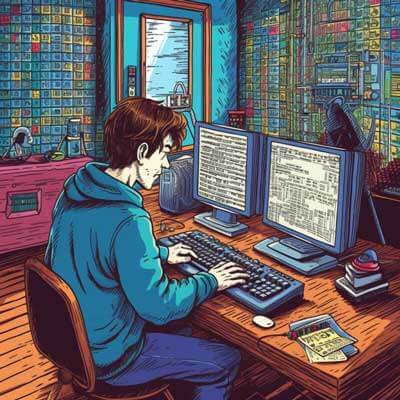
Table of Contents
When working with Docker, there may be times when you need to force a clean build of an image, ensuring that no cached layers are used. This can be useful in situations where you want to make sure that all dependencies and code changes are properly reflected in the final image. In this guide, we will explore several methods to achieve a clean build of a Docker image.
Method 1: Using the --no-cache Flag
One way to force Docker for a clean build is by using the --no-cache flag when running the docker build
command. This flag tells Docker to ignore any cached layers and rebuild the entire image from scratch. Here is an example command:
docker build --no-cache -t myapp:latest .
In the above command, the --no-cache
flag is specified to ensure a clean build. The -t
flag tags the resulting image with the name myapp
and the tag latest
. The .
at the end of the command specifies that the build context is the current directory.
Using the --no-cache
flag is a simple and straightforward way to force Docker for a clean build. However, keep in mind that this method can be time-consuming, especially if you have a large number of dependencies or if your Dockerfile has multiple layers.
Related Article: Quick Docker Cheat Sheet: Simplify Containerization
Method 2: Invalidating Cache for a Specific Build Stage
If your Dockerfile contains multiple build stages and you only want to invalidate the cache for a specific stage, you can use the --build-arg
flag to pass a build argument that changes between builds. This will cause Docker to consider the cache as invalid for that specific stage and rebuild it from scratch.
Here's an example to illustrate this method:
Dockerfile:
FROM python:3.9 AS builder WORKDIR /app COPY requirements.txt . RUN pip install --no-cache-dir -r requirements.txt COPY . . # Build stage 1 RUN echo "Building stage 1" # Build stage 2 ARG CACHE_INVALIDATOR RUN echo "Building stage 2 with CACHE_INVALIDATOR=${CACHE_INVALIDATOR}"
Build command:
docker build --build-arg CACHE_INVALIDATOR=$(date +%s) -t myapp:latest .
In the above example, the Dockerfile has two build stages. The first stage installs the necessary Python dependencies, while the second stage prints a message indicating the current build stage and the value of the CACHE_INVALIDATOR
build argument.
Using this method allows you to selectively invalidate the cache for specific build stages, reducing the overall build time while still achieving a clean build for the desired stages.
Method 3: Modifying File Contents to Invalidate Cache
Another approach to force Docker for a clean build is by modifying the contents of a file that is used in the build process. By changing the file's content, you can ensure that Docker considers it as changed and rebuilds any subsequent layers that depend on it.
Here's an example to illustrate this method:
Dockerfile:
FROM node:14 AS builder WORKDIR /app COPY package.json . RUN npm install COPY . . # Build stage 1 RUN echo "Building stage 1" # Build stage 2 RUN echo "Building stage 2"
Build command:
docker build -t myapp:latest . # Modify a file used in the build process echo "Modified content" >> package.json # Build again to force Docker for a clean build docker build -t myapp:latest .
In the above example, the Dockerfile has two build stages. The first stage installs the necessary Node.js dependencies, while the second stage prints a message indicating the current build stage.
After building the image for the first time, we modify the package.json
file by appending some content. This change ensures that Docker considers the file as modified, and any subsequent layers that depend on it will be rebuilt.
Best Practices
When forcing Docker for a clean build, it's important to consider the following best practices:
1. Use caching wisely: Docker caching can greatly improve build times, especially when working with large projects or dependencies. However, it's important to strike a balance between utilizing the cache and ensuring that changes are properly reflected in the resulting image.
2. Be mindful of the build context: The build context in Docker is the set of files and directories that are sent to the Docker daemon for building the image. Avoid including unnecessary files or directories in the build context, as it can slow down the build process.
3. Leverage multi-stage builds: Docker's multi-stage build feature allows you to create smaller and more optimized images by separating the build environment from the runtime environment. By utilizing multi-stage builds, you can reduce the complexity of your Dockerfile and improve overall build performance.
4. Automate build processes: To ensure consistency and reproducibility, consider automating your build processes using tools like Docker Compose or CI/CD pipelines. This helps in avoiding manual mistakes and ensures that every build starts from a clean state.