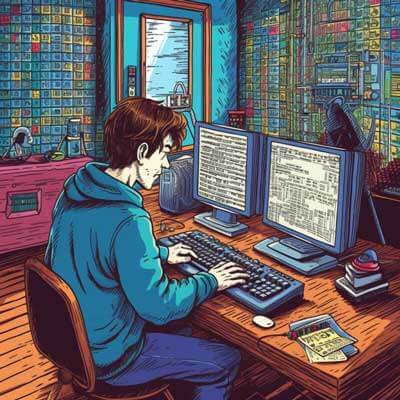
Table of Contents
Introduction to Stored Procedures in MySQL
Stored procedures are a powerful feature of MySQL that allow you to encapsulate SQL code into reusable modules. They provide a way to define and execute a set of SQL statements as a single unit, which can be called multiple times from different parts of your application. Stored procedures offer several benefits such as improved performance, code reusability, and enhanced security.
Related Article: How to Insert Multiple Rows in a MySQL Database
The Syntax of Stored Procedures
The syntax of stored procedures in MySQL follows a specific structure. A stored procedure begins with the keyword "CREATE PROCEDURE" followed by the procedure name and parameter list (if any). Inside the procedure, you can write SQL statements to perform various operations on the database. The syntax for creating a stored procedure is as follows:
CREATE PROCEDURE procedure_name ([parameter_list]) BEGIN -- SQL statements END;
Here is an example of a stored procedure that selects all records from a table called "customers":
CREATE PROCEDURE select_all_customers() BEGIN SELECT * FROM customers; END;
Creating Stored Procedures
To create a stored procedure in MySQL, you can use the "CREATE PROCEDURE" statement followed by the procedure name and the parameter list (if any). Inside the procedure, you can write SQL statements to perform various operations on the database. Here is an example of creating a stored procedure that inserts a new customer record into a table:
CREATE PROCEDURE insert_customer( IN customer_name VARCHAR(255), IN email VARCHAR(255) ) BEGIN INSERT INTO customers (name, email) VALUES (customer_name, email); END;
Executing Stored Procedures
Once a stored procedure is created, you can execute it using the "CALL" statement followed by the procedure name and the parameter values (if any). Here is an example of executing the "select_all_customers" procedure:
CALL select_all_customers();
If the stored procedure has input parameters, you need to pass the values when calling it. For example, to execute the "insert_customer" procedure:
CALL insert_customer('John Doe', 'john@example.com');
Related Article: How to Create a Database from the Command Line Using Psql
Modifying and Deleting Stored Procedures
To modify an existing stored procedure, you can use the "ALTER PROCEDURE" statement followed by the procedure name and the updated code. For example, to add a new SQL statement to the "select_all_customers" procedure:
ALTER PROCEDURE select_all_customers() BEGIN SELECT * FROM customers; SELECT COUNT(*) FROM customers; END;
To delete a stored procedure, you can use the "DROP PROCEDURE" statement followed by the procedure name. For example, to delete the "select_all_customers" procedure:
DROP PROCEDURE select_all_customers;
Use Case: Stored Procedures for Data Validation
Stored procedures are commonly used for data validation in MySQL. They allow you to define rules and constraints for data integrity. For example, you can create a stored procedure that checks if a new customer record meets certain criteria before inserting it into the database. Here is an example of a stored procedure that validates the age of a customer:
CREATE PROCEDURE validate_customer_age( IN customer_age INT ) BEGIN IF customer_age < 18 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Customers must be at least 18 years old.'; END IF; END;
In this example, if the age of the customer is less than 18, an error will be thrown.
Use Case: Stored Procedures for Data Transformation
Stored procedures can also be used for data transformation in MySQL. They allow you to perform complex operations on data and generate new results. For example, you can create a stored procedure that calculates the total revenue for a given period of time. Here is an example of a stored procedure that calculates the total revenue:
CREATE PROCEDURE calculate_total_revenue( IN start_date DATE, IN end_date DATE, OUT total DECIMAL(10,2) ) BEGIN SELECT SUM(price) INTO total FROM orders WHERE order_date BETWEEN start_date AND end_date; END;
In this example, the total revenue for the specified period of time will be calculated and stored in the "total" output parameter.
