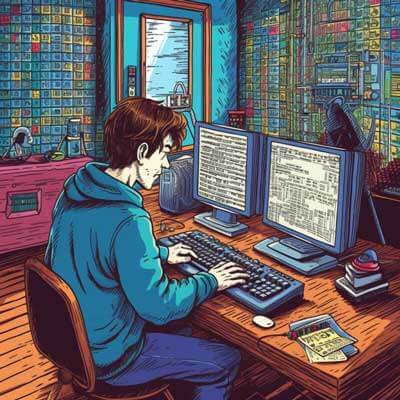
Table of Contents
Introduction to SQL Data Types
SQL data types are used to define the type of data that can be stored in a database table. Each data type has its own characteristics and storage requirements. Understanding SQL data types is essential for designing efficient and reliable databases.
In PostgreSQL, a popular open-source relational database management system, there are various data types available to store different kinds of data. These data types include integers, floating-point numbers, characters, dates, booleans, arrays, JSON, XML, ranges, UUIDs, network addresses, bits, enumerated types, composite types, geometric types, and even spatial data types using the PostGIS extension.
Related Article: Impact of Joins on Missing Data in SQL Databases
List of SQL Data Types in PostgreSQL
PostgreSQL provides a wide range of data types to accommodate different types of data. Here is a list of some commonly used SQL data types in PostgreSQL:
- Integer Data Types: INT, SMALLINT, BIGINT
- Numeric Data Types: NUMERIC, DECIMAL
- Character Data Types: CHAR, VARCHAR, TEXT
- Date and Time Data Types: DATE, TIME, TIMESTAMP
- Boolean Data Type: BOOLEAN
- Array Data Types: ARRAY
- JSON Data Type: JSON
- XML Data Type: XML
- Range Data Types: INT4RANGE, INT8RANGE, NUMRANGE, TSRANGE, TSTZRANGE, DATERANGE
- UUID Data Type: UUID
- Network Address Data Types: INET, CIDR, MACADDR
- Bit Data Types: BIT, BIT VARYING
- Enumerated Data Types: ENUM
- Composite Data Types: ROW
- Geometric Data Types: POINT, LINE, LSEG, BOX, PATH, POLYGON, CIRCLE
- PostGIS Data Types: GEOMETRY, GEOGRAPHY
Working with Integer Data Types
Integer data types in PostgreSQL are used to store whole numbers. Here are some commonly used integer data types:
- INT: This data type is used to store signed integers with a range of -2147483648 to +2147483647.
- SMALLINT: This data type is used to store signed integers with a range of -32768 to +32767.
- BIGINT: This data type is used to store signed integers with a range of -9223372036854775808 to +9223372036854775807.
Example 1: Creating a table with an integer column
CREATE TABLE employees ( employee_id INT, employee_name VARCHAR(100), age INT );
Example 2: Inserting data into the integer column
INSERT INTO employees (employee_id, employee_name, age) VALUES (1, 'John Doe', 30);
Working with Numeric Data Types
Numeric data types in PostgreSQL are used to store numbers with a specified precision and scale. Here are some commonly used numeric data types:
- NUMERIC: This data type is used to store arbitrary precision numbers with a user-specified precision and scale.
- DECIMAL: This data type is used to store fixed precision and scale numbers.
Example 1: Creating a table with a numeric column
CREATE TABLE products ( product_id INT, product_name VARCHAR(100), price NUMERIC(10, 2) );
Example 2: Inserting data into the numeric column
INSERT INTO products (product_id, product_name, price) VALUES (1, 'Widget', 9.99);
Related Article: Tutorial: Working with SQL Data Types in MySQL
Working with Character Data Types
Character data types in PostgreSQL are used to store alphanumeric data. Here are some commonly used character data types:
- CHAR: This data type is used to store fixed-length character strings.
- VARCHAR: This data type is used to store variable-length character strings.
- TEXT: This data type is used to store unlimited length character strings.
