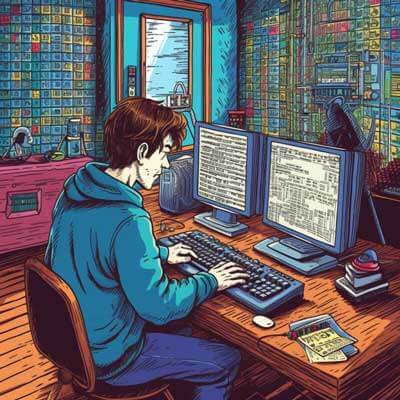
Table of Contents
In PostgreSQL, a while loop is a control structure that allows you to repeatedly execute a block of code as long as a specified condition is true. The condition is evaluated before each iteration, and if it evaluates to true, the loop body is executed. Once the condition becomes false, the loop terminates, and the program execution continues with the next statement after the loop.
While loops are commonly used in programming to iterate over a set of values or perform a specific task until a certain condition is met. They provide a flexible and useful way to automate repetitive tasks and process data efficiently.
What are the advantages of using a while loop in PostgreSQL?
Using a while loop in PostgreSQL offers several advantages:
1. Flexibility: While loops allow you to perform complex operations and manipulate data based on dynamic conditions. This flexibility makes them suitable for a wide range of use cases, such as data transformation, data validation, and batch processing.
2. Efficiency: By iterating over a set of values or records, while loops can process large amounts of data efficiently. They enable you to perform operations on each item in the set without the need for manual iteration.
3. Automation: While loops automate repetitive tasks by executing a block of code multiple times. This reduces the need for manual intervention and improves productivity.
4. Control: While loops give you fine-grained control over the execution flow. You can specify the condition that determines when the loop should terminate, allowing you to control the logic and behavior of your program.
Related Article: Tutorial: ON for JOIN SQL in Databases
How do I use a while loop in PostgreSQL?
To use a while loop in PostgreSQL, you can use the LOOP statement, which is followed by the loop body enclosed in a block. Within the loop body, you can include any valid SQL statements or procedural code.
Here's the basic syntax of a while loop in PostgreSQL:
LOOP -- loop body -- code statements -- conditional statements -- control flow statements EXIT WHEN condition; END LOOP;
In this syntax, the loop body consists of the code statements that you want to execute repeatedly. The condition is a Boolean expression that determines whether the loop should continue or terminate. You can use any valid SQL or procedural code to define the condition.
Here's an example that demonstrates the usage of a while loop in PostgreSQL:
DECLARE counter INT := 1; BEGIN LOOP RAISE NOTICE 'Counter: %', counter; counter := counter + 1; EXIT WHEN counter > 5; END LOOP; END;
In this example, the while loop iterates from 1 to 5 and prints the value of the counter variable using the RAISE NOTICE statement. The loop terminates when the counter exceeds 5.
Code Snippet: Using a while loop to iterate through a table in PostgreSQL
DECLARE record table_name%ROWTYPE; BEGIN FOR record IN SELECT * FROM table_name LOOP -- loop body -- code statements -- access record fields END LOOP; END;
In this code snippet, a FOR loop is used to iterate through all the rows in the table table_name
. The record
variable is declared as the same data type as the table's row type using the %ROWTYPE
attribute. Within the loop body, you can access the fields of each record using dot notation.
Example: Using a while loop to update records in PostgreSQL
DECLARE record table_name%ROWTYPE; BEGIN FOR record IN SELECT * FROM table_name LOOP -- loop body -- code statements UPDATE table_name SET column1 = new_value WHERE current_of record; END LOOP; END;
In this example, a FOR loop is used to iterate through all the rows in the table table_name
. Within the loop body, an UPDATE statement is executed to update the value of column1
for the current row using the current_of
clause.
Related Article: How to Create a Database from the Command Line Using Psql
Best Practices for using while loops in PostgreSQL
When using while loops in PostgreSQL, it is important to follow these best practices to ensure efficient and correct execution:
1. Use caution with infinite loops: While loops can potentially result in infinite loops if the loop termination condition is not properly defined. Make sure to define a condition that will eventually become false to avoid infinite loops.
2. Minimize database round trips: While loops that involve database operations should be designed to minimize the number of round trips to the database. This can be achieved by batching operations or using bulk operations where possible.
3. Optimize loop performance: If you are working with large datasets, consider optimizing the loop performance by using appropriate indexes, limiting the number of rows returned, and avoiding unnecessary calculations or operations within the loop body.
4. Use transactions when necessary: If your while loop involves multiple database operations that need to be executed atomically, consider using transactions to ensure data consistency and integrity.
5. Test and validate loop logic: Before deploying your while loop in a production environment, thoroughly test and validate the loop logic to ensure that it behaves as expected and handles all possible scenarios.
Troubleshooting common issues with while loops in PostgreSQL
While loops can sometimes introduce issues or errors in your PostgreSQL code. Here are some common troubleshooting tips for while loops:
1. Verify loop termination condition: Make sure that the loop termination condition is correctly defined and will eventually evaluate to false. Check for any logical errors or missing conditions that may cause the loop to run indefinitely.
2. Check loop body logic: Review the code statements within the loop body to ensure that they are correct and handle all possible scenarios. Check for any potential errors, such as invalid SQL syntax or incorrect variable assignments.
3. Debug loop variables: If you are experiencing unexpected behavior or errors within the loop, consider adding debug statements or printing loop variables to help identify the issue. This can help you pinpoint any incorrect values or unexpected behaviors.
4. Monitor resource usage: While loops that process large datasets or perform complex operations can consume significant system resources. Monitor the resource usage, such as CPU and memory, to ensure that the loop does not cause performance issues or resource exhaustion.
5. Seek community support: If you encounter persistent issues or errors with while loops in PostgreSQL, consider seeking help from the PostgreSQL community. Forums, mailing lists, and online communities are great resources for getting assistance and advice from experienced PostgreSQL users and developers.