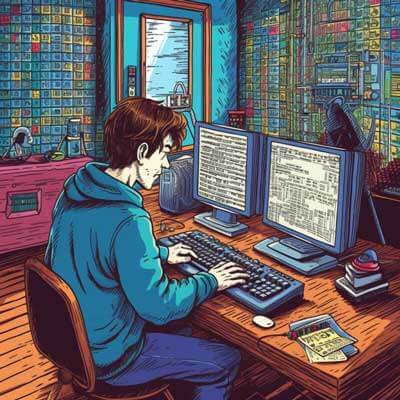
Table of Contents
Introduction to Managing Secrets with Docker
The management of secrets is a critical aspect of application security. Docker provides a robust solution for managing secrets through its built-in feature called Docker Secrets. With Docker Secrets, you can securely store sensitive information such as API keys, database passwords, and SSL certificates, ensuring that they are only accessible to authorized containers.
Docker Secrets provides a secure and scalable way to manage secrets by encrypting them both at rest and in transit. These secrets are only made available to the services or containers that need them, minimizing the risk of exposure.
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
Example: Creating a Docker Secret
To create a Docker secret, you can use the docker secret create
command followed by the name of the secret and the path to the file containing the secret's value. For example:
$ echo "mysecretpassword" | docker secret create db_password -
In this example, we create a secret named db_password
with the value "mysecretpassword".
Example: Using a Docker Secret in a Service
Once you have created a secret, you can use it in your services or containers. To use a secret in a service, you need to specify it in the service's configuration file using either Swarm mode or Compose files.
Here is an example of how you can use a Docker secret in a Compose file:
version: '3' services: app: image: myapp secrets: - db_password secrets: db_password: external: true
In this example, we define a service named app
that uses the myapp
image. The service references the db_password
secret using the secrets
section. The external: true
flag indicates that we are referencing an external secret.
When to Use Docker Secrets
Docker Secrets is particularly useful in scenarios where sensitive information needs to be securely provided to containers or services. Here are some common use cases for Docker Secrets:
1. Database Credentials: Storing database usernames and passwords as secrets ensures that only authorized containers can access them, reducing the risk of unauthorized access.
2. API Keys and Tokens: Secrets can be used to store API keys and tokens required for authentication with external services, preventing accidental exposure in logs or code repositories.
3. SSL Certificates: By storing SSL certificates as secrets, you can ensure that they are only accessible to the containers that require them for secure communication.
Related Article: How to Force Docker for a Clean Build of an Image
Example: Using Docker Secrets with a MySQL Service
Let's consider an example of how Docker Secrets can be used with a MySQL service. We'll create a stack using Docker Compose that includes a MySQL service with its credentials stored as secrets.
Here is an example of a docker-compose.yml
file:
version: '3' services: db: image: mysql environment: MYSQL_ROOT_PASSWORD_FILE: /run/secrets/db_root_password MYSQL_DATABASE: mydb secrets: - db_root_password secrets: db_root_password: file: ./db_root_password.txt
In this example, we define a service named db
using the MySQL image. The root password for the MySQL server is specified using the MYSQL_ROOT_PASSWORD_FILE
environment variable, which references the db_root_password
secret. The value of the secret is stored in a file named db_root_password.txt
.
Basic Usage of Docker Secrets
Using Docker Secrets involves creating secrets and managing their lifecycle within your application stack. Let's explore some basic usage scenarios and commands related to Docker Secrets.
Creating a Docker Secret
To create a Docker secret, you can use the docker secret create
command followed by the name of the secret and the value. The value can be provided through a file or via standard input.
Here is an example of creating a secret using a file:
$ echo "mysecretpassword" | docker secret create db_password -
In this example, we create a secret named db_password
with the value "mysecretpassword". The -
after the secret name indicates that the value is provided through standard input.
Listing Docker Secrets
You can list all the secrets in your Docker environment using the docker secret ls
command. This command displays information such as the name and creation date of each secret.
$ docker secret ls
In this example, all available secrets will be listed.
Related Article: Quick Docker Cheat Sheet: Simplify Containerization
List of Docker Secret Features in Detail
Docker Secrets provides several features that enhance its usability and security. Let's explore some of these features in detail:
External Secrets
External secrets allow you to reference secrets stored outside your Docker Swarm or Compose files. This feature is useful when you want to reuse existing secrets managed by external tools or services.
To use an external secret, you need to define it in your service configuration and set external: true
.
Here is an example of using an external secret in a Compose file:
version: '3' services: app: image: myapp secrets: - db_password secrets: db_password: external: true
In this example, we define an external secret named db_password
that is managed externally.
Secret Rotation
Secret rotation involves updating or changing secrets periodically to maintain security. Docker Secrets supports secret rotation by allowing you to update the value of a secret without affecting the running services.
To update a secret, you can use the docker secret update
command followed by the name of the secret and the new value.
$ echo "newsecretpassword" | docker secret update db_password -
In this example, we update the db_password
secret with a new value.
Best Practices for Managing Docker Secrets
When working with Docker Secrets, it is essential to follow best practices to ensure secure and efficient management. Here are some best practices for managing Docker Secrets:
1. Limit Access: Only grant access to secrets to containers or services that require them. Avoid giving unnecessary access to secrets.