Example 1: Creating a table with a character column
CREATE TABLE customers ( customer_id INT, customer_name VARCHAR(100), email TEXT );
Example 2: Inserting data into the character column
INSERT INTO customers (customer_id, customer_name, email) VALUES (1, 'Jane Smith', 'jane@example.com');
Working with Date and Time Data Types
Date and time data types in PostgreSQL are used to store date and time values. Here are some commonly used date and time data types:
- DATE: This data type is used to store dates.
- TIME: This data type is used to store times of day.
- TIMESTAMP: This data type is used to store both date and time values.
Example 1: Creating a table with a date and time column
CREATE TABLE orders ( order_id INT, order_date DATE, order_time TIME, order_timestamp TIMESTAMP );
Example 2: Inserting data into the date and time columns
INSERT INTO orders (order_id, order_date, order_time, order_timestamp) VALUES (1, '2022-01-01', '12:30:00', '2022-01-01 12:30:00');
Working with Boolean Data Type
The boolean data type in PostgreSQL is used to store true or false values. It is commonly used to represent binary choices or conditions. Here is an example of working with the boolean data type:
Example: Creating a table with a boolean column
CREATE TABLE tasks ( task_id INT, task_name VARCHAR(100), is_completed BOOLEAN );
Example: Inserting data into the boolean column
INSERT INTO tasks (task_id, task_name, is_completed) VALUES (1, 'Finish project', true);
Working with Array Data Types
Array data types in PostgreSQL are used to store arrays of values. An array can be one-dimensional or multi-dimensional. Here is an example of working with the array data type:
Example: Creating a table with an array column
CREATE TABLE students ( student_id INT, student_name VARCHAR(100), grades INT[] );
Example: Inserting data into the array column
INSERT INTO students (student_id, student_name, grades) VALUES (1, 'John Doe', '{85, 90, 95}');
Related Article: Tutorial: Using isNumeric Function in PostgreSQL
Working with JSON Data Type
The JSON data type in PostgreSQL is used to store JSON (JavaScript Object Notation) data. JSON is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. Here is an example of working with the JSON data type:
Example: Creating a table with a JSON column
CREATE TABLE employees ( employee_id INT, employee_name VARCHAR(100), employee_info JSON );
Example: Inserting data into the JSON column
INSERT INTO employees (employee_id, employee_name, employee_info) VALUES (1, 'John Doe', '{"age": 30, "department": "IT"}');
Working with XML Data Type
The XML data type in PostgreSQL is used to store XML (Extensible Markup Language) data. XML is a markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable. Here is an example of working with the XML data type:
Example: Creating a table with an XML column
CREATE TABLE books ( book_id INT, book_title VARCHAR(100), book_content XML );
Example: Inserting data into the XML column
INSERT INTO books (book_id, book_title, book_content) VALUES (1, 'Introduction to SQL', '<book><chapter>1</chapter><chapter>2</chapter></book>');
Working with Range Data Types
Range data types in PostgreSQL are used to store ranges of values. There are various range data types available in PostgreSQL, such as INT4RANGE, INT8RANGE, NUMRANGE, TSRANGE, TSTZRANGE, and DATERANGE. Here is an example of working with range data types:
Example: Creating a table with a range column
CREATE TABLE temperature_readings ( reading_id INT, reading_date DATE, temperature INT4RANGE );
Example: Inserting data into the range column
INSERT INTO temperature_readings (reading_id, reading_date, temperature) VALUES (1, '2022-01-01', '[0, 10)');
Working with UUID Data Type
The UUID data type in PostgreSQL is used to store universally unique identifiers (UUIDs). A UUID is a 128-bit number that is unique across all devices and time. Here is an example of working with the UUID data type:
Example: Creating a table with a UUID column
CREATE TABLE users ( user_id UUID, user_name VARCHAR(100) );
Example: Inserting data into the UUID column
INSERT INTO users (user_id, user_name) VALUES ('123e4567-e89b-12d3-a456-426614174000', 'John Doe');
Related Article: Integrating PostgreSQL While Loop into Database Operations
Working with Network Address Data Types
