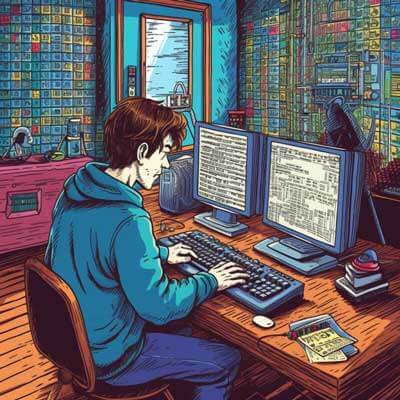
Table of Contents
Introduction
When working with Cassandra, it is important to have efficient methods for timing queries in order to optimize performance and ensure consistent results. In this article, we will explore different techniques and best practices for timing queries in Cassandra.
Related Article: How to Use MySQL Query String Contains
What is Cassandra database and how does it differ from traditional databases?
Cassandra is a highly scalable, distributed NoSQL database designed to handle large amounts of data across multiple commodity servers while providing high availability and fault tolerance. It differs from traditional databases in several ways:
- Data Model: Cassandra uses a flexible schema-less data model, allowing for dynamic and sparse columns, which is different from the rigid, predefined schema of traditional databases.
- Distribution: Cassandra uses a peer-to-peer distributed architecture, where data is spread across multiple nodes in a cluster, providing high availability and fault tolerance. Traditional databases typically use a master-slave architecture.
- Scalability: Cassandra is designed to scale horizontally by adding more nodes to the cluster, allowing it to handle massive amounts of data and high write and read throughput. Traditional databases often have limitations in terms of scalability.
- Consistency: Cassandra offers tunable consistency, allowing users to choose between strong consistency and eventual consistency based on their requirements. Traditional databases usually provide strong consistency by default.
What are the advantages of using NoSQL databases like Cassandra?
NoSQL databases like Cassandra offer several advantages over traditional relational databases:
- Scalability: NoSQL databases can scale horizontally by distributing data across multiple nodes, allowing them to handle large amounts of data and high traffic loads.
- Flexibility: NoSQL databases have a flexible schema, allowing for easy and dynamic changes to the data model without requiring schema migrations.
- High Availability: NoSQL databases are designed to provide high availability and fault tolerance, ensuring that data is accessible even in the event of node failures.
- Performance: NoSQL databases, including Cassandra, are optimized for high performance, especially for write-heavy workloads.
How can I improve the performance of my queries?
To improve the performance of your queries in Cassandra, consider the following techniques:
1. Use appropriate data modeling: Properly designing your data model is key to achieving good query performance in Cassandra. This involves identifying the primary key, partition key, and clustering columns based on your access patterns.
Example:
CREATE TABLE users ( user_id UUID PRIMARY KEY, name TEXT, age INT, email TEXT );
2. Avoid unnecessary data retrieval: Only retrieve the data you need for a particular query to minimize network overhead and improve performance. Use the SELECT clause to specify the columns you require.
Example:
SELECT name, age FROM users WHERE user_id = ?;
3. Use secondary indexes sparingly: Secondary indexes can impact performance and should be used judiciously. They are best suited for low-cardinality columns.
Example:
CREATE INDEX ON users (age);
4. Batch multiple queries together: If you need to perform multiple queries, consider using batch operations to reduce network round trips and improve performance.
Example:
BEGIN BATCH INSERT INTO users (user_id, name, age, email) VALUES (?, ?, ?, ?); UPDATE users SET age = ? WHERE user_id = ?; APPLY BATCH;
5. Tune consistency levels: Adjust the consistency levels based on your requirements. Using lower consistency levels can improve performance but may result in eventual consistency.
Example:
SELECT name, age FROM users WHERE user_id = ? CONSISTENCY ONE;
Related Article: Tutorial: Full Outer Join versus Join in SQL
What are some best practices for optimizing queries in Cassandra?
To optimize queries in Cassandra, consider the following best practices:
1. Use denormalization: Denormalize your data model to avoid expensive joins and improve query performance. Duplicate data across multiple tables to optimize for different query patterns.
Example:
CREATE TABLE users_by_age ( age INT, user_id UUID, name TEXT, email TEXT, PRIMARY KEY (age, user_id) );
2. Leverage materialized views: Materialized views allow you to create multiple representations of your data, optimized for different query patterns. They automatically maintain the view's data based on the underlying table.
Example:
CREATE MATERIALIZED VIEW users_by_age AS SELECT age, user_id, name, email FROM users WHERE age IS NOT NULL AND user_id IS NOT NULL PRIMARY KEY (age, user_id);
3. Use appropriate data types: Choose the most appropriate data types for your columns to optimize storage and query performance. Use compact data types like UUID or INT when possible.
Example:
CREATE TABLE users ( user_id UUID PRIMARY KEY, name TEXT, age INT, email TEXT );
4. Monitor and optimize cluster performance: Regularly monitor your cluster's performance using tools like nodetool and DataStax OpsCenter. Optimize cluster configuration parameters and hardware resources based on the observed performance metrics.
Example:
nodetool status nodetool tpstats
5. Use compression and compaction: Enable compression to reduce storage requirements and improve read performance. Configure compaction strategies based on your workload and data access patterns.
Example:
compression: sstable_compression: LZ4Compressor compaction: class: SizeTieredCompactionStrategy
How should I approach data modeling in Cassandra?