2. Encrypt Communication: Ensure that communication between Swarm nodes and services is encrypted using TLS encryption.
3. Regularly Rotate Secrets: Rotate secrets periodically to minimize the risk of compromise. Use tools or scripts to automate this process.
4. Monitor Secret Usage: Regularly audit and monitor how secrets are being used within your application stack. This helps identify any unauthorized access attempts or unusual behavior.
Related Article: How to Use the Host Network in Docker Compose
Example: Using Docker Secrets with Nginx Proxy
Let's consider an example of how Docker Secrets can be used with an Nginx proxy server that requires SSL certificates stored as secrets.
Here is an example of a docker-compose.yml
file:
version: '3' services: proxy: image: nginx ports: - 80:80 - 443:443 volumes: - ./certs:/etc/nginx/certs secrets: - ssl_certificate - ssl_certificate_key secrets: ssl_certificate: file: ./certs/mydomain.crt ssl_certificate_key: file: ./certs/mydomain.key
In this example, we define a service named proxy
using the Nginx image. The SSL certificate and private key are stored as secrets and mounted into the container at /etc/nginx/certs
.
Performance Considerations for Docker Secrets
While Docker Secrets provides a secure way to manage sensitive information, it is important to consider the performance implications when using secrets in your applications.
Secret Caching
Docker Secrets implements secret caching to improve performance. When a service references a secret, Docker caches its value on the node where the service runs. This reduces the need for frequent communication with the Swarm manager.
Optimizing Secret Usage
To optimize performance when using Docker Secrets, consider the following:
- Minimize Secret Access: Avoid unnecessary access to secrets by limiting access only to services or containers that require them.
- Reduce Secret Size: Keep secrets as small as possible to minimize network traffic and memory usage.
- Cache Secrets Locally: If possible, cache secret values within your application to reduce reliance on frequent lookups.
Related Article: Copying a Directory to Another Using the Docker Add Command
Code Snippet Ideas for Integrating Docker Secrets
Integrating Docker Secrets into your application code requires handling and accessing secrets securely. Here are some code snippets that demonstrate various integration scenarios:
Using Docker SDKs
If you're using Docker SDKs like Docker Engine API or Docker Python SDK, you can retrieve secret values programmatically using their respective APIs.
Here is an example using the Docker Python SDK:
import docker client = docker.from_env() secret = client.secrets.get('db_password') value = secret.attrs['Spec']['Data']
In this example, we use the Docker Python SDK to retrieve the value of a secret named db_password
.
Using Environment Variables
One common approach to using Docker Secrets in your code is by exposing them as environment variables within your containers.
Here is an example of accessing a secret value as an environment variable in Python:
import os db_password = os.environ['DB_PASSWORD']
In this example, the DB_PASSWORD
environment variable contains the value of the db_password
secret.
Use Cases for Docker Secrets
Docker Secrets can be applied to various use cases where sensitive information needs to be securely managed. Let's explore some common use cases:
1. Microservices: In a microservices architecture, each service may require its own set of credentials or tokens. Docker Secrets provide a secure way to manage these secrets per service.
2. Continuous Integration/Continuous Deployment (CI/CD): CI/CD pipelines often require access to sensitive information such as API keys or deployment configurations. By using Docker Secrets, these secrets can be securely stored and accessed by pipeline stages.
3. Distributed Applications: In distributed applications, multiple services may need access to shared secrets like SSL certificates or database credentials. Docker Secrets facilitate secure sharing and management of such secrets across different services.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Example: Using Docker Secrets with a Node.js Application
Let's consider an example of how Docker Secrets can be used with a Node.js application that requires database credentials stored as secrets.
const express = require('express'); const mysql = require('mysql'); const app = express(); const dbPassword = process.env.DB_PASSWORD; const connection = mysql.createConnection({ host: 'localhost', user: 'root', password: dbPassword, database: 'mydb', }); app.get('/', (req, res) => { connection.query('SELECT * FROM users', (err, results) => { if (err) throw err; res.json(results); }); }); app.listen(3000, () => { console.log('Server started on port 3000'); });
In this example, the Node.js application accesses the DB_PASSWORD
environment variable to retrieve the value of the db_password
secret.
Advanced Techniques for Working with Docker Secrets
As you become more experienced with Docker Secrets, you may encounter advanced scenarios that require additional techniques. Here are some advanced techniques for working with Docker Secrets:
Custom Secret Management Tools
While Docker provides built-in support for managing secrets, you may need to integrate with external or custom secret management tools. This allows you to leverage existing infrastructure or implement specialized workflows.
Encrypted Secrets
If you require an extra layer of security for your secrets, you can encrypt them using tools like HashiCorp Vault or AWS Key Management Service (KMS). These tools provide encryption capabilities and access controls for secret management.
Related Article: How to Use Docker Exec for Container Commands
Secrets in Multi-Node Swarm Clusters
When working with multi-node Swarm clusters, consider how secrets are distributed across nodes. You can use external key-value stores like etcd or Consul to store and distribute secrets across multiple Swarm manager nodes.