Network address data types in PostgreSQL are used to store IP addresses, MAC addresses, and network addresses. There are three network address data types available in PostgreSQL: INET, CIDR, and MACADDR. Here is an example of working with network address data types:
Example: Creating a table with a network address column
CREATE TABLE devices ( device_id INT, device_name VARCHAR(100), ip_address INET );
Example: Inserting data into the network address column
INSERT INTO devices (device_id, device_name, ip_address) VALUES (1, 'Router', '192.168.0.1');
Working with Bit Data Types
Bit data types in PostgreSQL are used to store fixed-length bit strings or bit arrays. There are two bit data types available in PostgreSQL: BIT and BIT VARYING. Here is an example of working with bit data types:
Example: Creating a table with a bit column
CREATE TABLE permissions ( permission_id INT, permission_bits BIT(8) );
Example: Inserting data into the bit column
INSERT INTO permissions (permission_id, permission_bits) VALUES (1, B'10101010');
Working with Enumerated Data Types
Enumerated data types in PostgreSQL are used to define a list of possible values that a column can take. An enumerated data type is a user-defined data type that consists of a static, ordered set of values. Here is an example of working with enumerated data types:
Example: Creating a table with an enumerated column
CREATE TYPE gender AS ENUM ('Male', 'Female'); CREATE TABLE employees ( employee_id INT, employee_name VARCHAR(100), employee_gender gender );
Example: Inserting data into the enumerated column
INSERT INTO employees (employee_id, employee_name, employee_gender) VALUES (1, 'John Doe', 'Male');
Working with Composite Data Types
Composite data types in PostgreSQL are used to define a custom data type that can contain multiple fields. A composite data type is similar to a struct or record in other programming languages. Here is an example of working with composite data types:
Example: Creating a composite type
CREATE TYPE address AS ( street VARCHAR(100), city VARCHAR(100), state VARCHAR(100), zip VARCHAR(10) ); CREATE TABLE customers ( customer_id INT, customer_name VARCHAR(100), customer_address address );
Example: Inserting data into the composite type column
INSERT INTO customers (customer_id, customer_name, customer_address) VALUES (1, 'John Doe', ROW('123 Main St', 'City', 'State', '12345'));
Related Article: How to Restore a Postgresql Backup File Using the Command Line
Working with Geometric Data Types
Geometric data types in PostgreSQL are used to store geometric shapes such as points, lines, polygons, circles, and more. PostgreSQL provides a rich set of geometric data types and functions for working with spatial data. Here is an example of working with geometric data types:
Example: Creating a table with a geometric column
CREATE TABLE buildings ( building_id INT, building_name VARCHAR(100), building_shape POLYGON );
Example: Inserting data into the geometric column
INSERT INTO buildings (building_id, building_name, building_shape) VALUES (1, 'Office Building', 'POLYGON((0 0, 0 10, 10 10, 10 0, 0 0))');
Working with PostGIS Data Types
PostGIS is an extension for PostgreSQL that adds support for geographic objects, allowing you to store and query spatial data. PostGIS provides a set of data types and functions for working with spatial data. Here is an example of working with PostGIS data types:
Example: Creating a table with a PostGIS column
CREATE TABLE cities ( city_id INT, city_name VARCHAR(100), city_location GEOMETRY(Point, 4326) );
Example: Inserting data into the PostGIS column
INSERT INTO cities (city_id, city_name, city_location) VALUES (1, 'New York', ST_GeomFromText('POINT(-74.0060 40.7128)', 4326));
Using SQL Types in PostgreSQL
To use SQL types in PostgreSQL, you need to specify the appropriate data type when creating tables or columns. SQL types provide a way to enforce data integrity and define the structure of your database. Here are some examples of using SQL types in PostgreSQL:
Example: Creating a table with SQL types
CREATE TABLE employees ( employee_id INT, employee_name VARCHAR(100), hire_date DATE, salary NUMERIC(10, 2) );
Example: Inserting data into a table with SQL types
INSERT INTO employees (employee_id, employee_name, hire_date, salary) VALUES (1, 'John Doe', '2022-01-01', 50000.00);