When approaching data modeling in Cassandra, consider the following guidelines:
1. Identify your query patterns: Understand the different types of queries you need to support and their access patterns. This will help you design your data model accordingly.
2. Normalize or denormalize: Normalize your data model for transactional consistency or denormalize it for query performance. Choose the approach that best suits your application's requirements.
3. Think in terms of queries, not tables: Design your data model based on the queries you need to perform, rather than trying to fit your data into predefined tables.
4. Use composite primary keys: Utilize composite primary keys to model relationships and optimize query performance. This involves combining multiple columns to form a unique identifier for each row.
Example:
CREATE TABLE user_followers ( user_id UUID, follower_id UUID, PRIMARY KEY (user_id, follower_id) );
5. Avoid hotspots: Distribute your data evenly across partitions to avoid hotspots, which can lead to performance bottlenecks. Use techniques like token-aware routing and random partitioner to achieve even data distribution.
What is the role of distributed systems in Cassandra?
Cassandra is designed as a distributed system, where data is distributed across multiple nodes in a cluster. The role of distributed systems in Cassandra includes:
1. Scalability: By distributing data across multiple nodes, Cassandra can handle large amounts of data and high traffic loads. Adding more nodes to the cluster allows for horizontal scalability.
2. Fault tolerance: Distributed systems like Cassandra are designed to be fault-tolerant. Data is replicated across multiple nodes, ensuring that it remains accessible even in the event of node failures.
3. High availability: Cassandra provides high availability by allowing multiple replicas of data across different nodes. This ensures that data can be accessed even if some nodes are temporarily unavailable.
4. Consistency: Distributed systems like Cassandra provide tunable consistency, allowing users to choose between strong consistency and eventual consistency based on their requirements.
What is the CAP theorem and how does it relate to Cassandra?
The CAP theorem, also known as Brewer's theorem, states that in a distributed system, it is impossible to simultaneously guarantee consistency (C), availability (A), and partition tolerance (P). Cassandra is designed to provide high availability and partition tolerance, sacrificing a certain level of consistency.
Cassandra achieves high availability and partition tolerance by using a distributed architecture, where data is replicated across multiple nodes. It employs a highly scalable and decentralized peer-to-peer model, allowing it to handle large amounts of data and traffic.
While Cassandra provides tunable consistency, allowing users to choose between strong consistency and eventual consistency, it inherently leans towards eventual consistency to ensure high availability and fault tolerance.
Related Article: How to Create a Database from the Command Line Using Psql
How does Cassandra ensure consistency in a distributed environment?
Cassandra ensures consistency in a distributed environment through its replication strategy, consistency levels, and conflict resolution mechanisms.
1. Replication strategy: Cassandra uses a configurable replication strategy to determine how data is replicated across nodes in a cluster. Replicas are placed on different nodes to ensure fault tolerance and high availability.
Example:
replication: class: NetworkTopologyStrategy datacenter1: 3 datacenter2: 2
2. Consistency levels: Cassandra provides tunable consistency levels, allowing users to define the level of consistency required for their read and write operations. Consistency levels range from ONE (weakest) to ALL (strongest).
Example:
SELECT name, age FROM users WHERE user_id = ? CONSISTENCY ONE;
3. Conflict resolution: In situations where inconsistent data may be written to different replicas due to network partitions or other issues, Cassandra employs a last-write-wins conflict resolution mechanism. The most recent write is considered the correct version of the data.
What is partitioning in Cassandra and why is it important?
Partitioning in Cassandra refers to the process of distributing data across multiple nodes in a cluster based on the partition key. Each partition is responsible for storing a subset of the data. Partitioning is important in Cassandra for several reasons:
1. Scalability: Partitioning allows Cassandra to scale horizontally by distributing data across multiple nodes. Each node is responsible for a subset of the data, enabling Cassandra to handle large amounts of data and high traffic loads.
2. Load balancing: Partitioning ensures even data distribution across nodes, preventing hotspots and balancing the load across the cluster. This helps maintain performance and prevents bottlenecks.
3. Fault tolerance: Data replication is based on partitions, with replicas placed on different nodes. In the event of node failures, data can be retrieved from other replicas, ensuring fault tolerance and high availability.
4. Performance: Partitioning enables parallel processing of queries across multiple nodes, improving read and write performance. Each node only needs to process the data within its assigned partition.
How does replication work in Cassandra and why is it necessary?
Replication in Cassandra is the process of creating and maintaining multiple copies of data across different nodes in a cluster. Replication is necessary for several reasons:
1. Fault tolerance: By replicating data across multiple nodes, Cassandra ensures that data remains accessible even in the event of node failures. If a node goes down, data can be retrieved from other replicas.
2. High availability: Replication allows for multiple replicas of data, ensuring that data can be accessed even if some nodes are temporarily unavailable. This provides high availability and reduces downtime.
3. Data durability: Replication ensures data durability by storing multiple copies of data across different nodes. This protects against data loss in the event of hardware failures or other disasters.
4. Consistency: Replication plays a role in maintaining consistency in a distributed environment. Cassandra allows users to define the consistency level for their read and write operations, ensuring that data consistency requirements are met.
Example replication configuration:
replication: class: NetworkTopologyStrategy datacenter1: 3 datacenter2: 2
In the above example, the replication strategy is set to NetworkTopologyStrategy, and the data is replicated across different data centers. Each data center has a specified replication factor, indicating the number of replicas to maintain.
Additional Resources
- Difference between NoSQL and SQL databases