Use Case: Stored Procedures for Complex Query Execution
Stored procedures can be used to execute complex queries in MySQL. They allow you to encapsulate complex logic into a single unit, making it easier to manage and maintain. For example, you can create a stored procedure that returns the top 10 customers based on their total order amount. Here is an example of a stored procedure that executes a complex query:
CREATE PROCEDURE get_top_customers() BEGIN SELECT customers.name, SUM(orders.amount) AS total_amount FROM customers JOIN orders ON customers.id = orders.customer_id GROUP BY customers.id ORDER BY total_amount DESC LIMIT 10; END;
In this example, the stored procedure returns the names of the top 10 customers along with their total order amount.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Best Practice: Naming Conventions for Stored Procedures
When naming stored procedures in MySQL, it is important to follow a consistent naming convention. This helps improve code readability and maintainability. Here are some best practices for naming stored procedures:
- Use descriptive names that accurately describe the purpose of the stored procedure.
- Prefix the name with a verb to indicate the action performed by the stored procedure (e.g., "get_", "insert_", "update_", "delete_").
- Use underscores to separate words in the name for better readability.
For example, a stored procedure that retrieves customer details could be named "get_customer_details".
Best Practice: Commenting in Stored Procedures
Adding comments to your stored procedures in MySQL is a good practice that helps improve code understandability and maintainability. Comments provide additional information about the purpose and functionality of the stored procedure. Here is an example of adding comments to a stored procedure:
CREATE PROCEDURE get_customer_details() BEGIN -- This stored procedure retrieves customer details from the database. SELECT * FROM customers; END;
In this example, the comment provides a brief description of what the stored procedure does.
Best Practice: Error Handling in Stored Procedures
Error handling is an important aspect of writing robust stored procedures in MySQL. It helps identify and handle errors gracefully, preventing unexpected behavior and ensuring data integrity. MySQL provides several error-handling mechanisms that can be used in stored procedures, such as the "SIGNAL" statement and the "DECLARE" statement. Here is an example of error handling in a stored procedure:
CREATE PROCEDURE delete_customer( IN customer_id INT ) BEGIN DECLARE EXIT HANDLER FOR SQLEXCEPTION BEGIN -- Handle the exception ROLLBACK; SELECT 'An error occurred: ' || SQLSTATE || ' - ' || SQLERRM; END; START TRANSACTION; DELETE FROM customers WHERE id = customer_id; COMMIT; END;
In this example, an exception handler is defined to handle any exceptions that occur during the execution of the stored procedure. If an exception is raised, the transaction is rolled back and an error message is returned.
Real World Example: Implementing User Authentication with Stored Procedures
One common use case for stored procedures in MySQL is implementing user authentication. Stored procedures can be used to securely store and validate user credentials. Here is an example of a stored procedure that checks if a username and password combination is valid:
CREATE PROCEDURE authenticate_user( IN username VARCHAR(255), IN password VARCHAR(255), OUT is_valid INT ) BEGIN SELECT COUNT(*) INTO is_valid FROM users WHERE username = username AND password = password; END;
In this example, the stored procedure checks if the specified username and password combination exists in the "users" table. If a match is found, the "is_valid" output parameter will be set to 1, indicating a valid authentication.
Related Article: How To Use the SQL Select Where For String Matching
Real World Example: Creating a Robust Search Feature with Stored Procedures
Stored procedures can be used to implement a robust search feature in MySQL. They allow you to define complex search queries and handle different search criteria. Here is an example of a stored procedure that searches for customers based on different criteria:
CREATE PROCEDURE search_customers( IN search_term VARCHAR(255) ) BEGIN SELECT * FROM customers WHERE name LIKE CONCAT('%', search_term, '%') OR email LIKE CONCAT('%', search_term, '%'); END;
In this example, the stored procedure searches for customers whose name or email matches the specified search term. The "%" wildcard is used to match any characters before and after the search term.
Performance Consideration: Stored Procedures versus Ad Hoc Queries
When it comes to performance, stored procedures in MySQL offer several advantages over ad hoc queries. Stored procedures are precompiled and stored in the database, which reduces the overhead of parsing and optimizing SQL statements each time they are executed. This can result in improved performance, especially for complex queries that are executed frequently. Additionally, stored procedures can be cached by the database server, further enhancing performance.
Performance Consideration: Stored Procedures and MySQL Query Optimizer
The MySQL query optimizer plays a crucial role in optimizing the execution of stored procedures. It analyzes the SQL statements inside the stored procedure and generates an execution plan that is most efficient for retrieving the desired results. The query optimizer takes into account various factors such as table statistics, indexes, and query complexity to determine the best execution plan. By using stored procedures, you can leverage the query optimizer's capabilities to improve the performance of your database queries.