Example Queries Using Integer Data Types
Example 1: Selecting all employees with an age greater than 30
SELECT * FROM employees WHERE age > 30;
Example 2: Updating the age of an employee
UPDATE employees SET age = 35 WHERE employee_id = 1;
Related Article: Working With PostgreSQL: Extracting Day of Week
Example Queries Using Numeric Data Types
Example 1: Selecting all products with a price greater than $10
SELECT * FROM products WHERE price > 10.00;
Example 2: Calculating the average price of all products
SELECT AVG(price) FROM products;
Example Queries Using Character Data Types
Example 1: Selecting all customers with a name starting with 'J'
SELECT * FROM customers WHERE customer_name LIKE 'J%';
Example 2: Updating the email of a customer
UPDATE customers SET email = 'jane.doe@example.com' WHERE customer_id = 1;
Example Queries Using Date and Time Data Types
Example 1: Selecting all orders placed on a specific date
SELECT * FROM orders WHERE order_date = '2022-01-01';
Example 2: Calculating the total order amount for each day
SELECT order_date, SUM(order_amount) FROM orders GROUP BY order_date;
Example Queries Using Boolean Data Type
Example 1: Selecting all completed tasks
SELECT * FROM tasks WHERE is_completed = true;
Example 2: Updating the status of a task
UPDATE tasks SET is_completed = true WHERE task_id = 1;
Related Article: How to Disable IPv6 in PostgreSQL Databases
Example Queries Using Array Data Types
Example 1: Selecting all students with a grade of 90
SELECT * FROM students WHERE grades @> ARRAY[90];
Example 2: Updating the grades of a student
UPDATE students SET grades = ARRAY[85, 90, 95] WHERE student_id = 1;
Example Queries Using JSON Data Type
Example 1: Selecting all employees in the IT department
SELECT * FROM employees WHERE employee_info ->> 'department' = 'IT';
Example 2: Updating the age of an employee stored in JSON
UPDATE employees SET employee_info = jsonb_set(employee_info, '{age}', '35'::jsonb) WHERE employee_id = 1;
Example Queries Using XML Data Type
Example 1: Selecting all books with a specific chapter
SELECT * FROM books WHERE book_content @> '<book><chapter>1</chapter></book>';
Example 2: Updating the content of a book stored in XML
UPDATE books SET book_content = xmlquery('transform copy $v := $d modify (for $i in $v/book/chapter return replace value of node $i with 2) return $v' passing book_content as "d" returning content) WHERE book_id = 1;
Example Queries Using Range Data Types
Example 1: Selecting all temperature readings within a specific range
SELECT * FROM temperature_readings WHERE temperature @> 5;
Example 2: Updating the temperature range of a reading
UPDATE temperature_readings SET temperature = '[0, 20)' WHERE reading_id = 1;
Related Article: How to Check & Change the DB Directory in PostgreSQL
Example Queries Using UUID Data Type
Example 1: Selecting a user by UUID
SELECT * FROM users WHERE user_id = '123e4567-e89b-12d3-a456-426614174000';
Example 2: Generating a new UUID for a user
UPDATE users SET user_id = uuid_generate_v4() WHERE user_id = '123e4567-e89b-12d3-a456-426614174000';
Example Queries Using Network Address Data Types
Example 1: Selecting all devices with a specific IP address
SELECT * FROM devices WHERE ip_address = '192.168.0.1';
Example 2: Updating the IP address of a device
UPDATE devices SET ip_address = '192.168.0.2' WHERE device_id = 1;
Example Queries Using Bit Data Types
Example 1: Selecting all permissions with a specific bit pattern
SELECT * FROM permissions WHERE permission_bits = B'10101010';
Example 2: Updating the permission bits
UPDATE permissions SET permission_bits = B'01010101' WHERE permission_id = 1;
Example Queries Using Enumerated Data Types
Example 1: Selecting all employees with a specific gender
SELECT * FROM employees WHERE employee_gender = 'Male';
Example 2: Updating the gender of an employee
UPDATE employees SET employee_gender = 'Female' WHERE employee_id = 1;
Related Article: How to Implement Database Sharding in PostgreSQL
Example Queries Using Composite Data Types
Example 1: Selecting all customers with a specific city
SELECT * FROM customers WHERE customer_address.city = 'City';
Example 2: Updating the address of a customer
UPDATE customers SET customer_address = ROW('456 Main St', 'New City', 'State', '54321') WHERE customer_id = 1;
Example Queries Using Geometric Data Types
