TABLE sensor_data_2 PARTITION OF sensor_data FOR VALUES WITH (MODULUS 4, REMAINDER 1); -- ...
This code snippet demonstrates how to create a hash-partitioned table in PostgreSQL. The sensor_data
table is partitioned based on the sensor_id
column using the HASH
method, with separate partitions for each modulus and remainder combination.
2. Using Consistent Hashing for Sharding
import hashlib def shard_key(key): md5_hash = hashlib.md5(key.encode('utf-8')).hexdigest() return int(md5_hash, 16) % 1024 def get_shard(key): shard_key = shard_key(key) # Determine the shard based on the shard key # ... # Example usage shard = get_shard("user123")
This code snippet demonstrates how to use consistent hashing for sharding in PostgreSQL. The shard_key
function calculates a consistent hash based on a given key, and the get_shard
function determines the shard based on the shard key.
Code Snippet Ideas: Sharding in PostgreSQL – Part 4
Here are some more code snippet ideas to help you with sharding in PostgreSQL:
1. Creating a Custom Sharding Function
CREATE OR REPLACE FUNCTION custom_sharding_function(key INTEGER) RETURNS INTEGER AS $$ BEGIN -- Custom sharding logic based on the key -- ... RETURN shard_id; END; $$ LANGUAGE plpgsql;
This code snippet demonstrates how to create a custom sharding function in PostgreSQL. The custom_sharding_function
takes a key as input and returns the shard ID based on the custom sharding logic.
2. Routing Queries to Specific Shards
CREATE OR REPLACE FUNCTION route_query_to_shard(query TEXT, shard_id INTEGER) RETURNS TABLE AS $$ BEGIN CASE shard_id WHEN 1 THEN RETURN QUERY EXECUTE format('SELECT * FROM shard1.%s', query); WHEN 2 THEN RETURN QUERY EXECUTE format('SELECT * FROM shard2.%s', query); -- ... END CASE; END; $$ LANGUAGE plpgsql;
This code snippet demonstrates how to route queries to specific shards in PostgreSQL. The route_query_to_shard
function takes a query and shard ID as input, and dynamically executes the query on the appropriate shard based on the shard ID.
Code Snippet Ideas: Sharding in PostgreSQL – Part 5
Here are some more code snippet ideas to help you with sharding in PostgreSQL:
1. Handling Errors in Sharded Databases
import psycopg2 def execute_query(query): try: # Execute the query on the appropriate shard # ... return result except psycopg2.Error as e: # Handle the error # ... # Example usage result = execute_query("SELECT * FROM my_table")
This code snippet demonstrates how to handle errors in sharded databases using psycopg2, a PostgreSQL adapter for Python. The execute_query
function executes a query on the appropriate shard and handles any errors that may occur.
2. Retry Logic for Sharded Databases
import psycopg2 import time def execute_query_with_retry(query, num_retries=3, retry_delay=1): for i in range(num_retries): try: # Execute the query on the appropriate shard # ... return result except psycopg2.Error as e: # Handle the error # ... time.sleep(retry_delay) # Example usage result = execute_query_with_retry("SELECT * FROM my_table")
This code snippet demonstrates how to implement retry logic for sharded databases using psycopg2 and Python. The execute_query_with_retry
function attempts to execute a query on the appropriate shard and retries a specified number of times with a delay between retries.