Advanced Technique: Stored Procedures for Dynamic SQL
Stored procedures in MySQL can be used to dynamically generate and execute SQL statements based on runtime conditions. This advanced technique allows you to build dynamic queries that adapt to different scenarios. Here is an example of a stored procedure that dynamically generates an SQL statement based on the input parameters:
CREATE PROCEDURE get_customers_by_country( IN country VARCHAR(255) ) BEGIN SET @sql = CONCAT('SELECT * FROM customers WHERE country = "', country, '"'); PREPARE stmt FROM @sql; EXECUTE stmt; DEALLOCATE PREPARE stmt; END;
In this example, the stored procedure dynamically builds an SQL statement to retrieve customers from a specific country. The "CONCAT" function is used to concatenate the input parameter with the SQL string, and the "PREPARE" statement is used to prepare the SQL statement for execution.
Related Article: How to Use the WHERE Condition in SQL Joins
Advanced Technique: Using Cursors in Stored Procedures
Cursors are a powerful feature of stored procedures in MySQL that allow you to process query results row by row. They provide a way to iterate over a result set and perform operations on each row individually. Cursors are particularly useful when dealing with large result sets or when complex data manipulation is required. Here is an example of using a cursor in a stored procedure to process customer orders:
CREATE PROCEDURE process_orders() BEGIN DECLARE done INT DEFAULT FALSE; DECLARE customer_id INT; DECLARE order_amount DECIMAL(10,2); DECLARE cur CURSOR FOR SELECT customer_id, amount FROM orders; DECLARE CONTINUE HANDLER FOR NOT FOUND SET done = TRUE; OPEN cur; read_loop: LOOP FETCH cur INTO customer_id, order_amount; IF done THEN LEAVE read_loop; END IF; -- Process the order for the customer -- ... END LOOP; CLOSE cur; END;
In this example, a cursor is declared to select customer orders from the "orders" table. The cursor is then opened, and a loop is used to fetch each row from the cursor. The loop continues until there are no more rows to fetch.
Code Snippet: Basic Stored Procedure
CREATE PROCEDURE select_all_customers() BEGIN SELECT * FROM customers; END;
Code Snippet: Stored Procedure with Input Parameters
CREATE PROCEDURE insert_customer( IN customer_name VARCHAR(255), IN email VARCHAR(255) ) BEGIN INSERT INTO customers (name, email) VALUES (customer_name, email); END;
Code Snippet: Stored Procedure with Output Parameters
CREATE PROCEDURE calculate_total_revenue( IN start_date DATE, IN end_date DATE, OUT total DECIMAL(10,2) ) BEGIN SELECT SUM(price) INTO total FROM orders WHERE order_date BETWEEN start_date AND end_date; END;
Related Article: Joining a View and a Table in SQL: A Tutorial
Code Snippet: Stored Procedure with IF-THEN-ELSE Logic
CREATE PROCEDURE validate_customer_age( IN customer_age INT ) BEGIN IF customer_age < 18 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Customers must be at least 18 years old.'; ELSE SELECT 'Customer age is valid.'; END IF; END;
Code Snippet: Stored Procedure with CURSOR
CREATE PROCEDURE process_orders() BEGIN DECLARE done INT DEFAULT FALSE; DECLARE customer_id INT; DECLARE order_amount DECIMAL(10,2); DECLARE cur CURSOR FOR SELECT customer_id, amount FROM orders; DECLARE CONTINUE HANDLER FOR NOT FOUND SET done = TRUE; OPEN cur; read_loop: LOOP FETCH cur INTO customer_id, order_amount; IF done THEN LEAVE read_loop; END IF; -- Process the order for the customer -- ... END LOOP; CLOSE cur; END;
Error Handling: Dealing with Syntax Errors in Stored Procedures
When writing stored procedures in MySQL, it is common to encounter syntax errors. These errors can be caused by typos, missing or misplaced keywords, or incorrect use of SQL statements. To deal with syntax errors, it is important to carefully review the code and ensure that it follows the correct syntax rules. In case of a syntax error, MySQL will provide an error message that indicates the line and position where the error occurred. By examining the error message and reviewing the code, you can identify and fix syntax errors in your stored procedures.
Error Handling: Handling Runtime Errors in Stored Procedures
Runtime errors can occur during the execution of stored procedures in MySQL. These errors can be caused by various factors such as invalid input parameters, database connection issues, or data integrity violations. To handle runtime errors, you can use error-handling mechanisms provided by MySQL, such as the "DECLARE" statement and the "SIGNAL" statement. By defining exception handlers and specifying how to handle different types of errors, you can ensure that your stored procedures handle runtime errors gracefully and provide meaningful error messages to the user.