Example 1: Selecting all buildings within a specific polygon
SELECT * FROM buildings WHERE building_shape @> 'POINT(5 5)';
Example 2: Updating the shape of a building
UPDATE buildings SET building_shape = 'POLYGON((0 0, 0 10, 10 10, 10 0, 0 0))' WHERE building_id = 1;
Example Queries Using PostGIS Data Types
Example 1: Selecting all cities within a specific radius
SELECT * FROM cities WHERE ST_DWithin(city_location, ST_MakePoint(-73.935242, 40.730610)::geography, 10000);
Example 2: Updating the location of a city
UPDATE cities SET city_location = ST_GeomFromText('POINT(-74.0060 40.7128)', 4326) WHERE city_id = 1;
Use Cases for SQL Data Types
SQL data types are used in various use cases to store and manipulate data in a database. Here are some common use cases for SQL data types:
- Storing and querying customer information in an e-commerce application
- Tracking and analyzing financial transactions in a banking system
- Managing employee records in a human resources system
- Storing and querying sensor data in an Internet of Things (IoT) application
- Logging and analyzing user activity in a web analytics system
- Storing and querying spatial data in a geographic information system (GIS)
- Managing inventory and product information in a retail system
- Storing and querying multimedia data such as images and videos
Related Article: How to Update Records in MySQL with a Select Query
Best Practices for Working with SQL Data Types
When working with SQL data types in PostgreSQL, it is important to follow best practices to ensure data integrity and optimize database performance. Here are some best practices for working with SQL data types:
1. Choose the appropriate data type for each column based on the nature of the data it will store.
2. Use constraints and validations to enforce data integrity and prevent invalid data from being inserted into the database.
3. Avoid using generic data types like TEXT when a more specific data type is available.
4. Use appropriate indexing strategies for columns that are frequently used in queries to improve query performance.
5. Normalize your database schema to eliminate data redundancy and improve data consistency.
6. Regularly update statistics on your tables to ensure the query optimizer has up-to-date information for query planning.
7. Use appropriate data type functions and operators to manipulate and query data efficiently.
8. Be mindful of storage requirements for different data types and consider the impact on disk space and performance.
9. Use date and time functions to perform date calculations and manipulations instead of manipulating date strings directly.
10. Regularly monitor and optimize your database configuration to ensure efficient use of system resources.
Real World Examples of SQL Data Types
SQL data types are used in real-world applications to store and manipulate data. Here are some real-world examples of SQL data types:
1. In a social media application, VARCHAR data type is used to store user names and TEXT data type is used to store user posts.
2. In a healthcare system, DATE data type is used to store patient birth dates and NUMERIC data type is used to store medical test results.
3. In a logistics application, INTEGER data type is used to store product quantities and DECIMAL data type is used to store shipping weights.
4. In a customer relationship management (CRM) system, BOOLEAN data type is used to store customer preferences and ENUM data type is used to store lead status.
5. In a geolocation service, POINT data type is used to store latitude and longitude coordinates and GEOMETRY data type is used to store geometric shapes.
6. In a financial application, TIMESTAMP data type is used to store transaction timestamps and MONEY data type is used to store monetary values.
Performance Considerations for SQL Data Types
When designing a database schema and working with SQL data types, it is important to consider performance implications. Here are some performance considerations for SQL data types:
1. Choose the most appropriate data type for each column to minimize storage requirements and improve query performance.
2. Be mindful of the size of data types, especially when dealing with large tables or high-volume data. Smaller data types can reduce storage space and improve I/O performance.
3. Avoid using TEXT or VARCHAR data types for columns that have a fixed length, as it may lead to wasted storage space.
4. Use appropriate indexing strategies for columns that are frequently used in queries to improve query performance.
5. Be cautious when using wide VARCHAR or TEXT columns, as they can impact query performance due to increased disk I/O and memory consumption.
6. Consider using numeric data types with appropriate precision and scale to avoid rounding errors and unnecessary storage space.
7. Regularly update statistics on your tables to ensure the query optimizer has up-to-date information for query planning.
8. Use appropriate data type functions and operators to manipulate and query data efficiently.
9. Be aware of the impact of data type conversions and ensure they are performed efficiently when necessary.
10. Regularly monitor and optimize your database configuration to ensure efficient use of system resources.
Advanced Techniques for SQL Data Types
Working with SQL data types in PostgreSQL opens up opportunities for advanced techniques and optimizations. Here are some advanced techniques for working with SQL data types:
1. Use indexes and partial indexes to optimize queries involving specific data types or patterns.
2. Utilize functional indexes to index the result of a function or expression on a column.
3. Take advantage of the JSONB data type in PostgreSQL for efficient storage and querying of JSON data.
4. Use the hstore extension to store and query key-value pairs efficiently.
5. Explore the capabilities of the PostGIS extension for advanced spatial data analysis and querying.
6. Utilize the full-text search capabilities in PostgreSQL for efficient text searching and indexing.
7. Experiment with user-defined data types to create custom data types tailored to your specific application needs.
8. Use table partitioning to improve query performance and manage large data sets efficiently.
9. Consider using materialized views to precompute and store the results of complex queries for faster retrieval.
10. Use stored procedures and user-defined functions to encapsulate complex data manipulations and calculations.
Related Article: Tutorial: Managing PostgreSQL Databases with Vacuumdb
Code Snippet Ideas for Working with SQL Data Types (1/5)
1. Convert a string to an integer:
SELECT CAST('42' AS INTEGER);
2. Concatenate two strings:
SELECT 'Hello, ' || 'World!';
Code Snippet Ideas for Working with SQL Data Types (2/5)
3. Extract the day from a date:
SELECT EXTRACT(DAY FROM '2022-01-01'::DATE);
4. Calculate the age based on a birth date:
SELECT AGE('1990-01-01'::DATE);
Code Snippet Ideas for Working with SQL Data Types (3/5)
5. Find the maximum value in a column:
SELECT MAX(price) FROM products;
6. Group rows by a specific column and calculate the average:
SELECT category, AVG(price) FROM products GROUP BY category;
Code Snippet Ideas for Working with SQL Data Types (4/5)
7. Find all rows where a column is null:
SELECT * FROM employees WHERE department IS NULL;
8. Order rows by a specific column in descending order:
SELECT * FROM customers ORDER BY last_name DESC;
Related Article: Tutorial: Inserting Multiple Rows in PostgreSQL
Code Snippet Ideas for Working with SQL Data Types (5/5)
9. Join two tables based on a common column:
SELECT * FROM orders JOIN customers ON orders.customer_id = customers.customer_id;
10. Use a subquery to filter rows based on a condition:
SELECT * FROM products WHERE category_id IN (SELECT category_id FROM categories WHERE category_name = 'Electronics');
Error Handling for SQL Data Types
When working with SQL data types, it is important to handle errors that may occur during data manipulation or querying. Here are some common error handling techniques for SQL data types:
1. Use try-catch blocks in your application code to catch and handle database errors.
2. Validate user input to ensure it matches the expected data type before executing queries.
3. Use constraints and validations in your database schema to enforce data integrity and prevent invalid data from being inserted.
4. Handle data conversion errors by checking for null values and using appropriate error handling mechanisms.
5. Use proper error logging and reporting to track and diagnose errors in your database.
6. Provide meaningful error messages to users to help them understand and resolve any issues.
7. Use stored procedures and user-defined functions to encapsulate complex data manipulations and handle errors within the database.
8. Regularly monitor and review database logs for any errors or warnings.
9. Consider using a database connection pool that automatically handles connection errors and retries.
10. Follow best practices for database backup and recovery to minimize the impact of potential errors.